Installing PostgreSQL on CentOS: A Friendly Guide Hey there, fellow tech enthusiasts! Ever wanted to dive into the world of databases and manage your data like a pro? Well, you’ve come to the right place! Today, we’re going to walk through the process of installing PostgreSQL on CentOS. PostgreSQL is a powerful, open-source relational database management system (RDBMS) known for […]
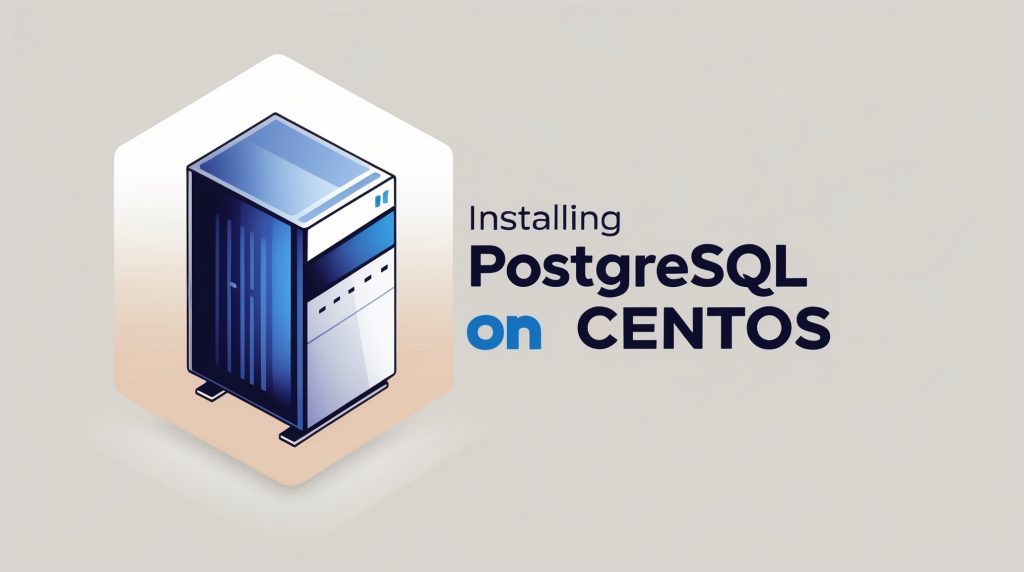