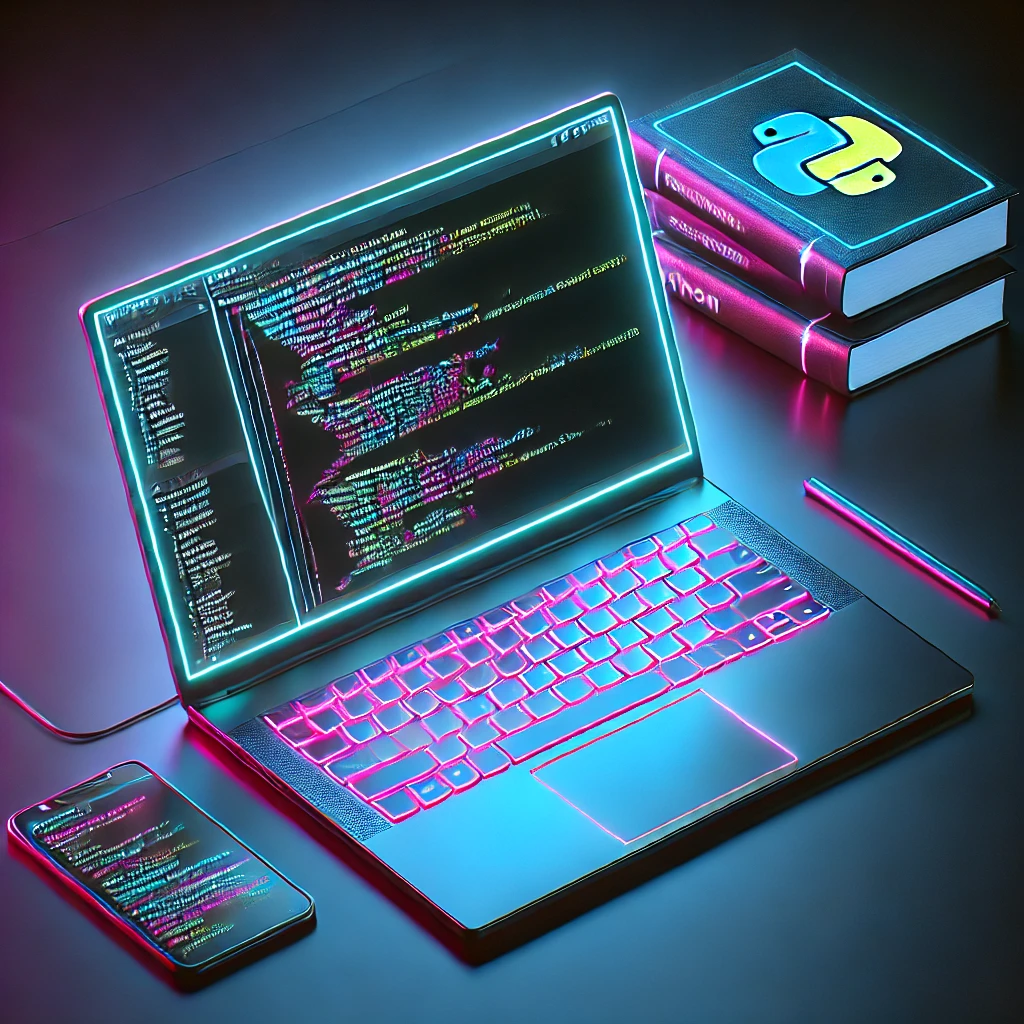
5 Awesome AI Projects You Can Build with Python Libraries
Artificial Intelligence (AI) is revolutionizing the tech industry, and Python is at the forefront of this movement. With its simplicity and a plethora of libraries, Python is the go-to language for AI enthusiasts. Whether you’re a college student or a young professional looking to enhance your skills, here are five amazing AI projects you can build using Python libraries. These projects not only boost your resume but also deepen your understanding of AI.
1. Chatbot with Natural Language Processing
Chatbots are everywhere, from customer service to personal assistants. Building a chatbot is an excellent way to dive into Natural Language Processing (NLP) and understand how AI interacts with human language.
Setting Up Your Environment
First, install the necessary libraries:
pip install nltk
pip install chatterbot
pip install chatterbot_corpus
Creating a Basic Chatbot
Here’s a simple example using the ChatterBot
library:
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
# Create a new chatbot instance
chatbot = ChatBot('MyChatBot')
# Train the chatbot with English corpus data
trainer = ChatterBotCorpusTrainer(chatbot)
trainer.train('chatterbot.corpus.english')
# Get a response to a user input
response = chatbot.get_response('Hello, how are you today?')
print(response)
Enhancing Your Chatbot with NLTK
The Natural Language Toolkit (NLTK) library is powerful for processing and analyzing human language data. You can enhance your chatbot by adding more advanced NLP capabilities:
import nltk
from nltk.chat.util import Chat, reflections
# Define a set of pairs for patterns and responses
pairs = [
[
r"my name is (.*)",
["Hello %1, how are you today ?",]
],
[
r"what is your name ?",
["My name is ChatBot.",]
]
]
# Initialize the chatbot
chat = Chat(pairs, reflections)
# Start the chatbot conversation
def chatbot_conversation():
print("Hi, I'm the chatbot. Type 'quit' to exit.")
while True:
user_input = input(">")
if user_input.lower() == 'quit':
break
response = chat.respond(user_input)
print(response)
chatbot_conversation()
2. Image Recognition with Convolutional Neural Networks
Image recognition is a core task in computer vision, and Convolutional Neural Networks (CNNs) are the backbone of most modern image recognition systems. Using the TensorFlow and Keras libraries, you can build a simple image classifier.
Installing TensorFlow and Keras
pip install tensorflow
pip install keras
Building an Image Classifier
Here’s a step-by-step guide to building a basic image classifier:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Conv2D, Flatten, MaxPooling2D
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Define the model
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Prepare the data
train_datagen = ImageDataGenerator(rescale=1./255)
training_set = train_datagen.flow_from_directory('dataset/training_set', target_size=(64, 64), batch_size=32, class_mode='binary')
# Train the model
model.fit(training_set, epochs=5)
Making Predictions
After training, you can use the model to make predictions on new images:
import numpy as np
from tensorflow.keras.preprocessing import image
# Load the image
test_image = image.load_img('dataset/single_prediction/cat_or_dog_1.jpg', target_size=(64, 64))
test_image = image.img_to_array(test_image)
test_image = np.expand_dims(test_image, axis=0)
# Make a prediction
result = model.predict(test_image)
if result[0][0] == 1:
prediction = 'dog'
else:
prediction = 'cat'
print(prediction)
3. Stock Price Prediction with LSTM Networks
Predicting stock prices is a fascinating AI challenge. Long Short-Term Memory (LSTM) networks are particularly suited for this task due to their ability to capture temporal dependencies in data.
Installing Required Libraries
pip install numpy
pip install pandas
pip install tensorflow
pip install matplotlib
Loading and Preprocessing Data
Let’s start by loading and preprocessing the stock price data:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.preprocessing import MinMaxScaler
# Load the data
data = pd.read_csv('stock_prices.csv')
data = data['Open'].values.reshape(-1, 1)
# Scale the data
scaler = MinMaxScaler(feature_range=(0, 1))
scaled_data = scaler.fit_transform(data)
# Prepare the training data
train_data = scaled_data[0:int(len(data)*0.8)]
x_train = []
y_train = []
for i in range(60, len(train_data)):
x_train.append(train_data[i-60:i, 0])
y_train.append(train_data[i, 0])
x_train, y_train = np.array(x_train), np.array(y_train)
x_train = np.reshape(x_train, (x_train.shape[0], x_train.shape[1], 1))
Building and Training the LSTM Model
Now, let’s build and train the LSTM model:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, LSTM, Dropout
# Define the model
model = Sequential([
LSTM(units=50, return_sequences=True, input_shape=(x_train.shape[1], 1)),
Dropout(0.2),
LSTM(units=50, return_sequences=False),
Dropout(0.2),
Dense(units=1)
])
# Compile the model
model.compile(optimizer='adam', loss='mean_squared_error')
# Train the model
model.fit(x_train, y_train, epochs=25, batch_size=32)
Making Predictions
Finally, you can use the trained model to predict future stock prices:
# Prepare the test data
test_data = scaled_data[int(len(data)*0.8)-60:]
x_test = []
y_test = data[int(len(data)*0.8):]
for i in range(60, len(test_data)):
x_test.append(test_data[i-60:i, 0])
x_test = np.array(x_test)
x_test = np.reshape(x_test, (x_test.shape[0], x_test.shape[1], 1))
# Make predictions
predictions = model.predict(x_test)
predictions = scaler.inverse_transform(predictions)
# Plot the results
plt.plot(y_test, color='blue', label='Actual Stock Price')
plt.plot(predictions, color='red', label='Predicted Stock Price')
plt.title('Stock Price Prediction')
plt.xlabel('Time')
plt.ylabel('Stock Price')
plt.legend()
plt.show()
4. Sentiment Analysis with NLP
Sentiment analysis helps determine the sentiment behind a piece of text, whether it’s positive, negative, or neutral. This project uses the NLTK library to perform sentiment analysis on movie reviews.
Installing NLTK
pip install nltk
Loading and Preprocessing Data
First, we need to load and preprocess the data:
import nltk
from nltk.corpus import movie_reviews
from nltk.classify import NaiveBayesClassifier
from nltk.classify.util import accuracy
# Download the movie reviews dataset
nltk.download('movie_reviews')
# Create a list of documents, each containing a list of words and a label
documents = [(list(movie_reviews.words(fileid)), category)
for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
# Shuffle the documents
import random
random.shuffle(documents)
# Extract features from the words
all_words = nltk.FreqDist(word.lower() for word in movie_reviews.words())
word_features = list(all_words.keys())[:2000]
def document_features(document):
document_words = set(document)
features = {}
for word in word_features:
features[word] = (word in document_words)
return features
featuresets = [(document_features(d), c) for (d, c) in documents]
# Split the data into training and testing sets
train_set, test_set = featuresets[100:], featuresets[:100]
Training and Evaluating the Model
Now, let’s train and evaluate the Naive Bayes classifier:
# Train the classifier
classifier = NaiveBayesClassifier.train(train_set)
# Evaluate the classifier
print(f'Accuracy: {accuracy(classifier, test_set) * 100}%')
# Show the most informative features
classifier.show_most_informative_features(10)
Using the Classifier for Sentiment Analysis
You can use the trained classifier to analyze the sentiment of new movie reviews:
def analyze_sentiment(review):
words = review.split()
features = document_features(words)
return classifier.classify(features)
# Test the function
new_review = "This movie was a fantastic journey with amazing characters and a compelling story."
print(analyze_sentiment(new_review))
5. Speech Recognition with Deep Learning
Speech recognition is a fascinating AI application where the computer can understand and process human speech. Using the SpeechRecognition
and TensorFlow
libraries, you can build a basic speech recognition system.
Installing Required Libraries
pip install SpeechRecognition
pip install tensorflow
pip install pyaudio
Building a Speech Recognition Model
Let’s start by recognizing speech from an audio file using the SpeechRecognition
library:
import speech_recognition as sr
# Initialize the recognizer
recognizer = sr.Recognizer()
def recognize_speech_from_file(file_path):
# Load the audio file
audio_file = sr.AudioFile(file_path)
with audio_file as source:
recognizer.adjust_for_ambient_noise(source)
audio = recognizer.record(source)
# Recognize speech using Google Web Speech API
try:
text = recognizer.recognize_google(audio)
print(f"Recognized Speech: {text}")
except sr.RequestError:
print("API request error.")
except sr.UnknownValueError:
print("Unable to recognize speech.")
# Test the function
recognize_speech_from_file('path_to_audio_file.wav')
Training a Deep Learning Model for Speech Recognition
For more advanced speech recognition, you can train a deep learning model using TensorFlow. Here’s how you can preprocess the data and build a model:
import tensorflow as tf
from tensorflow.keras.layers import Dense, LSTM, Dropout
from tensorflow.keras.optimizers import Adam
from sklearn.preprocessing import LabelEncoder
import numpy as np
# Load your dataset (example with synthetic data)
# In practice, you would load a dataset like the Common Voice or LibriSpeech
features = np.random.rand(1000, 50) # Example feature data
labels = np.random.randint(0, 10, 1000) # Example labels
# Encode the labels
label_encoder = LabelEncoder()
labels = label_encoder.fit_transform(labels)
# Split the data into training and testing sets
split = int(0.8 * len(features))
x_train, x_test = features[:split], features[split:]
y_train, y_test = labels[:split], labels[split:]
# Define the model
model = tf.keras.Sequential([
LSTM(128, return_sequences=True, input_shape=(50, 1)),
Dropout(0.2),
LSTM(128, return_sequences=False),
Dropout(0.2),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer=Adam(), loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(x_train, y_train, epochs=20, batch_size=32, validation_data=(x_test, y_test))
# Evaluate the model
loss, accuracy = model.evaluate(x_test, y_test)
print(f"Test Accuracy: {accuracy * 100}%")
Making Predictions
After training, you can use the model to recognize speech from new audio data:
def predict_speech(audio_data):
# Preprocess the audio data
audio_features = extract_features(audio_data) # Assume you have a function for feature extraction
audio_features = np.expand_dims(audio_features, axis=0)
# Make a prediction
prediction = model.predict(audio_features)
predicted_label = np.argmax(prediction, axis=1)
return label_encoder.inverse_transform(predicted_label)
# Test the function with new audio data
new_audio_data = np.random.rand(50) # Example audio data
print(predict_speech(new_audio_data))
Conclusion
Building AI projects with Python is both fun and educational. The five projects outlined above provide a diverse set of applications from chatbots and image recognition to stock price prediction, sentiment analysis, and speech recognition. These projects utilize various Python libraries and frameworks, helping you gain hands-on experience in different AI fields. By working on these projects, you’ll not only enhance your coding skills but also deepen your understanding of how AI can solve real-world problems.
Happy coding! If you have any questions or need further assistance, feel free to reach out. Let’s build some amazing AI projects together!
Disclaimer: The code snippets provided in this blog are for educational purposes. Ensure you have the appropriate datasets and permissions for their usage. Please report any inaccuracies so we can correct them promptly.