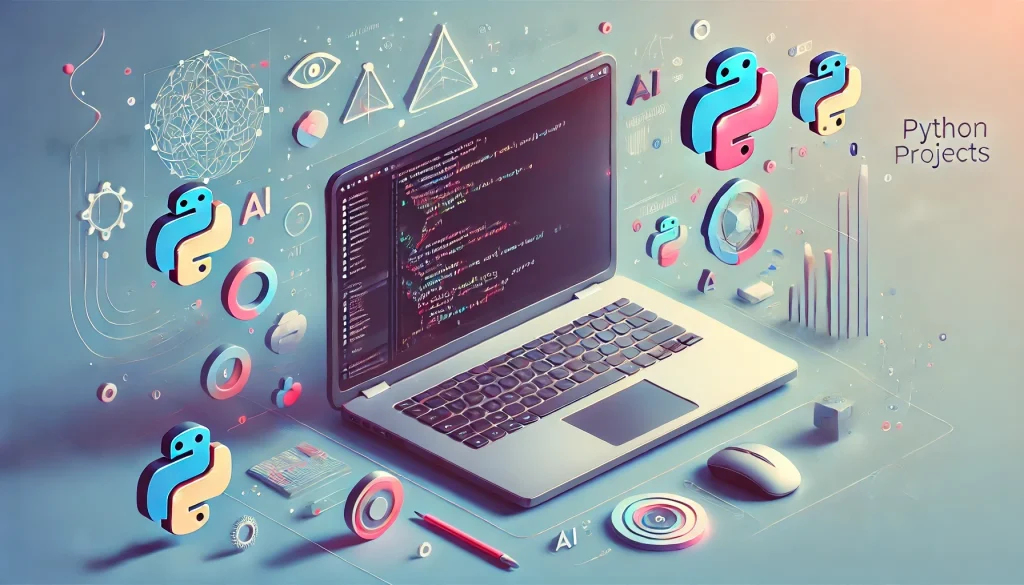
5 Fun Python Projects to Kickstart Your AI Journey
Hey there, future AI expert! Are you excited to dive into the world of Artificial Intelligence (AI) but don’t know where to start? You’re in the right place. Python is your best friend when it comes to learning AI because of its simplicity and extensive libraries. Here are five fun and intriguing Python projects that will help you get started on your AI journey. Let’s make learning AI an adventure!
1. Chatbot with Natural Language Processing (NLP)
Introduction to Chatbots
Creating a chatbot is a fantastic way to get your hands dirty with AI. Chatbots are conversational agents that can interact with users and provide responses based on their inputs. They are used everywhere, from customer service to personal assistants.
What You’ll Learn
- Basics of Natural Language Processing (NLP)
- How to use libraries like NLTK and SpaCy
- Building a simple chatbot
Getting Started
First, you’ll need to install the necessary libraries. Open your terminal and run:
pip install nltk spacy
Next, let’s import the libraries and download the necessary data for NLTK:
import nltk
nltk.download('punkt')
nltk.download('wordnet')
Creating a Basic Chatbot
Here’s a simple example to get you started:
import random
import nltk
from nltk.chat.util import Chat, reflections
pairs = [
['my name is (.*)', ['Hello %1, how can I help you today?']],
['hi|hello|hey', ['Hello!', 'Hi there!', 'Hey!']],
['what is your name?', ['I am a chatbot created to assist you.']],
['how are you?', ['I am doing great, thank you! How about you?']],
['(.*) your favorite color?', ['My favorite color is blue.']],
['bye|goodbye', ['Goodbye! Have a great day!']]
]
chatbot = Chat(pairs, reflections)
def chat():
print("Hi! I am your chatbot. Type something to start a conversation.")
while True:
user_input = input("You: ")
if user_input.lower() in ['bye', 'goodbye']:
print("Bot: Goodbye! Have a great day!")
break
response = chatbot.respond(user_input)
print(f"Bot: {response}")
chat()
This simple script uses predefined patterns and responses to simulate a conversation. As you become more comfortable, you can expand the chatbot’s capabilities by integrating more advanced NLP techniques.
2. Image Recognition with Convolutional Neural Networks (CNNs)
Introduction to Image Recognition
Image recognition is a field of AI that involves teaching machines to recognize and categorize images. This is used in various applications, such as facial recognition, object detection, and more.
What You’ll Learn
- Basics of Convolutional Neural Networks (CNNs)
- How to use TensorFlow and Keras
- Building and training an image classifier
Getting Started
First, install TensorFlow and Keras:
pip install tensorflow keras
Building an Image Classifier
Let’s build a simple image classifier that can recognize handwritten digits using the MNIST dataset.
import tensorflow as tf
from tensorflow.keras import datasets, layers, models
import matplotlib.pyplot as plt
# Load the dataset
(train_images, train_labels), (test_images, test_labels) = datasets.mnist.load_data()
# Preprocess the data
train_images = train_images.reshape((60000, 28, 28, 1)).astype('float32') / 255
test_images = test_images.reshape((10000, 28, 28, 1)).astype('float32') / 255
# Build the model
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=5)
# Evaluate the model
test_loss, test_acc = model.evaluate(test_images, test_labels)
print(f"Test accuracy: {test_acc}")
This code sets up a simple CNN to classify handwritten digits. As you progress, you can explore more complex datasets and model architectures.
3. Sentiment Analysis with Machine Learning
Introduction to Sentiment Analysis
Sentiment analysis involves determining the sentiment or emotion behind a piece of text. This is widely used in social media monitoring, customer feedback analysis, and more.
What You’ll Learn
- Basics of text classification
- Using libraries like Scikit-learn and NLTK
- Building a sentiment analysis model
Getting Started
Install the necessary libraries:
pip install scikit-learn nltk
Building a Sentiment Analysis Model
Here’s a simple example to classify movie reviews as positive or negative.
import nltk
from nltk.corpus import movie_reviews
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
# Download the movie reviews dataset
nltk.download('movie_reviews')
# Load the data
documents = [(list(movie_reviews.words(fileid)), category)
for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
random.shuffle(documents)
# Preprocess the data
all_words = nltk.FreqDist(w.lower() for w in movie_reviews.words())
word_features = list(all_words)[:2000]
def document_features(document):
document_words = set(document)
features = {}
for word in word_features:
features[f'contains({word})'] = (word in document_words)
return features
featuresets = [(document_features(d), c) for (d, c) in documents]
train_set, test_set = train_test_split(featuresets, test_size=0.25, random_state=42)
# Train the model
classifier = nltk.NaiveBayesClassifier.train(train_set)
# Test the model
accuracy = nltk.classify.accuracy(classifier, test_set)
print(f"Model Accuracy: {accuracy * 100:.2f}%")
This example uses the NLTK library to perform sentiment analysis on movie reviews. As you advance, you can use more sophisticated algorithms and larger datasets.
4. Recommender System with Collaborative Filtering
Introduction to Recommender Systems
Recommender systems suggest items to users based on their preferences. They are used in various applications, such as e-commerce, streaming services, and social media.
What You’ll Learn
- Basics of collaborative filtering
- Using the Surprise library
- Building a movie recommendation system
Getting Started
Install the Surprise library:
pip install scikit-surprise
Building a Movie Recommender
Here’s a simple example to recommend movies based on user ratings.
from surprise import Dataset, Reader
from surprise import KNNBasic
from surprise.model_selection import train_test_split
from surprise import accuracy
# Load the dataset
data = Dataset.load_builtin('ml-100k')
trainset, testset = train_test_split(data, test_size=0.25)
# Use KNN algorithm for collaborative filtering
algo = KNNBasic()
algo.fit(trainset)
# Test the model
predictions = algo.test(testset)
accuracy.rmse(predictions)
# Making predictions for a specific user
user_id = str(196)
item_id = str(302)
prediction = algo.predict(user_id, item_id)
print(f"Predicted rating for user {user_id} on item {item_id}: {prediction.est}")
This code uses the Surprise library to build a simple recommender system. As you become more comfortable, you can experiment with different algorithms and datasets.
5. Voice Assistant with Speech Recognition
Introduction to Voice Assistants
Voice assistants like Siri and Alexa can recognize and respond to voice commands. Building a voice assistant is an exciting project that combines various AI techniques.
What You’ll Learn
- Basics of speech recognition
- Using libraries like SpeechRecognition and pyttsx3
- Building a simple voice assistant
Getting Started
Install the necessary libraries:
pip install SpeechRecognition pyttsx3
Building a Voice Assistant
Here’s a simple example to create a voice assistant that can respond to basic commands.
import speech_recognition as sr
import pyttsx3
# Initialize the recognizer and engine
recognizer = sr.Recognizer()
engine = pyttsx3.init()
def speak(text):
engine.say(text)
engine.runAndWait()
def listen():
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
command = recognizer.recognize_google(audio)
print(f"You said: {command}")
return command
except sr.UnknownValueError:
print("Sorry, I didn't catch that.")
return ""
except sr.RequestError:
print("Sorry, my speech service is down.")
return ""
# Main loop
def main():
while True:
command = listen()
if "hello" in command.lower():
speak("Hello! How can I assist you today?")
elif "your name" in command.lower():
speak("I am your voice assistant.")
elif "time" in command.lower():
from datetime import datetime
now = datetime.now().strftime("%H:%M:%S")
speak(f"The current time is {now}.")
elif "stop" in command.lower() or "bye" in command.lower():
speak("Goodbye! Have a great day!")
break
else:
speak("I'm not sure how to respond to that.")
if __name__ == "__main__":
main()
This simple voice assistant recognizes basic commands and responds accordingly. As you progress, you can add more functionalities and integrate other AI techniques to enhance its capabilities.
Conclusion
Embarking on your AI journey with Python is both exciting and rewarding. These five projects—creating a chatbot, building an image recognition system, performing sentiment analysis, developing a recommender system, and making a voice assistant—are great ways to get started. Each project introduces you to essential AI concepts and techniques, setting a solid foundation for more advanced explorations.
Remember, practice is key. Experiment with these projects, tweak the code, and add new features. The more you play around with these projects, the more confident you’ll become in your AI skills.
Happy coding, and may your AI journey be as fun and fulfilling as possible!
If you enjoyed this blog or have any questions, feel free to leave a comment. Don’t forget to share this with your friends who might be interested in learning AI with Python. Stay curious and keep exploring!
Disclaimer: The code provided in this blog is for educational purposes only. While we’ve made efforts to ensure accuracy, AI is a rapidly evolving field, and there might be inaccuracies or outdated information. Report any inaccuracies so we can correct them promptly.