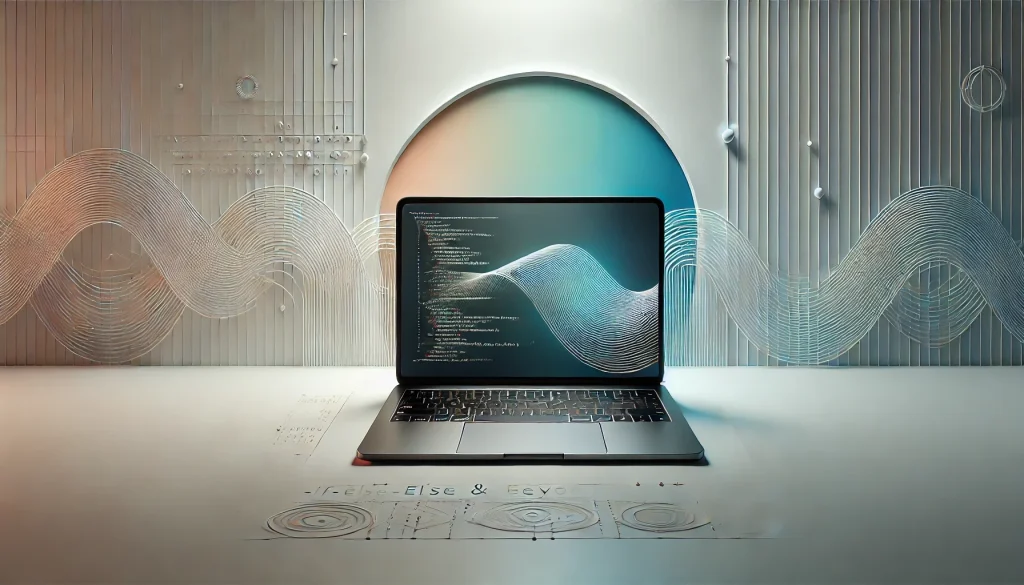
If-Else & Beyond: Mastering Control Flow for Smarter AI
Imagine a world where machines could make decisions as effortlessly as humans do. Well, guess what? We’re not too far from that reality! The key to unlocking this potential lies in mastering control flow in artificial intelligence. But what exactly is control flow, and why is it so crucial for developing smarter AI systems? In this blog post, we’ll dive deep into the world of control structures, exploring everything from the basic if-else statements to more advanced techniques that are shaping the future of AI. Whether you’re a seasoned programmer or just dipping your toes into the vast ocean of artificial intelligence, this guide will help you navigate the complexities of control flow and empower you to create more intelligent, responsive, and efficient AI systems. So, buckle up and get ready for an exciting journey through the maze of decision-making in AI!
The Fundamentals: Understanding Control Flow in Programming
Before we dive into the nitty-gritty of control flow in AI, let’s take a step back and understand what control flow means in the broader context of programming. At its core, control flow refers to the order in which individual statements, instructions, or function calls are executed in a program. It’s the backbone of any software application, determining how a program runs from start to finish. Think of it as the traffic controller of your code, directing which parts should run, when they should run, and under what conditions. Without proper control flow, our programs would be like cars without steering wheels โ moving, but with no real direction or purpose.
The building blocks of control flow
When we talk about control flow, we’re essentially discussing the various structures and statements that allow us to manipulate the execution path of our programs. These building blocks include conditional statements (like if-else), loops (such as for and while), function calls, and exception handling mechanisms. Each of these elements plays a crucial role in shaping how our programs behave and respond to different inputs and conditions. By mastering these fundamental concepts, we lay the groundwork for creating more sophisticated and intelligent AI systems that can adapt and make decisions based on complex scenarios.
Why control flow matters in AI
Now, you might be wondering, “Why is control flow so important in the context of AI?” The answer lies in the very nature of artificial intelligence itself. AI systems are designed to mimic human decision-making processes, and at the heart of decision-making is the ability to evaluate conditions, choose between alternatives, and execute different actions based on those choices. Control flow structures provide the mechanisms for implementing these decision-making capabilities in our AI algorithms. They allow us to create systems that can analyze data, recognize patterns, and make informed decisions โ all critical components of intelligent behavior.
The Classic If-Else: The Foundation of Decision-Making in AI
Let’s start our journey into control flow with the most fundamental decision-making structure: the if-else statement. This simple yet powerful construct forms the backbone of logical reasoning in programming and is particularly crucial in AI applications. At its core, an if-else statement allows a program to execute different code blocks based on whether a specific condition is true or false. It’s like a fork in the road, directing the flow of the program down one path or another depending on the circumstances.
Anatomy of an if-else statement
To understand how if-else statements work in practice, let’s break down their structure. An if-else statement typically consists of three main parts: the condition, the code block to execute if the condition is true, and the code block to execute if the condition is false. In many programming languages, it looks something like this:
if condition:
# Code to execute if the condition is true
else:
# Code to execute if the condition is false
This simple structure allows us to create branching logic in our programs, enabling them to make decisions based on various inputs or states.
Real-world applications in AI
In the context of AI, if-else statements play a crucial role in implementing decision trees, rule-based systems, and other logical inference mechanisms. For example, in a natural language processing application, we might use if-else statements to categorize user input:
if "weather" in user_input:
provide_weather_forecast()
elif "news" in user_input:
show_latest_news()
else:
ask_for_clarification()
This simple example demonstrates how if-else statements can help AI systems interpret and respond to user queries intelligently. By chaining multiple if-else statements or nesting them within each other, we can create increasingly complex decision-making processes that form the basis of many AI algorithms.
Beyond Binary: Introducing Switch-Case and Pattern Matching
While if-else statements are incredibly useful, they can become unwieldy when dealing with multiple conditions or complex decision trees. This is where more advanced control structures like switch-case statements and pattern matching come into play. These constructs allow us to handle multiple conditions more elegantly and efficiently, making our code more readable and maintainable in the process.
The power of switch-case
Switch-case statements provide a cleaner alternative to long chains of if-else statements when we need to compare a single variable against multiple possible values. Instead of writing numerous if-else conditions, we can use a switch statement to list out all the possible cases and their corresponding actions. This is particularly useful in AI systems that need to categorize inputs or choose between multiple predefined actions based on specific conditions.
Here’s a simple example of how a switch-case statement might be used in an AI-powered customer service chatbot:
switch (customer_issue):
case "billing":
redirect_to_billing_department()
case "technical":
initiate_troubleshooting_process()
case "sales":
connect_to_sales_representative()
default:
offer_general_assistance()
This structure makes it easy to add new cases or modify existing ones without cluttering our code with excessive if-else statements.
Pattern matching: A step towards more flexible AI
Taking things a step further, pattern matching is an even more powerful and flexible control flow technique that’s gaining popularity in AI programming. Pattern matching allows us to compare complex data structures against predefined patterns and execute code based on those matches. This is particularly useful in natural language processing, computer vision, and other AI domains where we need to recognize and respond to complex patterns in data.
For example, in a language like Scala (which is often used in AI development), we might use pattern matching to analyze and respond to different types of user input:
userInput match {
case Email(sender, subject, body) => processEmail(sender, subject, body)
case Tweet(username, content) => analyzeTweet(username, content)
case VoiceCommand(command) => executeVoiceCommand(command)
case _ => handleUnknownInput()
}
This approach allows our AI systems to handle a wide variety of input types and structures with elegant, easy-to-read code. By leveraging pattern matching, we can create more flexible and adaptable AI algorithms that can handle complex, real-world scenarios with ease.
Looping for Learning: Iteration in AI Algorithms
Now that we’ve explored decision-making structures, let’s turn our attention to another crucial aspect of control flow in AI: loops. Iteration is a fundamental concept in programming, and it’s especially important in AI algorithms that need to process large amounts of data, train on multiple examples, or refine their outputs through repeated cycles. Loops allow our AI systems to perform repetitive tasks efficiently, learn from experience, and improve their performance over time.
The workhorse of AI: The for loop
The for loop is perhaps the most commonly used iteration structure in AI programming. It’s particularly useful when we know in advance how many times we want to repeat a certain operation. In machine learning algorithms, for loops are often used to iterate over training data, update model parameters, or perform cross-validation. Here’s a simple example of how a for loop might be used in a basic neural network training process:
for epoch in range(num_epochs):
for batch in training_data:
predictions = model.forward(batch)
loss = calculate_loss(predictions, batch.targets)
model.backward(loss)
model.update_parameters()
if epoch % evaluation_interval == 0:
evaluate_model(model, validation_data)
This nested for loop structure allows us to iterate over multiple epochs of training, processing each batch of data and updating our model accordingly. It’s a simple yet powerful way to implement the iterative learning process that’s at the heart of many AI algorithms.
While loops: For when we don’t know how long it’ll take
While for loops are great when we know the number of iterations in advance, sometimes in AI we need to continue a process until a certain condition is met, regardless of how many iterations it takes. This is where while loops come in handy. While loops continue executing a block of code as long as a specified condition remains true. They’re particularly useful in optimization algorithms, reinforcement learning, and other AI techniques where we’re trying to achieve a specific goal or level of performance.
For example, in a gradient descent algorithm, we might use a while loop to continue optimizing until we reach a desired level of accuracy:
while current_error > target_error:
update_parameters()
current_error = calculate_error(model, data)
if iterations > max_iterations:
break
This structure allows our AI algorithm to keep refining its performance until it reaches the desired level of accuracy, or until we hit a predefined maximum number of iterations.
Recursion: The Art of Self-Reference in AI
As we delve deeper into advanced control flow techniques, we encounter one of the most powerful and mind-bending concepts in programming: recursion. Recursion occurs when a function calls itself as part of its execution. This self-referential nature makes recursion an incredibly powerful tool for solving complex problems, especially in AI where we often deal with hierarchical structures or problems that can be broken down into smaller, similar subproblems.
The recursive mindset
To understand recursion, we need to shift our thinking from linear, step-by-step processes to a more holistic, divide-and-conquer approach. The key to effective recursion is identifying the base case (the simplest version of the problem that can be solved directly) and the recursive case (where the problem is broken down into a smaller version of itself). This approach allows us to solve complex problems by continually breaking them down until we reach a solvable base case.
Recursion in AI: From search algorithms to natural language processing
In AI, recursion finds applications in various domains. One classic example is in search algorithms, such as depth-first search, which is often used in game-playing AIs or pathfinding algorithms. Here’s a simple implementation of a recursive depth-first search:
def depth_first_search(node):
if is_goal(node):
return node
for child in get_children(node):
result = depth_first_search(child)
if result is not None:
return result
return None
This recursive function keeps exploring deeper into the search space until it either finds the goal or exhausts all possibilities. The beauty of this approach lies in its simplicity and elegance โ complex tree or graph structures can be traversed with just a few lines of code.
Another area where recursion shines in AI is natural language processing, particularly in parsing and understanding the structure of sentences. Recursive neural networks, for instance, use the principle of recursion to process hierarchical structures in language. These networks can build up an understanding of a sentence by recursively combining the meanings of its constituent parts, much like how humans understand language by breaking it down into phrases and clauses.
Exception Handling: Graceful Error Management in AI Systems
As we continue our journey through control flow in AI, we can’t overlook the importance of handling unexpected situations and errors. In the real world, AI systems often have to deal with unpredictable inputs, network failures, resource constraints, and a host of other potential issues. This is where exception handling comes into play, providing a structured way to detect, respond to, and recover from errors in our AI algorithms.
The try-except pattern: Catching and handling errors
The most common approach to exception handling is the try-except pattern. This structure allows us to “try” to execute a block of code that might raise an exception, and then “except” or catch any errors that occur, handling them gracefully. Here’s a basic example of how this might look in a Python-based AI system:
try:
result = complex_ai_operation(input_data)
process_result(result)
except ValueError as e:
log_error("Invalid input data", e)
request_new_input()
except ResourceExhaustedError as e:
log_error("Out of computational resources", e)
scale_down_operation()
except Exception as e:
log_error("Unexpected error occurred", e)
fallback_to_safe_state()
This structure allows our AI system to handle various types of errors differently, logging the issue, taking appropriate corrective action, and potentially retrying the operation or falling back to a safe state.
Balancing robustness and performance
While exception handling is crucial for building robust AI systems, it’s important to strike a balance between error handling and performance. Excessive use of try-except blocks can slow down our algorithms, especially in performance-critical sections of code. As AI developers, we need to carefully consider where exception handling is most necessary and beneficial, focusing on critical operations and external interactions where errors are more likely to occur.
Asynchronous Programming: Unleashing Parallelism in AI
In the world of AI, we often deal with complex, computationally intensive tasks that can benefit greatly from parallelism and asynchronous execution. Asynchronous programming allows our AI systems to perform multiple operations concurrently, making better use of available resources and potentially speeding up processing times significantly. This is particularly important in scenarios like real-time data processing, distributed machine learning, or handling multiple AI agents simultaneously.
Coroutines and async/await: The building blocks of asynchronous AI
Many modern programming languages provide built-in support for asynchronous programming through coroutines and the async/await syntax. These constructs allow us to write asynchronous code that looks and behaves much like synchronous code, making it easier to reason about and maintain. Here’s an example of how we might use async/await in a Python-based AI system that processes multiple data streams concurrently:
async def process_data_stream(stream_id):
while True:
data = await get_next_data_chunk(stream_id)
if data is None:
break
result = await ai_model.process(data)
await store_result(stream_id, result)
async def main():
streams = [process_data_stream(i) for i in range(num_streams)]
await asyncio.gather(*streams)
This asynchronous approach allows our AI system to efficiently handle multiple data streams in parallel, switching between them as needed without blocking on I/O operations.
Promises and futures: Managing asynchronous computations
In addition to async/await, many AI systems leverage promises or futures to manage asynchronous computations. These constructs represent the result of an asynchronous operation that may not have completed yet. They allow us to chain operations, handle errors, and coordinate multiple asynchronous tasks. Here’s a conceptual example of how promises might be used in a distributed AI system:
function trainModelShard(shard) {
return new Promise((resolve, reject) => {
// Asynchronous training logic here
});
}
Promise.all(dataShardskeys().map(trainModelShard))
.then(results => combineModelShards(results))
.then(finalModel => deployModel(finalModel))
.catch(error => handleTrainingError(error));
This approach allows us to train multiple shards of a large AI model in parallel, combine the results, and deploy the final model, all while handling potential errors gracefully.
Functional Programming: Embracing Immutability and Pure Functions in AI
As we push the boundaries of AI development, many developers are turning to functional programming paradigms to create more predictable, testable, and scalable AI systems. Functional programming emphasizes immutability (data that cannot be changed after it’s created) and pure functions (functions that always produce the same output for a given input, without side effects). These principles can lead to AI algorithms that are easier to reason about, debug, and parallelize.
Immutability: Safeguarding against unexpected changes
Immutability is a powerful concept in AI development, especially when dealing with large, complex data structures or distributed systems. By ensuring that data cannot be modified once created, we can avoid a whole class of bugs related to unexpected state changes. This is particularly valuable in machine learning pipelines, where maintaining the integrity of training data and model parameters is crucial. Here’s a simple example of how we might use immutable data structures in a Python-based AI system:
from immutables import Map
def update_model_parameters(current_params, gradients):
return current_params.update({
'weights': current_params['weights'] + gradients['weights'],
'biases': current_params['biases'] + gradients['biases']
})
initial_params = Map({'weights': np.zeros((100, 100)), 'biases': np.zeros(100)})
new_params = update_model_parameters(initial_params, gradients)
In this example, we use an immutable Map to store our model parameters. The update_model_parameters
function doesn’t modify the original parameters but instead returns a new Map with updated values. This approach ensures that we always have a clear history of parameter changes and eliminates the risk of accidental modifications.
Pure functions: The building blocks of predictable AI
Pure functions are another key concept in functional programming that can greatly benefit AI development. A pure function always produces the same output for a given input and has no side effects. This property makes pure functions highly predictable, easy to test, and perfect for parallel execution. In the context of AI, pure functions can be used to implement various components of our algorithms, from data preprocessing to model evaluation.
Here’s an example of how we might use pure functions in a machine learning context:
def normalize_features(features):
return (features - np.mean(features, axis=0)) / np.std(features, axis=0)
def compute_accuracy(predictions, labels):
return np.mean(predictions == labels)
def train_model(model, features, labels, epochs):
for _ in range(epochs):
normalized_features = normalize_features(features)
predictions = model.predict(normalized_features)
accuracy = compute_accuracy(predictions, labels)
model = model.update(normalized_features, labels)
return model, accuracy
In this example, normalize_features
and compute_accuracy
are pure functions that always produce the same output for the same input. The train_model
function, while not strictly pure due to the iterative nature of training, still follows functional principles by returning a new model rather than modifying an existing one.
Reactive Programming: Responding to Data Streams in Real-Time AI
As AI systems become more integrated into real-time applications and services, the ability to efficiently handle continuous streams of data becomes increasingly important. This is where reactive programming shines, providing a paradigm for building AI systems that can respond to data streams in a flexible and efficient manner.
The observer pattern: Foundation of reactive systems
At the heart of reactive programming is the observer pattern, where objects (called observers) are notified automatically of any state changes in the objects they’re observing (called observables). This pattern is particularly useful in AI systems that need to process and react to real-time data streams. Here’s a simple example of how we might implement this pattern in a Python-based AI system:
class DataStream:
def __init__(self):
self._observers = []
def subscribe(self, observer):
self._observers.append(observer)
def notify(self, data):
for observer in self._observers:
observer.update(data)
class AIModel:
def update(self, data):
processed_data = self.preprocess(data)
prediction = self.predict(processed_data)
self.take_action(prediction)
data_stream = DataStream()
model = AIModel()
data_stream.subscribe(model)
# In a real-time system, this would be called as new data arrives
data_stream.notify(new_data)
This structure allows our AI model to automatically process and respond to new data as it becomes available, without the need for explicit polling or checking.
Reactive extensions: Powerful tools for stream processing
Building on the observer pattern, reactive extensions (often abbreviated as Rx) provide a rich set of operators for composing and transforming asynchronous data streams. These tools are particularly valuable in AI systems that need to perform complex operations on real-time data streams. Here’s an example of how we might use RxPY (the Python implementation of Rx) in an AI context:
import rx
from rx import operators as ops
def preprocess(data):
# Preprocessing logic here
return processed_data
def predict(data):
# Prediction logic here
return prediction
def take_action(prediction):
# Action logic here
pass
rx.from_(data_stream).pipe(
ops.map(lambda data: preprocess(data)),
ops.map(lambda processed_data: predict(processed_data)),
ops.filter(lambda prediction: prediction.confidence > 0.8),
ops.map(lambda prediction: take_action(prediction))
).subscribe()
This reactive approach allows us to create a processing pipeline that can efficiently handle a continuous stream of data, applying preprocessing, making predictions, filtering based on confidence, and taking actions, all in a declarative and composable manner.
Conclusion
As we’ve explored in this deep dive into control flow for AI, there’s a rich tapestry of techniques and paradigms available to AI developers. From the fundamental if-else statements to advanced concepts like functional and reactive programming, each approach offers unique benefits and is suited to different aspects of AI development.
The key to building truly intelligent and efficient AI systems lies in understanding this full spectrum of control flow techniques and knowing when and how to apply them. By combining these approaches judiciously, we can create AI systems that are not only powerful and flexible but also maintainable, scalable, and robust.
As the field of AI continues to evolve at a breakneck pace, staying up-to-date with these control flow techniques and paradigms is crucial. They form the backbone of how we implement complex decision-making processes, handle vast amounts of data, and create AI systems that can adapt and respond to the ever-changing real-world environments they operate in.
So, whether you’re developing the next breakthrough in natural language processing, creating adaptive game AI, or building real-time data analysis systems, remember that mastering control flow is key to unlocking the full potential of artificial intelligence. Keep exploring, keep learning, and keep pushing the boundaries of what’s possible with AI!
Disclaimer: This blog post is intended for educational purposes only. While we strive for accuracy, the field of AI is rapidly evolving, and some concepts or techniques mentioned may become outdated over time. Always refer to the latest documentation and best practices when implementing AI systems. If you notice any inaccuracies in this post, please report them so we can correct them promptly.