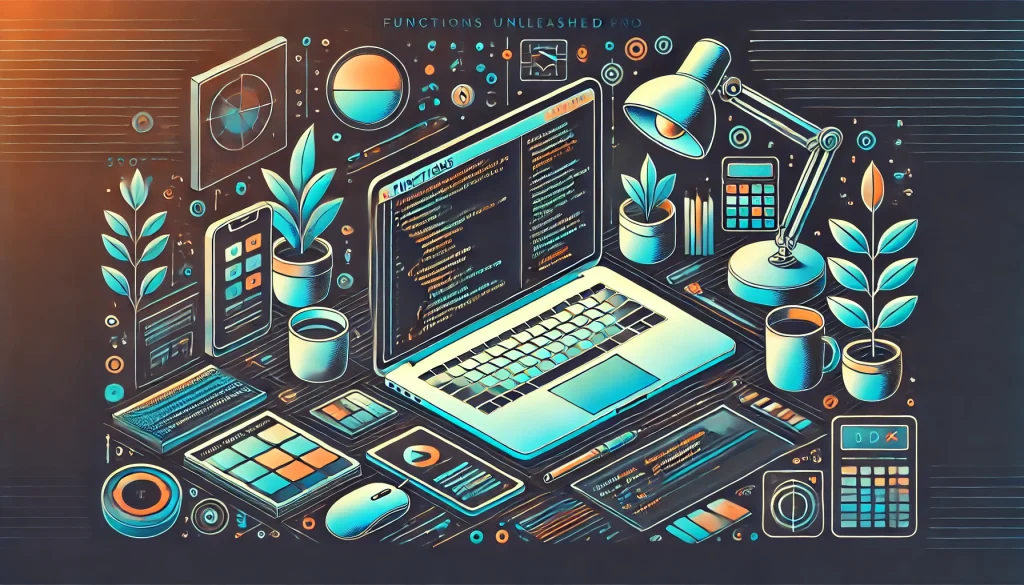
Functions Unleashed: Streamlining Your AI Code Like a Pro
In the ever-evolving world of artificial intelligence and machine learning, writing efficient and maintainable code is crucial. As AI projects grow in complexity, developers often find themselves wrestling with unwieldy codebases that are difficult to debug, scale, and collaborate on. This is where the power of functions comes into play. By leveraging functions effectively, you can transform your AI code from a tangled mess into a streamlined masterpiece. In this blog post, we’ll dive deep into the art of using functions to optimize your AI code, exploring best practices, advanced techniques, and real-world examples that will take your coding skills to the next level.
The Foundation: Understanding Functions in AI Development
Before we dive into the nitty-gritty of optimizing your AI code with functions, let’s take a moment to refresh our understanding of what functions are and why they’re so important in the context of AI development. At their core, functions are reusable blocks of code that perform specific tasks. They allow you to organize your code into manageable chunks, promote code reuse, and make your programs more modular and easier to understand. In the world of AI, where complex algorithms and data processing pipelines are the norm, functions become even more critical.
The building blocks of AI code
Functions serve as the building blocks of your AI code, encapsulating logic for tasks like data preprocessing, model training, feature extraction, and prediction. By breaking down your AI algorithms into smaller, focused functions, you create a more modular and flexible codebase. This modularity not only makes your code easier to read and maintain but also allows for easier testing and debugging. Imagine trying to troubleshoot a 1000-line monolithic script versus a well-organized collection of functions, each responsible for a specific part of your AI pipeline. The latter scenario is undoubtedly more manageable and less likely to induce headaches.
Promoting code reuse and reducing redundancy
One of the biggest advantages of using functions in AI development is the ability to reuse code across different parts of your project or even across multiple projects. Instead of copy-pasting the same code snippets for common tasks like data normalization or model evaluation, you can create functions that encapsulate these operations. This approach not only saves time but also reduces the risk of introducing errors through inconsistent implementations. When you need to update or optimize a particular operation, you only need to modify the function in one place, and the changes will propagate throughout your codebase.
Best Practices for Creating Functions in AI Code
Now that we’ve established the importance of functions in AI development, let’s explore some best practices for creating and using functions effectively in your code. These guidelines will help you write cleaner, more maintainable, and more efficient AI code.
Keep it focused: Single Responsibility Principle
When creating functions for your AI code, it’s essential to adhere to the Single Responsibility Principle (SRP). This principle states that a function should have one, and only one, reason to change. In other words, each function should be responsible for a single, well-defined task. For example, instead of creating a monolithic function that loads data, preprocesses it, trains a model, and evaluates the results, break these tasks into separate functions. This approach not only makes your code more modular but also improves readability and makes it easier to test and debug individual components of your AI pipeline.
Choose descriptive and meaningful names
The importance of choosing good function names cannot be overstated. A well-named function acts as documentation, immediately conveying its purpose to anyone reading the code. In AI development, where complex algorithms and data transformations are common, clear and descriptive function names become even more crucial. Avoid vague names like “process_data()” and opt for more specific names like “normalize_feature_vectors()” or “train_random_forest_classifier()”. Remember, you’re not just writing code for the computer to execute; you’re writing it for other developers (including your future self) to understand and maintain.
Use type hints and docstrings
Python’s type hints and docstrings are powerful tools for improving the clarity and maintainability of your AI code. Type hints provide information about the expected types of function parameters and return values, making it easier to catch type-related errors early and improve code readability. Docstrings, on the other hand, allow you to document the purpose, parameters, return values, and any important details about your functions. In AI development, where complex mathematical operations and data transformations are common, well-written docstrings can be invaluable for understanding the intricacies of your code.
Optimize function arguments
When designing functions for your AI code, pay careful attention to how you handle function arguments. Use default values for parameters that have common settings, allowing users of your function to specify only the necessary arguments. Consider using keyword arguments for functions with many parameters to improve readability and reduce the chances of errors. For functions that may need to accept a variable number of arguments, leverage Python’s *args and **kwargs syntax. These techniques will make your functions more flexible and easier to use across different parts of your AI project.
Advanced Function Techniques for AI Development
As you become more comfortable with using functions in your AI code, it’s time to explore some advanced techniques that can take your coding skills to the next level. These approaches will help you write more elegant, efficient, and powerful AI code.
Embrace functional programming concepts
Functional programming concepts can be particularly useful in AI development, where data transformations and mathematical operations are common. Functions like map(), filter(), and reduce() allow you to apply operations to collections of data in a concise and efficient manner. For example, instead of using a for loop to normalize a list of feature vectors, you could use map() with a normalization function. This approach not only makes your code more readable but can also lead to performance improvements, especially when working with large datasets.
Leverage decorators for code reuse and abstraction
Decorators are a powerful feature in Python that allow you to modify or enhance the behavior of functions without changing their core implementation. In AI development, decorators can be used for a variety of purposes, such as timing function execution, implementing caching for expensive computations, or adding logging capabilities to your functions. By using decorators, you can separate cross-cutting concerns from your core AI logic, resulting in cleaner and more maintainable code. For instance, you could create a decorator that automatically caches the results of a feature extraction function, improving performance when working with large datasets.
Explore higher-order functions
Higher-order functions, which are functions that can accept other functions as arguments or return functions as results, are incredibly powerful tools in AI development. They allow you to create more flexible and reusable code by abstracting common patterns and behaviors. For example, you could create a higher-order function that applies a given transformation to a dataset, allowing you to easily swap out different preprocessing techniques without changing the overall structure of your code. This approach is particularly useful when experimenting with different AI algorithms or data processing techniques.
Implement generator functions for memory efficiency
When working with large datasets or complex AI models, memory management becomes crucial. Generator functions, which allow you to iterate over a sequence of values without storing the entire sequence in memory, can be a game-changer in such scenarios. By using generator functions for tasks like data loading or feature extraction, you can process large datasets in a memory-efficient manner. This approach is particularly useful when working with streaming data or when you need to apply transformations to datasets that are too large to fit in memory.
Real-World Examples: Functions in Action
Now that we’ve covered both fundamental and advanced function techniques, let’s explore some real-world examples of how functions can be used to streamline and optimize AI code. These examples will demonstrate the practical application of the concepts we’ve discussed and provide inspiration for your own AI projects.
Example 1: Building a modular data preprocessing pipeline
Data preprocessing is a critical step in any AI project, and it’s an area where functions can really shine. Let’s look at how we can create a modular and flexible preprocessing pipeline using functions:
import numpy as np
from typing import List, Callable
def normalize_features(data: np.ndarray) -> np.ndarray:
"""
Normalize features using min-max scaling.
Args:
data (np.ndarray): Input feature array
Returns:
np.ndarray: Normalized feature array
"""
min_vals = np.min(data, axis=0)
max_vals = np.max(data, axis=0)
return (data - min_vals) / (max_vals - min_vals)
def handle_missing_values(data: np.ndarray, strategy: str = 'mean') -> np.ndarray:
"""
Handle missing values in the dataset.
Args:
data (np.ndarray): Input feature array
strategy (str): Strategy for handling missing values ('mean', 'median', or 'mode')
Returns:
np.ndarray: Array with missing values handled
"""
if strategy == 'mean':
return np.nan_to_num(data, nan=np.nanmean(data, axis=0))
elif strategy == 'median':
return np.nan_to_num(data, nan=np.nanmedian(data, axis=0))
elif strategy == 'mode':
return np.nan_to_num(data, nan=np.nanmode(data, axis=0)[0])
else:
raise ValueError("Invalid strategy. Choose 'mean', 'median', or 'mode'.")
def preprocess_pipeline(data: np.ndarray, steps: List[Callable]) -> np.ndarray:
"""
Apply a series of preprocessing steps to the input data.
Args:
data (np.ndarray): Input feature array
steps (List[Callable]): List of preprocessing functions to apply
Returns:
np.ndarray: Preprocessed feature array
"""
for step in steps:
data = step(data)
return data
# Usage example
raw_data = np.random.rand(100, 5)
preprocessed_data = preprocess_pipeline(raw_data, [
handle_missing_values,
normalize_features
])
In this example, we’ve created separate functions for different preprocessing tasks and a higher-order function (preprocess_pipeline) that allows us to easily combine and reorder these tasks. This modular approach makes it easy to experiment with different preprocessing strategies and adapt the pipeline to different datasets or AI models.
Example 2: Implementing a flexible model evaluation framework
Evaluating AI models often involves repeating similar steps with different algorithms or hyperparameters. Functions can help create a flexible and reusable evaluation framework:
from typing import Callable, Dict, Any
from sklearn.model_selection import cross_val_score
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
def train_and_evaluate(model: Any, X: np.ndarray, y: np.ndarray,
cv: int = 5, scoring: str = 'accuracy') -> Dict[str, float]:
"""
Train a model using cross-validation and evaluate its performance.
Args:
model: Scikit-learn compatible model object
X (np.ndarray): Feature matrix
y (np.ndarray): Target vector
cv (int): Number of cross-validation folds
scoring (str): Scoring metric for cross-validation
Returns:
Dict[str, float]: Dictionary of evaluation metrics
"""
cv_scores = cross_val_score(model, X, y, cv=cv, scoring=scoring)
model.fit(X, y)
y_pred = model.predict(X)
return {
'cv_score_mean': np.mean(cv_scores),
'cv_score_std': np.std(cv_scores),
'accuracy': accuracy_score(y, y_pred),
'precision': precision_score(y, y_pred, average='weighted'),
'recall': recall_score(y, y_pred, average='weighted'),
'f1_score': f1_score(y, y_pred, average='weighted')
}
def compare_models(models: Dict[str, Any], X: np.ndarray, y: np.ndarray,
cv: int = 5, scoring: str = 'accuracy') -> Dict[str, Dict[str, float]]:
"""
Compare multiple models on the same dataset.
Args:
models (Dict[str, Any]): Dictionary of model names and objects
X (np.ndarray): Feature matrix
y (np.ndarray): Target vector
cv (int): Number of cross-validation folds
scoring (str): Scoring metric for cross-validation
Returns:
Dict[str, Dict[str, float]]: Dictionary of model names and their evaluation metrics
"""
results = {}
for name, model in models.items():
results[name] = train_and_evaluate(model, X, y, cv, scoring)
return results
# Usage example
from sklearn.ensemble import RandomForestClassifier
from sklearn.svm import SVC
X, y = load_your_dataset() # Replace with your data loading function
models = {
'Random Forest': RandomForestClassifier(),
'SVM': SVC()
}
evaluation_results = compare_models(models, X, y)
This example demonstrates how functions can be used to create a flexible model evaluation framework. The train_and_evaluate function encapsulates the process of training and evaluating a single model, while the compare_models function allows for easy comparison of multiple models. This approach makes it simple to experiment with different models and evaluation metrics, promoting more thorough and efficient AI development.
Optimizing Function Performance in AI Code
As your AI projects grow in complexity and scale, optimizing the performance of your functions becomes increasingly important. Let’s explore some techniques for improving the efficiency of your functions in AI code.
Vectorization: Harnessing the power of NumPy
When working with numerical data in AI applications, vectorization can significantly improve the performance of your functions. Instead of using loops to perform operations on individual elements, leverage NumPy’s vectorized operations to process entire arrays at once. This approach not only makes your code more concise but also takes advantage of optimized, low-level implementations for better performance. Here’s an example of how vectorization can improve a simple function:
import numpy as np
import time
# Non-vectorized function
def euclidean_distance_loop(x1, x2):
return np.sqrt(np.sum([(a - b) ** 2 for a, b in zip(x1, x2)]))
# Vectorized function
def euclidean_distance_vectorized(x1, x2):
return np.sqrt(np.sum((x1 - x2) ** 2))
# Performance comparison
x1 = np.random.rand(1000000)
x2 = np.random.rand(1000000)
start = time.time()
result_loop = euclidean_distance_loop(x1, x2)
end = time.time()
print(f"Loop version: {end - start:.6f} seconds")
start = time.time()
result_vectorized = euclidean_distance_vectorized(x1, x2)
end = time.time()
print(f"Vectorized version: {end - start:.6f} seconds")
The vectorized version of the function will typically be orders of magnitude faster, especially for large arrays. When writing functions for AI tasks like feature extraction or distance calculations, always consider if there’s a vectorized alternative to your implementation.
Caching and memoization
In AI development, you may encounter functions that perform expensive computations but are called multiple times with the same arguments. In such cases, caching the results of these functions can lead to significant performance improvements. Python’s functools.lru_cache decorator provides an easy way to add caching to your functions:
from functools import lru_cache
import time
@lru_cache(maxsize=None)
def expensive_ai_operation(x):
time.sleep(2) # Simulate a time-consuming operation
return x ** 2
# First call: takes about 2 seconds
start = time.time()
result1 = expensive_ai_operation(10)
end = time.time()
print(f"First call: {end - start:.6f} seconds")
# Second call with the same argument: returns immediately
start = time.time()
result2 = expensive_ai_operation(10)
end = time.time()
print(f"Second call: {end - start:.6f} seconds")
By using caching, you can avoid redundant computations and significantly speed up your AI code, especially when working with recursive algorithms or functions that are called repeatedly during model training or evaluation.
Profiling and optimization
To identify performance bottlenecks in your AI functions, it’s essential to use profiling tools. Python’s cProfile module and third-party tools like line_profiler can help you pinpoint which functions or lines of code are consuming the most time. Once you’ve identified the bottlenecks, you can focus on optimizing those specific functions. Here’s a simple example of using cProfile
import cProfile
import pstats
def ai_pipeline():
# Your AI pipeline implementation here
pass
cProfile.run('ai_pipeline()', 'ai_profile_stats')
stats = pstats.Stats('ai_profile_stats')
stats.sort_stats('cumulative').print_stats(10) # Print top 10 time-consuming functions
By profiling your AI code, you can make data-driven decisions about which functions to optimize, ensuring that you’re focusing your efforts where they’ll have the most impact.
Collaborative Development: Functions as a Team Player
In the world of AI development, collaboration is key. Functions play a crucial role in making your code more accessible and understandable to team members. Let’s explore how functions can enhance collaborative AI development.
Creating a shared function library
As your AI project grows, you’ll likely find yourself reusing certain functions across different modules or even different projects. Creating a shared function library can significantly improve code reuse and maintainability. Here’s an example of how you might structure a shared library for common AI tasks:
#ai_utils.py
import numpy as np
from typing import List, Tuple
def train_test_split(X: np.ndarray, y: np.ndarray, test_size: float = 0.2,
random_state: int = None) -> Tuple[np.ndarray, np.ndarray, np.ndarray, np.ndarray]:
"""
Split the dataset into training and testing sets.
Args:
X (np.ndarray): Feature matrix
y (np.ndarray): Target vector
test_size (float): Proportion of the dataset to include in the test split
random_state (int): Seed for the random number generator
Returns:
Tuple[np.ndarray, np.ndarray, np.ndarray, np.ndarray]: X_train, X_test, y_train, y_test
"""
np.random.seed(random_state)
indices = np.random.permutation(len(X))
test_size = int(len(X) * test_size)
test_indices = indices[:test_size]
train_indices = indices[test_size:]
return X[train_indices], X[test_indices], y[train_indices], y[test_indices]
def cross_validation(model: Any, X: np.ndarray, y: np.ndarray,
n_splits: int = 5) -> List[float]:
"""
Perform cross-validation on the given model and dataset.
Args:
model: Scikit-learn compatible model object
X (np.ndarray): Feature matrix
y (np.ndarray): Target vector
n_splits (int): Number of cross-validation splits
Returns:
List[float]: List of scores for each split
"""
scores = []
for i in range(n_splits):
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=1/n_splits)
model.fit(X_train, y_train)
scores.append(model.score(X_test, y_test))
return scores
# Add more utility functions as needed
By creating a shared library of well-documented, type-hinted functions, you make it easier for team members to understand and use common AI operations. This approach promotes consistency across your project and reduces the likelihood of bugs caused by multiple implementations of the same functionality.
Documenting functions for team understanding
Clear documentation is crucial for collaborative AI development. When writing functions, invest time in creating comprehensive docstrings that explain the purpose of the function, its parameters, return values, and any important details or caveats. Here’s an example of a well-documented function for feature selection:
from typing import List, Tuple
import numpy as np
from sklearn.feature_selection import SelectKBest, f_classif
def select_top_features(X: np.ndarray, y: np.ndarray, k: int) -> Tuple[np.ndarray, List[int]]:
"""
Select the top k features based on ANOVA F-value.
This function uses the ANOVA F-value statistic to select the k features
that have the strongest relationship with the target variable. It's suitable
for classification tasks with numerical features.
Args:
X (np.ndarray): Feature matrix of shape (n_samples, n_features)
y (np.ndarray): Target vector of shape (n_samples,)
k (int): Number of top features to select
Returns:
Tuple[np.ndarray, List[int]]: A tuple containing:
- np.ndarray: Transformed X matrix with only the selected features
- List[int]: Indices of the selected features
Raises:
ValueError: If k is greater than the number of features in X
Example:
>>> X = np.random.rand(100, 10)
>>> y = np.random.randint(0, 2, 100)
>>> X_selected, selected_indices = select_top_features(X, y, k=5)
>>> print(f"Selected feature indices: {selected_indices}")
>>> print(f"Shape of transformed X: {X_selected.shape}")
"""
if k > X.shape[1]:
raise ValueError(f"k ({k}) must be <= number of features ({X.shape[1]})")
selector = SelectKBest(f_classif, k=k)
X_selected = selector.fit_transform(X, y)
selected_indices = selector.get_support(indices=True)
return X_selected, selected_indices.tolist()
By providing detailed documentation, you make it easier for team members to understand and correctly use your functions, even if they weren’t involved in writing the original code.
Conclusion
As we’ve explored throughout this blog post, functions are a powerful tool for streamlining and optimizing your AI code. By breaking down complex algorithms into modular, reusable functions, you can create more maintainable, efficient, and collaborative AI projects. From basic best practices like adhering to the Single Responsibility Principle and using descriptive names, to advanced techniques like leveraging decorators and optimizing performance through vectorization, the strategic use of functions can elevate your AI development to new heights.
Remember, the goal is not just to write code that works, but to create a codebase that is easy to understand, modify, and scale as your AI projects grow in complexity. By embracing the power of functions, you’re not only improving your current code but also investing in the future of your AI development efforts.
As you continue your journey in AI development, challenge yourself to look for opportunities to refactor your code using the function techniques we’ve discussed. Experiment with different approaches, measure their impact on performance and readability, and share your insights with your team. The more you practice and refine your function-writing skills, the more natural and intuitive it will become to structure your AI code in a clean, efficient manner.
Happy coding, and may your AI functions be ever elegant and powerful!
Disclaimer: The code examples and techniques presented in this blog post are for educational purposes only. While we strive for accuracy, the field of AI is rapidly evolving, and best practices may change over time. Always consult the latest documentation and research when implementing AI solutions in production environments. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.