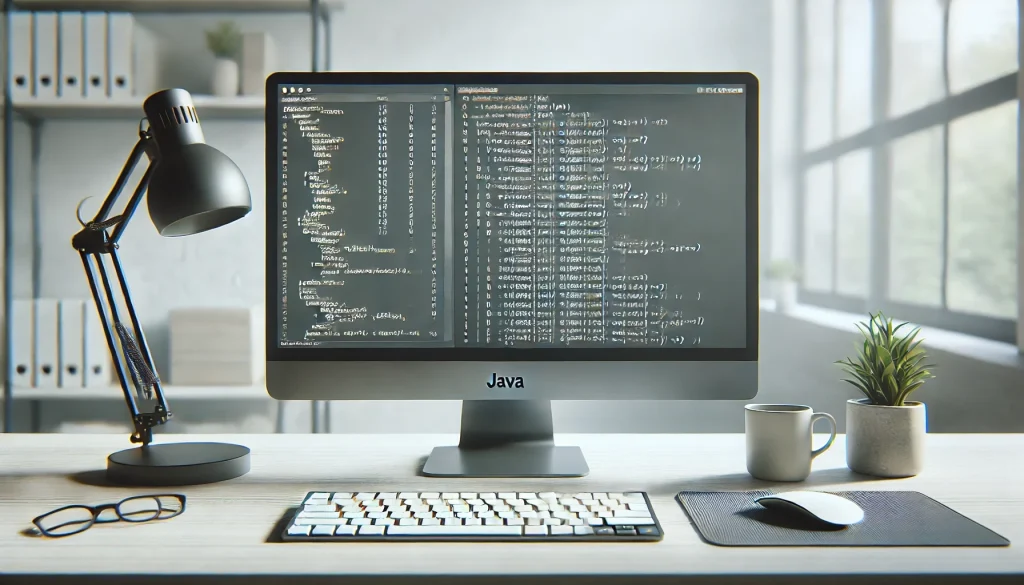
Java Arrays: Your First Step in Organized Data
Hey there, fellow code enthusiasts! Today, we’re diving into the world of Java arrays – the unsung heroes of organized data in programming. Whether you’re just starting your coding journey or looking to brush up on your skills, understanding arrays is crucial. They’re like the Swiss Army knife of data structures, helping you store and manipulate collections of information with ease. So, grab your favorite beverage, get comfy, and let’s embark on this exciting adventure into the realm of Java arrays!
What Are Java Arrays, Anyway?
Picture this: you’re planning a party and need to keep track of your guest list. Instead of scribbling names on random scraps of paper, wouldn’t it be great to have a neat, organized list? That’s exactly what arrays do in the programming world! An array in Java is like a container that holds multiple items of the same type. It’s a fundamental data structure that allows you to store and access multiple values of the same data type using a single variable name. Think of it as a row of boxes, each containing a piece of data, all neatly labeled and easy to access. Arrays help you manage collections of data efficiently, making your code cleaner and more organized. They’re the building blocks for more complex data structures and are essential in countless programming scenarios, from simple list management to complex algorithms.
Why Should You Care About Arrays?
Now, you might be wondering, “Why should I bother learning about arrays?” Well, my friend, arrays are like the backbone of data organization in programming. They’re used everywhere – from storing user inputs and managing game states to handling large datasets in scientific applications. Learning to work with arrays will significantly boost your programming skills and open doors to more advanced concepts. Arrays help you write more efficient code, reduce repetition, and handle large amounts of data with ease. Plus, they’re a stepping stone to understanding more complex data structures like lists, sets, and maps. Mastering arrays will make you a more versatile and capable programmer, ready to tackle a wide range of coding challenges.
Creating Your First Array
Let’s roll up our sleeves and create our first array! In Java, there are a few ways to declare and initialize an array. Here’s the basic syntax:
// Declaration
dataType[] arrayName;
// Initialization
arrayName = new dataType[arraySize];
// Or, you can combine declaration and initialization
dataType[] arrayName = new dataType[arraySize];
Let’s break this down with a real example. Say we want to create an array to store the ages of five friends:
int[] ages = new int[5];
Here, we’ve created an array called ages
that can hold 5 integer values. It’s like setting up 5 empty boxes, ready to be filled with age data. But wait, there’s more! You can also create an array and fill it with values in one go:
int[] ages = {25, 30, 22, 28, 33};
This shorthand notation creates the array and populates it with the given values. It’s like buying a pre-packed set of boxes, each already containing an age. Pretty neat, right? Remember, all elements in an array must be of the same data type. So, if you’re storing ages as integers, every element needs to be an integer.
Accessing and Modifying Array Elements
Now that we’ve created our array, how do we actually use it? Accessing elements in an array is straightforward – we use the index of the element. In Java, array indices start at 0, not 1. This means the first element is at index 0, the second at index 1, and so on. Here’s how you can access and modify array elements:
int[] ages = {25, 30, 22, 28, 33};
// Accessing an element
System.out.println("The first age is: " + ages[0]); // Outputs: 25
// Modifying an element
ages[2] = 23;
System.out.println("The updated third age is: " + ages[2]); // Outputs: 23
It’s crucial to remember that array indices go from 0 to (array length – 1). Trying to access an index outside this range will result in an ArrayIndexOutOfBoundsException
. This is Java’s way of saying, “Hey, you’re trying to access a box that doesn’t exist!” Always be mindful of your array’s size to avoid these pesky exceptions.
Looping Through Arrays
One of the most powerful features of arrays is the ability to process all elements efficiently using loops. This is where arrays really shine, allowing you to perform operations on multiple data points with just a few lines of code. Let’s explore two common ways to loop through arrays:
Using a for loop:
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println("Element at index " + i + ": " + numbers[i]);
}
This traditional for
loop uses the array’s length property to iterate through each element. It’s versatile and gives you control over the index, which can be useful in many scenarios.
Using an enhanced for loop (for-each loop):
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println("Current element: " + number);
}
The enhanced for
loop, introduced in Java 5, provides a more concise and readable way to iterate through arrays. It’s especially useful when you need to process each element without needing to know its index. These looping techniques are fundamental to working with arrays effectively. They allow you to perform operations like summing all elements, finding maximum or minimum values, or applying a transformation to each element with ease.
Multidimensional Arrays: Arrays of Arrays
Just when you thought arrays couldn’t get any cooler, let me introduce you to multidimensional arrays! These are essentially arrays of arrays, allowing you to create tables or matrices of data. The most common type is a two-dimensional array, which you can think of as a grid or a table with rows and columns. Here’s how you can create and use a 2D array:
// Creating a 2D array
int[][] matrix = new int[3][3];
// Initializing a 2D array
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
// Accessing elements in a 2D array
System.out.println("Element at row 1, column 2: " + matrix[1][2]); // Outputs: 6
// Looping through a 2D array
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println(); // Move to the next line after each row
}
Multidimensional arrays are incredibly useful for representing complex data structures like game boards, spreadsheets, or mathematical matrices. They allow you to organize data in a more intuitive, table-like format. However, remember that with great power comes great responsibility – always be careful with your indices to avoid out-of-bounds errors!
Array Methods and Utilities
Java provides several built-in methods and utilities to make working with arrays even easier. These tools can save you time and effort when performing common operations on arrays. Let’s explore some of the most useful ones:
Arrays.toString():
This method converts an array to a string representation, which is great for printing or debugging.
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(numbers)); // Outputs: [1, 2, 3, 4, 5]
Arrays.sort():
This method sorts the elements of an array in ascending order.
int[] numbers = {5, 2, 8, 1, 9};
Arrays.sort(numbers);
System.out.println(Arrays.toString(numbers)); // Outputs: [1, 2, 5, 8, 9]
Arrays.fill():
This method fills an array with a specific value.
int[] numbers = new int[5];
Arrays.fill(numbers, 10);
System.out.println(Arrays.toString(numbers)); // Outputs: [10, 10, 10, 10, 10]
Arrays.binarySearch():
This method searches for a specific element in a sorted array and returns its index.
int[] numbers = {1, 2, 3, 4, 5};
int index = Arrays.binarySearch(numbers, 3);
System.out.println("Index of 3: " + index); // Outputs: 2
These utility methods are part of the java.util.Arrays
class and can significantly simplify your array operations. They’re optimized for performance and can handle large arrays efficiently. Using these built-in methods not only makes your code more readable but also more reliable, as they’ve been thoroughly tested and optimized by Java’s developers.
Common Pitfalls and How to Avoid Them
As with any programming concept, there are some common mistakes that developers often make when working with arrays. Let’s go through some of these pitfalls and learn how to avoid them:
Array Index Out of Bounds:
This is probably the most common error when working with arrays. It occurs when you try to access an array element using an index that’s either negative or greater than or equal to the array’s length.
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]); // Throws ArrayIndexOutOfBoundsException
To avoid this, always ensure that your index is within the valid range (0 to array.length – 1). When looping, use the array’s length property to set the loop’s upper bound.
Forgetting That Arrays Are Zero-Indexed:
In Java, array indices start at 0, not 1. This can be confusing, especially for beginners.
int[] numbers = {10, 20, 30};
System.out.println("First element: " + numbers[0]); // Correct: 10
System.out.println("Last element: " + numbers[numbers.length - 1]); // Correct: 30
Always remember that the last element’s index is array.length - 1
, not array.length
.
Not Initializing Array Elements:
When you create an array without initializing its elements, Java assigns default values (0 for numeric types, false for boolean, null for objects). This can lead to unexpected behavior if you forget to initialize elements before using them.
int[] numbers = new int[5];
System.out.println(numbers[2]); // Outputs: 0 (default value for int)
Make sure to initialize array elements with meaningful values before using them in your program.
Comparing Arrays with ==:
Using ==
compares array references, not their contents. To compare array contents, use Arrays.equals()
for one-dimensional arrays or Arrays.deepEquals()
for multi-dimensional arrays.
int[] arr1 = {1, 2, 3};
int[] arr2 = {1, 2, 3};
System.out.println(arr1 == arr2); // Outputs: false
System.out.println(Arrays.equals(arr1, arr2)); // Outputs: true
By being aware of these common pitfalls and knowing how to avoid them, you’ll write more robust and error-free code when working with arrays. Remember, practice makes perfect, so don’t be discouraged if you encounter these issues – they’re all part of the learning process!
Arrays vs. ArrayList: When to Use Which?
As you delve deeper into Java programming, you’ll encounter another data structure called ArrayList. While both arrays and ArrayList serve the purpose of storing collections of elements, they have some key differences. Let’s compare them to understand when to use each:
Arrays:
- Have a fixed size once created
- Can store both primitive types and objects
- Generally offer better performance for basic operations
- Syntax:
int[] numbers = new int[5];
ArrayList:
- Can dynamically resize
- Can only store objects (uses wrapper classes for primitives)
- Offers more built-in methods for manipulation
- Syntax:
ArrayList<Integer> numbers = new ArrayList<>();
Here’s a quick example to illustrate the difference:
// Using an Array
int[] arrayNumbers = new int[3];
arrayNumbers[0] = 1;
arrayNumbers[1] = 2;
arrayNumbers[2] = 3;
// arrayNumbers[3] = 4; // This would cause an ArrayIndexOutOfBoundsException
// Using an ArrayList
ArrayList<Integer> listNumbers = new ArrayList<>();
listNumbers.add(1);
listNumbers.add(2);
listNumbers.add(3);
listNumbers.add(4); // This works fine, ArrayList grows dynamically
So, when should you use each? Use arrays when you know the exact size of your collection in advance and it won’t change. Arrays are great for performance-critical applications where you’re doing a lot of basic operations like accessing elements by index. On the other hand, use ArrayList when you need a dynamic size, when you’re frequently adding or removing elements, or when you need the additional utility methods that ArrayList provides. ArrayList is more flexible but comes with a slight performance overhead compared to arrays.
Real-World Applications of Arrays
Arrays aren’t just academic concepts – they’re widely used in real-world programming scenarios. Let’s explore some practical applications to give you a better idea of how arrays are used in actual software development:
1. Image Processing:
In image processing applications, 2D arrays are often used to represent pixels. Each element in the array corresponds to a pixel, storing color or intensity information.
int[][] image = new int[height][width];
// Each element represents a pixel's color value
2. Game Development:
Arrays are extensively used in game development. For example, a chess game might use a 2D array to represent the board:
String[][] chessBoard = new String[8][8];
chessBoard[0][0] = "Rook";
chessBoard[0][1] = "Knight";
// ... and so on
3. Data Analysis:
When working with large datasets, arrays can be used to store and manipulate data efficiently. For instance, storing temperature readings:
double[] temperatures = new double[365]; // Storing daily temperatures for a year
4. Inventory Management:
Arrays can be used to keep track of items in an inventory system:
String[] inventory = {"Apple", "Banana", "Orange", "Grape", "Mango"};
int[] quantities = {50, 40, 30, 60, 20};
5. Audio Processing:
In audio applications, arrays are used to store audio samples:
short[] audioSamples = new short[44100]; // Storing 1 second of audio at 44.1kHz
These examples just scratch the surface of how arrays are used in real-world applications. As you progress in your programming journey, you’ll discover many more scenarios where arrays prove invaluable. Their simplicity and efficiency make them a go-to choice for many programming tasks, from simple data storage to complex algorithmic operations.
Advanced Array Techniques
As you become more comfortable with basic array operations, it’s time to explore some advanced techniques that can take your array manipulation skills to the next level. These techniques are often used in more complex programming scenarios and can significantly improve the efficiency and readability of your code.
Copying Arrays:
Sometimes you need to create a copy of an array. Java provides several ways to do this:
int[] original = {1, 2, 3, 4, 5};
// Using Arrays.copyOf()
int[] copy1 = Arrays.copyOf(original, original.length);
// Using System.arraycopy()
int[] copy2 = new int[original.length];
System.arraycopy(original, 0, copy2, 0, original.length);
// Using clone() method
int[] copy3 = original.clone();
Each method has its use cases. Arrays.copyOf()
is convenient for creating a copy with the same or different length. System.arraycopy()
is more flexible, allowing you to copy a portion of an array. The clone()
method creates a shallow copy, which is fine for primitive types but can be tricky with object arrays.
Array Slicing:
While Java doesn’t have built-in slicing like some other languages, you can achieve similar functionality using Arrays.copyOfRange()
:
int[] numbers = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
int[] slice = Arrays.copyOfRange(numbers, 3, 7);
System.out.println(Arrays.toString(slice)); // Outputs: [3, 4, 5, 6]
This creates a new array containing elements from index 3 (inclusive) to 7 (exclusive) of the original array.
Parallel Array Sorting:
For large arrays, Java provides a parallel sorting method that can significantly speed up the sorting process on multi-core processors:
int[] largeArray = new int[1000000];
// Fill array with random numbers
for (int i = 0; i < largeArray.length; i++) {
largeArray[i] = (int) (Math.random() * 1000000);
}
Arrays.parallelSort(largeArray);
This method uses the Fork/Join framework to sort the array in parallel, potentially offering better performance for large datasets.
Using Arrays with Streams:
Java 8 introduced streams, which can be used with arrays to perform powerful operations:
int[] numbers = {1, 2, 3, 4, 5};
// Calculate sum of squares
int sumOfSquares = Arrays.stream(numbers)
.map(n -> n * n)
.sum();
System.out.println("Sum of squares: " + sumOfSquares);
// Find max value
int max = Arrays.stream(numbers)
.max()
.getAsInt();
System.out.println("Max value: " + max);
Streams provide a concise way to perform complex operations on arrays, including filtering, mapping, and reducing.
These advanced techniques showcase the versatility of arrays in Java. As you incorporate these methods into your coding repertoire, you’ll find yourself able to tackle more complex programming challenges with ease and elegance.
Wrapping Up: Your Array Adventure Begins!
And there you have it, folks – a comprehensive dive into the world of Java arrays! We’ve journeyed from the basics of creating and accessing arrays, through looping and multidimensional arrays, all the way to advanced techniques and real-world applications. Arrays are like the Swiss Army knife in a programmer’s toolkit – versatile, efficient, and incredibly useful in a wide range of scenarios.
Remember, mastering arrays is a crucial step in your programming journey. They form the foundation for understanding more complex data structures and algorithms. As you continue to practice and explore, you’ll find arrays popping up everywhere in your code, helping you organize and manipulate data with ease.
Don’t be discouraged if you find some concepts challenging at first. Like any skill, working with arrays gets easier with practice. Try creating small projects that utilize arrays in different ways – maybe a simple game, a data analysis tool, or an inventory management system. The more you use arrays, the more comfortable and creative you’ll become with them.
As you move forward, keep exploring and learning. Java is a vast language with many powerful features, and arrays are just the beginning. They’re your first step into the exciting world of data structures, opening doors to more advanced concepts like lists, sets, maps, and beyond.
So, what are you waiting for? Fire up your IDE, create some arrays, and start coding! Your journey to becoming a Java master has just begun, and arrays are your trusty companions along the way. Happy coding, and may your arrays always be in bounds!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, programming languages and best practices can evolve. Always refer to the official Java documentation for the most up-to-date information. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly. Remember, the best way to learn is by doing – so don’t hesitate to experiment with the concepts discussed here in your own Java projects!