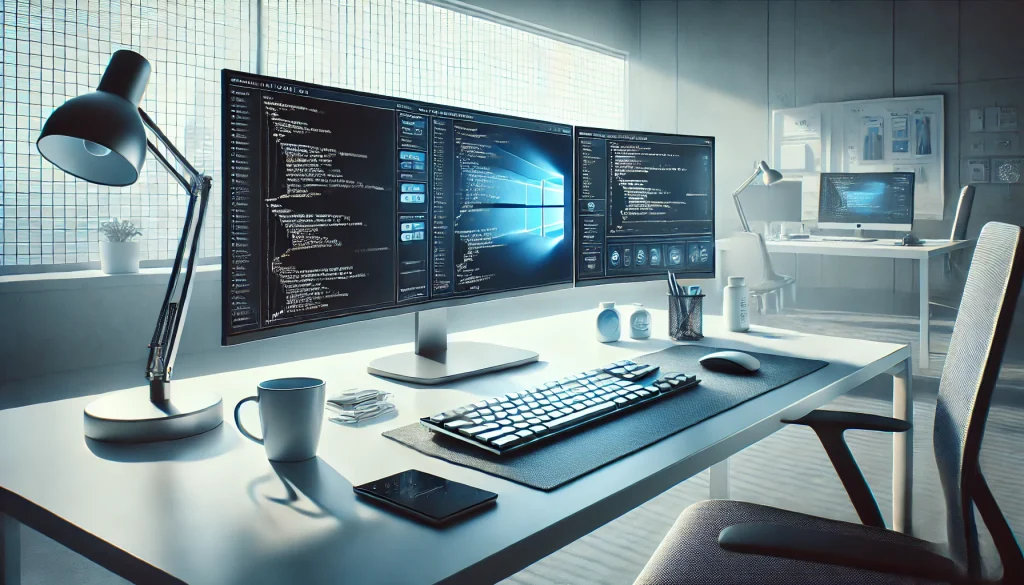
How DevOps Makes Life Easier
Welcome to the world of DevOps, where automation reigns supreme and efficiency is the name of the game. If you’ve ever found yourself drowning in repetitive tasks, juggling multiple tools, or struggling to keep up with the ever-evolving tech landscape, you’re in for a treat. In this blog post, we’ll dive deep into how DevOps practices and automation can transform your workflow, boost productivity, and ultimately make your life as a developer or operations professional a whole lot easier. So, grab a cup of coffee, sit back, and let’s explore the magical realm of Automation Nation!
The DevOps Revolution: More Than Just a Buzzword
You’ve probably heard the term “DevOps” thrown around in tech circles, but what does it really mean? At its core, DevOps is a set of practices that combines software development (Dev) and IT operations (Ops) to shorten the systems development life cycle and provide continuous delivery with high software quality. It’s not just about tools or processes; it’s a cultural shift that emphasizes collaboration, automation, and continuous improvement.
Breaking Down Silos: Gone are the days when developers and operations teams worked in isolation. DevOps breaks down these traditional silos, fostering a culture of shared responsibility and collaboration. This means faster problem-solving, improved communication, and a more streamlined workflow from idea to production.
Continuous Everything: With DevOps, we embrace the concept of “continuous” – continuous integration, continuous delivery, and continuous deployment. This approach allows teams to release new features and updates more frequently and reliably, keeping pace with user demands and market trends.
Automation at the Heart: Perhaps the most transformative aspect of DevOps is its emphasis on automation. By automating repetitive tasks, we free up valuable time and resources, reduce human error, and ensure consistency across our development and deployment processes.
The Power of Automation: Work Smarter, Not Harder
Let’s face it: as much as we love coding and building cool stuff, there are some tasks that are just plain tedious. Enter automation, the superhero of the DevOps world. By leveraging automation tools and practices, we can offload repetitive tasks to machines, allowing us to focus on more creative and strategic work.
Continuous Integration and Deployment: One of the most powerful applications of automation in DevOps is in the realm of continuous integration and deployment (CI/CD). With CI/CD pipelines, code changes are automatically built, tested, and deployed to production environments. This not only speeds up the development process but also ensures that new features and bug fixes reach users faster.
Here’s a simple example of a CI/CD pipeline using Jenkins:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'ansible-playbook deploy.yml'
}
}
}
}
This pipeline automatically builds the project, runs tests, and deploys the application using Ansible, all triggered by a code commit. Imagine doing all of this manually for every change – that’s a recipe for burnout!
Infrastructure as Code: Another game-changing aspect of DevOps automation is Infrastructure as Code (IaC). With IaC, we define and manage our infrastructure using code, just like we do with application software. This allows for version control, easy replication of environments, and consistent infrastructure across development, testing, and production.
Here’s a simple example using Terraform to create an AWS EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "DevOps-Example"
}
}
With this code, we can version control our infrastructure, easily replicate it across environments, and make changes with confidence. No more manual clicking through console interfaces or forgetting crucial configuration details!
DevOps Tools: Your New Best Friends
The DevOps landscape is rich with tools designed to make our lives easier. From version control systems to containerization platforms, these tools are the building blocks of our automated workflows. Let’s explore some of the key players in the DevOps toolchain and how they contribute to our Automation Nation.
Git: Version Control on Steroids: At the heart of any good DevOps workflow is a robust version control system, and Git reigns supreme in this domain. Git allows teams to collaborate efficiently, track changes, and maintain multiple versions of their codebase. But its power really shines when integrated with other DevOps tools.
For example, using Git hooks, we can trigger automated actions on certain events. Here’s a pre-commit hook that runs tests before allowing a commit:
#!/bin/sh
npm test
# $? stores exit value of the last command
if [ $? -ne 0 ]; then
echo "Tests must pass before commit!"
exit 1
fi
This simple script ensures that all tests pass before a developer can commit their changes, catching potential issues early in the development cycle.
Docker: Containerization for the Win: Containerization has revolutionized how we package and deploy applications, and Docker is at the forefront of this revolution. By encapsulating an application and its dependencies in a container, we ensure consistency across different environments and simplify deployment.
Here’s a basic Dockerfile for a Node.js application:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
With this Dockerfile, we can build a container image that includes our application and all its dependencies, ready to be deployed anywhere that supports Docker.
Kubernetes: Orchestrating the Container Symphony: While Docker solves the problem of application packaging and portability, Kubernetes takes it a step further by providing a platform for automating deployment, scaling, and management of containerized applications. With Kubernetes, we can define our desired state in a declarative manner and let the system handle the rest.
Here’s a simple Kubernetes deployment yaml:
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: myapp:1.0
ports:
- containerPort: 80
This yaml file defines a deployment that ensures three replicas of our application are always running, automatically handling scaling and recovery from failures.
Monitoring and Logging: Because What You Can’t Measure, You Can’t Improve
In the world of DevOps, visibility is key. We need to know what’s happening in our systems at all times, and that’s where monitoring and logging come into play. By automating the collection and analysis of metrics and logs, we can quickly identify issues, track performance, and make data-driven decisions.
Prometheus: Metrics Collection Made Easy: Prometheus has become the de facto standard for metrics collection in the DevOps world. Its pull-based architecture and powerful query language make it an excellent choice for monitoring containerized environments.
Here’s a simple example of how to instrument a Python application for Prometheus:
from prometheus_client import start_http_server, Counter
REQUEST_COUNT = Counter('request_count', 'Total number of requests')
def process_request():
# Process the request here
REQUEST_COUNT.inc()
if __name__ == '__main__':
start_http_server(8000)
# Your application logic here
This code sets up a counter to track the number of requests and exposes metrics on port 8000, which Prometheus can scrape.
ELK Stack: Centralized Logging for the Win: The ELK (Elasticsearch, Logstash, Kibana) stack provides a powerful solution for centralized logging. By aggregating logs from all our systems in one place, we can easily search, analyze, and visualize our log data.
Here’s a simple Logstash configuration to collect logs from a file and send them to Elasticsearch:
input {
file {
path => "/var/log/myapp.log"
start_position => "beginning"
}
}
filter {
grok {
match => { "message" => "%{TIMESTAMP_ISO8601:timestamp} %{LOGLEVEL:log_level} %{GREEDYDATA:message}" }
}
}
output {
elasticsearch {
hosts => ["localhost:9200"]
index => "myapp-logs-%{+YYYY.MM.dd}"
}
}
This configuration collects logs from a file, parses them to extract structured data, and sends them to Elasticsearch, where they can be visualized and analyzed using Kibana.
Security in the Age of Automation: DevSecOps
As we automate more of our processes, it’s crucial not to forget about security. Enter DevSecOps, an approach that integrates security practices within the DevOps process. By automating security checks and making security a shared responsibility across the entire development lifecycle, we can build more secure systems without sacrificing speed.
Automated Security Scanning: Tools like SonarQube can be integrated into our CI/CD pipelines to automatically scan code for vulnerabilities and quality issues. Here’s an example of how to include a SonarQube scan in a Jenkins pipeline:
stage('SonarQube Analysis') {
steps {
withSonarQubeEnv('SonarQube') {
sh "${scannerHome}/bin/sonar-scanner"
}
}
}
This stage runs a SonarQube analysis as part of our CI/CD process, ensuring that security checks are performed automatically with every code change.
Secret Management: Keeping secrets (like API keys and passwords) secure is a critical aspect of DevSecOps. Tools like HashiCorp Vault can be used to manage secrets securely and integrate with our automation workflows.
Here’s an example of how to use Vault in a Python application:
import hvac
client = hvac.Client(url='http://localhost:8200', token='my-token')
# Reading a secret
secret = client.secrets.kv.v2.read_secret_version(path='my-secret')
password = secret['data']['data']['password']
# Using the secret
# ...
By using a tool like Vault, we can ensure that secrets are never hard-coded in our application or configuration files, reducing the risk of accidental exposure.
The Human Side of DevOps: Culture and Collaboration
While we’ve focused a lot on tools and automation, it’s important to remember that DevOps is as much about culture as it is about technology. The most sophisticated automation setup in the world won’t help if your team isn’t on board with the DevOps philosophy.
Fostering a Culture of Collaboration: DevOps breaks down the traditional silos between development and operations teams. This requires a shift in mindset, where everyone takes responsibility for the entire software lifecycle. Tools like Slack and Microsoft Teams can facilitate this collaboration, but the real change needs to happen in how we approach our work.
Continuous Learning and Improvement: The tech landscape is constantly evolving, and so should we. Encouraging a culture of continuous learning and improvement is crucial in DevOps. This might involve regular knowledge sharing sessions, attending conferences, or participating in online courses.
Embracing Failure: In a DevOps culture, failure is seen as an opportunity to learn and improve, not something to be feared. By implementing practices like blameless post-mortems, we can turn incidents into valuable learning experiences for the entire team.
The Future of DevOps: AI and Machine Learning
As we look to the future, the integration of AI and machine learning into DevOps practices promises to take automation to the next level. From predictive analytics for system performance to automated incident response, the possibilities are exciting.
AIOps: AI-Powered Operations: AIOps platforms use machine learning and big data analytics to automate IT operations processes, including event correlation, anomaly detection, and causality determination. This can help teams identify and resolve issues faster, often before they impact users.
Predictive Analytics: By analyzing historical data, machine learning models can predict future system behavior, allowing teams to proactively address potential issues. For example, we might use a simple Python script with scikit-learn to predict server load:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
import pandas as pd
# Load historical data
data = pd.read_csv('server_load.csv')
# Prepare features and target
X = data[['time_of_day', 'day_of_week', 'active_users']]
y = data['server_load']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Train model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Use predictions to proactively scale resources
# ...
This simple example demonstrates how we might use machine learning to predict server load and proactively scale resources.
Embracing the Automation Nation
As we’ve explored in this journey through the Automation Nation, DevOps and automation have the power to transform how we build, deploy, and maintain software. From CI/CD pipelines to infrastructure as code, from containerization to AI-powered operations, the tools and practices of DevOps offer a path to greater efficiency, reliability, and innovation.
But remember, DevOps is not just about tools or processes. It’s a mindset, a culture of collaboration and continuous improvement. As you embark on your own DevOps journey, keep in mind that the goal is not just to automate for the sake of automation, but to create a more efficient, responsive, and enjoyable work environment for everyone involved in the software development lifecycle.
So, are you ready to join the Automation Nation? The future of software development is here, and it’s automated, collaborative, and continuously improving. Welcome aboard!
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, technologies and best practices in the DevOps field are constantly evolving. Always refer to official documentation and consult with experts when implementing DevOps practices in your organization. If you notice any inaccuracies in this post, please report them so we can correct them promptly.