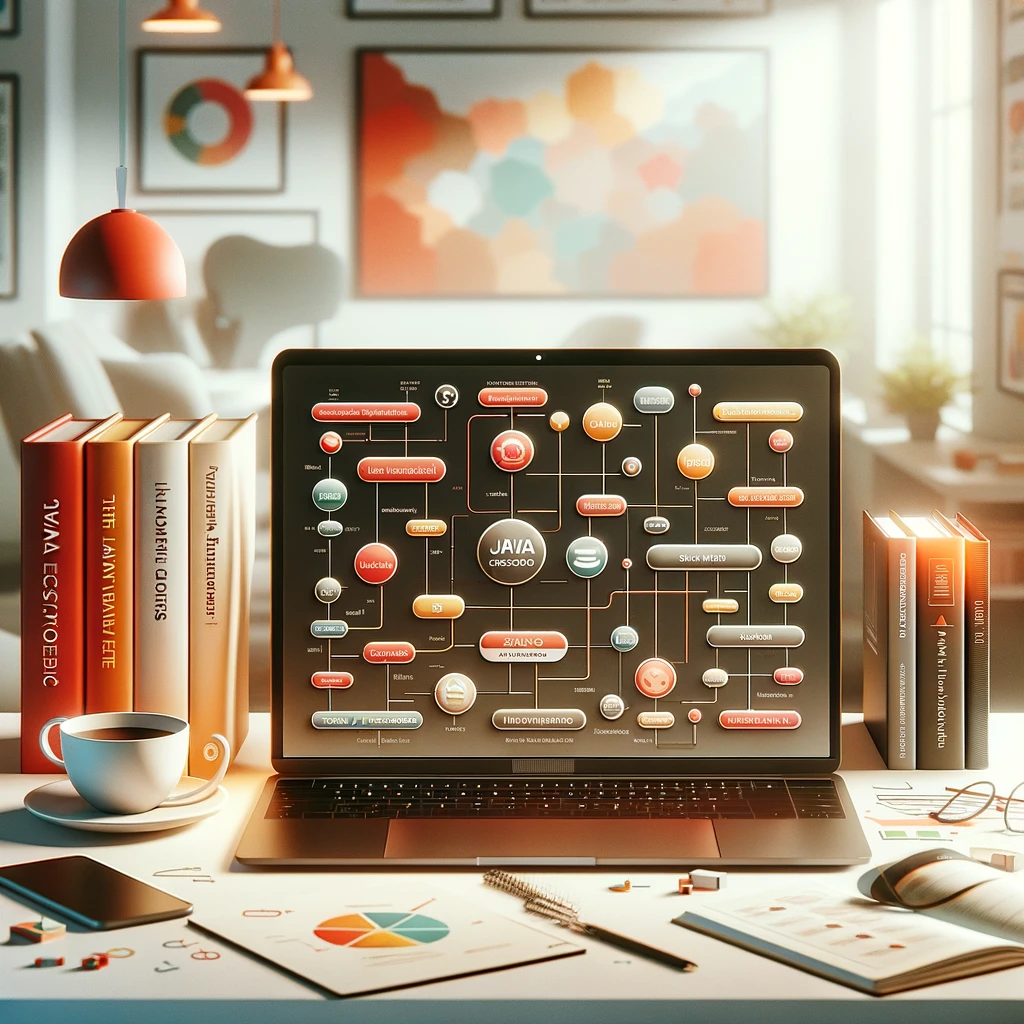
The Java Ecosystem: Libraries, Frameworks, and Tools – A Developer’s Guide
Welcome, fellow developers and curious minds! Today, we’re diving deep into the rich and expansive ecosystem that surrounds one of the most popular programming languages in the world: Java. If you’ve ever dipped your toes into the world of software development, chances are you’ve heard of Java. But did you know that Java is so much more than just a programming language? It’s a thriving ecosystem filled with libraries, frameworks, and tools that can make your life as a developer easier, more productive, and dare I say, even more enjoyable. In this blog post, we’ll explore this vast landscape, uncovering the gems that make Java such a powerful and versatile platform for building everything from simple desktop applications to complex enterprise systems. Whether you’re a seasoned Java pro or just starting your coding journey, there’s something here for everyone. So, grab your favorite beverage, get comfortable, and let’s embark on this exciting adventure through the Java ecosystem!
The Foundation: Java Development Kit (JDK)
Before we dive into the exciting world of libraries and frameworks, let’s start with the bedrock of the Java ecosystem: the Java Development Kit, or JDK. Think of the JDK as your trusty Swiss Army knife – it’s the essential toolkit that every Java developer needs to have in their arsenal. The JDK includes the Java Runtime Environment (JRE), which allows you to run Java applications, as well as development tools like the Java compiler (javac) and debugger (jdb). But that’s not all! It also comes packed with a treasure trove of core libraries that provide fundamental functionality for tasks like input/output operations, networking, and data structures.
Getting Started with the JDK
Setting up your development environment with the JDK is your first step into the world of Java. Here’s a quick example of how you can check your JDK version and compile a simple Java program:
public class HelloJavaEcosystem {
public static void main(String[] args) {
System.out.println("Welcome to the Java Ecosystem!");
}
}
To compile and run this program, you’d use the following commands in your terminal:
javac HelloJavaEcosystem.java
java HelloJavaEcosystem
This simple example barely scratches the surface of what you can do with the JDK, but it’s a great starting point for your Java journey. As we progress through this blog post, you’ll see how the JDK serves as the foundation for more advanced libraries and frameworks.
Building Blocks: Essential Java Libraries
Now that we’ve got our feet wet with the JDK, let’s explore some of the essential libraries that form the building blocks of many Java applications. These libraries are like Lego pieces – they provide pre-built functionality that you can use to construct more complex systems without reinventing the wheel.
Apache Commons: Your Swiss Army Knife
One of the most popular and versatile collections of libraries in the Java world is Apache Commons. It’s like having a Swiss Army knife in your code – there’s a tool for almost everything! Apache Commons is divided into several modules, each focusing on a specific area of functionality. Let’s take a look at a few examples:
- Commons Lang: This module provides utility classes for Java’s core classes, making it easier to work with strings, numbers, and objects. Here’s a quick example of how you can use StringUtils to check if a string is empty:
import org.apache.commons.lang3.StringUtils;
public class StringExample {
public static void main(String[] args) {
String text = "";
if (StringUtils.isEmpty(text)) {
System.out.println("The string is empty!");
}
}
}
- Commons IO: When it comes to input/output operations, Commons IO has got you covered. It simplifies file handling, making it a breeze to read, write, and manipulate files. Here’s how you can easily read the contents of a file:
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
public class FileExample {
public static void main(String[] args) {
try {
String content = FileUtils.readFileToString(new File("example.txt"), "UTF-8");
System.out.println("File contents: " + content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
- Commons Collections: This module extends Java’s collection framework, providing new interfaces, implementations, and utilities. One particularly useful class is MultiValueMap, which allows you to associate multiple values with a single key:
import org.apache.commons.collections4.MultiValuedMap;
import org.apache.commons.collections4.multimap.ArrayListValuedHashMap;
public class MultiMapExample {
public static void main(String[] args) {
MultiValuedMap<String, String> map = new ArrayListValuedHashMap<>();
map.put("fruits", "apple");
map.put("fruits", "banana");
map.put("vegetables", "carrot");
System.out.println("Fruits: " + map.get("fruits"));
System.out.println("Vegetables: " + map.get("vegetables"));
}
}
These examples just scratch the surface of what Apache Commons can do. As you explore more of its modules, you’ll find tools for everything from math operations to configuration management.
Google Guava: Enhancing Java’s Core
Another powerhouse in the Java library world is Google Guava. Developed by Google, this library aims to fill in the gaps in Java’s standard libraries and provide additional utilities that make coding more efficient and less error-prone. Let’s look at a few areas where Guava shines:
- Collections: Guava extends Java’s collection framework with new collection types and utility methods. For example, the ImmutableList class allows you to create unmodifiable lists easily:
import com.google.common.collect.ImmutableList;
public class GuavaCollectionsExample {
public static void main(String[] args) {
ImmutableList<String> list = ImmutableList.of("apple", "banana", "cherry");
System.out.println("Fruits: " + list);
// Attempting to modify the list will throw an UnsupportedOperationException
// list.add("date"); // This would cause an error
}
}
- Preconditions: Guava’s Preconditions class provides methods for checking method parameters and state. This can help you catch errors early and make your code more robust:
import com.google.common.base.Preconditions;
public class PreconditionsExample {
public static void divide(int a, int b) {
Preconditions.checkArgument(b != 0, "Divisor cannot be zero");
System.out.println("Result: " + (a / b));
}
public static void main(String[] args) {
divide(10, 2); // Works fine
divide(10, 0); // Throws IllegalArgumentException
}
}
- Caching: Guava provides a powerful caching mechanism that can help improve the performance of your applications. Here’s a simple example of how to create and use a cache:
import com.google.common.cache.Cache;
import com.google.common.cache.CacheBuilder;
import java.util.concurrent.TimeUnit;
public class CacheExample {
public static void main(String[] args) {
Cache<String, String> cache = CacheBuilder.newBuilder()
.maximumSize(100)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
cache.put("key", "value");
String result = cache.getIfPresent("key");
System.out.println("Cached value: " + result);
}
}
These libraries are just the tip of the iceberg when it comes to the Java ecosystem. As you become more comfortable with these building blocks, you’ll find yourself reaching for them frequently to solve common programming challenges.
Web Development: Frameworks and Tools
Now that we’ve covered some essential libraries, let’s shift gears and explore the exciting world of web development in Java. The Java ecosystem offers a rich selection of frameworks and tools that can help you build robust, scalable web applications. Whether you’re creating a simple REST API or a complex enterprise system, there’s a framework out there to suit your needs.
Spring Framework: The Swiss Army Knife of Java Web Development
When it comes to Java web development, the Spring Framework is often the first name that comes to mind. Spring is like a Swiss Army knife for Java developers – it provides a comprehensive programming and configuration model for modern Java-based enterprise applications. Let’s look at some key features of Spring:
- Dependency Injection: Spring’s core feature is its Inversion of Control (IoC) container, which manages the lifecycle of Java objects and their dependencies. Here’s a simple example of how you can use dependency injection in Spring:
import org.springframework.stereotype.Component;
import org.springframework.beans.factory.annotation.Autowired;
@Component
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User findUser(Long id) {
return userRepository.findById(id);
}
}
- Spring Boot: Spring Boot is a project within the Spring ecosystem that makes it easy to create stand-alone, production-grade Spring-based applications. It takes an opinionated view of the Spring platform, which allows you to get started with minimum fuss. Here’s a simple Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class HelloWorldApplication {
@GetMapping("/")
public String hello() {
return "Hello, Spring Boot!";
}
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
}
- Spring Data: This module of Spring makes it easy to work with different data stores, from relational databases to NoSQL stores. Here’s an example of how you can define a repository interface using Spring Data JPA:
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
With just this interface, Spring Data JPA will automatically provide implementations for common CRUD operations and the custom findByUsername
method.
Jakarta EE: The Enterprise Standard
While Spring is incredibly popular, it’s not the only player in the Java web development space. Jakarta EE (formerly known as Java EE) is the official standard for enterprise Java development. It provides a set of specifications that define a comprehensive platform for developing and running enterprise applications. Let’s look at a few key components of Jakarta EE:
- Servlets: These are the foundation of web development in Jakarta EE. Here’s a simple servlet example:
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/hello")
public class HelloServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
response.getWriter().println("<h1>Hello, Jakarta EE!</h1>");
}
}
- JavaServer Faces (JSF): This is a component-based UI framework for building web applications. Here’s a simple JSF page:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:head>
<title>Hello JSF</title>
</h:head>
<h:body>
<h:form>
<h:inputText value="#{helloBean.name}"/>
<h:commandButton value="Say Hello" action="#{helloBean.sayHello}"/>
<h:outputText value="#{helloBean.message}"/>
</h:form>
</h:body>
</html>
- Java Persistence API (JPA): This provides a standard way to manage relational data in Java applications. Here’s an example of a JPA entity:
import jakarta.persistence.Entity;
import jakarta.persistence.Id;
import jakarta.persistence.GeneratedValue;
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
private String username;
private String email;
// Getters and setters...
}
These frameworks and tools represent just a fraction of what’s available for web development in the Java ecosystem. As you explore further, you’ll discover additional frameworks like Play Framework, Micronaut, and Quarkus, each with its own strengths and use cases.
Data Persistence: ORM and Database Tools
In the world of software development, data is king. And when it comes to working with data in Java applications, the ecosystem provides a wealth of tools and libraries to make your life easier. Let’s explore some of the key players in the realm of data persistence.
Hibernate: The ORM Powerhouse
Hibernate is arguably the most popular Object-Relational Mapping (ORM) tool in the Java world. It allows you to work with relational databases using Java objects, effectively bridging the gap between object-oriented programming and relational database management systems. Here’s a taste of what you can do with Hibernate:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateExample {
public static void main(String[] args) {
SessionFactory factory = new Configuration()
.configure("hibernate.cfg.xml")
.addAnnotatedClass(User.class)
.buildSessionFactory();
try (Session session = factory.getCurrentSession()) {
session.beginTransaction();
// Create a new user
User user = new User("johndoe", "john@example.com");
session.save(user);
// Retrieve the user
User retrievedUser = session.get(User.class, user.getId());
System.out.println("Retrieved user: " + retrievedUser.getUsername());
session.getTransaction().commit();
}
}
}
In this example, we’re using Hibernate to save a new User object to the database and then retrieve it. Hibernate takes care of all the SQL generation and object mapping behind the scenes, allowing you to focus on your business logic rather than database interactions.
MyBatis: SQL Mapper with a Twist
While ORMs like Hibernate are great for many use cases, sometimes you need more control over your SQL. This is where MyBatis shines. It’s a SQL mapper framework that gives you full control over your database queries while still providing the convenience of mapping results to Java objects. Here’s a quick example:
// In your XML mapper file (UserMapper.xml)
<mapper namespace="com.example.UserMapper">
<select id="getUserById" resultType="com.example.User">
SELECT id, username, email FROM users WHERE id = #{id}
</select>
</mapper>
// In your Java code
public interface UserMapper {
User getUserById(int id);
}
public class MyBatisExample {
public static void main(String[] args) {
SqlSession session = sqlSessionFactory.openSession();
try {
UserMapper mapper = session.getMapper(UserMapper.class);
User user = mapper.getUserById(1);
System.out.println("User: " + user.getUsername());
} finally {
session.close();
}
}
}
In this example, we define our SQL query in an XML file and use MyBatis to execute it and map the results to a User object. This approach gives you the flexibility to write complex SQL queries while still benefiting from object mapping.
jOOQ: Type-Safe SQL in Java
For those who love the power of SQL but want the safety of compile-time checks, jOOQ (Java Object Oriented Querying) offers a unique solution. It allows you to write SQL queries using a fluent Java API, providing type safety and autocompletion in your IDE. Here’s what it looks like in action:
import static org.jooq.impl.DSL.*;
import org.jooq.DSLContext;
import org.jooq.Record;
import org.jooq.Result;
public class JooqExample {
public static void main(String[] args) {
DSLContext create = DSL.using(connection, SQLDialect.MYSQL);
Result result = create.select()
.from(table("users"))
.where(field("username").eq("johndoe"))
.fetch();
for (Record r : result) {
Integer id = r.getValue(field("id", Integer.class));
String username = r.getValue(field("username", String.class));
System.out.println("User " + id + ": " + username);
}
}
}
With jOOQ, you get the best of both worlds: the power and flexibility of SQL, combined with the type safety and refactoring support of Java. It’s particularly useful for complex queries and for teams that prefer to work closely with their database schema.
Testing: Ensuring Quality in Your Java Applications
No discussion of the Java ecosystem would be complete without mentioning testing. Java has a rich set of tools and frameworks for testing, allowing you to ensure the quality and reliability of your code. Let’s explore some of the most popular options.
JUnit: The Testing Backbone
JUnit is the de facto standard for unit testing in Java. It provides a simple and elegant way to write and run tests. Here’s a basic example of a JUnit 5 test:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3), "2 + 3 should equal 5");
}
@Test
public void testDivision() {
Calculator calc = new Calculator();
assertThrows(ArithmeticException.class, () -> calc.divide(1, 0),
"Division by zero should throw ArithmeticException");
}
}
JUnit 5 introduces a number of new features, including support for lambda expressions, improved assertions, and better integration with IDEs and build tools.
Mockito: Mocking Made Easy
When unit testing, you often need to isolate the code you’re testing from its dependencies. This is where mocking frameworks like Mockito come in handy. Mockito allows you to create mock objects easily, set expectations, and verify behavior. Here’s a quick example:
import static org.mockito.Mockito.; import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*;
public class UserServiceTest {
@Test
public void testFindUser() {
// Create a mock UserRepository
UserRepository mockRepository = mock(UserRepository.class);
// Set up the mock behavior
when(mockRepository.findById(1L)).thenReturn(new User(1L, "John Doe"));
// Create the service with the mock repository
UserService userService = new UserService(mockRepository);
// Test the service
User user = userService.findUser(1L);
assertNotNull(user);
assertEquals("John Doe", user.getName());
// Verify that the mock method was called
verify(mockRepository).findById(1L);
}
}
Selenium: Web Application Testing
For testing web applications, Selenium is a popular choice. It allows you to automate browser interactions, making it possible to test your web application’s UI and functionality. Here’s a simple example of using Selenium with Java:
}
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class WebAppTest {
@Test
public void testLoginPage() {
WebDriver driver = new ChromeDriver();
try {
driver.get("http://example.com/login");
WebElement usernameField = driver.findElement(By.id("username"));
WebElement passwordField = driver.findElement(By.id("password"));
WebElement loginButton = driver.findElement(By.id("login-button"));
usernameField.sendKeys("testuser");
passwordField.sendKeys("password123");
loginButton.click();
// Wait for the page to load and check for successful login
WebElement welcomeMessage = driver.findElement(By.id("welcome-message"));
assertTrue(welcomeMessage.isDisplayed());
assertEquals("Welcome, testuser!", welcomeMessage.getText());
} finally {
driver.quit();
}
}
}
Build Tools and Dependency Management
In the Java ecosystem, managing your project’s build process and dependencies is crucial. Two of the most popular tools for this purpose are Maven and Gradle.
Maven: Convention Over Configuration
Maven is a powerful project management tool that embraces the principle of “Convention over Configuration”. It provides a standard way to build projects, manage dependencies, and share information. Here’s what a simple Maven `pom.xml` file might look like:
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
</dependencies>
Gradle: Flexible and Powerful
Gradle is a more flexible build tool that uses a Groovy or Kotlin-based DSL for defining builds. It’s known for its performance and extensibility. Here’s an example of a simple Gradle build file (`build.gradle`):
groovy
plugins {
id 'java'
}
group 'com.example'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.8.1'
testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.8.1'
}
test {
useJUnitPlatform()
}
Both Maven and Gradle handle dependency management, project building, testing, and deployment. The choice between them often comes down to personal preference and project requirements.
Embracing the Java Ecosystem
As we’ve seen throughout this journey, the Java ecosystem is vast and varied. From essential libraries like Apache Commons and Google Guava to powerful frameworks like Spring and Jakarta EE, from ORM tools like Hibernate to SQL mappers like MyBatis and jOOQ, and from testing frameworks like JUnit and Mockito to build tools like Maven and Gradle – there’s a tool or library for almost every conceivable task in Java development.
The beauty of this ecosystem lies in its diversity and interoperability. You can mix and match different libraries and frameworks to create the perfect stack for your project. Want to use Spring Boot with MyBatis for data access and JUnit with Mockito for testing? Go for it! Prefer Jakarta EE with Hibernate and Selenium for your web application testing? That’s a great choice too!
As you continue your journey in the Java world, don’t be afraid to explore and experiment with different tools and libraries. The Java ecosystem is constantly evolving, with new projects emerging and existing ones improving. Stay curious, keep learning, and most importantly, enjoy the process of building amazing things with Java!
Remember, the tools and libraries we’ve discussed here are just the tip of the iceberg. There’s always more to discover in the Java ecosystem. So keep coding, keep exploring, and keep pushing the boundaries of what you can create with Java!
Disclaimer: While every effort has been made to ensure the accuracy of the information in this blog post, the Java ecosystem is vast and constantly evolving. Some details may become outdated over time. We encourage readers to consult official documentation for the most up-to-date information on specific libraries and tools. If you notice any inaccuracies, please report them so we can correct them promptly.