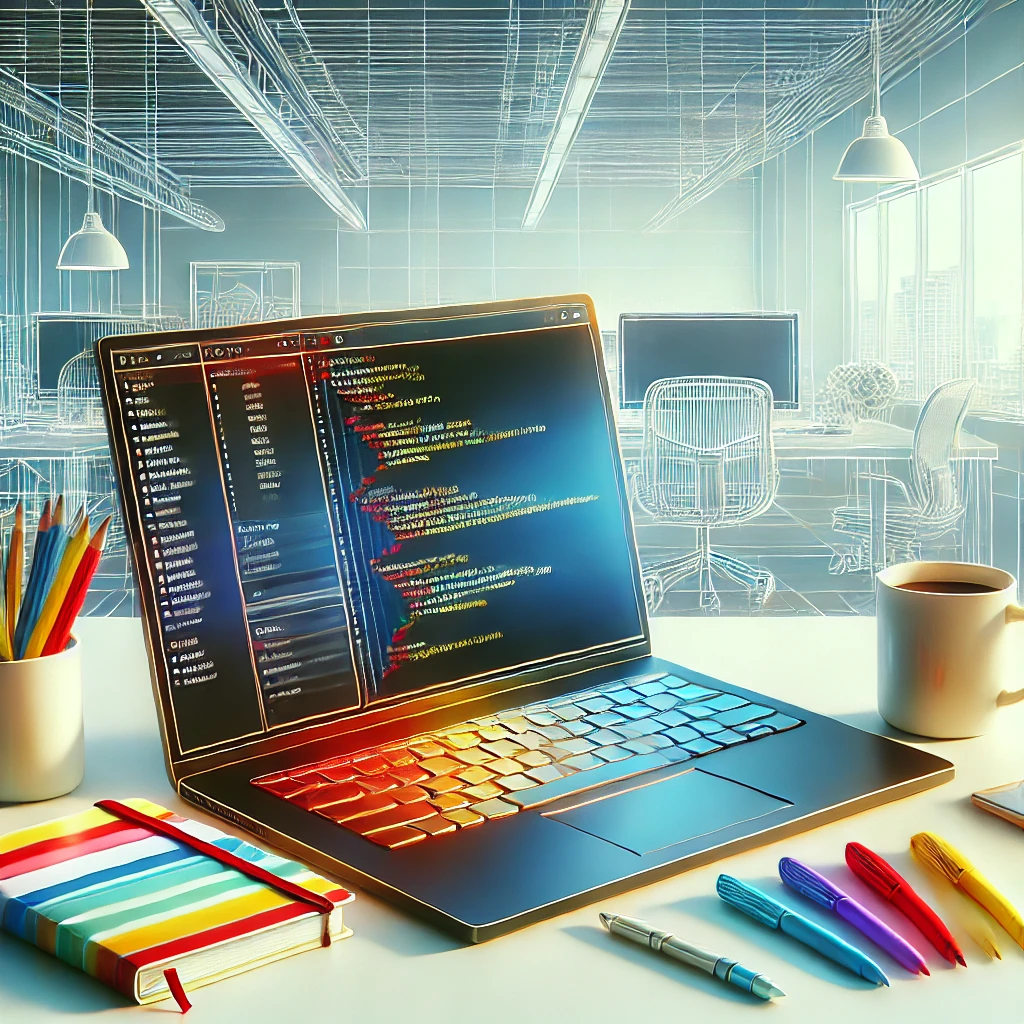
Java for Web Development
Have you ever wondered how some of the most robust and scalable web applications are built? Well, you’re in for a treat! Today, we’re diving into the exciting realm of Java web development. Whether you’re a curious beginner or a seasoned developer looking to expand your toolkit, this blog post will take you on a journey through the fundamentals of using Java for creating dynamic and powerful web applications.
Java has been a cornerstone of enterprise-level web development for decades, and for good reason. Its “write once, run anywhere” philosophy, coupled with its robust ecosystem and unparalleled scalability, makes it a go-to choice for developers worldwide. But don’t worry if these terms sound a bit overwhelming – we’ll break everything down into bite-sized, easy-to-digest pieces.
In this comprehensive guide, we’ll explore why Java continues to be a popular choice for web development, delve into the essential tools and frameworks that make Java web development a breeze, and even get our hands dirty with some code examples. So, grab your favorite caffeinated beverage (java, perhaps?), and let’s embark on this exciting journey into the world of Java web development!
Why Choose Java for Web Development?
Before we dive into the nitty-gritty details, let’s address the elephant in the room: Why should you consider Java for web development when there are so many other options out there? It’s a fair question, and the answer lies in Java’s unique combination of features and benefits that make it particularly well-suited for creating web applications.
First and foremost, Java’s platform independence is a game-changer. The famous slogan “Write Once, Run Anywhere” (WORA) isn’t just a catchy phrase – it’s a fundamental principle that sets Java apart. When you develop a Java application, you can run it on any platform that supports the Java Virtual Machine (JVM), whether it’s Windows, macOS, Linux, or even some mobile devices. This cross-platform compatibility is a massive advantage in our increasingly diverse technological landscape.
But that’s just the tip of the iceberg. Java’s robustness and scalability make it an ideal choice for enterprise-level applications. Large corporations and government institutions often choose Java for their mission-critical systems because of its ability to handle heavy loads and scale seamlessly. The language’s strong typing and exception handling mechanisms also contribute to creating more stable and secure applications – a crucial factor in today’s cyber-security-conscious world.
Moreover, Java boasts an extensive ecosystem of libraries and frameworks that can significantly speed up your development process. Whether you need to handle database operations, implement complex business logic, or create responsive user interfaces, chances are there’s a Java library or framework that can help you do it efficiently. This rich ecosystem, combined with excellent documentation and a supportive community, makes Java a developer-friendly choice for web development.
Getting Started: Setting Up Your Java Web Development Environment
Now that we’ve piqued your interest in Java web development, let’s roll up our sleeves and get our development environment set up. Don’t worry – it’s not as daunting as it might sound. We’ll walk through this process step-by-step, ensuring you have everything you need to start coding your Java web applications.
Step 1: Install Java Development Kit (JDK)
The first thing you’ll need is the Java Development Kit (JDK). The JDK includes the Java Runtime Environment (JRE), which is necessary to run Java applications, as well as development tools like the Java compiler. Head over to the official Oracle website or adopt OpenJDK, download the latest version of the JDK for your operating system, and follow the installation instructions.
Step 2: Choose an Integrated Development Environment (IDE)
While you can write Java code in any text editor, using an Integrated Development Environment (IDE) can significantly boost your productivity. Popular choices for Java development include:
- Eclipse: A free, open-source IDE with a wide range of plugins
- IntelliJ IDEA: Offers both free (Community) and paid (Ultimate) versions with powerful features
- NetBeans: Another free, open-source IDE that’s particularly good for beginners
Choose the one that feels most comfortable for you. For this guide, we’ll use IntelliJ IDEA, but the concepts will be applicable regardless of your chosen IDE.
Step 3: Set Up a Web Server
For Java web development, you’ll need a web server to run and test your applications. Apache Tomcat is a popular choice due to its simplicity and robust features. Download Tomcat from the official Apache website and follow the installation guide for your operating system.
Step 4: Configure Your IDE for Web Development
Most modern IDEs make it easy to set up web development projects. In IntelliJ IDEA, for example, you can create a new project and select “Java Enterprise” as the project type. This will guide you through setting up your web application structure and connecting it to your Tomcat server.
With these steps completed, you’re ready to start your Java web development journey! Let’s dive into some code to get a feel for what Java web development looks like in practice.
Your First Java Web Application: Hello, World!
What better way to start our journey than with the classic “Hello, World!” example? But this time, we’re going to give it a web twist. We’ll create a simple servlet that displays “Hello, World!” in a web browser. This will give you a taste of how Java can be used to generate dynamic web content.
First, let’s create a new Java class called HelloWorldServlet
. This class will extend HttpServlet
, which is part of the Java Servlet API:
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class HelloWorldServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head><title>Hello, World!</title></head>");
out.println("<body>");
out.println("<h1>Hello, World!</h1>");
out.println("</body></html>");
}
}
This servlet overrides the doGet
method, which is called when a GET request is made to the servlet. It sets the content type to HTML and uses a PrintWriter
to output a simple HTML page with the message “Hello, World!”.
Next, we need to configure our web application to use this servlet. In a file called web.xml
(usually located in the WEB-INF
directory), add the following:
<web-app>
<servlet>
<servlet-name>HelloWorld</servlet-name>
<servlet-class>HelloWorldServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloWorld</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
</web-app>
This configuration tells the web server to map requests to the /hello
URL to our HelloWorldServlet
.
Now, when you deploy this application to your Tomcat server and navigate to http://localhost:8080/your-app-name/hello
in your web browser, you should see the “Hello, World!” message displayed.
Congratulations! You’ve just created your first Java web application. It may seem simple, but this example demonstrates some key concepts in Java web development, including:
- Using servlets to handle HTTP requests
- Generating dynamic HTML content
- Configuring URL mappings for your web application
As we progress through this guide, we’ll build on these concepts to create more complex and interactive web applications. But for now, take a moment to bask in the glory of your first step into the world of Java web development!
Certainly! I’ll continue with the rest of the blog post, picking up where we left off:
Understanding Java Web Development Frameworks
Now that we’ve dipped our toes into Java web development with a simple servlet, let’s explore some of the frameworks that make building complex web applications more manageable. Java’s ecosystem is rich with frameworks that can significantly streamline your development process, each with its own strengths and use cases.
Spring Framework
The Spring Framework is arguably the most popular Java framework for building enterprise-level web applications. It provides a comprehensive programming and configuration model for modern Java-based enterprise applications. Let’s look at a simple example of how Spring can simplify our “Hello, World!” application:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloWorldController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
In this Spring example, we’ve achieved the same result as our servlet with much less code. The @RestController
annotation tells Spring that this class is a controller that handles web requests, and the @GetMapping("/hello")
annotation maps GET requests to the “/hello” URL to our hello()
method.
Spring’s dependency injection and inversion of control principles make it easier to manage complex applications and promote loose coupling between components. It also provides excellent support for building RESTful web services, which are crucial in modern web development.
JavaServer Faces (JSF)
JavaServer Faces is a component-based framework for building user interfaces for web applications. It’s particularly useful for creating dynamic and interactive web pages. Here’s a simple JSF example:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:head>
<title>Hello World JSF</title>
</h:head>
<h:body>
<h:form>
<h:inputText value="#{helloBean.name}"></h:inputText>
<h:commandButton value="Say Hello" action="#{helloBean.sayHello}"></h:commandButton>
<h:outputText value="#{helloBean.message}"></h:outputText>
</h:form>
</h:body>
</html>
This JSF page creates a simple form with an input field, a button, and an output area. The #{helloBean.name}
syntax binds the input to a property in a Java bean, allowing for easy data handling and manipulation.
Apache Struts
Apache Struts is another popular framework for developing Java web applications. It follows the Model-View-Controller (MVC) design pattern, which helps in organizing your code for better maintainability. Here’s a basic Struts action:
public class HelloWorldAction extends ActionSupport {
private String message;
public String execute() {
setMessage("Hello, World!");
return SUCCESS;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
In Struts, actions handle the business logic, and the execute()
method is called when the action is invoked. The return value (SUCCESS
in this case) determines which view should be displayed next.
Handling Data: Java Database Connectivity (JDBC)
No web application is complete without data persistence. Java Database Connectivity (JDBC) is the Java API for connecting and executing queries with a database. Let’s look at a simple example of how to use JDBC to interact with a database:
import java.sql.*;
public class JDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "username";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM users")) {
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String email = rs.getString("email");
System.out.println(id + ", " + name + ", " + email);
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
This example demonstrates how to connect to a MySQL database, execute a SELECT query, and process the results. JDBC provides a consistent way to interact with different types of databases, making it easier to switch between database systems if needed.
Building RESTful Web Services with Java
In modern web development, RESTful web services play a crucial role in creating scalable and interoperable applications. Java provides excellent support for building RESTful services, especially when combined with frameworks like Spring Boot. Let’s create a simple RESTful service:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.*;
@SpringBootApplication
@RestController
public class UserService {
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
// In a real application, you would fetch this from a database
return new User(id, "John Doe", "john@example.com");
}
@PostMapping("/users")
public User createUser(@RequestBody User user) {
// In a real application, you would save this to a database
System.out.println("Creating user: " + user);
return user;
}
public static void main(String[] args) {
SpringApplication.run(UserService.class, args);
}
}
class User {
private Long id;
private String name;
private String email;
// Constructor, getters, and setters omitted for brevity
}
This example creates a simple RESTful service with two endpoints: one for getting a user by ID, and another for creating a new user. Spring Boot takes care of a lot of the boilerplate code, allowing you to focus on your business logic.
Securing Your Java Web Applications
Security is a critical aspect of web development. Java provides robust security features, and frameworks like Spring Security make it easier to implement authentication and authorization in your web applications. Here’s a basic example of how to secure a Spring Boot application:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.provisioning.InMemoryUserDetailsManager;
import org.springframework.security.web.SecurityFilterChain;
@Configuration
@EnableWebSecurity
public class SecurityConfig {
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
return http.build();
}
@Bean
public UserDetailsService userDetailsService() {
UserDetails user =
User.withDefaultPasswordEncoder()
.username("user")
.password("password")
.roles("USER")
.build();
return new InMemoryUserDetailsManager(user);
}
}
This configuration sets up basic authentication for a Spring Boot application. It allows unauthenticated access to the home page, but requires authentication for all other pages. It also sets up a simple in-memory user store with one user.
Testing Your Java Web Applications
Testing is an essential part of the development process, and Java provides excellent tools for testing web applications. JUnit is the most widely used testing framework for Java, and when combined with tools like Mockito for mocking dependencies, it becomes a powerful toolset for ensuring the quality of your web applications.
Here’s a simple example of a JUnit test for a Spring Boot controller:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.test.web.servlet.MockMvc;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.content;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@WebMvcTest(HelloWorldController.class)
public class HelloWorldControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testHelloEndpoint() throws Exception {
mockMvc.perform(get("/hello"))
.andExpect(status().isOk())
.andExpect(content().string("Hello, World!"));
}
}
This test uses Spring’s MockMvc to simulate HTTP requests to our controller, allowing us to test our web application without actually starting a web server.
Deploying Your Java Web Application
Once you’ve developed and tested your Java web application, the final step is deployment. There are several ways to deploy Java web applications, depending on your specific needs and infrastructure. Here are a few common options:
- Traditional Application Servers: You can deploy your application to a Java application server like Apache Tomcat, JBoss, or GlassFish. These servers provide a runtime environment for your Java web applications.
- Cloud Platforms: Many cloud platforms support Java web applications. For example, you can deploy Spring Boot applications to platforms like Heroku, Google Cloud Platform, or Amazon Web Services with minimal configuration.
- Docker Containers: Containerization has become increasingly popular for deploying applications. You can package your Java web application into a Docker container, which can then be deployed to any environment that supports Docker.
Here’s a simple Dockerfile for a Spring Boot application:
FROM openjdk:11-jre-slim
COPY target/my-app.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
This Dockerfile creates an image based on the OpenJDK 11 JRE, copies your application JAR file into the image, and sets up the command to run your application when a container is started from this image.
The Power of Java for Web Development
As we’ve explored in this comprehensive guide, Java offers a robust and versatile platform for web development. From its cross-platform compatibility and extensive ecosystem to its powerful frameworks and excellent support for enterprise-level applications, Java continues to be a top choice for developers worldwide.
We’ve only scratched the surface of what’s possible with Java web development. As you continue your journey, you’ll discover even more tools, frameworks, and techniques that can help you build sophisticated, scalable, and secure web applications.
Remember, the key to mastering Java web development is practice. Start with small projects, experiment with different frameworks, and don’t be afraid to dig into the documentation. With time and experience, you’ll be crafting complex web applications with ease.
So, are you ready to harness the power of Java for your next web development project? The possibilities are endless, and the journey promises to be as rewarding as it is challenging. Happy coding!
Disclaimer: This blog post is intended for educational purposes only. While we strive for accuracy, technology evolves rapidly, and some information may become outdated over time. Always refer to official documentation and stay updated with the latest developments in Java and web development. If you notice any inaccuracies, please report them so we can correct them promptly.