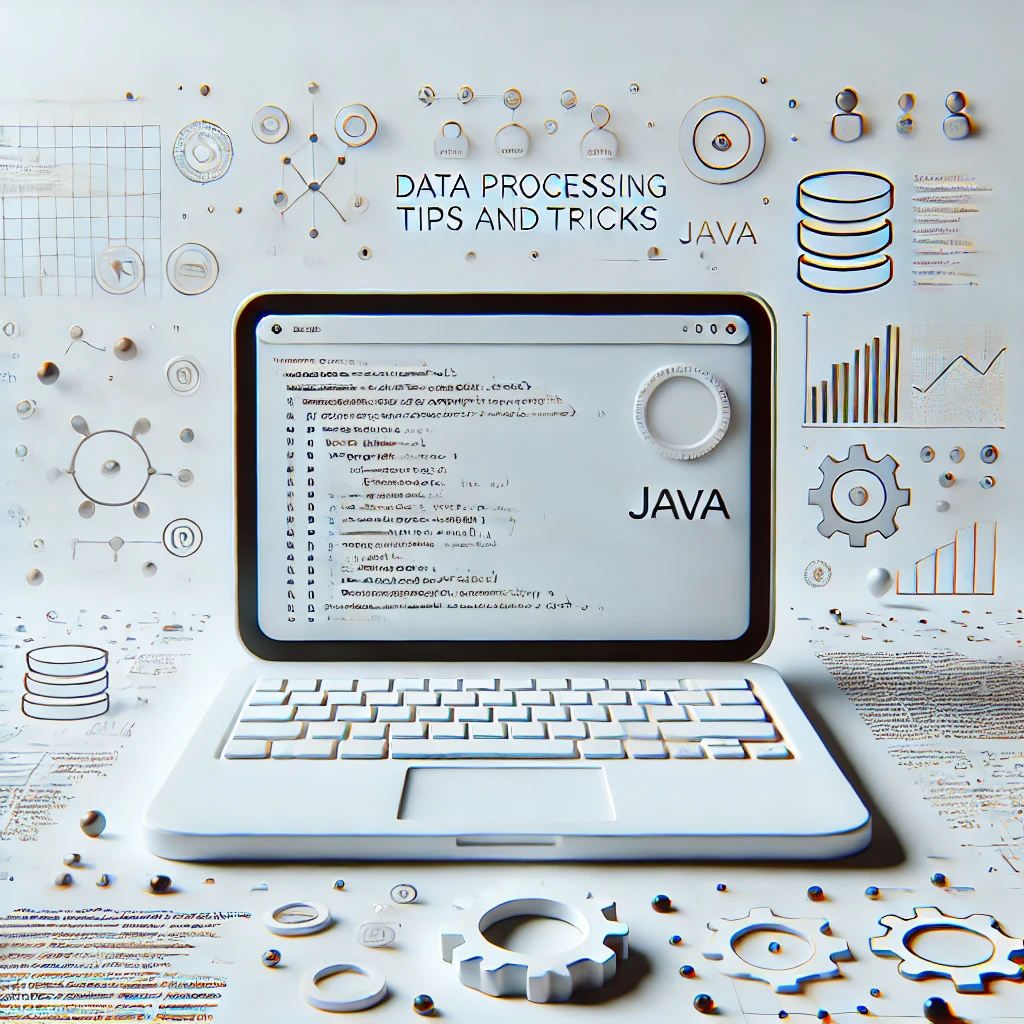
Data Processing with Java: Tips and Tricks for Efficient Handling of Big Data.
In today’s data-driven world, the ability to efficiently process and analyze large volumes of information is more crucial than ever. Enter Java, a versatile and robust programming language that has stood the test of time. With its rich ecosystem of libraries and frameworks, Java offers powerful tools for tackling even the most complex data processing challenges. Whether you’re a seasoned developer or just starting your journey in the world of big data, this blog will equip you with valuable tips and tricks to supercharge your Java-based data processing projects. We’ll explore everything from basic concepts to advanced techniques, all while keeping things practical and engaging. So, grab your favorite beverage, fire up your IDE, and let’s dive into the fascinating world of data processing with Java!
Understanding the Basics: Java’s Data Processing Foundations
Before we jump into the nitty-gritty of advanced techniques, let’s take a moment to appreciate the solid foundation Java provides for data processing. At its core, Java offers a rich set of data structures and utility classes that form the bedrock of any data manipulation task. From the versatile ArrayList to the lightning-fast HashMap, Java’s collections framework provides a Swiss Army knife for developers dealing with data. But it’s not just about storing data – Java’s stream API, introduced in Java 8, revolutionized the way we think about data processing by enabling functional-style operations on streams of elements. This powerful feature allows for concise and expressive code, making complex data transformations a breeze. Let’s take a quick look at a simple example to illustrate the power of streams:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Eve");
names.stream()
.filter(name -> name.length() > 4)
.map(String::toUpperCase)
.forEach(System.out::println);
In this snippet, we’re filtering a list of names, transforming them to uppercase, and printing the results – all in a single, readable line of code. This is just a taste of what Java can do when it comes to data processing, and we’re only scratching the surface!
Harnessing the Power of Java Libraries for Data Processing
One of Java’s greatest strengths lies in its extensive ecosystem of libraries and frameworks. When it comes to data processing, there’s no need to reinvent the wheel – chances are, someone has already created a powerful tool to tackle your specific problem. Let’s explore some of the most popular and useful libraries that can take your data processing game to the next level.
Apache Commons CSV: Taming the CSV Beast
Comma-Separated Values (CSV) files are ubiquitous in the world of data, and parsing them efficiently can be a real headache. Enter Apache Commons CSV, a library that makes working with CSV files a breeze. Let’s look at a quick example of how to read a CSV file using this library:
try (Reader reader = new FileReader("data.csv");
CSVParser csvParser = new CSVParser(reader, CSVFormat.DEFAULT.withHeader())) {
for (CSVRecord record : csvParser) {
String name = record.get("Name");
int age = Integer.parseInt(record.get("Age"));
System.out.println(name + " is " + age + " years old.");
}
} catch (IOException e) {
e.printStackTrace();
}
With just a few lines of code, we’ve parsed a CSV file, extracted structured data, and processed it. Apache Commons CSV handles all the tricky bits like dealing with quoted fields and different CSV formats, allowing you to focus on what really matters – working with your data.
Jackson: JSON Wrangling Made Easy
In the age of web services and APIs, JSON has become the lingua franca of data exchange. Jackson, a high-performance JSON processor for Java, is your go-to library for all things JSON. Whether you’re parsing JSON responses from an API or serializing complex Java objects to JSON, Jackson has got you covered. Here’s a quick example of how to parse a JSON string and extract data:
ObjectMapper mapper = new ObjectMapper();
String json = "{\"name\":\"John Doe\",\"age\":30,\"city\":\"New York\"}";
JsonNode rootNode = mapper.readTree(json);
String name = rootNode.get("name").asText();
int age = rootNode.get("age").asInt();
System.out.println(name + " is " + age + " years old.");
With Jackson, you can easily navigate complex JSON structures, convert between JSON and Java objects, and even customize the serialization process to suit your needs. It’s an indispensable tool in any Java developer’s data processing toolkit.
Optimizing Performance: Tips for Handling Large Datasets
When it comes to processing big data, performance is key. Java’s “write once, run anywhere” philosophy doesn’t mean you have to sacrifice speed for portability. With the right techniques and a bit of know-how, you can make your Java data processing applications fly. Let’s explore some tips and tricks to optimize your code for handling large datasets.
Parallel Processing with Java Streams
Remember the Stream API we mentioned earlier? It’s not just for simple operations – it can also be a powerful tool for parallel processing. By leveraging multi-core processors, you can significantly speed up operations on large datasets. Here’s an example of how to use parallel streams to count the number of elements meeting a certain condition:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
long count = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.count();
System.out.println("Number of even numbers: " + count);
By simply changing stream()
to parallelStream()
, we’ve enabled parallel processing. Java takes care of splitting the work across multiple threads, potentially providing a significant performance boost for large datasets. However, it’s important to note that parallelization isn’t always faster – for small datasets or operations with significant overhead, sequential processing might actually be more efficient. As with all optimizations, measure and profile your code to ensure you’re actually getting the benefits you expect.
Memory Management: Dealing with Out-of-Memory Errors
When processing large datasets, one of the most common issues you might encounter is running out of memory. Java’s garbage collector is great, but it’s not magic – if you’re not careful, you can still exhaust your JVM’s heap space. Here are a few strategies to help manage memory effectively:
- Use streams and lazy evaluation: Instead of loading an entire dataset into memory, use streams to process data on-the-fly.
- Implement custom iterators: For very large datasets, implement your own iterator that loads data in chunks from a database or file.
- Consider memory-mapped files: For file-based data processing, memory-mapped files can provide efficient access to large files without loading them entirely into memory.
- Tune JVM parameters: Adjusting settings like the maximum heap size (-Xmx) can help accommodate larger datasets.
Here’s a simple example of using a custom iterator to process a large file line by line without loading it all into memory:
public class LargeFileIterator implements Iterator<String> {
private BufferedReader reader;
private String nextLine;
public LargeFileIterator(String filename) throws IOException {
this.reader = new BufferedReader(new FileReader(filename));
this.nextLine = reader.readLine();
}
@Override
public boolean hasNext() {
return nextLine != null;
}
@Override
public String next() {
String line = nextLine;
try {
nextLine = reader.readLine();
if (nextLine == null) {
reader.close();
}
} catch (IOException e) {
throw new RuntimeException(e);
}
return line;
}
}
// Usage:
LargeFileIterator iterator = new LargeFileIterator("large_file.txt");
while (iterator.hasNext()) {
String line = iterator.next();
// Process the line
}
This approach allows you to process files that are much larger than your available memory, as you’re only keeping one line in memory at a time.
Advanced Techniques: Leveraging Java for Complex Data Processing Tasks
Now that we’ve covered some foundational concepts and optimization techniques, let’s dive into more advanced territory. Java’s flexibility and power really shine when tackling complex data processing tasks. Whether you’re dealing with time series data, implementing machine learning algorithms, or processing streaming data in real-time, Java has the tools and libraries to get the job done.
Time Series Analysis with JFreeChart
Time series data is everywhere, from stock market trends to IoT sensor readings. JFreeChart is a powerful library for creating professional-quality charts in Java applications, and it’s particularly well-suited for visualizing time series data. Here’s a quick example of how to create a simple time series chart:
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.time.Day;
import org.jfree.data.time.TimeSeries;
import org.jfree.data.time.TimeSeriesCollection;
import javax.swing.JFrame;
import java.util.Random;
public class TimeSeriesExample extends JFrame {
public TimeSeriesExample(String title) {
super(title);
TimeSeries series = new TimeSeries("Random Data");
Random random = new Random();
Day current = new Day(1, 1, 2023);
for (int i = 0; i < 365; i++) {
series.add(current, random.nextDouble() * 100.0);
current = (Day) current.next();
}
TimeSeriesCollection dataset = new TimeSeriesCollection(series);
JFreeChart chart = ChartFactory.createTimeSeriesChart(
"Random Time Series Data",
"Date",
"Value",
dataset,
true,
true,
false
);
ChartPanel chartPanel = new ChartPanel(chart);
chartPanel.setPreferredSize(new java.awt.Dimension(800, 600));
setContentPane(chartPanel);
}
public static void main(String[] args) {
TimeSeriesExample demo = new TimeSeriesExample("Time Series Demo");
demo.pack();
demo.setVisible(true);
}
}
This code creates a simple time series chart with random data over a year. JFreeChart handles all the complexities of date formatting and scaling, allowing you to focus on your data and analysis.
Machine Learning with Weka
Machine learning is transforming the way we approach data analysis, and Java developers aren’t left out of the fun. Weka is a collection of machine learning algorithms for data mining tasks, written in Java. It provides tools for data preprocessing, classification, regression, clustering, and visualization. Here’s a simple example of how to use Weka to train a decision tree classifier:
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class WekaExample {
public static void main(String[] args) throws Exception {
// Load the dataset
DataSource source = new DataSource("path/to/your/dataset.arff");
Instances data = source.getDataSet();
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1);
}
// Create and train the classifier
J48 tree = new J48();
tree.buildClassifier(data);
// Print the decision tree
System.out.println(tree);
}
}
This example loads a dataset in ARFF format (Weka’s native file format), trains a J48 decision tree classifier, and prints the resulting tree. Weka provides a wealth of algorithms and tools for every stage of the machine learning pipeline, from data preprocessing to model evaluation.
Real-Time Data Processing with Apache Kafka
In today’s fast-paced digital world, processing data in real-time has become increasingly important. Apache Kafka, a distributed streaming platform, is a powerful tool for building real-time data pipelines and streaming applications. While Kafka itself is written in Scala, it provides excellent Java APIs that allow you to easily integrate it into your Java applications.
Let’s look at a simple example of how to produce and consume messages using Kafka in Java:
import org.apache.kafka.clients.producer.*;
import org.apache.kafka.clients.consumer.*;
import org.apache.kafka.common.serialization.StringDeserializer;
import org.apache.kafka.common.serialization.StringSerializer;
import java.time.Duration;
import java.util.Collections;
import java.util.Properties;
public class KafkaExample {
public static void main(String[] args) {
String bootstrapServers = "localhost:9092";
String topic = "test-topic";
// Producer configuration
Properties producerProps = new Properties();
producerProps.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, bootstrapServers);
producerProps.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
producerProps.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
// Create producer
KafkaProducer<String, String> producer = new KafkaProducer<>(producerProps);
// Send a message
producer.send(new ProducerRecord<>(topic, "key", "Hello, Kafka!"));
producer.close();
// Consumer configuration
Properties consumerProps = new Properties();
consumerProps.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, bootstrapServers);
consumerProps.put(ConsumerConfig.GROUP_ID_CONFIG, "test-group");
consumerProps.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
consumerProps.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
// Create consumer
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(consumerProps);
consumer.subscribe(Collections.singletonList(topic));
// Consume messages
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.printf("Received message: key = %s, value = %s%n", record.key(), record.value());
}
}
}
}
This example demonstrates how to set up a Kafka producer to send messages and a consumer to receive them. In a real-world scenario, you’d likely have separate applications for producing and consuming, possibly running on different machines in a distributed system.
Best Practices for Robust Data Processing Applications
As we wrap up our journey through the world of Java data processing, let’s discuss some best practices that will help you build robust, maintainable, and efficient applications. These tips will serve you well whether you’re working on a small personal project or a large-scale enterprise application.
Error Handling and Logging
When dealing with data processing, especially with large datasets or complex operations, things can and will go wrong. Proper error handling and logging are crucial for diagnosing and fixing issues. Here are some tips:
- Use specific exceptions: Instead of catching generic Exception classes, catch and handle specific exceptions. This makes your error handling more precise and your code more readable.
- Log meaningful information: When logging errors, include relevant context such as input data, current state, and stack traces. This will make debugging much easier.
- Use a robust logging framework: Libraries like SLF4J with Logback provide powerful features for managing logs in production environments.
Here’s an example of good error handling and logging practices:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class DataProcessor {
private static final Logger logger = LoggerFactory.getLogger(DataProcessor.class);
public void processData(String data) {
try {
// Process the data
int result = someRiskyOperation(data);
logger.info("Data processed successfully. Result: {}", result);
} catch (NumberFormatException e) {
logger.error("Invalid number format in input data: {}", data, e);
throw new IllegalArgumentException("Invalid input data", e);
}catch (IOException e) {
logger.error("IO error occurred while processing data: {}", data, e);
throw new RuntimeException("Error processing data", e);
}
}
private int someRiskyOperation(String data) throws NumberFormatException, IOException {
// Simulating some risky operation
return Integer.parseInt(data);
}
}
This example demonstrates how to use a logger to record both successful operations and errors, providing context that will be invaluable for troubleshooting.
Unit Testing for Data Processing Logic
Writing unit tests for your data processing logic is crucial for ensuring the correctness and robustness of your application. JUnit is the de facto standard for unit testing in Java. Here’s an example of how you might write a unit test for a data processing method:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class DataProcessorTest {
@Test
void testProcessData_validInput() {
DataProcessor processor = new DataProcessor();
assertDoesNotThrow(() -> processor.processData("123"));
}
@Test
void testProcessData_invalidInput() {
DataProcessor processor = new DataProcessor();
assertThrows(IllegalArgumentException.class, () -> processor.processData("abc"));
}
}
These tests verify that our processData
method behaves correctly for both valid and invalid inputs. Writing comprehensive tests like these can catch bugs early and make refactoring much safer.
Code Organization and Design Patterns
As your data processing applications grow in complexity, good code organization becomes increasingly important. Here are some tips and design patterns that can help keep your code clean and maintainable:
- Single Responsibility Principle: Each class or method should have a single, well-defined responsibility. For example, separate your data loading, processing, and output logic into different classes.
- Strategy Pattern: Use this pattern to define a family of algorithms, encapsulate each one, and make them interchangeable. This is particularly useful for implementing different data processing strategies that can be swapped out at runtime.
- Builder Pattern: When dealing with complex objects or configurations, use the Builder pattern to create them step by step. This can make your code more readable and less error-prone.
- Factory Pattern: Use factories to encapsulate object creation logic, especially when the exact type of object to be created isn’t known until runtime.
Here’s a quick example of how you might use the Strategy pattern for different data processing algorithms:
interface DataProcessingStrategy {
void process(List<String> data);
}
class SortingStrategy implements DataProcessingStrategy {
public void process(List<String> data) {
Collections.sort(data);
}
}
class FilteringStrategy implements DataProcessingStrategy {
public void process(List<String> data) {
data.removeIf(item -> item.length() < 5);
}
}
class DataProcessor {
private DataProcessingStrategy strategy;
public DataProcessor(DataProcessingStrategy strategy) {
this.strategy = strategy;
}
public void processData(List<String> data) {
strategy.process(data);
}
}
// Usage
DataProcessor sorter = new DataProcessor(new SortingStrategy());
DataProcessor filter = new DataProcessor(new FilteringStrategy());
This approach allows you to easily add new processing strategies without modifying existing code, adhering to the Open-Closed Principle.
Empowering Your Data Processing Journey with Java
As we’ve explored throughout this blog, Java provides a robust and versatile platform for tackling a wide range of data processing challenges. From its built-in collections and streams to powerful third-party libraries, Java equips developers with the tools they need to efficiently handle everything from small datasets to big data applications.
We’ve covered a lot of ground, from basic concepts to advanced techniques. We’ve seen how to optimize performance with parallel streams and memory management strategies, how to leverage libraries for specific tasks like CSV parsing and JSON processing, and how to apply Java to complex scenarios like time series analysis and machine learning.
Remember, the key to successful data processing isn’t just about knowing the tools – it’s about applying them effectively. Always consider the specific requirements of your project, the characteristics of your data, and the constraints of your environment when choosing your approach.
As you continue your journey in Java data processing, don’t be afraid to experiment with different techniques and libraries. The Java ecosystem is vast and constantly evolving, with new tools and frameworks emerging all the time. Stay curious, keep learning, and most importantly, enjoy the process of turning raw data into valuable insights!
Whether you’re building a small data analysis tool or a large-scale data processing pipeline, the skills and knowledge you’ve gained here will serve as a solid foundation. So go forth, write some code, crunch some numbers, and unlock the power of your data with Java!
Disclaimer: The code examples and techniques presented in this blog are for educational purposes and may need to be adapted for use in production environments. While every effort has been made to ensure accuracy, technologies and best practices in the field of data processing are continually evolving. Readers are encouraged to consult official documentation and conduct their own research when implementing these concepts in real-world scenarios. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.