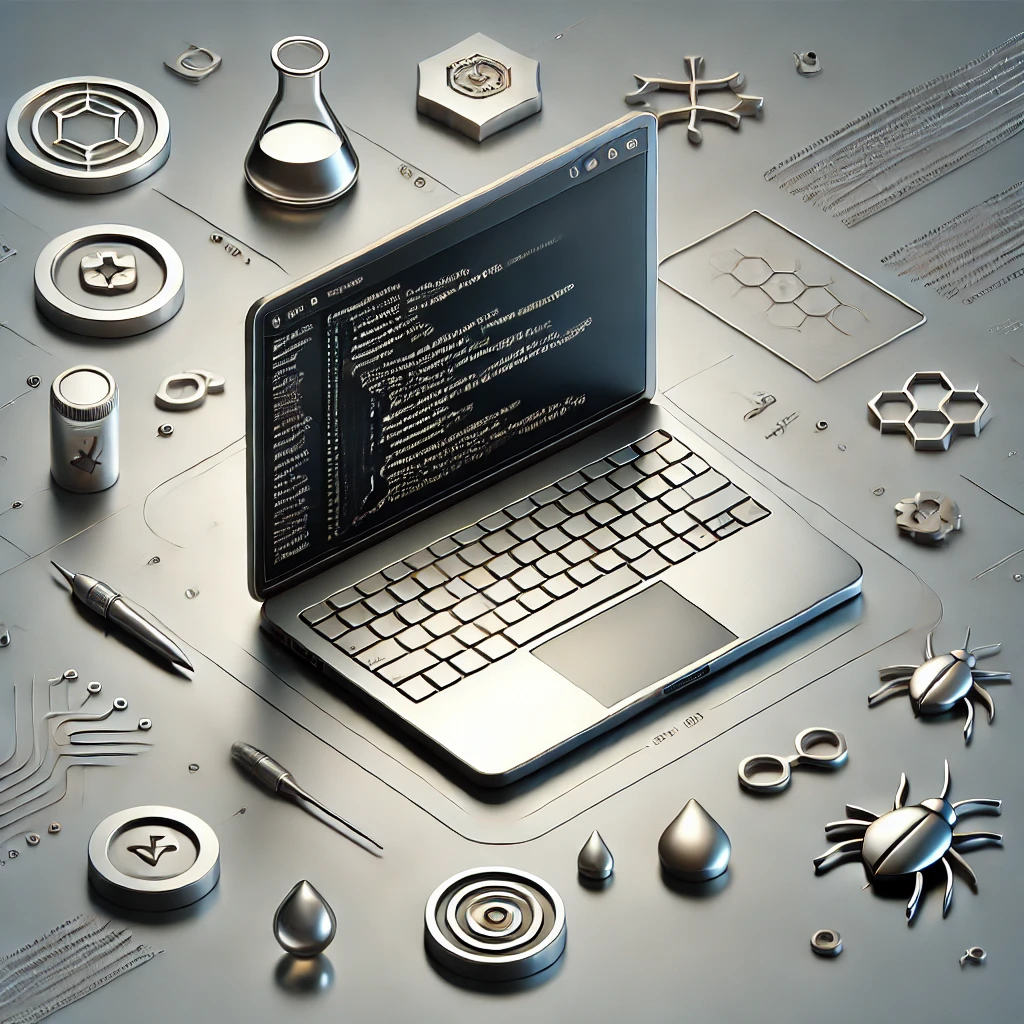
Testing Java Code
Today’s topic might not sound as exciting as creating flashy user interfaces or building complex algorithms, but trust me, it’s absolutely crucial for any serious Java developer. We’re talking about testing your Java code. Now, I know what you’re thinking – “Testing? Boooring!” But hold on to your keyboards, because I’m about to show you why testing is not only important but can actually be pretty interesting too.
Think about it: how many times have you written a piece of code, thought it was perfect, only to find out later that it breaks in ways you never imagined? Or worse, how often have you hesitated to refactor your code because you were afraid of breaking something? This is where testing comes to the rescue. It’s like having a safety net that allows you to code with confidence, knowing that if something goes wrong, your tests will catch it.
But testing isn’t just about catching bugs. It’s about improving the overall quality of your code, making it more maintainable, and even helping you design better software. In this blog post, we’ll explore the basics of testing Java code, from understanding different types of tests to writing your first unit tests. We’ll also look at some best practices and tools that can make your testing journey smoother. So, buckle up and get ready to level up your Java skills!
Understanding Different Types of Tests
Before we dive into the nitty-gritty of writing tests, let’s take a moment to understand the different types of tests you might encounter in the Java world. It’s like having different tools in your toolbox – each serves a specific purpose and is useful in different situations.
Unit Tests
First up, we have unit tests. These are the bread and butter of testing in Java. Unit tests focus on testing individual components or methods in isolation. They’re like checking each Lego brick before you start building your masterpiece. The idea is to ensure that each small part of your code works correctly on its own. Unit tests are typically fast, easy to write, and give you immediate feedback on the correctness of your code.
Integration Tests
Next, we have integration tests. These tests are a step up from unit tests and focus on how different components of your system work together. It’s like making sure all the gears in a clock mesh properly. Integration tests are crucial for catching issues that might arise when different parts of your code interact with each other, such as database connections, API calls, or service interactions.
Functional Tests
Moving up the testing pyramid, we have functional tests. These tests verify that your system meets the functional requirements and behaves as expected from an end-user perspective. Functional tests often involve testing entire user scenarios or workflows. They’re like test-driving a car to make sure all features work as advertised.
Performance Tests
Last but not least, we have performance tests. These tests are all about ensuring your Java application can handle the expected load and perform efficiently. Performance tests help you identify bottlenecks, measure response times, and ensure your application can scale. It’s like stress-testing a bridge to make sure it can handle rush hour traffic.
Understanding these different types of tests is crucial because each serves a different purpose and helps catch different kinds of issues. In this blog post, we’ll focus primarily on unit testing, as it forms the foundation of a solid testing strategy. But keep in mind that a well-rounded testing approach often involves a combination of these different test types.
Setting Up Your Testing Environment
Now that we’ve covered the types of tests, let’s get our hands dirty and set up our testing environment. Don’t worry; it’s easier than you might think!
Choosing a Testing Framework
In the Java world, there are several testing frameworks available, but the most popular and widely used is JUnit. JUnit is like the Swiss Army knife of Java testing – it’s versatile, easy to use, and comes with a ton of features out of the box. For this blog post, we’ll be using JUnit 5, the latest version of this awesome framework.
To get started with JUnit 5, you’ll need to add it to your project’s dependencies. If you’re using Maven, you can add the following to your pom.xml file:
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
</dependencies>
If you’re using Gradle, you can add this to your build.gradle file:
dependencies {
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.8.2'
testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.8.2'
}
test {
useJUnitPlatform()
}
Setting Up Your Project Structure
Now that we have JUnit in our project, let’s talk about project structure. In Java projects, it’s common to have a separate directory for your test code. This keeps your tests organized and separate from your main code. Typically, you’ll have a structure like this:
src
├── main
│ └── java
│ └── com
│ └── example
│ └── YourMainCode.java
└── test
└── java
└── com
└── example
└── YourTestCode.java
This structure allows you to mirror your main code structure in your test directory, making it easy to find and organize your tests.
Writing Your First Test
Alright, now that we have our environment set up, let’s write our first test! We’ll start with a simple example. Let’s say we have a Calculator class with an add method. Here’s what our main code might look like:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Now, let’s write a test for this method. We’ll create a CalculatorTest class in our test directory:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result, "2 + 3 should equal 5");
}
}
Let’s break this down:
- We import the necessary JUnit classes.
- We create a test method and annotate it with
@Test
. - Inside the test method, we create an instance of our Calculator class.
- We call the
add
method with some test inputs. - We use the
assertEquals
method to check if the result matches our expected output.
When you run this test, JUnit will execute the testAdd
method and report whether the test passed or failed. If the add
method returns 5 for inputs 2 and 3, the test will pass. If it returns any other value, the test will fail, and JUnit will show you the expected and actual values.
Congratulations! You’ve just written your first Java unit test. It might seem simple, but this is the foundation of test-driven development and robust software engineering practices.
Writing Effective Unit Tests
Now that we’ve dipped our toes into the world of Java testing, let’s dive deeper and explore how to write effective unit tests. Remember, the goal of unit testing is not just to increase your code coverage, but to actually improve the quality and reliability of your code.
The AAA Pattern
When writing unit tests, it’s helpful to follow a pattern. One popular pattern is the AAA (Arrange-Act-Assert) pattern. This pattern helps structure your tests in a clear and consistent way. Here’s how it works:
- Arrange: Set up the objects and data you need for your test.
- Act: Perform the action you’re testing.
- Assert: Check that the result matches your expectations.
Let’s look at an example. Say we have a User
class with a method to calculate the user’s age based on their birth year:
public class User {
private String name;
private int birthYear;
public User(String name, int birthYear) {
this.name = name;
this.birthYear = birthYear;
}
public int calculateAge(int currentYear) {
return currentYear - birthYear;
}
}
Now, let’s write a test for the calculateAge
method using the AAA pattern:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class UserTest {
@Test
public void testCalculateAge() {
// Arrange
User user = new User("John Doe", 1990);
int currentYear = 2024;
// Act
int age = user.calculateAge(currentYear);
// Assert
assertEquals(34, age, "Age calculation is incorrect");
}
}
This structure makes your tests easy to read and understand, which is crucial when you or your team members need to maintain or update tests in the future.
Testing Edge Cases
One common mistake in testing is only testing the “happy path” – the scenario where everything works as expected. But in the real world, things don’t always go smoothly. That’s why it’s important to test edge cases and potential error scenarios.
Let’s extend our User
class with a method that validates the birth year:
public class User {
// ... previous code ...
public boolean isValidBirthYear(int birthYear) {
int currentYear = java.time.Year.now().getValue();
return birthYear > 1900 && birthYear <= currentYear;
}
}
Now, let’s write some tests for this method, including edge cases:
public class UserTest {
@Test
public void testIsValidBirthYear_ValidYear() {
User user = new User("Jane Doe", 2000);
assertTrue(user.isValidBirthYear(2000), "Year 2000 should be valid");
}
@Test
public void testIsValidBirthYear_CurrentYear() {
User user = new User("Baby Doe", 2024);
assertTrue(user.isValidBirthYear(2024), "Current year should be valid");
}
@Test
public void testIsValidBirthYear_TooOld() {
User user = new User("Ancient One", 1900);
assertFalse(user.isValidBirthYear(1900), "Year 1900 should be invalid");
}
@Test
public void testIsValidBirthYear_FutureYear() {
User user = new User("Time Traveler", 2025);
assertFalse(user.isValidBirthYear(2025), "Future year should be invalid");
}
}
By testing these edge cases, we ensure that our isValidBirthYear
method behaves correctly in all scenarios, not just the most common ones.
Using Parameterized Tests
Sometimes, you might want to run the same test with different inputs. This is where parameterized tests come in handy. JUnit 5 provides a great way to create parameterized tests using the @ParameterizedTest
annotation.
Let’s create a parameterized test for our calculateAge
method:
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
import static org.junit.jupiter.api.Assertions.*;
public class UserTest {
@ParameterizedTest
@CsvSource({
"1990, 2024, 34",
"2000, 2024, 24",
"1980, 2024, 44",
"2024, 2024, 0"
})
public void testCalculateAge(int birthYear, int currentYear, int expectedAge) {
User user = new User("Test User", birthYear);
int age = user.calculateAge(currentYear);
assertEquals(expectedAge, age, "Age calculation is incorrect");
}
}
This single test method will run four times with different inputs, testing various scenarios in one go. It’s a powerful way to test multiple cases without repeating code.
Test-Driven Development (TDD)
Now that we’ve covered the basics of writing tests, let’s talk about a popular development methodology that puts testing at the forefront: Test-Driven Development, or TDD for short. TDD is like building a house by first creating a detailed blueprint – it helps ensure that your code does exactly what it’s supposed to do, no more, no less.
The TDD Cycle
TDD follows a simple cycle:
- Red: Write a failing test for the functionality you want to implement.
- Green: Write the minimum amount of code to make the test pass.
- Refactor: Improve the code while keeping the tests passing.
Let’s see this in action. Say we want to add a new feature to our User
class: a method to check if a user is an adult. We’ll start by writing a test:
public class UserTest {
@Test
public void testIsAdult_UserIs18() {
User user = new User("Adult User", 2006);
assertTrue(user.isAdult(2024), "User should be considered an adult at 18");
}
}
If we try to run this test, it will fail because we haven’t implemented the isAdult
method yet. That’s the “Red” phase.
Now, let’s implement the method to make the test pass:
public class User {
// ... previous code ...
public boolean isAdult(int currentYear) {
return calculateAge(currentYear) >= 18;
}
}
Run the test again, and it should pass. That’s the “Green” phase.
Finally, we can refactor if needed. In this case, our implementation is simple enough, so we might not need to refactor. But if we did, we’d make our changes while continually running the tests to ensure we don’t break anything.
Benefits of TDD
TDD might seem like extra work at first, but it comes with several benefits:
- It ensures that your code is testable from the start.
- It helps you think about edge cases and potential issues before you write your main code.
- It provides immediate feedback on whether your code is working as intended.
- It often leads to better design, as you’re forced to think about how your code will be used.
Remember, TDD is a skill that takes practice. Don’t worry if it feels awkward at first – with time, it can become a natural and valuable part of your development process.
Best Practices for Java Testing
As we wrap up our journey through the basics of Java testing, let’s talk about some best practices that can help you write better, more maintainable tests.
Keep Tests Simple and Focused
Each test should focus on one specific behavior or scenario. If you find yourself writing a test method that’s checking multiple things, consider breaking it into separate tests. This makes your tests easier to understand and maintain. It also helps isolate issues when a test fails.
For example, instead of this:
@Test
public void testUserFunctionality() {
User user = new User("John Doe", 1990);
assertEquals(34, user.calculateAge(2024), "Age calculation is incorrect");
assertTrue(user.isAdult(2024), "User should be an adult");
assertTrue(user.isValidBirthYear(1990), "Birth year should be valid");
}
Consider breaking it into separate tests:
@Test
public void testCalculateAge() {
User user = new User("John Doe", 1990);
assertEquals(34, user.calculateAge(2024), "Age calculation is incorrect");
}
@Test
public void testIsAdult() {
User user = new User("John Doe", 1990);
assertTrue(user.isAdult(2024), "User should be an adult");
}
@Test
public void testIsValidBirthYear() {
User user = new User("John Doe", 1990);
assertTrue(user.isValidBirthYear(1990), "Birth year should be valid");
}
Use Descriptive Test Names
Your test method names should clearly describe what they’re testing. A good practice is to use a naming convention like “testMethodName_StateUnderTest_ExpectedBehavior”. This makes it easier to understand what each test does without having to read the entire method.
For example:
@Test
public void testCalculateAge_UserBornIn1990_Returns34In2024() {
User user = new User("John Doe", 1990);
assertEquals(34, user.calculateAge(2024), "Age calculation is incorrect");
}
@Test
public void testIsAdult_UserIs18_ReturnsTrue() {
User user = new User("Jane Doe", 2006);
assertTrue(user.isAdult(2024), "User should be considered an adult at 18");
}
These names clearly communicate what the test is checking, making it easier for you and your team to understand and maintain the test suite.
Don’t Test Private Methods Directly
In Java, it’s generally not a good practice to test private methods directly. Private methods are implementation details and should be tested indirectly through public methods. If you find yourself wanting to test a private method, it might be a sign that the method should be refactored into a separate class or made public.
Instead of trying to test private methods, focus on testing the public interface of your classes. The behavior of private methods will be implicitly tested through the public methods that use them.
Use Setup and Teardown Methods
If you find yourself repeating the same setup code in multiple test methods, consider using JUnit’s @BeforeEach
and @AfterEach
annotations. These allow you to run code before and after each test method, respectively.
Here’s an example:
public class UserTest {
private User user;
@BeforeEach
public void setUp() {
user = new User("John Doe", 1990);
}
@Test
public void testCalculateAge() {
assertEquals(34, user.calculateAge(2024), "Age calculation is incorrect");
}
@Test
public void testIsAdult() {
assertTrue(user.isAdult(2024), "User should be an adult");
}
@AfterEach
public void tearDown() {
user = null;
}
}
This approach keeps your test methods clean and focused on the actual testing logic.
Use Assertions Effectively
JUnit provides a variety of assertion methods. Use the one that best fits your needs. For example, assertEquals
is great for comparing values, while assertTrue
and assertFalse
are good for boolean checks. For more complex objects, you might want to use assertThat
with custom matchers.
Here’s an example using different types of assertions:
@Test
public void testUserProperties() {
User user = new User("Alice", 2000);
assertEquals("Alice", user.getName(), "Name should match");
assertTrue(user.isValidBirthYear(2000), "Birth year should be valid");
assertFalse(user.isAdult(2010), "User should not be an adult in 2010");
assertNotNull(user.getEmail(), "Email should not be null");
}
Test Exception Handling
Don’t forget to test your error handling code. JUnit 5 provides a nice way to test exceptions:
@Test
public void testInvalidBirthYear_ThrowsIllegalArgumentException() {
assertThrows(IllegalArgumentException.class, () -> {
new User("Invalid User", 2050);
}, "Should throw IllegalArgumentException for future birth year");
}
This test passes if the constructor throws an IllegalArgumentException
when given an invalid birth year.
Mocking and Stubbing
As your Java applications grow more complex, you’ll often find that your classes depend on other classes or external services. Testing these dependencies can be challenging, which is where mocking comes in handy.
What is Mocking?
Mocking is creating objects that simulate the behavior of real objects. This is useful when the real objects are impractical to incorporate into the unit test. For example, if your class depends on a database connection, you wouldn’t want to connect to a real database for every test. Instead, you’d create a mock object that simulates the database behavior.
Using Mockito
One popular mocking framework for Java is Mockito. Let’s see how we can use Mockito to test a class that depends on an external service.
First, add Mockito to your project. If you’re using Maven, add this to your pom.xml:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.12.4</version>
<scope>test</scope>
</dependency>
Now, let’s say we have a UserService
class that depends on a DatabaseConnection
:
public class UserService {
private DatabaseConnection dbConnection;
public UserService(DatabaseConnection dbConnection) {
this.dbConnection = dbConnection;
}
public User getUserById(int id) {
return dbConnection.findUserById(id);
}
}
We can use Mockito to mock the DatabaseConnection
in our tests:
import org.junit.jupiter.api.Test;
import org.mockito.Mockito;
import static org.junit.jupiter.api.Assertions.*;
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUserById() {
// Create a mock DatabaseConnection
DatabaseConnection mockConnection = mock(DatabaseConnection.class);
// Define the behavior of the mock
User expectedUser = new User("John Doe", 1990);
when(mockConnection.findUserById(1)).thenReturn(expectedUser);
// Create the UserService with the mock DatabaseConnection
UserService userService = new UserService(mockConnection);
// Test the getUserById method
User result = userService.getUserById(1);
// Verify the result
assertEquals(expectedUser, result, "Should return the expected user");
// Verify that the mock method was called
verify(mockConnection).findUserById(1);
}
}
In this test, we’re creating a mock DatabaseConnection
, defining its behavior (what it should return when findUserById
is called), and then verifying that our UserService
uses it correctly.
Conclusion
Whew! We’ve covered a lot of ground in our journey through the basics of testing Java code. We’ve explored different types of tests, set up a testing environment with JUnit, written our first unit tests, delved into Test-Driven Development, discussed best practices, and even touched on mocking with Mockito.
Remember, testing is not just about finding bugs – it’s about building confidence in your code, improving its design, and making it easier to maintain and refactor. As you continue your Java development journey, make testing an integral part of your workflow. Start small, test often, and gradually build up your testing skills.
Don’t be discouraged if writing tests feels challenging at first. Like any skill, it takes practice. But trust me, the benefits are worth it. You’ll write better code, catch issues earlier, and sleep better at night knowing your applications are rock-solid.
So, what are you waiting for? Fire up your IDE, create a new test class, and start testing! Your future self (and your colleagues) will thank you for it.
Happy testing, Java enthusiasts!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information and code examples presented in this blog post, they are provided “as is” without warranty of any kind. The author and the website do not assume any responsibility or liability for any errors or omissions in the content. Readers are encouraged to verify and adapt the information to their specific needs. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.