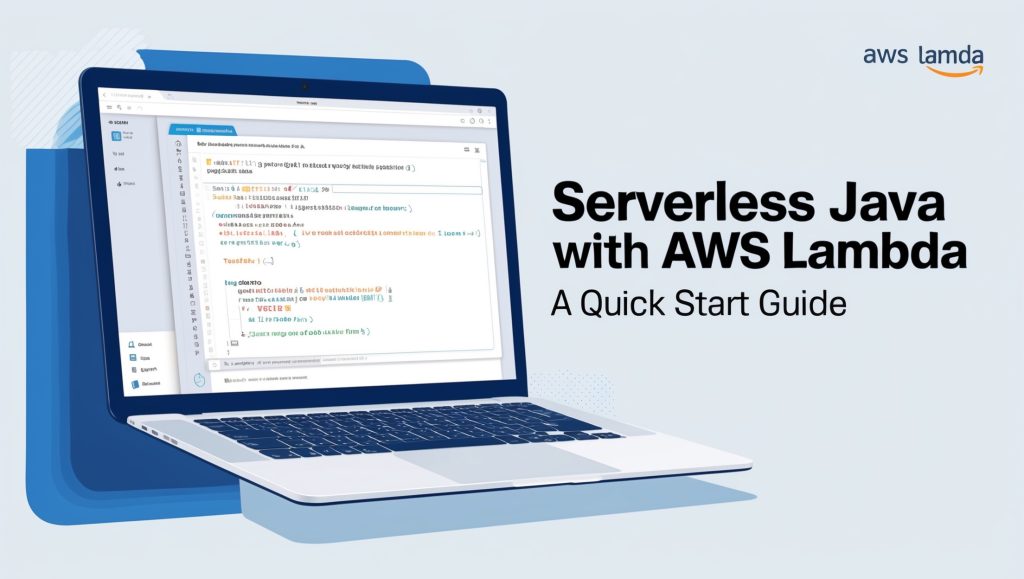
Serverless Java with AWS Lambda: A Quick Start Guide
In the ever-evolving landscape of cloud computing, serverless architecture has emerged as a game-changer, revolutionizing the way we build and deploy applications. At the forefront of this paradigm shift is AWS Lambda, a serverless compute service that’s been gaining tremendous traction among developers and organizations alike. But what if you’re a Java developer looking to dip your toes into the serverless waters? Fear not! This guide is tailored just for you.
Welcome to our comprehensive quick start guide on Serverless Java with AWS Lambda. Whether you’re a seasoned Java developer curious about serverless computing or a cloud enthusiast eager to harness the power of Java in a serverless environment, you’re in for an exciting journey. We’ll walk you through the fundamentals of serverless architecture, explore the capabilities of AWS Lambda, and dive deep into creating, deploying, and managing Java-based Lambda functions.
By the end of this guide, you’ll have a solid grasp of how to leverage your Java skills in a serverless context, opening up a world of possibilities for building scalable, efficient, and cost-effective applications. From setting up your development environment to deploying your first Lambda function, we’ve got you covered every step of the way. We’ll also explore best practices, common use cases, and potential challenges you might encounter in your serverless Java journey.
So, grab your favorite IDE, dust off your Java skills, and let’s embark on this serverless adventure together. Trust me, by the time we’re done, you’ll be wondering why you didn’t jump on the serverless bandwagon sooner!
Ready to transform the way you think about Java development? Let’s dive in and unlock the potential of Serverless Java with AWS Lambda!
Understanding Serverless Architecture
Before we dive into the specifics of AWS Lambda and Java, let’s take a moment to understand what serverless architecture really means. Contrary to what the name might suggest, serverless doesn’t mean there are no servers involved. Rather, it’s an approach to software design where developers can focus solely on writing code without worrying about the underlying infrastructure.
In a serverless model, the cloud provider (in our case, AWS) takes care of all server management tasks. This includes provisioning, scaling, patching, and maintaining servers. As a developer, you simply write your code and deploy it to the cloud provider’s platform, which then executes it on demand.
Key benefits of serverless architecture:
- Reduced operational overhead: With no servers to manage, your team can focus on developing features rather than maintaining infrastructure.
- Automatic scaling: Serverless platforms automatically scale your application based on demand, ensuring optimal performance during traffic spikes.
- Cost-efficiency: You only pay for the actual compute time your code uses, not for idle server time.
- Faster time to market: With less time spent on infrastructure concerns, you can deploy new features and applications more quickly.
While serverless architecture offers numerous advantages, it’s not without its challenges. Cold starts, limited execution duration, and potential vendor lock-in are factors to consider. However, for many applications, the benefits far outweigh these concerns.
AWS Lambda: The Cornerstone of Serverless
AWS Lambda is Amazon’s serverless compute service, and it’s at the heart of serverless architecture on the AWS platform. Lambda allows you to run code without provisioning or managing servers. You can run code for virtually any type of application or backend service – all with zero administration.
How AWS Lambda works:
- You upload your code to Lambda or write it directly in the Lambda console.
- You set up your function to trigger from other AWS services, HTTP endpoints, or direct invocations.
- Lambda automatically runs your code only when needed and scales with the size of the workload.
- You pay only for the compute time you consume – there’s no charge when your code isn’t running.
Lambda supports several programming languages, including Java, which makes it an excellent choice for Java developers looking to embrace serverless architecture.
Why Java for AWS Lambda?
Java has been a staple in enterprise software development for decades, and for good reason. Its robustness, extensive ecosystem, and strong typing make it an excellent choice for building reliable and scalable applications. But how does it fare in a serverless environment like AWS Lambda?
Advantages of using Java with AWS Lambda:
- Mature ecosystem: Java’s vast library of tools and frameworks can be leveraged in your Lambda functions.
- Strong typing: Java’s compile-time type checking helps catch errors early, leading to more reliable code.
- Performance: While Java has a reputation for slow cold starts, recent improvements in both Java and Lambda have significantly reduced this issue.
- Familiarity: For teams already using Java, the learning curve for Lambda is much less steep.
While Java might not be the first language that comes to mind for serverless development, its strengths make it a compelling choice for many scenarios, especially in enterprise environments.
Setting Up Your Development Environment
Before we start coding, let’s ensure we have the right tools in place. Here’s what you’ll need to develop Java-based Lambda functions:
- Java Development Kit (JDK): AWS Lambda supports Java 8 and Java 11. Ensure you have the appropriate JDK installed.
- Integrated Development Environment (IDE): While you can use any text editor, an IDE like IntelliJ IDEA or Eclipse can significantly boost your productivity.
- AWS CLI: The AWS Command Line Interface is useful for interacting with AWS services.
- AWS SDK for Java: This provides Java APIs for AWS services.
- Maven or Gradle: These build tools will help manage dependencies and package your Lambda functions.
Setting up your project:
Let’s set up a basic Maven project for our Lambda function. Create a new directory for your project and add a pom.xml
file with the following content:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>lambda-java-example</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-lambda-java-core</artifactId>
<version>1.2.1</version>
</dependency>
</dependencies>
</project>
This pom.xml
file sets up a basic Java 11 project with the AWS Lambda Java Core Library as a dependency.
Creating Your First Java Lambda Function
Now that our environment is set up, let’s create a simple Lambda function. We’ll start with the classic “Hello, World!” example.
Create a new Java class in your project:
package com.example;
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class HelloWorldLambda implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
context.getLogger().log("Input: " + input);
return "Hello, " + input + "!";
}
}
This Lambda function takes a string input (a name) and returns a greeting. The RequestHandler
interface is part of the AWS Lambda Java Core Library and provides the handleRequest
method that Lambda will invoke.
Understanding the code:
- We implement the
RequestHandler
interface, specifyingString
as both the input and output type. - The
handleRequest
method is where our Lambda function’s logic resides. - We use the
Context
object to log information, which will appear in CloudWatch Logs. - The function returns a greeting string.
Deploying Your Lambda Function
With our function written, it’s time to deploy it to AWS Lambda. Here’s a step-by-step guide:
- Package your function: Run
mvn package
to create a JAR file of your project. - Create a Lambda function:
- Open the AWS Lambda console.
- Click “Create function”.
- Choose “Author from scratch”.
- Enter a function name (e.g., “HelloWorldLambda”).
- For runtime, select “Java 11”.
- For execution role, create a new role with basic Lambda permissions.
- Upload your code:
- In the “Function code” section, select “Upload a .zip or .jar file”.
- Upload the JAR file created by Maven.
- Set the Handler to “com.example.HelloWorldLambda::handleRequest”.
- Configure your function:
- Set the memory and timeout as needed (128 MB and 15 seconds are often sufficient for simple functions).
- Save your function.
Congratulations! You’ve just deployed your first Java Lambda function.
Testing and Monitoring Your Lambda Function
Now that our function is deployed, let’s test it and see how we can monitor its performance.
Testing in the AWS Console:
- In the Lambda function page, click the “Test” tab.
- Create a new test event with a JSON payload like
"World"
. - Click “Test” to run your function.
You should see the output “Hello, World!” along with execution details like duration and memory usage.
Monitoring with CloudWatch:
AWS automatically sends Lambda logs to CloudWatch. To view these:
- Go to the CloudWatch console.
- Navigate to “Logs” > “Log groups”.
- Find the log group for your Lambda function (it will be named
/aws/lambda/YourFunctionName
).
Here, you can see detailed logs of your function’s executions, including any custom log messages you’ve added.
Best Practices for Java Lambda Functions
As you continue developing Lambda functions in Java, keep these best practices in mind:
- Minimize cold starts: Initialize static resources outside the handler method to reduce cold start times.
- Reuse connections: If your function connects to a database or makes HTTP requests, reuse connections when possible.
- Keep functions small: Focus each function on a single task for better performance and easier maintenance.
- Use environment variables: Store configuration in environment variables rather than hardcoding values.
- Handle exceptions gracefully: Proper error handling ensures your function fails gracefully and provides useful information for debugging.
Here’s an example incorporating some of these practices:
package com.example;
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
import software.amazon.awssdk.services.dynamodb.DynamoDbClient;
import software.amazon.awssdk.services.dynamodb.model.PutItemRequest;
import software.amazon.awssdk.services.dynamodb.model.AttributeValue;
import java.util.HashMap;
import java.util.Map;
public class UserRegistrationLambda implements RequestHandler<User, String> {
private static final DynamoDbClient dynamoDb = DynamoDbClient.builder().build();
private static final String TABLE_NAME = System.getenv("USER_TABLE_NAME");
@Override
public String handleRequest(User user, Context context) {
try {
Map<String, AttributeValue> item = new HashMap<>();
item.put("id", AttributeValue.builder().s(user.getId()).build());
item.put("name", AttributeValue.builder().s(user.getName()).build());
item.put("email", AttributeValue.builder().s(user.getEmail()).build());
PutItemRequest putItemRequest = PutItemRequest.builder()
.tableName(TABLE_NAME)
.item(item)
.build();
dynamoDb.putItem(putItemRequest);
return "User registered successfully";
} catch (Exception e) {
context.getLogger().log("Error registering user: " + e.getMessage());
return "Error registering user";
}
}
}
class User {
private String id;
private String name;
private String email;
// Getters and setters omitted for brevity
}
This example demonstrates a Lambda function that registers a user in DynamoDB, incorporating several best practices like reusing the DynamoDB client, using environment variables, and proper error handling.
Common Use Cases and Examples
Java Lambda functions can be used in a wide variety of scenarios. Here are a few common use cases with brief code examples:
1. API Backend:
Lambda can serve as the backend for API Gateway. Here’s a simple example:
public class ApiHandler implements RequestHandler<APIGatewayProxyRequestEvent, APIGatewayProxyResponseEvent> {
@Override
public APIGatewayProxyResponseEvent handleRequest(APIGatewayProxyRequestEvent input, Context context) {
String body = input.getBody();
// Process the request...
APIGatewayProxyResponseEvent response = new APIGatewayProxyResponseEvent();
response.setStatusCode(200);
response.setBody("Request processed successfully");
return response;
}
}
2. Scheduled Tasks:
Lambda functions can be scheduled using CloudWatch Events. For example, a daily data cleanup task:
public class DataCleanupHandler implements RequestHandler<ScheduledEvent, String> {
@Override
public String handleRequest(ScheduledEvent event, Context context) {
// Perform data cleanup operations...
return "Data cleanup completed successfully";
}
}
3. Stream Processing:
Lambda can process data from streams like Kinesis or DynamoDB Streams:
public class StreamProcessor implements RequestHandler<DynamodbEvent, Void> {
@Override
public Void handleRequest(DynamodbEvent event, Context context) {
for (DynamodbEvent.DynamodbStreamRecord record : event.getRecords()) {
// Process each record...
}
return null;
}
}
These examples showcase the versatility of Java Lambda functions in different serverless scenarios.
Challenges and Considerations
While serverless Java with AWS Lambda offers many benefits, it’s important to be aware of potential challenges:
- Cold Starts: Java functions can experience longer cold start times compared to some other languages. This can be mitigated by using provisioned concurrency or keeping your deployment package small.
- Limited Execution Time: Lambda functions have a maximum execution time of 15 minutes. For longer-running tasks, consider breaking them into smaller functions or using Step Functions.
- Statelessness: Lambda functions are stateless by design. If you need to maintain state between invocations, you’ll need to use external services like DynamoDB.
- Debugging and Testing: Debugging serverless applications can be more challenging. Utilize AWS X-Ray and comprehensive logging to aid in troubleshooting.
- Vendor Lock-in: While Lambda is powerful, it’s specific to AWS. Consider using abstraction layers if portability is a concern.
Despite these challenges, the benefits of serverless often outweigh the drawbacks for many use cases.
Conclusion
Serverless Java with AWS Lambda represents a powerful paradigm shift in how we develop and deploy applications. By leveraging your existing Java skills in a serverless context, you can build scalable, efficient, and cost-effective solutions with minimal operational overhead.
We’ve covered a lot of ground in this guide – from understanding serverless architecture and AWS Lambda to creating, deploying, and best practices for Java Lambda functions. Remember, this is just the beginning of your serverless journey. As you continue to explore and experiment, you’ll discover even more ways to leverage the power of serverless Java.
The serverless landscape is continually evolving, with AWS regularly introducing new features and improvements. Stay curious, keep learning, and don’t be afraid to push the boundaries of what’s possible with serverless Java.
So, what are you waiting for? Take your Java skills, combine them with the power of AWS Lambda, and start building the next generation of cloud-native applications. The serverless world is your oyster!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this guide, technology and cloud services are rapidly evolving fields. Always refer to the official AWS documentation for the most up-to-date information. If you notice any inaccuracies in this guide, please report them so we can correct them promptly.