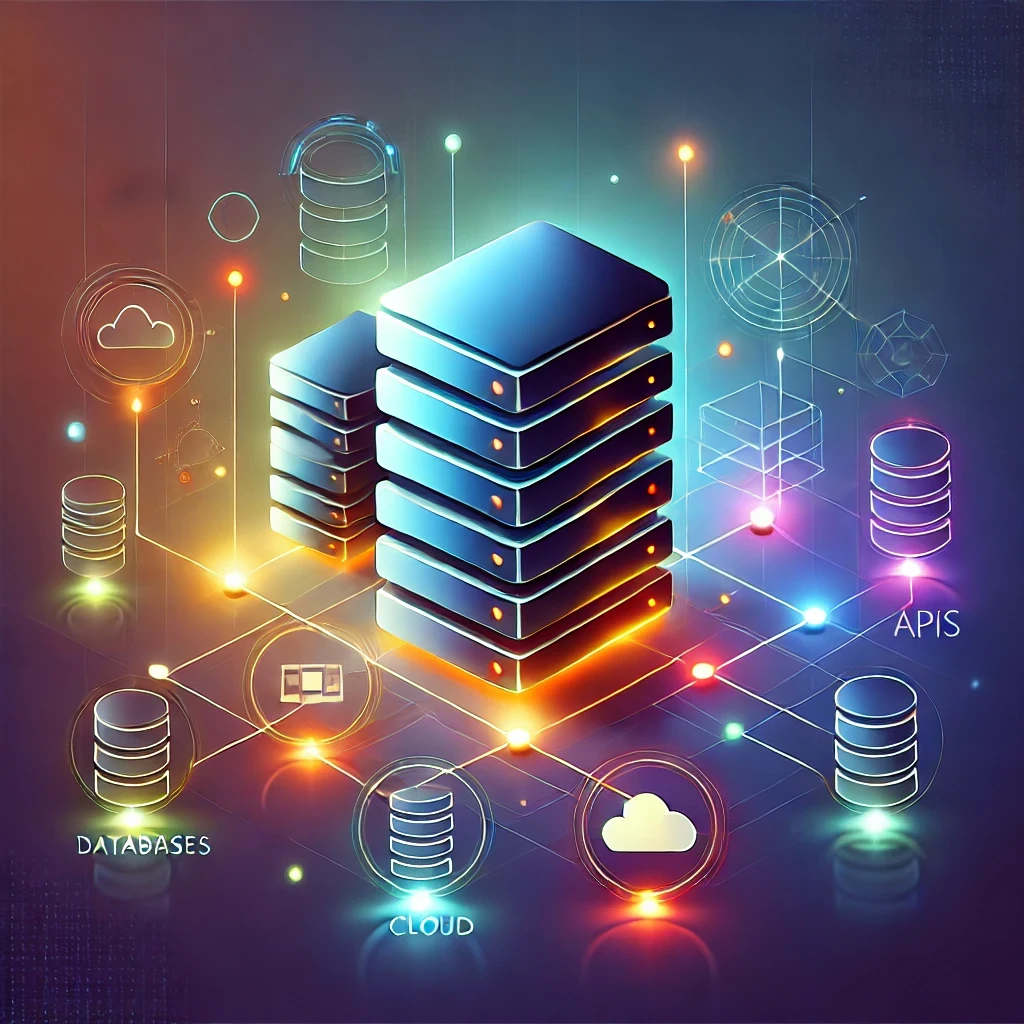
Integrating Java Application Servers with Other Technologies
Hey there, tech enthusiasts! Today, we’re diving deep into the world of Java application servers and exploring how to integrate them with other cutting-edge technologies. Whether you’re a seasoned Java developer or just starting your journey, this guide will help you understand the ins and outs of creating powerful, scalable applications by combining Java app servers with various tools and frameworks. So, grab your favorite beverage, and let’s embark on this exciting adventure!
What are Java Application Servers?
Before we jump into the integration part, let’s take a moment to understand what Java application servers are and why they’re so crucial in modern web development. Java application servers, also known as Java EE (Enterprise Edition) servers, are software frameworks that provide a runtime environment for Java applications. They handle various aspects of application management, including security, transaction processing, and resource allocation.
Some popular Java application servers include:
- Apache Tomcat
- WildFly (formerly JBoss)
- IBM WebSphere
- Oracle WebLogic
- GlassFish
These servers play a vital role in deploying and running Java web applications, providing a robust foundation for enterprise-level solutions. Now that we’ve got the basics covered let’s explore how we can integrate these powerhouses with other technologies to create even more impressive applications.
Integrating Java App Servers with Databases
One of the most common integrations you’ll encounter is connecting your Java application server to a database. This integration is crucial for storing and retrieving data in your applications. Let’s look at how you can integrate a Java app server with different types of databases.
Relational Databases (SQL)
Integrating Java app servers with relational databases like MySQL, PostgreSQL, or Oracle is a common practice. Here’s a simple example of how you can establish a connection to a MySQL database using JDBC (Java Database Connectivity):
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
public static void main(String[] args) {
try (Connection conn = getConnection()) {
System.out.println("Connected to the database successfully!");
} catch (SQLException e) {
System.err.println("Error connecting to the database: " + e.getMessage());
}
}
}
This code snippet demonstrates how to establish a connection to a MySQL database using JDBC. You’ll need to include the appropriate JDBC driver in your project’s classpath and configure your application server to use the database connection.
NoSQL Databases
In recent years, NoSQL databases have gained popularity due to their flexibility and scalability. Integrating Java app servers with NoSQL databases like MongoDB or Cassandra can provide powerful solutions for handling unstructured data. Here’s an example of how to connect to MongoDB using the official Java driver:
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoDatabase;
public class MongoDBConnection {
private static final String CONNECTION_STRING = "mongodb://localhost:27017";
private static final String DATABASE_NAME = "your_database";
public static MongoDatabase getDatabase() {
MongoClient mongoClient = MongoClients.create(CONNECTION_STRING);
return mongoClient.getDatabase(DATABASE_NAME);
}
public static void main(String[] args) {
MongoDatabase database = getDatabase();
System.out.println("Connected to MongoDB successfully!");
// Perform database operations here
}
}
This example shows how to establish a connection to a MongoDB database using the official Java driver. You’ll need to include the MongoDB Java driver in your project dependencies and configure your application server accordingly.
Integrating Java App Servers with Front-end Frameworks
In today’s web development landscape, creating dynamic and responsive user interfaces is crucial. Integrating Java app servers with modern front-end frameworks can help you achieve this goal. Let’s explore how to integrate Java app servers with popular front-end technologies.
React Integration
React is a popular JavaScript library for building user interfaces. Here’s how you can integrate a Java app server with a React front-end:
- Create a RESTful API using Java frameworks like Spring Boot or JAX-RS.
- Implement CORS (Cross-Origin Resource Sharing) to allow communication between your Java backend and React frontend.
- Use React to consume the API and render the user interface.
Here’s a simple example of a Spring Boot controller that exposes a RESTful API:
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public List<User> getUsers() {
// Fetch users from the database
return userService.getAllUsers();
}
@PostMapping("/users")
public User createUser(@RequestBody User user) {
// Create a new user
return userService.createUser(user);
}
}
On the React side, you can use the fetch
API or libraries like Axios to consume this API:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
axios.get('http://localhost:8080/api/users')
.then(response => setUsers(response.data))
.catch(error => console.error('Error fetching users:', error));
}, []);
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
export default UserList;
This React component fetches users from the Java backend and displays them in a list. By combining the power of Java app servers with the flexibility of React, you can create robust and interactive web applications.
Integrating Java App Servers with Microservices Architecture
Microservices architecture has revolutionized the way we design and deploy applications. Integrating Java app servers with microservices can help you create scalable and maintainable systems. Let’s explore how you can achieve this integration.
Spring Boot Microservices
Spring Boot is an excellent framework for building microservices in Java. Here’s an example of how you can create a simple microservice using Spring Boot:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
@GetMapping("/users")
public List<String> getUsers() {
return Arrays.asList("Alice", "Bob", "Charlie");
}
}
This simple microservice exposes a /users
endpoint that returns a list of user names. You can deploy multiple such microservices on different Java app servers and use tools like Spring Cloud for service discovery and configuration management.
Service Discovery with Eureka
To facilitate communication between microservices, you can use service discovery tools like Netflix Eureka. Here’s how you can integrate Eureka with your Spring Boot microservices:
- Add the Eureka client dependency to your project:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
- Enable Eureka client in your application:
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
@EnableDiscoveryClient
public class UserServiceApplication {
// ...
}
- Configure Eureka client properties in your
application.properties
file:
spring.application.name=user-service
eureka.client.serviceUrl.defaultZone=http://localhost:8761/eureka/
By integrating service discovery, your microservices can dynamically locate and communicate with each other, creating a flexible and scalable architecture.
Integrating Java App Servers with Message Queues
Message queues play a crucial role in building distributed systems. Integrating Java app servers with message queues can help you create loosely coupled, scalable applications. Let’s explore how to integrate Java app servers with Apache Kafka, a popular distributed streaming platform.
Apache Kafka Integration
Here’s an example of how you can integrate a Java application server with Apache Kafka:
- Add the Kafka client dependency to your project:
<dependency>
<groupId>org.apache.kafka</groupId>
<artifactId>kafka-clients</artifactId>
<version>3.0.0</version>
</dependency>
- Create a Kafka producer to send messages:
import org.apache.kafka.clients.producer.*;
import java.util.Properties;
public class KafkaProducerExample {
private static final String TOPIC = "my-topic";
private static final String BOOTSTRAP_SERVERS = "localhost:9092";
public static void main(String[] args) {
Properties props = new Properties();
props.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, BOOTSTRAP_SERVERS);
props.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringSerializer");
props.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
String message = "Hello, Kafka!";
ProducerRecord<String, String> record = new ProducerRecord<>(TOPIC, message);
producer.send(record, (metadata, exception) -> {
if (exception == null) {
System.out.println("Message sent successfully: " + metadata.offset());
} else {
System.err.println("Error sending message: " + exception.getMessage());
}
});
producer.close();
}
}
- Create a Kafka consumer to receive messages:
import org.apache.kafka.clients.consumer.*;
import java.time.Duration;
import java.util.Collections;
import java.util.Properties;
public class KafkaConsumerExample {
private static final String TOPIC = "my-topic";
private static final String BOOTSTRAP_SERVERS = "localhost:9092";
private static final String GROUP_ID = "my-group";
public static void main(String[] args) {
Properties props = new Properties();
props.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, BOOTSTRAP_SERVERS);
props.put(ConsumerConfig.GROUP_ID_CONFIG, GROUP_ID);
props.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringDeserializer");
props.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringDeserializer");
Consumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Collections.singletonList(TOPIC));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.println("Received message: " + record.value());
}
}
}
}
By integrating Kafka with your Java application server, you can build scalable, event-driven architectures that can handle high-throughput data streaming.
Integrating Java App Servers with Caching Solutions
Caching is an essential technique for improving application performance. Let’s explore how to integrate Java app servers with Redis, a popular in-memory data structure store that can be used as a cache.
Redis Integration
Here’s an example of how you can integrate Redis with a Java application server using the Jedis client:
- Add the Jedis dependency to your project:
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.7.0</version>
</dependency>
- Create a Redis connection pool and use it to cache data:
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
public class RedisExample {
private static final String REDIS_HOST = "localhost";
private static final int REDIS_PORT = 6379;
private static JedisPool jedisPool;
static {
JedisPoolConfig poolConfig = new JedisPoolConfig();
poolConfig.setMaxTotal(10);
poolConfig.setMaxIdle(5);
poolConfig.setMinIdle(1);
jedisPool = new JedisPool(poolConfig, REDIS_HOST, REDIS_PORT);
}
public static void setCache(String key, String value) {
try (Jedis jedis = jedisPool.getResource()) {
jedis.set(key, value);
}
}
public static String getCache(String key) {
try (Jedis jedis = jedisPool.getResource()) {
return jedis.get(key);
}
}
public static void main(String[] args) {
setCache("user:1", "John Doe");
String cachedValue = getCache("user:1");
System.out.println("Cached value: " + cachedValue);
}
}
This example demonstrates how to set up a Redis connection pool and use it to store and retrieve cached data. By integrating Redis with your Java application server, you can significantly improve the performance of your applications by reducing database load and speeding up frequently accessed data.
Integrating Java App Servers with Containerization Technologies
Containerization has become an essential part of modern application deployment. Let’s explore how to integrate Java app servers with Docker, a popular containerization platform.
Dockerizing a Java Application
Here’s an example of how you can containerize a Java application using Docker:
- Create a
Dockerfile
in your project root directory:
FROM openjdk:11-jre-slim
WORKDIR /app
COPY target/your-application.jar /app/app.jar
EXPOSE 8080
CMD ["java", "-jar", "app.jar"]
- Build your Java application:
mvn clean package
- Build the Docker image:
docker build -t your-java-app .
- Run the Docker container:
docker run -p 8080:8080 your-java-app
By containerizing your Java application, you can ensure consistent deployment across different environments and simplify the scaling process.
Integrating Java App Servers with Cloud Platforms
Cloud platforms offer scalable and flexible infrastructure for deploying Java applications. Let’s explore how to integrate Java app servers with Amazon Web Services (AWS), a popular cloud platform.
Deploying to AWS Elastic Beanstalk
AWS Elastic Beanstalk is a Platform as a Service (PaaS) offering that simplifies the deployment of Java applications. Here’s how you can deploy a Java application to Elastic Beanstalk:
- Package your application as a WAR file.
- Create an Elastic Beanstalk application and environment using the AWS Management Console or AWS CLI.
- Upload your WAR file to the Elastic Beanstalk environment.
Here’s an example of how to deploy using the AWS CLI:
# Create an Elastic Beanstalk environment
aws elasticbeanstalk create-environment
--application-name your-application-name
--environment-name your-environment-name
--solution-stack-name "64bit Amazon Linux 2 v3.2.16 running Corretto 11"
--option-settings file://env-config.json
# Deploy your application
aws elasticbeanstalk create-application-version \
--application-name your-application-name \
--version-label v1 \
--source-bundle S3Bucket="your-bucket",S3Key="your-application.war"
aws elasticbeanstalk update-environment \
--environment-name your-environment-name \
--version-label v1
By integrating your Java application server with AWS Elastic Beanstalk, you can take advantage of automatic scaling, load balancing, and easy deployment processes.
Integrating Java App Servers with Monitoring and Logging Tools
Effective monitoring and logging are crucial for maintaining and troubleshooting Java applications. Let’s explore how to integrate Java app servers with popular monitoring and logging tools.
Integrating with Prometheus and Grafana
Prometheus is an open-source monitoring system, and Grafana is a visualization tool that works well with Prometheus. Here’s how you can integrate them with your Java application:
1. Add the Micrometer Prometheus dependency to your project:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
<version>1.8.2</version>
</dependency>
2. Configure Prometheus metrics in your Spring Boot application:
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.prometheus.PrometheusConfig;
import io.micrometer.prometheus.PrometheusMeterRegistry;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MetricsConfig {
@Bean
public MeterRegistry meterRegistry() {
PrometheusMeterRegistry registry = new PrometheusMeterRegistry(PrometheusConfig.DEFAULT);
// Add custom metrics here
registry.gauge("my_custom_metric", 100);
return registry;
}
}
3. Expose a Prometheus metrics endpoint in your application:
import io.micrometer.prometheus.PrometheusMeterRegistry;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MetricsController {
private final PrometheusMeterRegistry prometheusMeterRegistry;
public MetricsController(PrometheusMeterRegistry prometheusMeterRegistry) {
this.prometheusMeterRegistry = prometheusMeterRegistry;
}
@GetMapping("/prometheus")
public String prometheusMetrics() {
return prometheusMeterRegistry.scrape();
}
}
4. Configure Prometheus to scrape metrics from your application by adding the following to your prometheus.yml file:
scrape_configs:
job_name: 'spring-boot-app'
metrics_path: '/prometheus' static_configs:
targets: ['localhost:8080']
5. Set up Grafana to visualize the metrics collected by Prometheus.
By integrating Prometheus and Grafana with your Java application server, you can gain valuable insights into your application’s performance and health.
Integrating Java App Servers with Security Frameworks
Security is a critical aspect of any application. Let’s explore how to integrate Java app servers with Spring Security, a powerful and customizable authentication and access-control framework.
Implementing Spring Security
Here’s an example of how to integrate Spring Security into your Java application:
- Add the Spring Security dependency to your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
2. Create a basic security configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.provisioning.InMemoryUserDetailsManager;
import org.springframework.security.web.SecurityFilterChain;
@Configuration
@EnableWebSecurity
public class SecurityConfig {
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests((requests) -> requests
.requestMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
)
.formLogin((form) -> form
.loginPage("/login")
.permitAll()
)
.logout((logout) -> logout.permitAll());
return http.build();
}
@Bean
public UserDetailsService userDetailsService() {
UserDetails user =
User.withDefaultPasswordEncoder()
.username("user")
.password("password")
.roles("USER")
.build();
return new InMemoryUserDetailsManager(user);
}
}
This configuration sets up basic authentication and authorization rules for your application. You can customize this further to meet your specific security requirements.
Bringing It All Together
We’ve covered a lot of ground in this comprehensive guide to integrating Java application servers with other technologies. From databases and front-end frameworks to microservices, caching solutions, and cloud platforms, we’ve explored various ways to enhance your Java applications.
Here’s a quick recap of the integrations we’ve discussed:
Technology | Integration Benefits |
---|---|
Databases (SQL/NoSQL) | Data persistence and retrieval |
Front-end Frameworks (React) | Dynamic and responsive user interfaces |
Microservices (Spring Boot) | Scalable and maintainable architecture |
Message Queues (Kafka) | Asynchronous communication and event-driven design |
Caching (Redis) | Improved performance and reduced database load |
Containerization (Docker) | Consistent deployment and scalability |
Cloud Platforms (AWS) | Scalable infrastructure and managed services |
Monitoring (Prometheus/Grafana) | Application insights and performance tracking |
Security (Spring Security) | Authentication and access control |
By leveraging these integrations, you can create robust, scalable, and feature-rich applications that meet the demands of modern software development. Remember, the key to successful integration is understanding your application’s requirements and choosing the right technologies that complement your Java application server.
As you embark on your integration journey, keep the following best practices in mind:
- Start small: Begin with simple integrations and gradually add complexity as you become more comfortable with each technology.
- Maintain modularity: Design your integrations in a way that allows for easy updates or replacements in the future.
- Consider performance: Always evaluate the performance impact of each integration and optimize as necessary.
- Prioritize security: Ensure that each integration adheres to security best practices and doesn’t introduce vulnerabilities.
- Document thoroughly: Keep detailed documentation of your integrations to facilitate maintenance and onboarding of new team members.
By following these guidelines and leveraging the power of various technologies, you can take your Java applications to new heights. The world of technology is ever-evolving, so stay curious, keep learning, and don’t be afraid to experiment with new integrations. Happy coding!
Disclaimer: The code examples and integrations described in this blog post are for educational purposes only. Always refer to the official documentation of each technology for the most up-to-date and accurate information. If you notice any inaccuracies or have suggestions for improvements, please report them so we can correct them promptly.