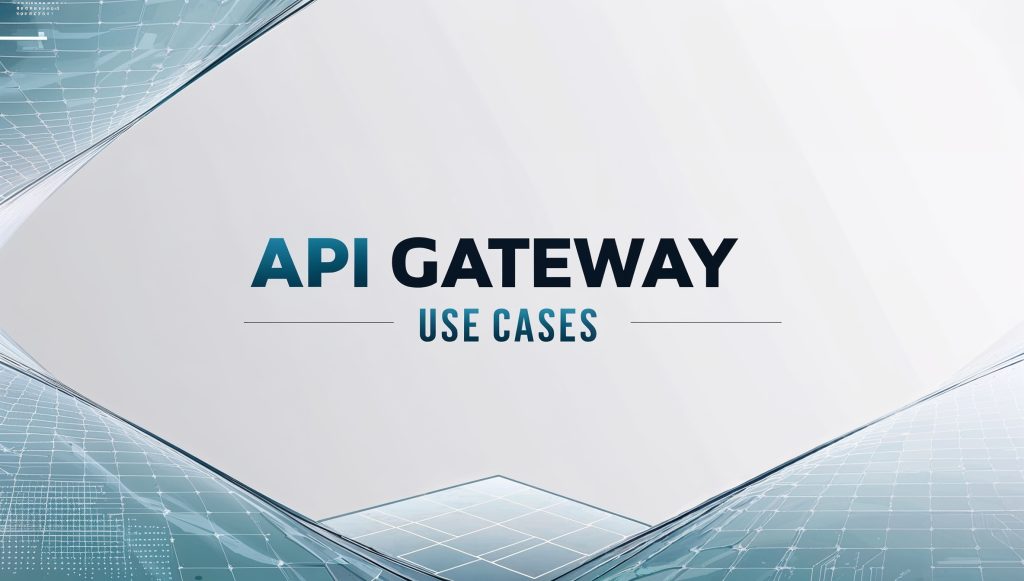
Unlocking the Power of API Gateways – API Gateway Use Cases
Hey there, fellow tech enthusiasts! Today, we’re going to embark on an exciting journey through the world of API gateways. If you’ve been wondering how these powerful tools can revolutionize your software architecture, you’re in for a treat. We’ll explore a variety of use cases that showcase the versatility and importance of API gateways in modern software development. So, grab your favorite beverage, get comfortable, and let’s dive in!
What’s All the Buzz About API Gateways?
Before we jump into the nitty-gritty of use cases, let’s take a moment to understand what an API gateway is and why it’s become such a hot topic in the tech world. An API gateway acts as a traffic cop for your microservices architecture, managing and routing requests to the appropriate services. It’s like having a super-smart receptionist for your APIs, handling everything from authentication to load balancing. But that’s just scratching the surface – API gateways are capable of so much more, and that’s exactly what we’re here to explore.
Security First: Protecting Your Digital Assets
In today’s interconnected world, security is paramount. API gateways play a crucial role in safeguarding your digital assets and ensuring that only authorized users can access your services. Let’s look at some key security-related use cases:
Authentication and Authorization
One of the primary security functions of an API gateway is to handle authentication and authorization. Instead of implementing these features in each of your microservices, you can centralize this functionality in the gateway. This approach not only simplifies your architecture but also ensures consistent security across all your services.
Here’s a simple example of how you might implement JWT (JSON Web Token) authentication in a Java-based API gateway using Spring Cloud Gateway:
@Configuration
public class SecurityConfig {
@Bean
public SecurityWebFilterChain springSecurityFilterChain(ServerHttpSecurity http) {
return http
.authorizeExchange()
.pathMatchers("/public/**").permitAll()
.anyExchange().authenticated()
.and()
.oauth2ResourceServer()
.jwt()
.and()
.build();
}
}
This configuration sets up a security filter chain that allows public access to paths starting with “/public/” and requires authentication for all other routes. It also configures the gateway to validate JWT tokens.
Rate Limiting and Throttling
Another critical security feature provided by API gateways is the ability to implement rate limiting and throttling. These mechanisms help protect your services from abuse, whether intentional (like DDoS attacks) or unintentional (like a buggy client application).
Let’s say you want to limit each client to 100 requests per minute. Here’s how you might configure that in Spring Cloud Gateway:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("rate_limit_route", r -> r
.path("/api/**")
.filters(f -> f
.requestRateLimiter(c -> c
.setRateLimiter(redisRateLimiter())
.setKeyResolver(userKeyResolver())))
.uri("lb://backend-service"))
.build();
}
@Bean
public RedisRateLimiter redisRateLimiter() {
return new RedisRateLimiter(100, 100);
}
@Bean
KeyResolver userKeyResolver() {
return exchange -> Mono.just(exchange.getRequest().getRemoteAddress().getAddress().getHostAddress());
}
This configuration sets up a route that applies rate limiting to all paths starting with “/api/”. It uses Redis to track request counts and limits each IP address to 100 requests per minute.
SSL/TLS Termination
SSL/TLS termination is another important security feature often handled by API gateways. By terminating SSL/TLS connections at the gateway level, you can offload the computationally expensive process of encryption and decryption from your backend services. This not only improves performance but also simplifies certificate management.
While the exact implementation details will depend on your specific API gateway and infrastructure, the concept remains the same: the gateway handles the secure connection with clients, and then communicates with backend services over a secure internal network.
Performance Optimization: Speeding Up Your APIs
API gateways aren’t just about security – they can also significantly boost the performance of your applications. Let’s explore some performance-related use cases:
Caching
Caching is a powerful technique for improving API performance, and API gateways are ideally positioned to implement it. By caching responses at the gateway level, you can reduce the load on your backend services and improve response times for frequently requested data.
Here’s an example of how you might configure caching in Spring Cloud Gateway:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("cache_route", r -> r
.path("/api/cached/**")
.filters(f -> f
.cache(c -> c
.keyResolver(new HeaderKeyResolver("x-user-id"))
.timeToLive(Duration.ofMinutes(5))))
.uri("lb://backend-service"))
.build();
}
This configuration sets up a route that caches responses for paths starting with “/api/cached/”. The cache key is based on the “x-user-id” header, and cached responses expire after 5 minutes.
Load Balancing
Load balancing is another crucial performance optimization that API gateways can provide. By distributing incoming requests across multiple instances of your backend services, you can improve both performance and reliability.
Spring Cloud Gateway integrates seamlessly with Spring Cloud LoadBalancer. Here’s a simple example:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("load_balanced_route", r -> r
.path("/api/**")
.uri("lb://backend-service"))
.build();
}
In this configuration, the “lb://” prefix in the URI tells Spring Cloud Gateway to use load balancing when routing requests to the backend service.
Request Aggregation
In a microservices architecture, a single client request might require data from multiple backend services. API gateways can aggregate these requests, reducing the number of round trips between the client and your services.
While Spring Cloud Gateway doesn’t provide built-in support for request aggregation, you can implement this functionality using a custom filter. Here’s a simplified example:
@Component
public class AggregatorFilter implements GatewayFilter, Ordered {
@Autowired
private WebClient.Builder webClientBuilder;
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
if (request.getPath().value().equals("/aggregate")) {
return aggregateResponses(exchange);
}
return chain.filter(exchange);
}
private Mono<Void> aggregateResponses(ServerWebExchange exchange) {
Mono<String> service1Response = callService("http://service1/api");
Mono<String> service2Response = callService("http://service2/api");
return Mono.zip(service1Response, service2Response)
.map(tuple -> {
String aggregatedResponse = "{\"service1\":" + tuple.getT1() +
",\"service2\":" + tuple.getT2() + "}";
return aggregatedResponse;
})
.flatMap(aggregatedResponse -> {
exchange.getResponse().getHeaders().setContentType(MediaType.APPLICATION_JSON);
return exchange.getResponse().writeWith(Mono.just(exchange.getResponse()
.bufferFactory().wrap(aggregatedResponse.getBytes())));
});
}
private Mono<String> callService(String url) {
return webClientBuilder.build()
.get()
.uri(url)
.retrieve()
.bodyToMono(String.class);
}
@Override
public int getOrder() {
return Ordered.LOWEST_PRECEDENCE;
}
}
This filter intercepts requests to “/aggregate”, calls two backend services, and combines their responses into a single JSON object.
Flexibility and Scalability: Adapting to Changing Needs
One of the greatest strengths of API gateways is their ability to provide flexibility and scalability to your architecture. Let’s explore some use cases that highlight these capabilities:
API Versioning
As your APIs evolve, you’ll likely need to support multiple versions simultaneously. API gateways can help manage this complexity by routing requests to the appropriate version of your service based on the client’s needs.
Here’s an example of how you might implement API versioning in Spring Cloud Gateway:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("v1_route", r -> r
.path("/v1/**")
.filters(f -> f.stripPrefix(1))
.uri("lb://service-v1"))
.route("v2_route", r -> r
.path("/v2/**")
.filters(f -> f.stripPrefix(1))
.uri("lb://service-v2"))
.build();
}
This configuration routes requests starting with “/v1/” to one service and requests starting with “/v2/” to another, allowing you to maintain multiple versions of your API simultaneously.
Protocol Translation
API gateways can act as a bridge between different protocols, allowing clients to communicate with your services using the protocol that best suits their needs. For example, you might have REST clients communicating with gRPC backend services.
While Spring Cloud Gateway doesn’t provide built-in support for gRPC, you can implement this functionality using a custom filter. Here’s a conceptual example:
@Component
public class RestToGrpcFilter implements GatewayFilter, Ordered {
@Autowired
private GrpcClient grpcClient;
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
if (request.getPath().value().equals("/grpc-service")) {
return handleGrpcRequest(exchange);
}
return chain.filter(exchange);
}
private Mono<Void> handleGrpcRequest(ServerWebExchange exchange) {
// Extract data from REST request
String data = extractDataFromRequest(exchange.getRequest());
// Make gRPC call
return grpcClient.callService(data)
.flatMap(grpcResponse -> {
// Convert gRPC response to REST response
String restResponse = convertToRestResponse(grpcResponse);
exchange.getResponse().getHeaders().setContentType(MediaType.APPLICATION_JSON);
return exchange.getResponse().writeWith(Mono.just(exchange.getResponse()
.bufferFactory().wrap(restResponse.getBytes())));
});
}
// ... implementation of extractDataFromRequest and convertToRestResponse methods
@Override
public int getOrder() {
return Ordered.LOWEST_PRECEDENCE;
}
}
This filter intercepts REST requests to “/grpc-service”, translates them to gRPC calls, and then converts the gRPC response back to a REST response.
A/B Testing
API gateways can facilitate A/B testing by routing a portion of traffic to different versions of your service. This allows you to test new features or optimizations with a subset of your users.
Here’s an example of how you might implement A/B testing in Spring Cloud Gateway:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("ab_test_route", r -> r
.path("/api/**")
.filters(f -> f
.requestWeight("group-a", 75)
.requestWeight("group-b", 25))
.uri("lb://backend-service"))
.build();
}
This configuration routes 75% of requests to “group-a” and 25% to “group-b”, allowing you to compare the performance or user satisfaction between two versions of your service.
Monitoring and Analytics: Gaining Insights from Your APIs
API gateways are not just about routing requests – they can also provide valuable insights into how your APIs are being used. Let’s explore some monitoring and analytics use cases:
Logging and Tracing
Centralized logging and tracing are essential for understanding the behavior of your distributed system. API gateways can capture detailed information about each request, including timing data, headers, and response codes.
Here’s an example of how you might implement logging in Spring Cloud Gateway:
@Component
public class LoggingFilter implements GlobalFilter, Ordered {
private static final Logger log = LoggerFactory.getLogger(LoggingFilter.class);
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
log.info("Request: {} {}", request.getMethod(), request.getURI());
return chain.filter(exchange)
.then(Mono.fromRunnable(() -> {
ServerHttpResponse response = exchange.getResponse();
log.info("Response: {}", response.getStatusCode());
}));
}
@Override
public int getOrder() {
return Ordered.LOWEST_PRECEDENCE;
}
}
This global filter logs information about each request and response passing through the gateway.
Metrics Collection
API gateways can collect a wide range of metrics, from simple request counts to detailed performance data. These metrics can be invaluable for monitoring the health and performance of your APIs.
Spring Cloud Gateway integrates with Micrometer, making it easy to collect and export metrics. Here’s an example configuration:
@Configuration
public class MetricsConfig {
@Bean
MeterRegistryCustomizer<MeterRegistry> metricsCommonTags() {
return registry -> registry.config().commonTags("application", "api-gateway");
}
@Bean
public TimedAspect timedAspect(MeterRegistry registry) {
return new TimedAspect(registry);
}
}
With this configuration in place, you can use the @Timed
annotation to measure the execution time of methods in your gateway.
Real-time Analytics
By analyzing the data flowing through your API gateway in real-time, you can gain valuable insights into user behavior, detect anomalies, and make data-driven decisions.
While implementing real-time analytics typically involves integrating with external systems, you can start by publishing events from your gateway. Here’s a conceptual example:
@Component
public class AnalyticsFilter implements GlobalFilter, Ordered {
@Autowired
private KafkaTemplate<String, String> kafkaTemplate;
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
// Publish event to Kafka
kafkaTemplate.send("api-events",
String.format("Request: %s %s", request.getMethod(), request.getURI()));
return chain.filter(exchange);
}
@Override
public int getOrder() {
return Ordered.LOWEST_PRECEDENCE;
}
}
This filter publishes an event to a Kafka topic for each request, which can then be consumed by a real-time analytics system.
Harnessing the Full Potential of API Gateways
As we’ve seen throughout this deep dive, API gateways are incredibly versatile tools that can address a wide range of challenges in modern software architecture. From enhancing security and optimizing performance to providing flexibility and valuable insights, API gateways have become an essential component of robust, scalable systems.
By leveraging these use cases and adapting them to your specific needs, you can create a more secure, efficient, and manageable API ecosystem. Remember, the examples we’ve explored are just the tip of the iceberg – the true power of API gateways lies in their flexibility and extensibility. As your system evolves, you’ll likely discover new and innovative ways to utilize your API gateway to solve complex problems and streamline your architecture.
As we wrap up this exploration of API gateway use cases, let’s recap some key takeaways:
- Security is paramount: API gateways provide a centralized point for implementing robust security measures, from authentication and authorization to rate limiting and SSL/TLS termination.
- Performance matters: Through features like caching, load balancing, and request aggregation, API gateways can significantly boost the performance and responsiveness of your APIs.
- Flexibility is key: API gateways enable you to adapt to changing requirements with ease, supporting scenarios like API versioning, protocol translation, and A/B testing.
- Insights drive improvement: By leveraging the monitoring and analytics capabilities of API gateways, you can gain valuable insights into your API usage and performance, enabling data-driven decision making.
- Centralization simplifies management: By centralizing cross-cutting concerns like security, monitoring, and routing, API gateways can significantly simplify the management of complex, distributed systems.
Embracing the Future of API Management
As we look to the future, it’s clear that API gateways will continue to play a crucial role in shaping the landscape of software architecture. With the rise of technologies like serverless computing, edge computing, and AI-driven analytics, API gateways are evolving to meet new challenges and unlock new possibilities.
Serverless Integration
API gateways are increasingly integrating with serverless platforms, allowing for seamless routing between traditional microservices and serverless functions. This opens up new possibilities for building highly scalable, event-driven architectures.
Here’s a conceptual example of how you might route requests to a serverless function using Spring Cloud Gateway:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("serverless_route", r -> r
.path("/serverless/**")
.filters(f -> f
.rewritePath("/serverless/(?<segment>.*)", "/${segment}")
.filter(new ServerlessFunctionFilter()))
.uri("http://serverless-platform"))
.build();
}
class ServerlessFunctionFilter implements GatewayFilter, Ordered {
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
// Logic to invoke serverless function
// This could involve making an HTTP request to a serverless platform's API
return chain.filter(exchange);
}
@Override
public int getOrder() {
return Ordered.HIGHEST_PRECEDENCE;
}
}
This configuration routes requests starting with “/serverless/” to a hypothetical serverless platform, with a custom filter to handle the invocation of serverless functions.
Edge Computing
As applications become more distributed, there’s a growing need to process data closer to its source. API gateways are expanding to support edge computing scenarios, allowing for low-latency processing and reduced bandwidth usage.
While implementing edge computing typically involves specialized infrastructure, you can start preparing your API gateway by implementing location-aware routing:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("edge_route", r -> r
.path("/edge/**")
.filter(new EdgeLocationFilter())
.uri("lb://edge-service"))
.build();
}
class EdgeLocationFilter implements GatewayFilter, Ordered {
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
String userLocation = exchange.getRequest().getHeaders().getFirst("X-User-Location");
String nearestEdgeLocation = determineNearestEdgeLocation(userLocation);
ServerHttpRequest request = exchange.getRequest().mutate()
.header("X-Edge-Location", nearestEdgeLocation)
.build();
return chain.filter(exchange.mutate().request(request).build());
}
private String determineNearestEdgeLocation(String userLocation) {
// Logic to determine the nearest edge location
// This could involve geolocation calculations or looking up predefined mappings
return "edge-location-1";
}
@Override
public int getOrder() {
return Ordered.HIGHEST_PRECEDENCE;
}
}
This example adds a custom header to route requests to the nearest edge location based on the user’s location.
AI-Driven API Management
As artificial intelligence and machine learning continue to advance, we’re seeing the emergence of AI-driven API management. This could involve using AI for anomaly detection, predictive scaling, or even automated API design and optimization.
While fully AI-driven API management is still emerging, you can start by implementing basic anomaly detection in your API gateway:
@Component
public class AnomalyDetectionFilter implements GlobalFilter, Ordered {
private final SimpleMovingAverage responseTimes = new SimpleMovingAverage(100);
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
long startTime = System.currentTimeMillis();
return chain.filter(exchange)
.then(Mono.fromRunnable(() -> {
long endTime = System.currentTimeMillis();
long responseTime = endTime - startTime;
double avgResponseTime = responseTimes.nextVal(responseTime);
if (responseTime > avgResponseTime * 2) {
log.warn("Anomaly detected: Response time {} ms is more than twice the moving average {} ms",
responseTime, avgResponseTime);
}
}));
}
@Override
public int getOrder() {
return Ordered.LOWEST_PRECEDENCE;
}
}
class SimpleMovingAverage {
private final int period;
private final Queue<Long> window = new LinkedList<>();
private long sum;
public SimpleMovingAverage(int period) {
this.period = period;
}
public double nextVal(long val) {
sum += val;
window.add(val);
if (window.size() > period) {
sum -= window.remove();
}
return sum / window.size();
}
}
This filter calculates a moving average of response times and logs a warning when a response time is more than twice the average, potentially indicating an anomaly.
As we conclude our journey through the world of API gateways, I hope you’re feeling inspired and equipped to leverage these powerful tools in your own projects. Remember, the use cases we’ve explored are just the beginning – the true potential of API gateways lies in how you apply them to solve your unique challenges and drive innovation in your organization.
So go forth, experiment, and push the boundaries of what’s possible with API gateways. The future of software architecture is in your hands, and it’s looking brighter than ever!
Disclaimer: The code examples provided in this blog post are for illustrative purposes only and may require additional configuration or dependencies to function in a production environment. Always ensure proper testing and security measures are in place before deploying any code to a live system. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.