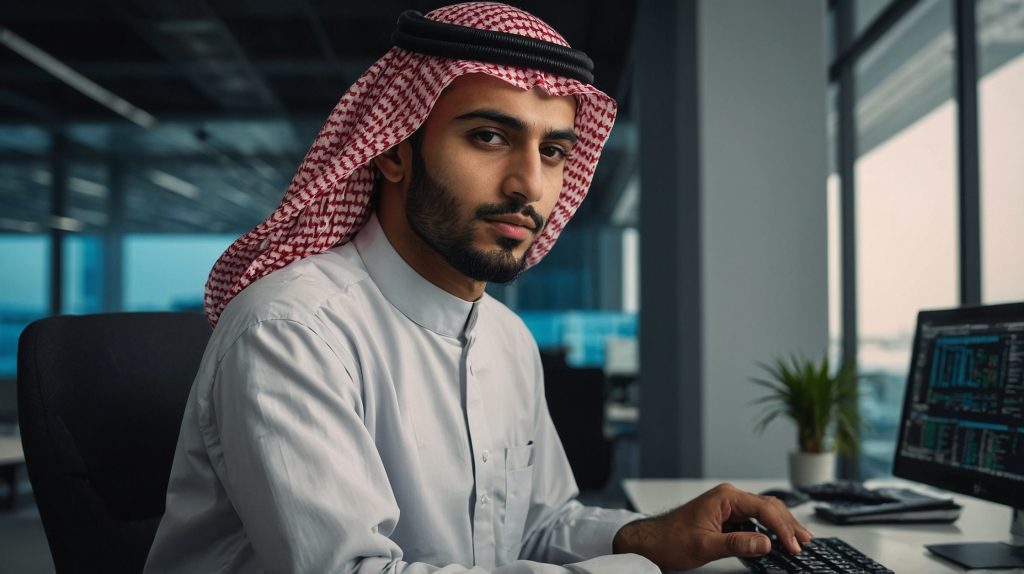
Common CI/CD Mistakes and How to Avoid Them
Today, we’re diving deep into the world of Continuous Integration and Continuous Deployment (CI/CD). If you’ve been in the tech game for a while, you know that CI/CD is like the secret sauce that keeps modern software development running smoothly. But here’s the thing: even the most seasoned pros can stumble when it comes to implementing and maintaining CI/CD pipelines. So, grab your favorite caffeinated beverage, and let’s explore some common CI/CD mistakes and, more importantly, how to steer clear of them.
The CI/CD Landscape: A Quick Refresher
Before we jump into the nitty-gritty of common mistakes, let’s take a moment to remind ourselves why CI/CD is such a big deal. In today’s fast-paced tech world, the ability to deliver high-quality software quickly and consistently is not just a nice-to-have – it’s a must-have. CI/CD pipelines automate the process of building, testing, and deploying code changes, allowing teams to catch bugs early, iterate faster, and maintain a steady flow of improvements to production.
But here’s the catch: setting up and maintaining an effective CI/CD pipeline isn’t always a walk in the park. It’s a complex beast that requires careful planning, constant attention, and a willingness to learn from mistakes. And trust me, there are plenty of mistakes to learn from!
Mistake #1: Neglecting Test Coverage
The Pitfall of Incomplete Testing
Picture this: you’ve set up your CI pipeline, and it’s humming along nicely. Code changes are being integrated smoothly, and you’re feeling pretty good about your setup. But then, disaster strikes. A critical bug slips through to production, causing downtime and frustrated users. What went wrong? More often than not, the culprit is inadequate test coverage.
Many teams fall into the trap of focusing solely on the “continuous integration” part of CI/CD, neglecting the crucial role that comprehensive testing plays in the process. They might run a few unit tests and call it a day, leaving gaping holes in their test coverage. This approach is like building a house with a solid foundation but forgetting to put a roof on it – you’re just asking for trouble.
Building a Robust Testing Strategy
So, how do we avoid this common pitfall? The key is to develop a comprehensive testing strategy that covers all bases. Here’s what that might look like:
- Unit Tests: Start with the basics. Ensure that individual components of your code are working as expected.
- Integration Tests: Don’t stop at unit tests. Make sure different parts of your application play nicely together.
- Functional Tests: Verify that your application meets the specified requirements and behaves correctly from a user’s perspective.
- Performance Tests: Don’t forget about speed and scalability. Include tests that check your application’s performance under various conditions.
- Security Tests: In today’s threat landscape, security testing is non-negotiable. Include automated security scans in your pipeline.
Here’s a simple example of how you might structure your testing stages in a CI/CD pipeline using a YAML configuration:
stages:
- build
- unit_test
- integration_test
- functional_test
- performance_test
- security_test
- deploy
build:
stage: build
script:
- ./build_script.sh
unit_test:
stage: unit_test
script:
- ./run_unit_tests.sh
integration_test:
stage: integration_test
script:
- ./run_integration_tests.sh
functional_test:
stage: functional_test
script:
- ./run_functional_tests.sh
performance_test:
stage: performance_test
script:
- ./run_performance_tests.sh
security_test:
stage: security_test
script:
- ./run_security_scans.sh
deploy:
stage: deploy
script:
- ./deploy_to_production.sh
only:
- main
Remember, the goal isn’t just to have a lot of tests – it’s to have meaningful tests that give you confidence in your code. Regularly review and update your test suite to ensure it’s keeping pace with your evolving application.
Mistake #2: Ignoring Environment Parity
The Danger of “It Works on My Machine”
We’ve all been there. You’ve developed a feature, tested it thoroughly on your local machine, and everything works perfectly. You push your changes, the CI pipeline runs green, and you deploy to production with confidence. But then, chaos ensues. The feature that worked flawlessly in development is now causing havoc in production. Welcome to the world of environment disparity.
One of the most insidious CI/CD mistakes is failing to maintain parity between development, staging, and production environments. It’s easy to fall into the trap of thinking that if something works in one environment, it’ll work in all of them. But the reality is often far messier.
Achieving True Environment Parity
So, how do we bridge this gap and ensure that our code behaves consistently across all environments? Here are some strategies to consider:
- Use Configuration Management Tools: Leverage tools like Ansible, Puppet, or Chef to define your infrastructure as code. This ensures that all environments are configured identically.
- Containerization: Docker containers can be a game-changer for maintaining environment parity. They allow you to package your application along with all its dependencies, ensuring consistency across different environments.
- Environment-Specific Configuration Files: Use configuration files to manage environment-specific settings, rather than hardcoding values.
- Automated Environment Provisioning: Set up scripts to automatically provision and configure your environments, reducing the risk of manual errors.
Let’s look at a simple example of how you might use Docker to maintain environment parity:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
With this Dockerfile, you can build a container that includes your application and all its dependencies. This container can then be run in any environment – development, staging, or production – ensuring consistency across the board.
Remember, achieving true environment parity is an ongoing process. Regularly audit your environments, update your configuration management scripts, and always test in an environment that’s as close to production as possible before deploying.
Mistake #3: Neglecting Security in the Pipeline
The Hidden Dangers of Insecure Pipelines
In the rush to implement CI/CD and speed up development cycles, security often takes a back seat. This is a dangerous oversight that can lead to severe consequences down the line. Your CI/CD pipeline has access to your codebase, deployment environments, and potentially sensitive data. If it’s not properly secured, it can become a prime target for attackers.
Many teams make the mistake of treating their CI/CD pipeline as just another development tool, failing to recognize it as a critical piece of infrastructure that requires robust security measures. This can lead to vulnerabilities like exposed secrets, insecure dependencies, and unauthorized access to production environments.
Building Security into Your CI/CD Pipeline
So, how do we ensure that our CI/CD pipeline is a fortress rather than a vulnerability? Here are some key strategies:
- Implement Least Privilege Access: Ensure that your CI/CD tools and processes have only the permissions they absolutely need. This limits the potential damage if a breach occurs.
- Secure Secrets Management: Never store secrets (like API keys or passwords) in your codebase or CI/CD configuration files. Instead, use a secure secrets management solution.
- Regular Security Scans: Incorporate automated security scans into your pipeline to catch vulnerabilities early.
- Secure Your Build Environments: Treat your build servers and agents with the same level of security as your production environments.
- Code Signing: Implement code signing to ensure the integrity of your artifacts throughout the pipeline.
Let’s look at an example of how you might incorporate security scans into your CI/CD pipeline using a popular tool like OWASP ZAP:
stages:
- build
- test
- security_scan
- deploy
build:
stage: build
script:
- ./build_script.sh
test:
stage: test
script:
- ./run_tests.sh
security_scan:
stage: security_scan
image: owasp/zap2docker-stable
script:
- zap-baseline.py -t https://your-staging-app.com -r security_report.html
artifacts:
paths: [security_report.html]
deploy:
stage: deploy
script:
- ./deploy_script.sh
only:
- main
In this example, we’re using OWASP ZAP to perform a baseline security scan against our staging environment before allowing a deployment to production. The scan results are saved as an artifact, allowing for easy review and tracking of security issues over time.
Remember, security isn’t a one-time task – it’s an ongoing process. Regularly review and update your security practices, stay informed about new threats, and foster a culture of security awareness within your team.
Mistake #4: Overlooking Monitoring and Observability
Flying Blind in Production
Picture this scenario: you’ve set up a slick CI/CD pipeline, your tests are passing with flying colors, and you’re deploying to production faster than ever. Everything seems great… until it’s not. Suddenly, users are reporting issues, but you have no idea what’s going wrong or where to start looking. Welcome to the world of inadequate monitoring and observability.
One of the most critical, yet often overlooked aspects of a robust CI/CD setup is the ability to monitor your applications effectively once they’re in production. Many teams focus so heavily on the “getting it out the door” part that they neglect to put proper systems in place to understand how their application is behaving in the wild.
Implementing Effective Monitoring and Observability
So, how do we avoid flying blind and ensure we have a clear picture of our application’s health and performance? Here are some key strategies:
- Implement Comprehensive Logging: Ensure your application is generating detailed, structured logs that can help you understand what’s happening under the hood.
- Set Up Real-Time Monitoring: Use tools that allow you to monitor key metrics in real-time, so you can spot issues as they arise.
- Implement Distributed Tracing: In microservices architectures, distributed tracing can be invaluable for understanding how requests flow through your system.
- Create Meaningful Dashboards: Develop dashboards that give you at-a-glance insights into the health and performance of your application.
- Set Up Alerting: Configure alerts for key metrics and error conditions, so you’re notified of issues before they impact users.
Let’s look at an example of how you might set up basic monitoring using Prometheus and Grafana in a Kubernetes environment:
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: prometheus
spec:
replicas: 1
selector:
matchLabels:
app: prometheus
template:
metadata:
labels:
app: prometheus
spec:
containers:
- name: prometheus
image: prom/prometheus:v2.30.3
ports:
- containerPort: 9090
---
apiVersion: v1
kind: Service
metadata:
name: prometheus
spec:
selector:
app: prometheus
ports:
- port: 9090
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: grafana
spec:
replicas: 1
selector:
matchLabels:
app: grafana
template:
metadata:
labels:
app: grafana
spec:
containers:
- name: grafana
image: grafana/grafana:8.2.2
ports:
- containerPort: 3000
---
apiVersion: v1
kind: Service
metadata:
name: grafana
spec:
selector:
app: grafana
ports:
- port: 3000
This YAML file sets up basic Prometheus and Grafana deployments in Kubernetes. Prometheus will collect metrics from your application, while Grafana provides a powerful interface for visualizing this data.
Remember, effective monitoring and observability is about more than just collecting data – it’s about deriving actionable insights from that data. Regularly review your monitoring setup, refine your dashboards, and ensure that the metrics you’re tracking align with your business and operational goals.
Mistake #5: Failing to Manage Dependencies Effectively
The Hidden Perils of Dependency Hell
In today’s software development landscape, we stand on the shoulders of giants. Almost every project relies on a multitude of third-party libraries and frameworks. While these dependencies can significantly speed up development, they can also introduce a whole new set of challenges when not managed properly.
Many teams make the mistake of treating dependency management as an afterthought. They might manually update dependencies on an ad-hoc basis, or worse, never update them at all. This can lead to a host of issues, from security vulnerabilities to compatibility problems, and can significantly complicate your CI/CD process.
Mastering Dependency Management
So, how do we tame the beast of dependency management and integrate it smoothly into our CI/CD pipeline? Here are some strategies to consider:
- Use a Dependency Management Tool: Leverage tools like npm for JavaScript, pip for Python, or Maven for Java to manage your dependencies effectively.
- Regularly Update Dependencies: Set up a schedule for reviewing and updating your dependencies. This helps you stay on top of security patches and new features.
- Use Version Pinning: Specify exact versions of dependencies to ensure consistency across environments.
- Implement Dependency Scanning: Incorporate tools that scan your dependencies for known vulnerabilities into your CI/CD pipeline.
- Maintain a Dependency Inventory: Keep track of all the dependencies your project uses, including their purposes and any associated licenses.
Let’s look at an example of how you might implement dependency scanning in a Node.js project using npm audit:
stages:
- build
- test
- security_scan
- deploy
build:
stage: build
script:
- npm install
test:
stage: test
script:
- npm test
security_scan:
stage: security_scan
script:
- npm audit
- if [ $(npm audit --json | jq '.metadata.vulnerabilities.high + .metadata.vulnerabilities.critical') -gt 0 ]; then exit 1; fi
deploy:
stage: deploy
script:
- ./deploy_script.sh
only:
- main
In this example, we’re using npm audit to check for vulnerabilities in our dependencies. If any high or critical vulnerabilities are found, the pipeline will fail, preventing the deployment of potentially insecure code.
Remember, effective dependency management is an ongoing process. Stay informed about updates and potential issues with your dependencies, and make dependency reviews a regular part of your development process.
Mistake #6: Ignoring Pipeline Performance
The Silent Productivity Killer
You’ve set up your CI/CD pipeline, and it’s dutifully building, testing, and deploying your code. But as your project grows, you start to notice something: your pipeline is getting slower… and slower… and slower. Before you know it, your team is spending more time waiting for the pipeline to finish than actually writing code. Welcome to the world of neglected pipeline performance.
Many teams make the mistake of setting up their CI/CD pipeline and then forgetting about it, assuming that if it’s working, it doesn’t need attention. But as your codebase grows and your tests multiply, an unoptimized pipeline can become a major bottleneck in your development process.
Optimizing Your CI/CD Pipeline
So, how do we ensure our pipeline stays lean and mean, even as our project scales? Here are some strategies to consider:
- Parallelize Where Possible: Run independent tasks concurrently to speed up overall execution time.
- Implement Caching: Cache dependencies and build artifacts to avoid unnecessary work in subsequent runs.
- Use Incremental Builds: Only rebuild what’s necessary based on what’s changed since the last run.
- Optimize Test Execution: Run the fastest tests first and use test splitting to distribute the load across multiple runners.
- Monitor and Analyze Pipeline Metrics: Keep track of pipeline execution times and resource usage to identify bottlenecks.
Let’s look at an example of how you might optimize a GitLab CI pipeline:
stages:
- build
- test
- deploy
variables:
CARGO_HOME: $CI_PROJECT_DIR/cargo
# Cache dependencies to speed up builds
cache:
paths:
- cargo/
- target/
build:
stage: build
script:
- cargo build --release
artifacts:
paths:
- target/release/myapp
# Run tests in parallel
test:
stage: test
parallel: 3
script:
- cargo test --partition $CI_NODE_INDEX/$CI_NODE_TOTAL
deploy:
stage: deploy
script:
- ./deploy.sh
only:
- main
In this example, we’re using caching to speed up dependency resolution, artifacts to pass build results between stages, and test parallelization to speed up the test execution.
Remember, pipeline performance isn’t a set-it-and-forget-it affair. Regularly review your pipeline’s performance metrics, and be prepared to make adjustments as your project evolves.
Mistake #7: Neglecting Rollback and Recovery Procedures
The Importance of Having an Escape Hatch
Picture this scenario: you’ve just deployed a new feature to production. Everything seemed fine in testing, but now users are reporting critical errors. Your team is scrambling to fix the issue, but in the meantime, your service is effectively down. If only you had a quick and reliable way to revert to the last known good state…
One of the most overlooked aspects of CI/CD is having robust rollback and recovery procedures. Many teams focus so heavily on moving forward that they forget to plan for when things go wrong. This can lead to extended downtime, data loss, and a whole lot of stress when issues inevitably arise in production.
Implementing Effective Rollback and Recovery
So, how do we ensure we’re not caught off guard when things go south? Here are some strategies to consider:
- Implement Blue-Green Deployments: This strategy involves having two identical production environments, with only one serving traffic at a time. This allows for quick rollbacks by simply switching traffic back to the previous environment.
- Use Feature Flags: Feature flags allow you to toggle features on and off without redeploying. This can be a lifesaver if a new feature is causing issues.
- Maintain Versioned Artifacts: Keep versioned copies of your application artifacts, so you can quickly deploy a previous version if needed.
- Automate Your Rollback Process: Don’t rely on manual steps in a crisis. Automate your rollback process so it can be triggered quickly and reliably.
- Regular Rollback Drills: Practice makes perfect. Regularly test your rollback procedures to ensure they work when you need them.
Let’s look at an example of how you might implement a simple rollback procedure in a bash script:
#!/bin/bash
# Define variables
DEPLOY_DIR="/var/www/myapp"
BACKUP_DIR="/var/www/myapp_backups"
TIMESTAMP=$(date +%Y%m%d_%H%M%S)
# Function to deploy new version
deploy_new_version() {
echo "Deploying new version..."
cp -r $DEPLOY_DIR $BACKUP_DIR/$TIMESTAMP
# Your deployment steps here
echo "Deployment complete."
}
# Function to rollback
rollback() {
echo "Rolling back to previous version..."
LATEST_BACKUP=$(ls -t $BACKUP_DIR | head -n1)
if [ -z "$LATEST_BACKUP" ]; then
echo "No backup found. Cannot rollback."
exit 1
fi
rm -rf $DEPLOY_DIR
cp -r $BACKUP_DIR/$LATEST_BACKUP $DEPLOY_DIR
echo "Rollback complete."
}
# Main script
case "$1" in
deploy)
deploy_new_version
;;
rollback)
rollback
;;
*)
echo "Usage: $0 {deploy|rollback}"
exit 1
;;
esac
exit 0
This script provides a simple way to deploy a new version while keeping a backup, and to rollback to the most recent backup if needed.
Remember, the goal isn’t just to have a rollback procedure – it’s to have one that you trust and can execute quickly under pressure. Regularly test and refine your rollback process to ensure it’s ready when you need it.
Conclusion: Embracing Continuous Improvement in CI/CD
As we wrap up our journey through common CI/CD mistakes, it’s important to remember that perfecting your CI/CD process is… well, a continuous process. The world of software development is always evolving, and your CI/CD pipeline needs to evolve with it.
The mistakes we’ve discussed – neglecting test coverage, ignoring environment parity, overlooking security, failing to implement proper monitoring, mismanaging dependencies, ignoring pipeline performance, and neglecting rollback procedures – are all too common. But they’re also all avoidable with the right mindset and approach.
Here are some key takeaways to keep in mind:
- Test, Test, and Test Again: Comprehensive testing is the backbone of a reliable CI/CD pipeline. Don’t skimp on it.
- Consistency is Key: Strive for consistency across all your environments to minimize surprises in production.
- Security is Not an Afterthought: Build security into your pipeline from the ground up.
- Visibility is Crucial: You can’t fix what you can’t see. Implement robust monitoring and observability practices.
- Manage Your Dependencies: Your project is only as strong as its weakest dependency. Keep them updated and secure.
- Performance Matters: An efficient pipeline is a happy pipeline. Regularly optimize for speed and resource usage.
- Always Have a Way Out: Implement and practice rollback procedures before you need them.
Remember, the goal of CI/CD isn’t just to deploy faster – it’s to deliver value to your users more efficiently and reliably. By avoiding these common mistakes and continuously refining your processes, you’ll be well on your way to CI/CD mastery.
So, go forth and automate, integrate, and deploy with confidence. And remember, in the world of CI/CD, the journey of improvement never really ends – and that’s part of what makes it so exciting!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, it should be understood that technology and best practices in the field of CI/CD are constantly evolving. The strategies and examples provided here are based on current industry standards and the author’s experience at the time of writing. Readers are encouraged to further research and adapt these concepts to their specific needs and circumstances. If you notice any inaccuracies or have suggestions for improvement, please report them so we can update the information promptly.