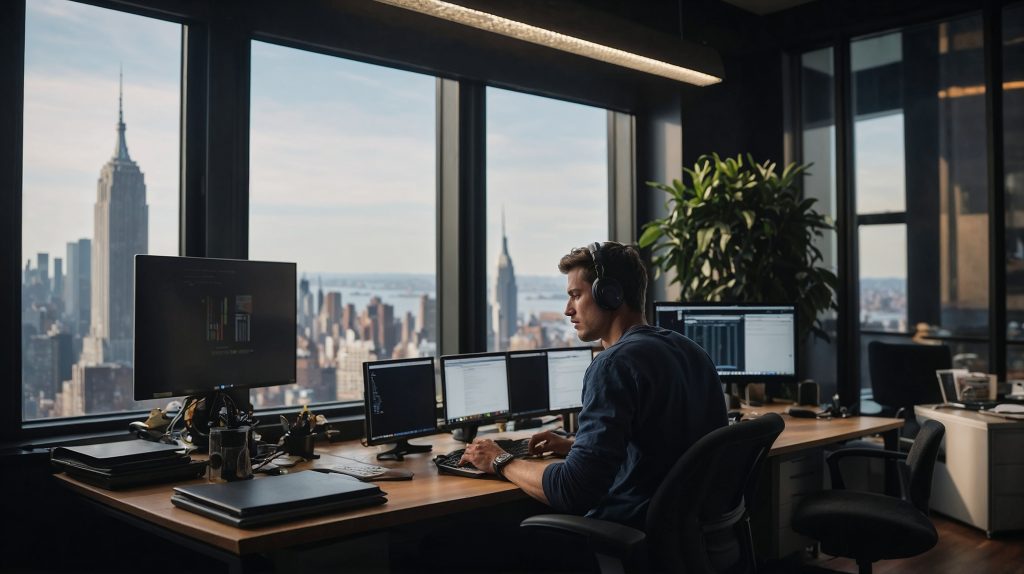
Setting Up Your First CI/CD Pipeline in Jenkins
Before we roll up our sleeves and get our hands dirty with Jenkins, let’s take a moment to understand what it is and why it’s such a big deal in the software development world. Jenkins is like that super-efficient, never-sleeping robot assistant you’ve always dreamed of having by your side. It automates the parts of software development related to building, testing, and deploying, facilitating continuous integration and continuous delivery. In simpler terms, Jenkins helps teams to work together more efficiently and deliver better software faster.
Imagine you’re working on a project with a team of developers. Without Jenkins, you might find yourself manually building and testing your code every time someone makes a change. That’s not only time-consuming but also prone to human error. Jenkins steps in to automate these repetitive tasks, allowing you to focus on what really matters – writing great code. It can automatically build your project, run tests, and even deploy your application to various environments. The best part? It does all this continuously, ensuring that your project is always in a deployable state.
Why Jenkins Stands Out:
Jenkins isn’t just another tool in the vast sea of development utilities. It’s popular for good reasons. First off, it’s open-source, which means it’s free to use and has a massive community contributing to its development and providing support. This community has also created thousands of plugins, extending Jenkins’ functionality to integrate with almost any tool you can think of in the software development lifecycle. Whether you’re using Git for version control, Docker for containerization, or AWS for cloud deployment, there’s likely a Jenkins plugin to make your life easier.
Moreover, Jenkins is incredibly flexible. It can be used for projects of all sizes and complexities. Whether you’re a solo developer working on a side project or part of a large enterprise team developing complex systems, Jenkins can be tailored to fit your needs. Its ability to distribute work across multiple machines helps in reducing build times and improving efficiency, which is crucial for large-scale projects.
Getting Started with Jenkins: Installation and Setup
Now that we understand why Jenkins is such a valuable tool, let’s roll up our sleeves and get it up and running on your machine. Don’t worry if you’re not a tech wizard – we’ll take it step by step, and before you know it, you’ll have Jenkins ready to go!
Step 1: Installing Java
First things first, Jenkins needs Java to run. It’s like fuel for our automation engine. Here’s how you can check if you have Java installed and install it if you don’t:
- Open your terminal or command prompt.
- Type
java -version
and hit Enter. - If you see version information, great! You have Java installed.
- If not, head over to the official Java website and download the latest version of Java Development Kit (JDK).
Step 2: Downloading and Installing Jenkins
Now that we have Java sorted, let’s get Jenkins on board:
- Visit the official Jenkins website (jenkins.io).
- Navigate to the download section and choose the package that matches your operating system.
- Once downloaded, run the installer and follow the prompts.
- During installation, you’ll be asked to choose a port number. The default is 8080, which is usually fine unless you have another application using that port.
Step 3: Accessing Jenkins for the First Time
Exciting times! You’re about to meet Jenkins face-to-face (well, screen-to-screen):
- Open your web browser and go to
http://localhost:8080
(or whatever port you chose during installation). - You’ll be greeted with a “Unlock Jenkins” screen. Don’t panic! Jenkins is just being security-conscious.
- You’ll need to enter an initial admin password. Jenkins will tell you where to find this password on your system. It’s usually in a file called
initialAdminPassword
. - Copy this password, paste it into the web interface, and click “Continue”.
Step 4: Customizing Your Jenkins Installation
Now, Jenkins will ask you how you’d like to set it up:
- Choose “Install suggested plugins” for a standard setup. This option installs a set of plugins that are commonly used and will be sufficient for most users.
- If you’re feeling adventurous or have specific needs, you can select “Select plugins to install” to customize your setup.
- Wait for the plugins to install. This might take a few minutes, so it’s a perfect time to refill your coffee!
Step 5: Creating Your Admin User
The final step in setting up Jenkins is creating your admin user:
- Fill in the details for your admin user account. This will be your main account for managing Jenkins, so make sure to use a strong password!
- Click “Save and Finish”.
- On the next page, click “Start using Jenkins”.
Congratulations! You’ve successfully installed and set up Jenkins. Take a moment to pat yourself on the back – you’ve just taken a huge step in modernizing your development workflow. In the next section, we’ll dive into creating your first Jenkins job and start building our CI/CD pipeline. Exciting times ahead!
Your First Jenkins Job: Hello, World!
Now that we have Jenkins up and running, let’s create our first job. We’ll start with something simple – a “Hello, World!” job. This will help us understand the basic structure of a Jenkins job and how to configure it.
Creating a New Job
- From the Jenkins dashboard, click on “New Item” in the left sidebar.
- Enter a name for your job, let’s call it “HelloWorld”.
- Select “Freestyle project” and click “OK”.
Configuring the Job
You’ll now see the job configuration page. This is where the magic happens! Let’s set it up:
- In the “Build” section, click on “Add build step” and select “Execute shell” (or “Execute Windows batch command” if you’re on Windows).
- In the command box that appears, type:
echo "Hello, World! This is my first Jenkins job!"
- Scroll down and click “Save”.
Running the Job
Congratulations! You’ve created your first Jenkins job. Now, let’s run it:
- Click on “Build Now” in the left sidebar.
- You should see a new build appear under “Build History”.
- Click on the build number, then click “Console Output” to see the results.
You should see your “Hello, World!” message in the console output. It’s a small step, but an important one – you’ve just automated a task with Jenkins!
Understanding Jenkins’ Core Concepts
Before we dive deeper into creating a CI/CD pipeline, let’s take a moment to understand some core concepts in Jenkins. This knowledge will serve as a foundation as we build more complex jobs and pipelines.
Jobs
Jobs (also called projects) are the fundamental unit of work in Jenkins. A job can be anything from a simple task like the “Hello, World!” example we just created, to a complex pipeline involving multiple steps and external systems. Jobs can be triggered manually, by external events (like a push to a Git repository), or on a schedule.
Builds
Every time a job runs, it creates a build. Each build has a unique number and keeps a record of what happened during that particular execution of the job. This includes things like the console output, any artifacts produced, and the status of the build (success, failure, or unstable).
Workspaces
Each job in Jenkins has a workspace, which is a directory on the Jenkins server where the job can do its work. This is where Jenkins checks out source code, compiles it, and runs tests. Understanding workspaces is crucial when you start dealing with more complex jobs that involve file manipulation.
Plugins
Plugins are what make Jenkins so versatile. They extend Jenkins’ functionality, allowing it to integrate with various tools and services. We installed some plugins during setup, but there are thousands more available. As you grow more comfortable with Jenkins, you’ll likely find yourself exploring and using various plugins to enhance your CI/CD pipeline.
Nodes and Executors
Jenkins can distribute builds across multiple machines, called nodes. The machine where Jenkins is installed is called the master node, and you can add additional slave nodes to distribute the workload. Each node has one or more executors, which determine how many jobs can run simultaneously on that node.
Pipeline
A pipeline in Jenkins is a suite of plugins that supports implementing and integrating continuous delivery pipelines into Jenkins. It provides an extensible set of tools for modeling simple-to-complex delivery pipelines “as code”. We’ll be diving deeper into pipelines in the next section.
Understanding these concepts will help you navigate Jenkins more effectively and make the most of its capabilities. As we move forward to create our CI/CD pipeline, you’ll see how these concepts come together to create a powerful automation workflow.
Building Your First CI/CD Pipeline
Now that we’ve got our feet wet with a simple job and understand the core concepts, let’s dive into creating a real CI/CD pipeline. We’ll create a pipeline for a simple Node.js application, taking it from source control all the way to deployment.
Step 1: Preparing Your Application
For this example, let’s assume you have a simple Node.js application in a Git repository. If you don’t have one, you can create a basic Express.js app. Here’s a simple app.js
file to get you started:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World from our CI/CD pipeline!');
});
app.listen(port, () => {
console.log(`App listening at http://localhost:${port}`);
});
Don’t forget to create a package.json
file and include a test script. Here’s a basic example:
{
"name": "my-cicd-app",
"version": "1.0.0",
"main": "app.js",
"scripts": {
"start": "node app.js",
"test": "echo 'Running tests...' && exit 0"
},
"dependencies": {
"express": "^4.17.1"
}
}
Push this code to your Git repository. We’ll use this as the basis for our pipeline.
Step 2: Creating a Pipeline Job in Jenkins
- From the Jenkins dashboard, click “New Item”.
- Enter a name for your pipeline (e.g., “MyApp-Pipeline”).
- Select “Pipeline” and click “OK”.
- In the configuration page, scroll down to the “Pipeline” section.
- Select “Pipeline script” from the “Definition” dropdown.
Step 3: Writing Your Pipeline Script
Now, let’s write our pipeline script. We’ll use the declarative pipeline syntax, which is more structured and easier to read:
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git 'https://github.com/yourusername/your-repo.git'
}
}
stage('Install Dependencies') {
steps {
sh 'npm install'
}
}
stage('Run Tests') {
steps {
sh 'npm test'
}
}
stage('Build') {
steps {
sh 'npm run build'
}
}
stage('Deploy') {
steps {
sh 'echo "Deploying application..."'
// Add actual deployment steps here
}
}
}
}
Let’s break down this pipeline:
- The
agent any
line tells Jenkins to run this pipeline on any available agent. - We have five stages: Checkout, Install Dependencies, Run Tests, Build, and Deploy.
- In the Checkout stage, we’re using the
git
step to clone our repository. - The Install Dependencies stage runs
npm install
to install our project dependencies. - The Run Tests stage executes our test script with
npm test
. - The Build stage would typically create a production build of our application. In a real-world scenario, this might involve transpiling, bundling, etc.
- The Deploy stage is where we’d put our deployment logic. For now, we’re just echoing a message.
Step 4: Running Your Pipeline
Save your pipeline configuration and click “Build Now”. Jenkins will execute each stage of your pipeline in order. You can watch the progress in the Stage View, which provides a visual representation of your pipeline execution.
Step 5: Interpreting the Results
After your pipeline runs, you’ll see the results of each stage. Green means success, red means failure. If a stage fails, the pipeline will typically stop at that point. This is incredibly useful for catching issues early in your development process.
You’ve just created your first CI/CD pipeline! This pipeline checks out your code, installs dependencies, runs tests, builds your application, and simulates a deployment. In a real-world scenario, you’d replace the echo in the Deploy stage with actual deployment logic, which might involve pushing to a production server, updating a Kubernetes cluster, or deploying to a cloud service.
Advanced Jenkins Pipeline Features
Now that we have a basic pipeline up and running, let’s explore some more advanced features that can make your pipeline even more powerful and flexible.
Using Environment Variables
Environment variables are a great way to make your pipeline more configurable and secure. You can define them at the pipeline level or within a stage:
pipeline {
agent any
environment {
NODE_ENV = 'production'
}
stages {
stage('Build') {
steps {
sh 'echo "Building for ${NODE_ENV} environment"'
}
}
}
}
Post Actions
The post
section lets you define actions that should occur after all stages have been executed, or after a specific condition:
pipeline {
agent any
stages {
// Your stages here
}
post {
always {
echo 'This will always run'
}
success {
echo 'This will run only if successful'
}
failure {
echo 'This will run only if failed'
}
}
}
Parallel Execution
For longer pipelines, you might want to run certain stages in parallel to speed things up:
stage('Test') {
parallel {
stage('Unit Tests') {
steps {
sh 'npm run test:unit'
}
}
stage('Integration Tests') {
steps {
sh 'npm run test:integration'
}
}
}
}
Input Steps
Sometimes, you might want human intervention in your pipeline. The input
step allows for this:
stage('Deploy to Production') {
steps {
input message: 'Deploy to production?'
sh './deploy.sh'
}
}
This will pause the pipeline and wait for user input before proceeding.
Using Shared Libraries
As your Jenkins setup grows, you might find yourself repeating code across different pipelines. Shared Libraries allow you to write reusable code that can be shared across multiple pipelines:
@Library('my-shared-library') _
pipeline {
agent any
stages {
stage('Build') {
steps {
mySharedBuildStep()
}
}
}
}
Here, mySharedBuildStep()
is a function defined in your shared library.
Best Practices for Jenkins Pipelines
As we wrap up our journey into Jenkins and CI/CD pipelines, let’s discuss some best practices that will help you create more efficient, maintainable, and secure pipelines.
Keep Your Pipeline Code in Version Control
Store your Jenkinsfile (the file containing your pipeline code) in your project’s Git repository. This practice, known as “Pipeline as Code,” allows you to version your pipeline along with your application code.
Use Declarative Syntax
While Jenkins supports both declarative and scripted pipeline syntax, declarative is generally easier to read and maintain, especially for complex pipelines.
Fail Fast
Structure your pipeline so that fast-running tests execute early. This helps identify issues quickly, saving time and resources.
Use Parameterized Builds
Parameterized builds allow you to pass variables to your pipeline at runtime, making your pipelines more flexible:
pipeline {
agent any
parameters {
string(name: 'DEPLOY_ENV', defaultValue: 'staging', description: 'Deployment environment')
}
stages {
stage('Deploy') {
steps {
sh "echo Deploying to ${params.DEPLOY_ENV}"
}
}
}
}
Secure Your Pipeline
Be careful about hardcoding sensitive information like passwords or API keys in your pipeline code. Instead, use Jenkins’ built-in credentials store:
stage('Deploy') {
steps {
withCredentials([usernamePassword(credentialsId: 'my-credentials-id', usernameVariable: 'USERNAME', passwordVariable: 'PASSWORD')]) {
sh "deploy.sh ${USERNAME} ${PASSWORD}"
}
}
}
Use Timeouts
Prevent your pipeline from hanging indefinitely by setting timeouts:
stage('Test') {
steps {
timeout(time: 1, unit: 'HOURS') {
sh 'npm test'
}
}
}
Clean Up Your Workspace
To prevent issues caused by leftover files from previous builds, consider cleaning your workspace at the beginning of each build:
pipeline {
agent any
options {
cleanWs()
}
stages {
// Your stages here
}
}
Troubleshooting Common Jenkins Issues
Even with the best setup, you might encounter issues with your Jenkins pipelines. Let’s look at some common problems and how to solve them.
Build Failures
If your build is failing, the first step is to check the console output. Jenkins provides detailed logs that can help identify the issue. Common causes of build failures include:
- Code issues: Syntax errors, failed tests, or compilation errors.
- Environment issues: Missing dependencies or incorrect environment variables.
- Resource issues: Not enough disk space or memory.
To resolve these, you might need to update your code, adjust your pipeline configuration, or allocate more resources to your Jenkins server.
Slow Builds
If your builds are taking too long, consider:
- Optimizing your tests: Can some tests be run in parallel? Are there unnecessary tests?
- Caching dependencies: Use Jenkins’ built-in caching mechanisms to avoid re-downloading dependencies for each build.
- Distributed builds: Use Jenkins’ distributed build feature to spread the workload across multiple machines.
Plugin Conflicts
With the vast array of plugins available for Jenkins, conflicts can sometimes occur. If you suspect a plugin is causing issues:
- Check the Jenkins system log for any error messages related to plugins.
- Try disabling recently added plugins to see if the issue resolves.
- Ensure all your plugins are up to date.
Connection Issues
If Jenkins is having trouble connecting to your source control or other external services:
- Check your network settings and firewall rules.
- Verify that the credentials Jenkins is using are correct and have not expired.
- Ensure the external service is accessible from the Jenkins server.
Remember, the Jenkins community is vast and helpful. If you’re stuck on an issue, don’t hesitate to seek help on the Jenkins forums or Stack Overflow.
Expanding Your Jenkins Knowledge
Congratulations! You’ve taken your first steps into the world of Jenkins and CI/CD pipelines. But this is just the beginning. As you continue your journey, here are some areas you might want to explore further:
1. Jenkins Configuration as Code
Jenkins Configuration as Code (JCasC) allows you to define your entire Jenkins configuration in a YAML file. This makes it easier to version control your Jenkins setup and quickly spin up new Jenkins instances.
2. Blue Ocean
Blue Ocean is a modern, visual pipeline editor for Jenkins. It provides a more user-friendly interface for creating and visualizing pipelines.
3. Docker Integration
Jenkins integrates well with Docker, allowing you to run your builds in isolated containers. This can help ensure consistency across different environments and simplify your build process.
4. Cloud Integration
Jenkins can be integrated with various cloud platforms like AWS, Google Cloud, and Azure. This allows you to dynamically spin up build agents in the cloud, providing more flexibility and scalability.
5. Security Best Practices
As you use Jenkins for more critical tasks, it’s important to understand how to secure your Jenkins instance. This includes topics like access control, securing credentials, and auditing.
Conclusion
We’ve covered a lot of ground in this blog post, from setting up Jenkins to creating your first CI/CD pipeline and exploring advanced features. Jenkins is a powerful tool that can significantly improve your development workflow, catching issues early and automating repetitive tasks.
Remember, the key to mastering Jenkins is practice. Start with simple pipelines and gradually add more complexity as you become more comfortable. Don’t be afraid to experiment – Jenkins is flexible enough to accommodate a wide variety of workflows and use cases.
As you continue your Jenkins journey, keep learning and stay up to date with the latest features and best practices. The world of CI/CD is constantly evolving, and Jenkins is evolving right along with it.
Happy automating!
Disclaimer: This blog post is intended for educational purposes only. While we strive for accuracy, technologies and best practices in the software development world can change rapidly. Always refer to the official Jenkins documentation for the most up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.