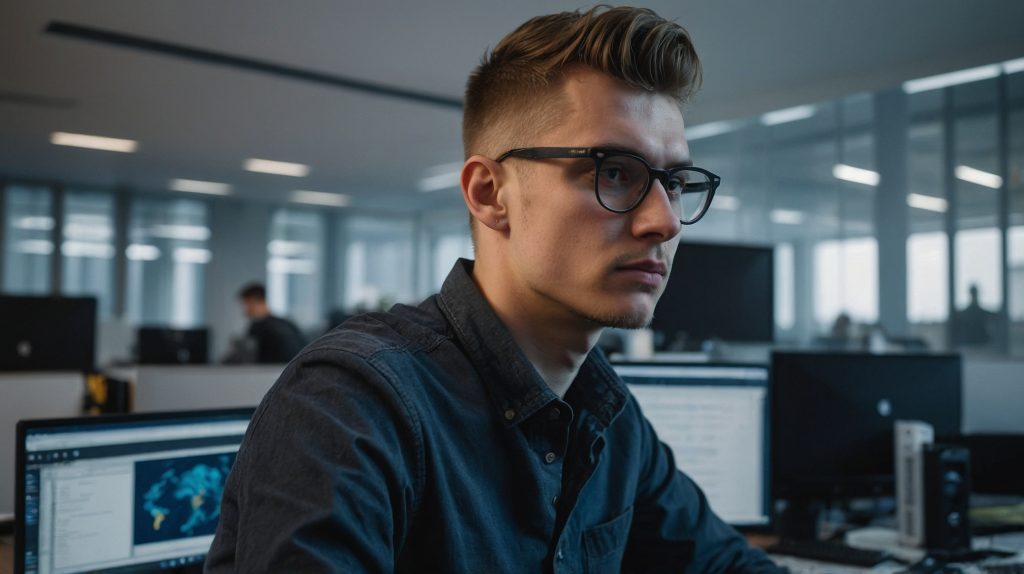
A Deep Dive into CI/CD for Modern Architectures
Buckle up, because we’re about to explore how Continuous Integration and Continuous Deployment (CI/CD) for microservices architecture can revolutionize your development process and take your microservices to the next level. Whether you’re a seasoned pro or just dipping your toes into the microservices pool, this guide has something for everyone. So, grab your favorite caffeinated beverage, and let’s dive in!
Understanding the Basics: CI/CD and Microservices Demystified
Before we get into the nitty-gritty, let’s make sure we’re all on the same page about what CI/CD and microservices actually mean. Think of this section as our foundation – we’ll build everything else on top of these core concepts.
What is CI/CD?
Continuous Integration (CI) and Continuous Deployment (CD) are like the dynamic duo of modern software development. CI is all about merging code changes frequently and automatically testing them to catch issues early. It’s like having a vigilant guard at the gate of your codebase, making sure only the good stuff gets through. CD takes things a step further by automatically deploying those verified changes to production or staging environments. Together, they form a seamless pipeline that takes your code from commit to production with minimal human intervention.
Imagine you’re baking a cake (stay with me here, I promise this analogy works). CI is like constantly tasting the batter as you add ingredients, making sure each addition improves the flavor. CD is like having a magical oven that not only bakes the cake perfectly but also serves it to your guests as soon as it’s ready. Pretty neat, right?
What are Microservices?
Now, let’s talk about microservices. If traditional monolithic applications are like a big, all-in-one Swiss Army knife, microservices are more like a well-organized toolbox. Each microservice is a small, independent service that focuses on doing one thing really well. These services communicate with each other through well-defined APIs, allowing for greater flexibility, scalability, and easier maintenance.
Think of it this way: instead of having one giant application that handles user authentication, data processing, and reporting, you’d have separate microservices for each of these functions. This modular approach allows teams to work independently, deploy frequently, and scale specific services as needed without affecting the entire system.
The Perfect Match: Why CI/CD and Microservices Are a Match Made in Tech Heaven
Now that we’ve got the basics down, you might be wondering, “Why are CI/CD and microservices such a great pair?” Well, my friend, they complement each other like peanut butter and jelly, Batman and Robin, or [insert your favorite iconic duo here].
Accelerated Development and Deployment
Microservices architecture breaks down your application into smaller, manageable pieces. This modular approach aligns perfectly with CI/CD practices. With CI/CD, you can independently build, test, and deploy each microservice, significantly speeding up your development and release cycles. Gone are the days of waiting for the entire monolithic application to be updated before pushing out new features or fixes.
Imagine you’re working on an e-commerce platform. With a microservices architecture and CI/CD pipeline, you could update the product recommendation service without touching the payment processing or inventory management services. This granular control allows for faster iterations and more frequent releases, keeping you ahead of the competition.
Enhanced Reliability and Fault Isolation
One of the biggest advantages of combining CI/CD with microservices is the improved reliability and fault isolation it offers. Since each microservice is developed, tested, and deployed independently, issues in one service are less likely to affect the entire system. Your CI pipeline can run targeted tests for each microservice, catching potential problems before they make it to production.
Let’s say you discover a bug in your user authentication service. In a monolithic application, fixing this could potentially impact the entire system. With microservices and a solid CI/CD pipeline, you can isolate the issue, fix it, and deploy the updated service without disrupting other functionalities. It’s like being able to replace a faulty light bulb without turning off the power to your entire house.
Scalability and Resource Optimization
Microservices shine when it comes to scalability, and CI/CD practices make scaling even smoother. Since each service can be deployed independently, you can easily scale specific components of your application based on demand. Your CI/CD pipeline can be configured to automatically deploy additional instances of a service when certain performance thresholds are met.
For example, during a flash sale on your e-commerce platform, you might need to scale up your product catalog and checkout services to handle increased traffic. With a well-implemented CI/CD pipeline for your microservices, this scaling can be automated, ensuring your application remains responsive even under heavy load.
Building Your CI/CD Pipeline for Microservices: A Step-by-Step Guide
Now that we’ve established why CI/CD and microservices are a match made in tech heaven, let’s roll up our sleeves and look at how to actually implement a CI/CD pipeline for your microservices architecture. Don’t worry; we’ll break it down into manageable steps and throw in some code examples to make things crystal clear.
Step 1: Version Control and Repository Structure
The foundation of any good CI/CD pipeline is a solid version control system. Git is the go-to choice for most teams, but the principles apply to other systems as well. When working with microservices, it’s crucial to have a well-organized repository structure.
There are two main approaches to structuring your microservices repositories:
- Monorepo: All microservices are stored in a single repository.
- Polyrepo: Each microservice has its own repository.
Both approaches have their pros and cons, and the choice often depends on your team size, project complexity, and organizational preferences. For this guide, we’ll use a polyrepo approach, as it aligns well with the independent nature of microservices.
Here’s an example of how your repository structure might look:
/
├── user-service/
├── product-service/
├── order-service/
├── payment-service/
└── recommendation-service/
Each service repository should contain its source code, tests, Dockerfile (for containerization), and CI/CD configuration files.
Step 2: Containerization with Docker
Containerization is a crucial step in creating a consistent and reproducible environment for your microservices. Docker is the most popular choice for containerization, so let’s look at a basic Dockerfile for one of our microservices:
# Use an official Node.js runtime as the base image
FROM node:14
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy the rest of the application code
COPY . .
# Expose the port the app runs on
EXPOSE 3000
# Define the command to run the app
CMD ["npm", "start"]
This Dockerfile sets up a Node.js environment, installs dependencies, and prepares the application to run. You’d create similar Dockerfiles for each of your microservices, adjusting as necessary for different languages or frameworks.
Step 3: Setting Up Continuous Integration
With our code structured and containerized, it’s time to set up the CI part of our pipeline. We’ll use GitHub Actions for this example, but the concepts are similar for other CI tools like Jenkins, GitLab CI, or CircleCI.
Create a .github/workflows/ci.yml
file in each microservice repository:
name: Continuous Integration
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm ci
- name: Run tests
run: npm test
- name: Build Docker image
run: docker build -t my-microservice .
- name: Run container tests
run: docker run my-microservice npm run test:integration
This workflow does the following:
- Triggers on pushes to the main branch or pull requests.
- Sets up a Node.js environment.
- Installs dependencies.
- Runs unit tests.
- Builds a Docker image.
- Runs integration tests inside the Docker container.
Step 4: Implementing Continuous Deployment
Now that we have our CI pipeline set up, let’s add the CD part. We’ll extend our GitHub Actions workflow to include deployment steps. This example assumes you’re deploying to a Kubernetes cluster:
# ... (previous CI steps)
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: us-west-2
- name: Login to Amazon ECR
id: login-ecr
uses: aws-actions/amazon-ecr-login@v1
- name: Build, tag, and push image to Amazon ECR
env:
ECR_REGISTRY: ${{ steps.login-ecr.outputs.registry }}
ECR_REPOSITORY: my-microservice
IMAGE_TAG: ${{ github.sha }}
run: |
docker build -t $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG .
docker push $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG
- name: Update Kubernetes deployment
run: |
aws eks get-token --cluster-name my-cluster | kubectl apply -f k8s/deployment.yml
This extended workflow:
- Configures AWS credentials (stored as GitHub secrets).
- Logs in to Amazon Elastic Container Registry (ECR).
- Builds and pushes the Docker image to ECR.
- Updates the Kubernetes deployment with the new image.
Remember to create the necessary Kubernetes deployment files (like k8s/deployment.yml
) for each microservice, specifying how it should be deployed and scaled in your cluster.
Step 5: Monitoring and Observability
A crucial part of any CI/CD pipeline, especially for microservices, is robust monitoring and observability. This helps you catch issues quickly and understand how your services are performing in production.
Consider integrating tools like:
- Prometheus for metrics collection
- Grafana for visualization
- ELK stack (Elasticsearch, Logstash, Kibana) for log management
- Jaeger or Zipkin for distributed tracing
Here’s a simple example of how you might add Prometheus metrics to a Node.js microservice:
const express = require('express');
const prometheus = require('prom-client');
const app = express();
// Create a Registry to register the metrics
const register = new prometheus.Registry();
// Create a counter metric
const httpRequestsTotal = new prometheus.Counter({
name: 'http_requests_total',
help: 'Total number of HTTP requests',
labelNames: ['method', 'path', 'status'],
registers: [register]
});
// Middleware to collect metrics
app.use((req, res, next) => {
res.on('finish', () => {
httpRequestsTotal.inc({
method: req.method,
path: req.path,
status: res.statusCode
});
});
next();
});
// Expose metrics endpoint
app.get('/metrics', async (req, res) => {
res.set('Content-Type', register.contentType);
res.end(await register.metrics());
});
// Your regular routes go here
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This setup creates a /metrics
endpoint that Prometheus can scrape to collect data about your service’s HTTP requests.
Best Practices for CI/CD in Microservices Architectures
Now that we’ve gone through the steps of setting up a CI/CD pipeline for microservices, let’s discuss some best practices to ensure your implementation is robust, efficient, and scalable. These tips will help you avoid common pitfalls and maximize the benefits of your CI/CD pipeline.
Embrace Infrastructure as Code (IaC)
When working with microservices and CI/CD, managing your infrastructure manually quickly becomes unsustainable. Embrace Infrastructure as Code (IaC) tools like Terraform, AWS CloudFormation, or Pulumi to define and manage your infrastructure. This approach ensures consistency across environments, makes your infrastructure version-controlled and reproducible, and allows for easy scaling and modifications.
Here’s a simple example of how you might define a Kubernetes namespace and a deployment for one of your microservices using Terraform:
provider "kubernetes" {
config_path = "~/.kube/config"
}
resource "kubernetes_namespace" "microservices" {
metadata {
name = "microservices"
}
}
resource "kubernetes_deployment" "user_service" {
metadata {
name = "user-service"
namespace = kubernetes_namespace.microservices.metadata[0].name
}
spec {
replicas = 3
selector {
match_labels = {
app = "user-service"
}
}
template {
metadata {
labels = {
app = "user-service"
}
}
spec {
container {
image = "your-registry/user-service:latest"
name = "user-service"
resources {
limits = {
cpu = "0.5"
memory = "512Mi"
}
requests = {
cpu = "250m"
memory = "50Mi"
}
}
port {
container_port = 8080
}
}
}
}
}
}
This Terraform configuration creates a namespace for your microservices and defines a deployment for the user service with three replicas. By using IaC, you can version control your infrastructure alongside your application code, making it easier to track changes and roll back if necessary.
Implement Feature Flags
Feature flags (also known as feature toggles) are a powerful technique that allows you to decouple deployment from release. They enable you to deploy code to production that isn’t yet ready for all users, giving you more control over feature rollouts and making it easier to perform A/B testing or canary releases.
Here’s a simple example of how you might implement feature flags in a Node.js microservice using the unleash-client
library:
const express = require('express');
const { initialize, isEnabled } = require('unleash-client');
const app = express();
// Initialize Unleash
initialize({
url: 'http://unleash.mycompany.com/api/',
appName: 'my-microservice',
instanceId: 'my-microservice-01',
});
app.get('/api/new-feature', (req, res) => {
if (isEnabled('newFeature')) {
res.send('Welcome to the new feature!');
} else {
res.send('This feature is not available yet.');
}
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, the /api/new-feature
endpoint checks if the newFeature
flag is enabled before deciding what response to send. You can control this flag through your Unleash dashboard, allowing you to gradually roll out the new feature to users without changing the code.
Automate Database Schema Changes
Managing database schema changes can be challenging in a microservices architecture, especially when you’re dealing with multiple services that may have their own databases. Automating these changes as part of your CI/CD pipeline can help ensure consistency and reduce the risk of errors during deployments.
Consider using database migration tools like Flyway, Liquibase, or Alembic to manage your schema changes. These tools allow you to version control your database schema and apply changes automatically during deployment.
Here’s an example of how you might set up Flyway migrations in a Java-based microservice:
import org.flywaydb.core.Flyway;
public class DatabaseMigration {
public static void migrate() {
Flyway flyway = Flyway.configure()
.dataSource("jdbc:postgresql://localhost:5432/mydb", "user", "password")
.load();
flyway.migrate();
}
}
You would then call this migrate()
method when your service starts up. Your migration scripts would be stored in a db/migration
directory in your project, with names like V1__Create_users_table.sql
:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(50) NOT NULL UNIQUE,
email VARCHAR(100) NOT NULL UNIQUE,
created_at TIMESTAMP WITH TIME ZONE DEFAULT CURRENT_TIMESTAMP
);
By integrating these migrations into your CI/CD pipeline, you ensure that your database schema is always in sync with your application code.
Implement Chaos Engineering
Chaos Engineering is the practice of intentionally introducing failures into your system to test its resilience. This is particularly important in microservices architectures, where there are many moving parts and potential points of failure.
Tools like Chaos Monkey (part of the Netflix Simian Army) or Gremlin can help you implement chaos engineering in your microservices environment. Here’s a simple example of how you might use the Chaos Monkey for Spring Boot in one of your Java microservices:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import de.codecentric.spring.boot.chaos.monkey.annotation.EnableChaosMonkey;
@SpringBootApplication
@EnableChaosMonkey
public class MyMicroserviceApplication {
public static void main(String[] args) {
SpringApplication.run(MyMicroserviceApplication.class, args);
}
}
With this setup, Chaos Monkey will randomly add latency to your service calls, throw exceptions, or kill your application. This helps you identify weaknesses in your system and improve its resilience.
Implement Blue-Green and Canary Deployments
Blue-green and canary deployments are strategies that can help you reduce risk when deploying new versions of your microservices. Blue-green deployments involve maintaining two identical production environments, with only one serving traffic at a time. Canary deployments involve gradually rolling out changes to a small subset of users before deploying to the entire infrastructure.
Here’s an example of how you might implement a blue-green deployment using Kubernetes:
apiVersion: v1
kind: Service
metadata:
name: my-service
spec:
selector:
app: my-app
version: blue
ports:
- protocol: TCP
port: 80
targetPort: 8080
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-blue
spec:
replicas: 3
selector:
matchLabels:
app: my-app
version: blue
template:
metadata:
labels:
app: my-app
version: blue
spec:
containers:
- name: my-app
image: my-registry/my-app:1.0
ports:
- containerPort: 8080
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-green
spec:
replicas: 3
selector:
matchLabels:
app: my-app
version: green
template:
metadata:
labels:
app: my-app
version: green
spec:
containers:
- name: my-app
image: my-registry/my-app:2.0
ports:
- containerPort: 8080
In this setup, you have two deployments (blue and green) and a service that initially points to the blue deployment. To switch to the green deployment, you would update the service’s selector:
spec:
selector:
app: my-app
version: green
This approach allows you to quickly roll back to the previous version if issues are detected with the new deployment.
Overcoming Common Challenges in CI/CD for Microservices
While CI/CD for microservices offers numerous benefits, it’s not without its challenges. Let’s discuss some common hurdles you might face and strategies to overcome them.
Managing Dependencies Between Microservices
As your microservices ecosystem grows, managing dependencies between services can become complex. Changes in one service might affect others, potentially breaking the entire system.
To address this:
- Implement robust API versioning to ensure backward compatibility.
- Use consumer-driven contract testing to catch integration issues early.
- Maintain comprehensive documentation of service dependencies.
Here’s an example of how you might implement API versioning in an Express.js application:
const express = require('express');
const app = express();
const v1Router = express.Router();
const v2Router = express.Router();
v1Router.get('/users', (req, res) => {
res.json({ version: 'v1', users: ['Alice', 'Bob'] });
});
v2Router.get('/users', (req, res) => {
res.json({ version: 'v2', users: [{ name: 'Alice', id: 1 }, { name: 'Bob', id: 2 }] });
});
app.use('/api/v1', v1Router);
app.use('/api/v2', v2Router);
app.listen(3000, () => console.log('Server running on port 3000'));
This setup allows you to maintain multiple versions of your API simultaneously, giving clients time to adapt to changes.
Ensuring Consistency Across Environments
Maintaining consistency across development, staging, and production environments can be challenging, especially when dealing with multiple microservices.
To address this:
- Use Infrastructure as Code (as discussed earlier) to ensure environment configurations are version-controlled and reproducible.
- Implement environment-specific configuration management.
- Use containerization to ensure consistency in runtime environments.
Here’s an example of how you might handle environment-specific configuration in a Node.js application:
const express = require('express');
const app = express();
// Load environment-specific configuration
const config = require(`./config/${process.env.NODE_ENV || 'development'}.js`);
app.get('/', (req, res) => {
res.send(`Hello from ${config.environmentName}!`);
});
app.listen(config.port, () => {
console.log(`Server running on port ${config.port}`);
});
With this setup, you can have separate configuration files for different environments (development.js, staging.js, production.js), ensuring that your application behaves correctly in each environment.
Managing Secrets and Sensitive Information
Securely managing secrets (like API keys, database credentials, etc.) becomes more complex in a microservices architecture with multiple deployment environments.
To address this:
- Use a secrets management tool like HashiCorp Vault or AWS Secrets Manager.
- Never store secrets in your version control system.
- Implement role-based access control (RBAC) to limit who can access sensitive information.
Here’s an example of how you might use AWS Secrets Manager in a Node.js application:
const AWS = require('aws-sdk');
const secretsManager = new AWS.SecretsManager();
async function getDatabaseCredentials() {
const params = {
SecretId: 'myapp/database-credentials'
};
try {
const data = await secretsManager.getSecretValue(params).promise();
return JSON.parse(data.SecretString);
} catch (error) {
console.error('Error retrieving secret:', error);
throw error;
}
}
async function connectToDatabase() {
const credentials = await getDatabaseCredentials();
// Use credentials to connect to the database
}
connectToDatabase();
This approach keeps your sensitive information out of your codebase and allows for easy rotation of secrets without code changes.
Final Thoughts
Implementing CI/CD for microservices architectures is no small feat, but the benefits are well worth the effort. By automating your build, test, and deployment processes, you can deliver high-quality software faster and more reliably than ever before.
Remember, the key to success lies in:
- Embracing automation at every step of the process
- Implementing robust testing strategies
- Using containerization for consistency
- Continuously monitoring and optimizing your pipeline
As you embark on your CI/CD journey, keep in mind that it’s not a destination, but a continuous process of improvement. Stay curious, keep learning, and don’t be afraid to experiment with new tools and techniques.
The world of microservices and CI/CD is constantly evolving, and what works best for your team today might change tomorrow. Stay flexible, keep an open mind, and always be ready to adapt your processes as your needs change and new technologies emerge.
So, are you ready to take your microservices to the next level with CI/CD? The future of software delivery is here, and it’s more exciting than ever. Happy coding, and may your deployments always be smooth and your services always available!
Disclaimer: This blog post is intended for educational purposes only. While we strive for accuracy, technologies and best practices in the field of CI/CD and microservices architectures are constantly evolving. Always refer to the most up-to-date documentation and consult with experts when implementing these practices in production environments. If you notice any inaccuracies in this post, please report them so we can correct them promptly.