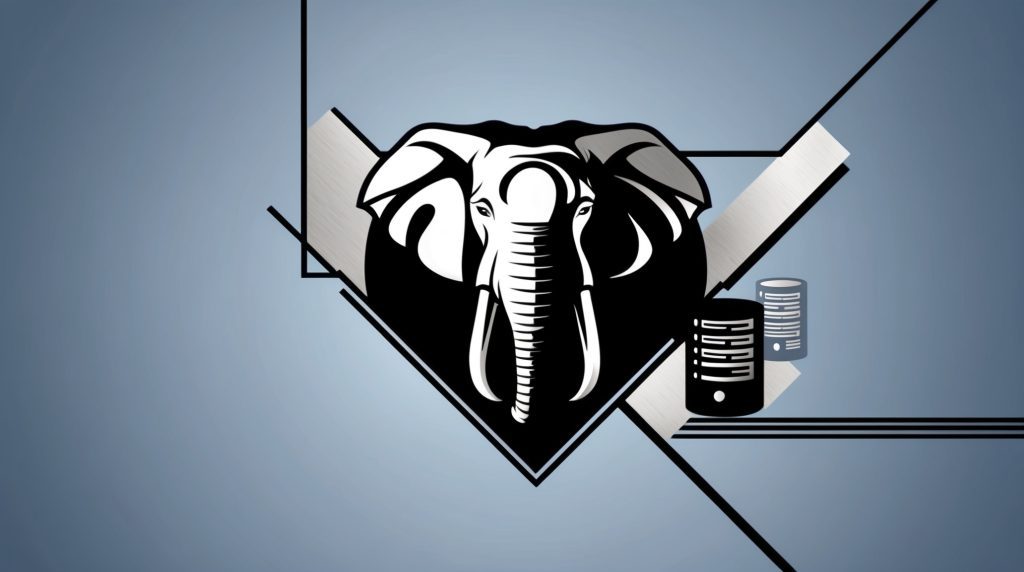
Essential PostgreSQL Commands Every Developer Should Know
PostgreSQL, often simply referred to as Postgres, is a powerful open-source relational database management system that has gained immense popularity among developers and organizations worldwide. Its robust features, extensibility, and adherence to SQL standards make it an excellent choice for a wide range of applications, from small-scale projects to large enterprise solutions. As a developer working with PostgreSQL, it’s crucial to have a solid understanding of the essential commands and concepts that form the foundation of database interactions. This comprehensive guide will walk you through the fundamental PostgreSQL commands, data types, and operators that every developer should be familiar with to effectively manage and manipulate data in their applications. By mastering these essential commands, you’ll be well-equipped to handle various database tasks, optimize queries, and build efficient database-driven applications. Whether you’re a beginner just starting with PostgreSQL or an experienced developer looking to refresh your knowledge, this blog post will serve as a valuable resource to enhance your database skills and productivity.
Connecting to PostgreSQL
Before diving into the commands, it’s essential to understand how to connect to a PostgreSQL database. There are multiple ways to interact with PostgreSQL, including the command-line interface (CLI) tool psql, graphical user interfaces (GUIs) like pgAdmin, and programmatic connections through various programming languages. For the purpose of this blog, we’ll focus on using psql, as it provides a direct and powerful way to interact with the database. To connect to a PostgreSQL database using psql, open your terminal or command prompt and use the following command:
psql -h hostname -p port -U username -d database_name
Replace hostname
, port
, username
, and database_name
with your specific connection details. If you’re connecting to a local PostgreSQL instance with default settings, you can simply use:
psql -U username -d database_name
Once connected, you’ll see the psql prompt, which typically looks like this:
database_name=>
This prompt indicates that you’re now connected to the specified database and ready to execute PostgreSQL commands.
Basic SQL Commands
PostgreSQL, like other relational database management systems, uses SQL (Structured Query Language) for interacting with the database. Let’s explore some of the most fundamental SQL commands that every developer should be familiar with.
SELECT Statement
The SELECT statement is used to retrieve data from one or more tables. It’s one of the most frequently used commands in SQL. Here’s the basic syntax:
SELECT column1, column2, ...
FROM table_name
WHERE condition;
For example, to retrieve all columns from a table named “employees”:
SELECT * FROM employees;
To select specific columns with a condition:
SELECT first_name, last_name, salary
FROM employees
WHERE department = 'IT';
The SELECT statement can be combined with various clauses and functions to perform complex queries, such as sorting, grouping, and joining tables.
INSERT Statement
The INSERT statement is used to add new rows of data into a table. The basic syntax is:
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...);
For example, to insert a new employee record:
INSERT INTO employees (first_name, last_name, email, hire_date, salary)
VALUES ('John', 'Doe', 'john.doe@example.com', '2023-01-15', 75000);
You can also insert multiple rows in a single statement:
INSERT INTO employees (first_name, last_name, email, hire_date, salary)
VALUES
('Jane', 'Smith', 'jane.smith@example.com', '2023-02-01', 80000),
('Mike', 'Johnson', 'mike.johnson@example.com', '2023-02-15', 72000);
UPDATE Statement
The UPDATE statement is used to modify existing data in a table. Its basic syntax is:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
For example, to update an employee’s salary:
UPDATE employees
SET salary = 85000
WHERE employee_id = 1001;
It’s crucial to use the WHERE clause carefully when updating records to avoid unintentionally modifying multiple rows.
DELETE Statement
The DELETE statement is used to remove rows from a table. The basic syntax is:
DELETE FROM table_name
WHERE condition;
For example, to delete an employee record:
DELETE FROM employees
WHERE employee_id = 1001;
As with the UPDATE statement, it’s essential to use the WHERE clause judiciously to prevent accidental deletion of multiple records.
Data Types in PostgreSQL
PostgreSQL supports a wide range of data types to accommodate various kinds of data. Understanding these data types is crucial for designing efficient database schemas and ensuring data integrity. Let’s explore some of the most commonly used data types in PostgreSQL.
Numeric Types
PostgreSQL offers several numeric data types to store numbers of different sizes and precision:
- INTEGER: Whole numbers between -2,147,483,648 and 2,147,483,647
- BIGINT: Large-range integer between -9,223,372,036,854,775,808 and 9,223,372,036,854,775,807
- NUMERIC or DECIMAL: Exact numeric values with user-specified precision
- REAL: Single precision floating-point number (4 bytes)
- DOUBLE PRECISION: Double precision floating-point number (8 bytes)
Example of using numeric types:
CREATE TABLE products (
product_id SERIAL PRIMARY KEY,
name VARCHAR(100),
price NUMERIC(10, 2),
weight REAL,
stock_quantity INTEGER
);
In this example, we use different numeric types for various attributes of a product.
Character Types
PostgreSQL provides several character data types to store textual data:
- CHAR(n): Fixed-length character string
- VARCHAR(n): Variable-length character string with a maximum length
- TEXT: Variable-length character string without a specified maximum length
Example of using character types:
CREATE TABLE users (
user_id SERIAL PRIMARY KEY,
username VARCHAR(50) UNIQUE,
password CHAR(60),
bio TEXT
);
In this example, we use VARCHAR for the username (with a maximum length of 50 characters), CHAR for a fixed-length password hash, and TEXT for a potentially long user biography.
Date and Time Types
PostgreSQL offers various data types to handle date and time information:
- DATE: Date (year, month, day)
- TIME: Time of day (hour, minute, second, microsecond)
- TIMESTAMP: Date and time (without time zone)
- TIMESTAMPTZ: Date and time with time zone
- INTERVAL: Time interval
Example of using date and time types:
CREATE TABLE events (
event_id SERIAL PRIMARY KEY,
event_name VARCHAR(100),
start_date DATE,
start_time TIME,
duration INTERVAL,
created_at TIMESTAMPTZ DEFAULT CURRENT_TIMESTAMP
);
This example demonstrates the use of different date and time types for managing event information.
Boolean Type
PostgreSQL provides a BOOLEAN data type to store true/false values:
CREATE TABLE tasks (
task_id SERIAL PRIMARY KEY,
description TEXT,
is_completed BOOLEAN DEFAULT FALSE
);
Array Type
PostgreSQL supports array types, allowing you to store multiple values of the same type in a single column:
CREATE TABLE products (
product_id SERIAL PRIMARY KEY,
name VARCHAR(100),
tags TEXT[]
);
INSERT INTO products (name, tags) VALUES ('Smartphone', ARRAY['electronics', 'mobile', 'gadget']);
These are just a few of the many data types available in PostgreSQL. Choosing the appropriate data type for each column in your tables is crucial for optimizing storage, ensuring data integrity, and improving query performance.
PostgreSQL Operators
Operators in PostgreSQL are used to perform various operations on data, such as comparisons, arithmetic calculations, and logical evaluations. Understanding these operators is essential for writing effective queries and manipulating data. Let’s explore some of the most commonly used operators in PostgreSQL.
Comparison Operators
Comparison operators are used to compare values and return boolean results:
- Equal (=)
- Not Equal (<> or !=)
- Less Than (<)
- Greater Than (>)
- Less Than or Equal (<=)
- Greater Than or Equal (>=)
Example usage:
SELECT * FROM employees WHERE salary > 50000;
SELECT * FROM products WHERE price BETWEEN 10 AND 50;
SELECT * FROM orders WHERE status <> 'Shipped';
Logical Operators
Logical operators are used to combine multiple conditions:
- AND
- OR
- NOT
Example usage:
SELECT * FROM employees WHERE department = 'Sales' AND salary > 60000;
SELECT * FROM products WHERE category = 'Electronics' OR category = 'Appliances';
SELECT * FROM customers WHERE NOT country = 'USA';
Arithmetic Operators
Arithmetic operators perform mathematical calculations:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulo (%)
Example usage:
SELECT product_name, price, price * 0.9 AS discounted_price FROM products;
SELECT order_id, total_amount, total_amount + (total_amount * 0.08) AS total_with_tax FROM orders;
String Operators
PostgreSQL provides several operators for working with string data:
- Concatenation (||)
- LIKE (pattern matching)
- ILIKE (case-insensitive pattern matching)
Example usage:
SELECT first_name || ' ' || last_name AS full_name FROM employees;
SELECT * FROM products WHERE name LIKE '%Phone%';
SELECT * FROM customers WHERE email ILIKE '%@gmail.com';
Array Operators
For working with array data types, PostgreSQL offers specific operators:
- Contains (@>)
- Is contained by (<@)
- Overlaps (&&)
Example usage:
SELECT * FROM products WHERE tags @> ARRAY['electronics'];
SELECT * FROM events WHERE attendees && ARRAY['John', 'Jane'];
JSON Operators
PostgreSQL has powerful support for JSON data, including several operators:
- -> (Get JSON object field as JSON)
- ->> (Get JSON object field as text)
- #> (Get JSON object at specified path)
- #>> (Get JSON object at specified path as text)
Example usage:
SELECT data->>'name' AS user_name FROM users WHERE data->>'age' = '30';
SELECT * FROM orders WHERE items @> '[{"product_id": 123}]'::jsonb;
Understanding and effectively using these operators will greatly enhance your ability to write complex and efficient queries in PostgreSQL. They allow you to perform sophisticated data manipulations, comparisons, and filtering operations, which are essential for extracting meaningful insights from your database.
Advanced PostgreSQL Commands
As you become more proficient with PostgreSQL, you’ll encounter more advanced commands and features that can significantly enhance your database management capabilities. Let’s explore some of these advanced commands and concepts.
Indexes
Indexes are crucial for improving query performance. They allow PostgreSQL to find and retrieve specific rows much faster than scanning the entire table. Here’s how you can create an index:
CREATE INDEX idx_employee_last_name ON employees (last_name);
This creates an index on the last_name column of the employees table. PostgreSQL supports various types of indexes, including B-tree, Hash, GiST, SP-GiST, GIN, and BRIN. Each type is optimized for different types of queries and data.
Views
Views are virtual tables based on the result of a SELECT query. They can simplify complex queries and provide an additional layer of security:
CREATE VIEW high_value_orders AS
SELECT order_id, customer_id, total_amount
FROM orders
WHERE total_amount > 1000;
You can then query this view as if it were a table:
SELECT * FROM high_value_orders WHERE customer_id = 123;
Stored Procedures
Stored procedures allow you to group one or more SQL statements into a named, reusable unit. In PostgreSQL, you can create stored procedures using PL/pgSQL:
CREATE OR REPLACE PROCEDURE transfer_funds(
sender_id INT,
recipient_id INT,
amount DECIMAL
)
LANGUAGE plpgsql
AS $$
BEGIN
-- Deduct amount from sender's account
UPDATE accounts SET balance = balance - amount
WHERE account_id = sender_id;
-- Add amount to recipient's account
UPDATE accounts SET balance = balance + amount
WHERE account_id = recipient_id;
COMMIT;
END;
$$;
You can then call this procedure using:
CALL transfer_funds(1001, 1002, 500.00);
Transactions
Transactions ensure that a series of SQL statements are executed as a single unit of work. If any statement within the transaction fails, all changes are rolled back:
BEGIN;
UPDATE accounts SET balance = balance - 500 WHERE account_id = 1001;
UPDATE accounts SET balance = balance + 500 WHERE account_id = 1002;
COMMIT;
If an error occurs between BEGIN and COMMIT, you can use ROLLBACK to undo the changes.
Window Functions
Window functions perform calculations across a set of rows that are related to the current row. They are powerful tools for analytics and reporting:
SELECT
employee_id,
department,
salary,
AVG(salary) OVER (PARTITION BY department) as avg_dept_salary,
salary - AVG(salary) OVER (PARTITION BY department) as salary_diff
FROM employees;
This query calculates the average salary for each department and the difference between each employee’s salary and their department’s average.
Common Table Expressions (CTEs)
CTEs provide a way to write auxiliary statements for use in a larger query. They can make complex queries more readable and maintainable:
WITH regional_sales AS (
SELECT region, SUM(amount) as total_sales
FROM orders
GROUP BY region
),
top_regions AS (
SELECT region
FROM regional_sales
WHERE total_sales > (SELECT SUM(total_sales) / 10 FROM regional_sales)
)
SELECT region, product, SUM(quantity) as product_units
FROM orders
WHERE region IN (SELECT region FROM top_regions)
GROUP BY region, product;
This query uses CTEs to first calculate regional sales, then identify top-performing regions, and finally report on product units sold in those regions.
Conclusion
PostgreSQL is a powerful and versatile database system that offers a wide range of features and capabilities. This blog post has covered the essential commands, data types, and operators that every developer working with PostgreSQL should know. From basic SQL operations like SELECT, INSERT, UPDATE, and DELETE to more advanced concepts like indexes, views, stored procedures, and window functions, we’ve explored the fundamental building blocks of effective database management with PostgreSQL.
By mastering these commands and concepts, you’ll be well-equipped to design efficient database schemas, write optimized queries, and build robust database-driven applications. Remember that PostgreSQL is continuously evolving, with new features and improvements being added in each release. As you grow more comfortable with these essentials, don’t hesitate to explore the PostgreSQL documentation for more advanced topics and cutting-edge features.
Whether you’re working on a small personal project or a large-scale enterprise application, the knowledge you’ve gained from this guide will serve as a solid foundation for your PostgreSQL journey. Keep practicing, experimenting with different scenarios, and staying updated with the latest PostgreSQL developments to become a proficient database developer.
Disclaimer: While every effort has been made to ensure the accuracy and completeness of the information presented in this blog post, database technologies and best practices may evolve over time. Readers are encouraged to consult the official PostgreSQL documentation for the most up-to-date and detailed information. If you notice any inaccuracies or have suggestions for improvement, please report them so we can promptly make the necessary corrections.