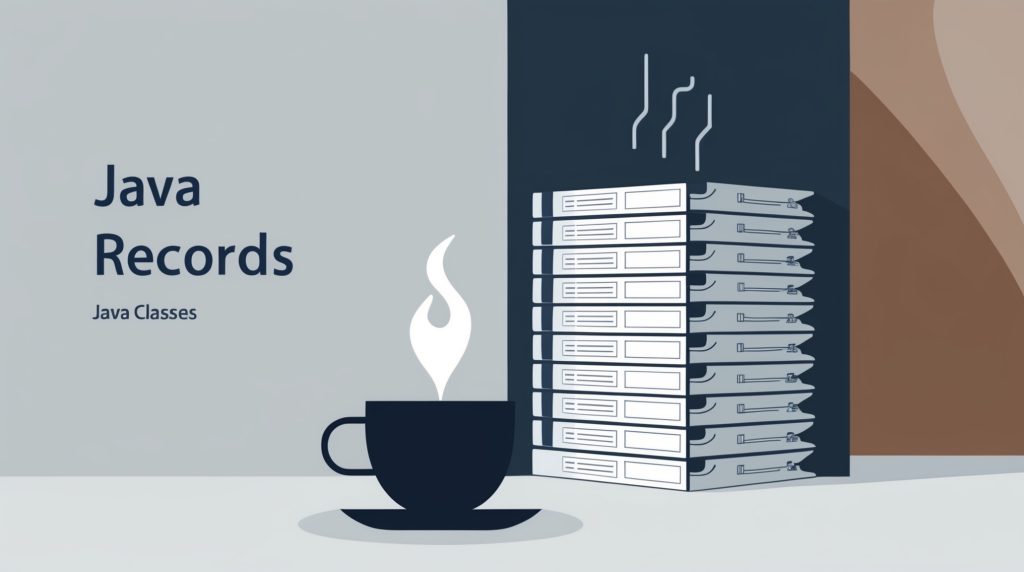
Exploring the Benefits and Use Cases of Java Records
Java Records, introduced as a preview feature in Java 14 and fully incorporated in Java 16, represent a significant evolution in how developers can create data-centric classes. This powerful addition to the Java language aims to simplify the process of declaring classes that are primarily used to store and transport immutable data. By reducing boilerplate code and enhancing readability, Java Records offer a concise and efficient way to model data objects. In this comprehensive exploration, we’ll delve into the intricacies of Java Records, examining their benefits, use cases, and how they compare to traditional Java classes. Whether you’re a seasoned Java developer or just starting your journey with the language, understanding Java Records can significantly streamline your code and improve your overall development experience.
What are Java Records?
Java Records are a special kind of class designed to serve as transparent carriers for immutable data. They provide a compact syntax for declaring classes that are intended to hold data without much additional functionality. The primary purpose of a record is to store data and provide a means to access it, without the need for extensive custom implementations of common methods like constructors, getters, equals(), hashCode(), and toString(). This concept aligns closely with what many developers refer to as “data transfer objects” (DTOs) or “value objects.”
Key Characteristics of Java Records:
- Immutability: By default, all fields in a record are final, ensuring that the data remains unchanged after initialization.
- Concise Syntax: Records significantly reduce the amount of boilerplate code typically required for data-centric classes.
- Automatic Method Generation: The compiler automatically generates methods like constructors, accessors, equals(), hashCode(), and toString().
- Transparency: The structure of a record is evident from its declaration, making it clear what data it contains.
- Serializable: Records implement the Serializable interface by default, facilitating easy data transfer across networks or storage.
Let’s look at a simple example to illustrate the syntax of a Java Record:
public record Person(String name, int age, String email) {}
This concise declaration is equivalent to a much longer traditional class that would include private final fields, a constructor, getter methods, and implementations of equals(), hashCode(), and toString(). The record syntax allows developers to focus on the essential aspects of the data structure without getting bogged down in repetitive code.
Benefits of Using Java Records
The introduction of Java Records brings several significant advantages to Java developers, enhancing both productivity and code quality. Let’s explore these benefits in detail:
1. Reduced Boilerplate Code
One of the most immediate and noticeable benefits of using Java Records is the dramatic reduction in boilerplate code. Traditional Java classes often require developers to write numerous lines of code for constructors, getter methods, equals(), hashCode(), and toString() implementations. With records, all of these are automatically generated by the compiler. This reduction in code volume not only saves time but also reduces the potential for errors that can occur when manually implementing these methods.
2. Improved Readability and Maintainability
The concise syntax of records makes the code more readable at a glance. Developers can quickly understand the structure and purpose of a record by looking at its declaration. This improved readability extends to maintainability as well. With less code to manage, there are fewer opportunities for bugs to creep in during updates or refactoring processes.
3. Enhanced Immutability
Java Records are designed with immutability in mind. All fields in a record are implicitly final, which promotes safer and more predictable code, especially in concurrent programming scenarios. Immutable objects are inherently thread-safe and can be freely shared across different parts of an application without concern for unexpected modifications.
4. Automatic Consistency Between Fields and Methods
In traditional classes, it’s easy for inconsistencies to arise between fields and their corresponding getter methods, especially as the class evolves over time. Records eliminate this problem by automatically generating accessor methods that directly correspond to the declared components. This ensures that the public API of the record always accurately reflects its internal state.
5. Simplified Equality Comparisons
The automatically generated equals() method in records compares all components, providing a straightforward and correct implementation of structural equality. This eliminates a common source of bugs in traditional Java classes where developers might forget to update the equals() method when adding new fields.
6. Easy-to-Use Data Transfer Objects
Records excel as data transfer objects (DTOs) in layered applications. Their concise syntax and automatic method generation make them ideal for passing data between different layers of an application, such as between the database layer and the service layer, or between a backend service and a frontend client.
7. Improved Performance
While not a primary goal of the feature, Java Records can lead to performance improvements in certain scenarios. The compiler has more information about the immutable nature of records, which can potentially enable optimizations that aren’t possible with regular classes.
8. Enhanced Pattern Matching
Records integrate well with pattern matching features introduced in recent Java versions. This synergy allows for more expressive and concise code when working with complex data structures.
Use Cases for Java Records
Java Records shine in various scenarios, particularly where data representation and transfer are the primary concerns. Let’s explore some common use cases where Java Records prove especially beneficial:
1. Data Transfer Objects (DTOs)
Java Records are ideally suited for creating DTOs, which are objects used to transfer data between different components or layers of an application. DTOs typically don’t contain business logic but serve as vessels for data. The immutable nature and automatic method generation of records make them perfect for this role.
Example:
public record UserDTO(long id, String username, String email) {}
This record can be used to transfer user data from a database layer to a service layer, or from a backend service to a frontend client, without the need for additional getters or setters.
2. API Responses
When designing RESTful APIs or any kind of service interface, records can be used to define the structure of response objects. Their built-in toString() method also makes them convenient for logging and debugging.
Example:
public record ApiResponse<T>(int statusCode, String message, T data) {}
This generic record can be used to wrap various types of API responses, providing a consistent structure across different endpoints.
3. Configuration Objects
Records are excellent for representing configuration settings or parameters. Their immutability ensures that configuration doesn’t change unexpectedly during runtime.
Example:
public record DatabaseConfig(String url, String username, int maxConnections) {}
This record encapsulates database configuration parameters, making it easy to pass around and use in different parts of an application.
4. Value Objects in Domain-Driven Design
In Domain-Driven Design (DDD), value objects are immutable objects that describe some characteristic or attribute but carry no concept of identity. Records are perfect for implementing such value objects.
Example:
public record Money(BigDecimal amount, Currency currency) {}
This record represents a monetary value, encapsulating both the amount and the currency.
5. Immutable Data Structures
When working with immutable data structures, such as in functional programming paradigms, records provide a clean and efficient way to represent nodes or elements.
Example:
public record TreeNode<T>(T value, TreeNode<T> left, TreeNode<T> right) {}
This record could be used to represent nodes in an immutable binary tree structure.
6. Event Objects in Event-Driven Systems
In event-driven architectures, events are often represented as immutable data objects. Records are ideal for this purpose, providing a clear and concise way to define event structures.
Example:
public record UserCreatedEvent(long userId, String username, Instant creationTime) {}
This record represents an event that could be published when a new user is created in the system.
7. Test Data
Records can simplify the creation of test data in unit tests. Their concise constructor syntax makes it easy to create instances with different combinations of data.
Example:
public record TestUser(String name, int age, boolean isPremium) {}
// In a test method
TestUser regularUser = new TestUser("Alice", 30, false);
TestUser premiumUser = new TestUser("Bob", 45, true);
8. Tuple-like Structures
While Java doesn’t have built-in tuple types, records can effectively serve this purpose, allowing you to group multiple values together without creating a full-fledged class.
Example:
public record Pair<T, U>(T first, U second) {}
// Usage
Pair<String, Integer> nameAge = new Pair<>("Charlie", 35);
Comparing Java Records to Traditional Classes
To fully appreciate the advantages of Java Records, it’s helpful to compare them directly with traditional Java classes. This comparison will highlight the syntactic differences and the reduction in boilerplate code that records offer. Let’s examine a scenario where we need to create a simple class to represent a point in a 2D space.
Traditional Class Approach:
public class Point {
private final int x;
private final int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Point point = (Point) o;
return x == point.x && y == point.y;
}
@Override
public int hashCode() {
return Objects.hash(x, y);
}
@Override
public String toString() {
return "Point{" +
"x=" + x +
", y=" + y +
'}';
}
}
Record Approach:
public record Point(int x, int y) {}
The difference is striking. The record declaration achieves the same functionality as the traditional class but with significantly less code. Let’s break down the key differences:
- Conciseness: The record declaration is a single line, compared to the 30+ lines required for the traditional class.
- Field Declaration: In the record, fields are declared as part of the record header. In the traditional class, they are explicitly declared as private final fields.
- Constructor: The record automatically generates a canonical constructor. In the traditional class, we had to manually write the constructor.
- Accessor Methods: The record automatically generates accessor methods (getX() and getY()). In the traditional class, these had to be explicitly written.
- equals() and hashCode(): These methods are automatically generated for records based on all components. In the traditional class, we had to manually implement them.
- toString(): The record generates a meaningful toString() method. In the traditional class, we had to override and implement it ourselves.
- Immutability: The record ensures all fields are final by default. In the traditional class, we had to explicitly declare fields as final to achieve immutability.
Advanced Features and Customizations of Java Records
While Java Records provide a lot of functionality out of the box, they also offer flexibility for more advanced use cases. Let’s explore some of the ways you can customize and extend records to fit more complex requirements:
1. Custom Constructors
Although records come with an automatically generated canonical constructor, you can define additional constructors for more complex initialization logic or parameter validation.
Example:
public record Rectangle(double length, double width) {
public Rectangle {
if (length <= 0 || width <= 0) {
throw new IllegalArgumentException("Length and width must be positive");
}
}
public Rectangle(double side) {
this(side, side); // Square
}
}
In this example, we’ve added validation to the canonical constructor and provided an additional constructor to create a square.
2. Custom Accessor Methods
While records automatically generate accessor methods, you can still define your own to provide additional functionality or alternative representations of the data.
Example:
public record Person(String firstName, String lastName) {
public String fullName() {
return firstName + " " + lastName;
}
}
Here, we’ve added a custom method to return the full name.
3. Static Fields and Methods
Records can include static fields and methods, which can be useful for constants or utility functions related to the record.
Example:
public record Circle(double radius) {
public static final double PI = 3.14159;
public static Circle unitCircle() {
return new Circle(1);
}
public double area() {
return PI * radius * radius;
}
}
This record includes a static field for PI and a static factory method for creating a unit circle.
4. Implementing Interfaces
Records can implement interfaces, allowing you to define additional behavior or conform to existing APIs.
Example:
public record Employee(String name, int id) implements Comparable<Employee> {
@Override
public int compareTo(Employee other) {
return Integer.compare(this.id, other.id);
}
}
This record implements the Comparable interface, allowing Employee instances to be sorted based on their ID.
5. Nested Records
You can define records within other classes or records, which can be useful for creating hierarchical data structures.
Example:
public record Department(String name, Manager manager) {
public record Manager(String name, int yearsOfExperience) {}
}
Here, we’ve defined a nested Manager record within the Department record.
6. Generic Records
Records can be generic, allowing for flexible and reusable data structures.
Example:
public record Pair<T, U>(T first, U second) {
public <V> Pair<V, U> mapFirst(Function<T, V> mapper) {
return new Pair<>(mapper.apply(first), second);
}
}
This generic record can hold two values of potentially different types and includes a method to transform the first value.
7. Annotations
Records support annotations, which can be useful for frameworks that rely on reflection or for documentation purposes.
Example:
public record UserDTO(
@NotNull String username,
@Email String email,
@Min(18) int age
) {}
In this example, we’ve used validation annotations that could be processed by a framework like Hibernate Validator.
Best Practices and Design Considerations
When working with Java Records, it’s important to follow best practices and consider certain design aspects to make the most of this feature. Here are some key points to keep in mind:
1. Use Records for Immutable Data
Records are designed to be immutable, which makes them ideal for representing unchangeable data. Avoid using records for mutable data structures, as this goes against their intended purpose and can lead to unexpected behavior.
2. Keep Records Focused
Records work best when they represent a clear, cohesive set of data. Avoid creating “giant” records with too many fields. If a record is becoming too large or complex, it might be a sign that you need to break it down into smaller, more focused records.
3. Consider Validation in Constructors
While records provide a compact way to define data structures, don’t neglect input validation. Use compact constructors to add validation logic without repeating the parameter list.
Example:
public record Person(String name, int age) {
public Person {
if (name == null || name.isEmpty()) {
throw new IllegalArgumentException("Name cannot be null or empty");
}
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
}
}
4. Leverage Pattern Matching
Records work well with pattern matching features in Java. Use this synergy to write more expressive and concise code when working with records.
Example:
if (shape instanceof Circle(var radius)) {
System.out.println("Area of circle: " + Math.PI * radius * radius);
} else if (shape instanceof Rectangle(var length, var width)) {
System.out.println("Area of rectangle: " + length * width);
}
5. Be Mindful of Inheritance Limitations
Remember that records cannot extend other classes (except for java.lang.Record) and cannot be extended. If you need inheritance, traditional classes might be more appropriate.
6. Use Records in APIs Judiciously
While records are great for DTOs and value objects, be cautious about exposing them directly in public APIs. If you anticipate that the structure might change in the future, consider using traditional classes or interfaces to maintain more flexibility.
7. Combine with Sealed Classes for Powerful Data Modeling
Records can be effectively combined with sealed classes to create powerful and expressive data models. This combination allows you to define a closed set of related records, which can be particularly useful in domain modeling.
Example:
public sealed interface Shape
permits Circle, Rectangle, Triangle {}
public record Circle(double radius) implements Shape {}
public record Rectangle(double width, double height) implements Shape {}
public record Triangle(double base, double height) implements Shape {}
This pattern allows you to create a closed hierarchy of shapes, which can be useful for exhaustive pattern matching and ensuring type safety.
Performance Considerations
While records primarily aim to reduce boilerplate and improve code clarity, they can also have performance implications. It’s important to understand these aspects when deciding to use records in performance-critical applications.
1. Memory Usage
Records can potentially lead to reduced memory usage compared to traditional classes, especially for small objects. This is because records don’t have the overhead of separate fields for storing data and can utilize more efficient memory layouts.
2. Object Creation
The creation of record instances can be more efficient than creating instances of equivalent traditional classes. This is because records have a more straightforward initialization process and don’t require separate method calls for setting fields.
3. Method Invocation
Accessor methods in records (automatically generated getter methods) can potentially be inlined by the JVM more easily than custom getter methods in traditional classes. This can lead to slightly faster access to record components in some scenarios.
4. Serialization
Records implement Serializable by default, which can be convenient but might have performance implications if not needed. If serialization is not required, you may want to explicitly make your record non-serializable to avoid any potential overhead.
Integrating Records with Existing Codebases
Introducing records into an existing codebase requires careful consideration. Here are some strategies for effective integration:
1. Gradual Adoption
Start by identifying classes that are primarily used as data carriers, such as DTOs or value objects. These are prime candidates for conversion to records.
2. Refactoring Existing Classes
When refactoring existing classes to records, be mindful of any code that relies on the internal structure of these classes. While the public API (getter methods) will remain the same, any code that directly accessed private fields will need to be updated.
3. Compatibility Considerations
If you’re working on a library or API that other code depends on, be cautious about changing classes to records, as this could break compatibility. Consider introducing records alongside existing classes initially, and gradually deprecate the old classes if possible.
4. Testing Strategy
When converting classes to records, ensure that you have a comprehensive test suite in place. This will help catch any unexpected behavior changes resulting from the conversion.
Limitations and Considerations
While records offer many benefits, it’s important to be aware of their limitations:
1. No Inheritance
Records cannot extend other classes (except java.lang.Record) and cannot be extended. If you need inheritance, traditional classes are still necessary.
2. All-or-Nothing Immutability
All fields in a record are final. If you need some mutable fields, you’ll need to use a traditional class or consider a different design approach.
3. Limited Customization of Generated Methods
While you can provide custom implementations of methods like equals() and hashCode(), doing so goes against the spirit of records and can lead to confusion.
4. Not Suitable for Entities
Records are not ideal for representing entities in JPA or other ORM frameworks, as these typically require mutable objects with no-arg constructors.
Future Directions
As Java continues to evolve, we can expect to see further enhancements and integrations related to records. Some potential areas for future development include:
- Better integration with serialization frameworks
- Enhanced pattern matching capabilities
- More advanced type inference in conjunction with records
- Potential for record-like structures with some mutable fields
Conclusion
Java Records represent a significant step forward in Java’s evolution, offering a powerful tool for creating concise, immutable data holders. By dramatically reducing boilerplate code, improving readability, and promoting better design practices, records have quickly become an essential feature for many Java developers.
As we’ve explored in this deep dive, records excel in scenarios ranging from simple data transfer objects to more complex domain modeling when combined with other Java features like sealed classes. Their benefits in terms of code clarity, reduced error potential, and potential performance improvements make them an attractive option for many use cases.
However, like any feature, records come with their own set of limitations and considerations. Understanding when to use records—and when to stick with traditional classes—is key to leveraging this feature effectively in your Java projects.
As you incorporate records into your codebase, remember to consider the design implications, performance aspects, and integration strategies we’ve discussed. By doing so, you’ll be well-equipped to make the most of this powerful feature and write cleaner, more maintainable Java code.
Disclaimer: This blog post is based on the current implementation of Java Records as of Java 16. Future Java versions may introduce changes or enhancements to the Records feature. While every effort has been made to ensure the accuracy of the information presented, readers are encouraged to consult the official Java documentation for the most up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.