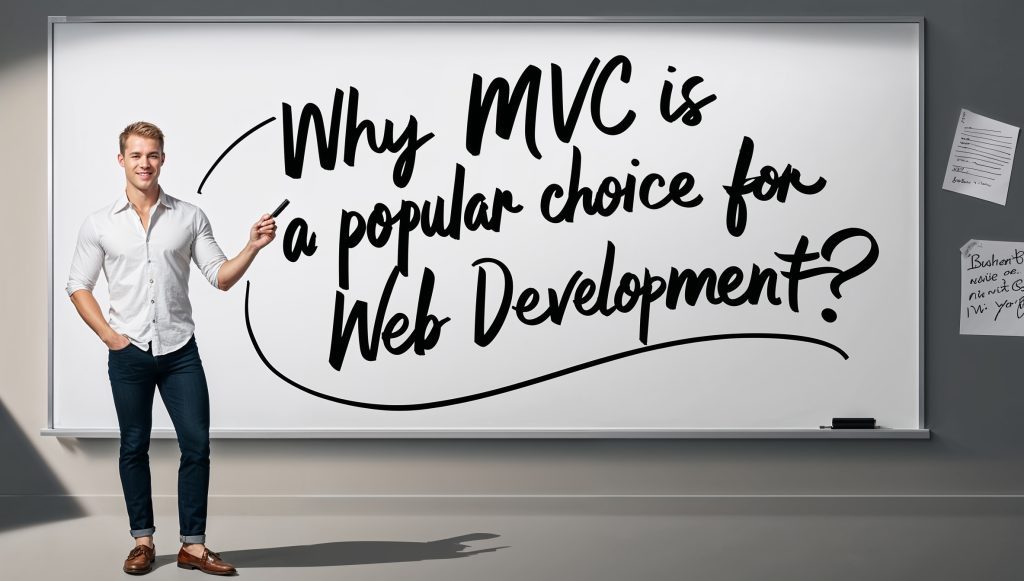
Why MVC is a Popular Choice for Web Development?
Model-View-Controller (MVC) has emerged as one of the most influential and widely adopted architectural patterns in modern web development. This battle-tested design pattern has stood the test of time since its inception in the 1970s, continuing to prove its worth across numerous frameworks and platforms. The pattern’s enduring popularity stems from its ability to effectively separate concerns, promote code organization, and enhance maintainability in web applications of all sizes. In this comprehensive guide, we’ll explore why MVC remains a go-to choice for developers and architects, examining its core principles, benefits, and practical implementations across different technologies.
Understanding the MVC Architecture
The MVC pattern divides an application into three distinct but interconnected components, each serving a specific purpose in the overall architecture. This separation of concerns is fundamental to understanding why MVC has become such a prevalent choice in web development. The Model component manages data and business logic, the View handles presentation and user interface elements, and the Controller orchestrates the interaction between the Model and View while processing user input. This triumvirate of components works in harmony to create a well-structured and maintainable application architecture.
The Three Pillars of MVC
Model:
The Model represents the application’s data structure and business logic. It operates independently of the user interface, maintaining data integrity and enforcing business rules. The Model notifies observers (typically Views) about any changes to its state, enabling automatic UI updates. Consider this example of a simple User Model in PHP:
class UserModel {
private $id;
private $username;
private $email;
private $password;
public function __construct($username, $email, $password) {
$this->username = $username;
$this->email = $email;
$this->password = password_hash($password, PASSWORD_DEFAULT);
}
public function save() {
// Database interaction logic
$sql = "INSERT INTO users (username, email, password)
VALUES (:username, :email, :password)";
// Execute query and return result
}
public function validate() {
// Validation logic
return empty($this->username) || empty($this->email) ? false : true;
}
}
View:
The View is responsible for presenting data to users in an appropriate format. It receives data from the Model through the Controller and renders it into a user interface. Here’s an example of a simple user profile view in HTML/PHP:
<!-- user_profile.php -->
<!DOCTYPE html>
<html>
<head>
<title>User Profile</title>
</head>
<body>
<div class="profile-container">
<h1><?php echo htmlspecialchars($user->username); ?></h1>
<div class="profile-details">
<p>Email: <?php echo htmlspecialchars($user->email); ?></p>
<p>Member since: <?php echo date('F j, Y', strtotime($user->created_at)); ?></p>
</div>
</div>
</body>
</html>
Controller:
The Controller acts as an intermediary between the Model and View, handling user input and updating both components accordingly. Here’s an example of a basic UserController:
class UserController {
private $userModel;
public function __construct() {
$this->userModel = new UserModel();
}
public function register() {
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$username = $_POST['username'];
$email = $_POST['email'];
$password = $_POST['password'];
$user = new UserModel($username, $email, $password);
if ($user->validate()) {
$user->save();
$this->redirect('/login');
} else {
$this->render('register', ['error' => 'Invalid input']);
}
}
$this->render('register');
}
private function render($view, $data = []) {
// Render view logic
include "views/$view.php";
}
}
Key Benefits of MVC Architecture
1. Enhanced Maintainability
The MVC pattern significantly improves code maintainability through its clear separation of concerns. Each component has a distinct responsibility, making it easier to identify and fix issues without affecting other parts of the application. When bugs occur, developers can quickly isolate the problem to a specific component, reducing debugging time and complexity. The modular nature of MVC also makes it easier to update or replace individual components without impacting the entire application.
2. Improved Testability
MVC’s component separation naturally facilitates unit testing. Each component can be tested in isolation, allowing for more thorough and focused testing strategies. Here’s an example of unit testing a User Model using PHPUnit:
class UserModelTest extends PHPUnit\Framework\TestCase {
public function testUserValidation() {
$user = new UserModel('testuser', 'test@example.com', 'password123');
$this->assertTrue($user->validate());
$invalidUser = new UserModel('', '', 'password123');
$this->assertFalse($invalidUser->validate());
}
public function testPasswordHashing() {
$password = 'password123';
$user = new UserModel('testuser', 'test@example.com', $password);
$this->assertTrue(password_verify($password, $user->getPassword()));
}
}
3. Better Code Organization
The MVC pattern provides a clear and logical structure for organizing code. This organization becomes particularly valuable as applications grow in size and complexity. Here’s a typical MVC project structure:
project/
├── models/
│ ├── UserModel.php
│ ├── ProductModel.php
│ └── OrderModel.php
├── views/
│ ├── users/
│ │ ├── profile.php
│ │ └── register.php
│ └── layouts/
│ └── main.php
├── controllers/
│ ├── UserController.php
│ ├── ProductController.php
│ └── OrderController.php
└── config/
└── database.php
Practical Implementation Across Different Frameworks
Modern web frameworks have embraced and enhanced the MVC pattern. Here’s how different frameworks implement MVC:
Laravel (PHP)
// Routes/web.php
Route::get('/users/{id}', 'UserController@show');
// UserController.php
class UserController extends Controller {
public function show($id) {
$user = User::find($id);
return view('users.show', compact('user'));
}
}
ASP.NET Core (C#)
// UserController.cs
public class UserController : Controller {
private readonly IUserService _userService;
public UserController(IUserService userService) {
_userService = userService;
}
public IActionResult Index() {
var users = _userService.GetAllUsers();
return View(users);
}
}
MVC Best Practices and Patterns
To maximize the benefits of MVC, consider these best practices:
Best Practice | Description | Example |
---|---|---|
Thin Controllers | Keep controllers focused on routing and coordination | Controllers should delegate business logic to services or models |
Fat Models | Place business logic in models | Models should contain data validation and business rules |
View Models | Use dedicated objects for view data | Create DTOs for data transfer between layers |
Service Layer | Implement services for complex operations | Use services to handle operations involving multiple models |
Comparison with Other Architectural Patterns
Pattern | Pros | Cons | Best Used For |
---|---|---|---|
MVC | Clear separation, well-established | Can be complex for small apps | Medium to large web applications |
MVP | Better testability than MVC | More complex than MVC | Applications with complex view logic |
MVVM | Great for reactive UIs | Learning curve | Modern single-page applications |
Modern MVC Implementation Example
Here’s a complete example of a modern MVC implementation using TypeScript and Express:
// User Model
interface IUser {
id: number;
username: string;
email: string;
}
class User implements IUser {
constructor(
public id: number,
public username: string,
public email: string
) {}
static validate(user: IUser): boolean {
return user.username.length > 0 &&
user.email.includes('@');
}
}
// User Controller
class UserController {
constructor(private userService: UserService) {}
async getUser(req: Request, res: Response): Promise<void> {
try {
const user = await this.userService.findById(req.params.id);
res.render('user/profile', { user });
} catch (error) {
res.status(404).render('error', {
message: 'User not found'
});
}
}
}
// User Service
class UserService {
async findById(id: string): Promise<User> {
// Database interaction logic
const userData = await db.users.findOne({ id });
return new User(
userData.id,
userData.username,
userData.email
);
}
}
Conclusion
The MVC architectural pattern continues to be a cornerstone of web development for good reasons. Its clear separation of concerns, enhanced maintainability, and improved testability make it an excellent choice for modern web applications. While newer patterns and variations have emerged, MVC’s fundamental principles remain relevant and valuable. By understanding and properly implementing MVC, developers can create more organized, maintainable, and scalable applications that stand the test of time.
Disclaimer: This article represents our current understanding of MVC architecture and its implementations. While we strive for accuracy, web development practices and frameworks evolve rapidly. If you notice any inaccuracies or have suggestions for improvements, please report them to our editorial team at support@felixrante.com. Last updated: October 2024.