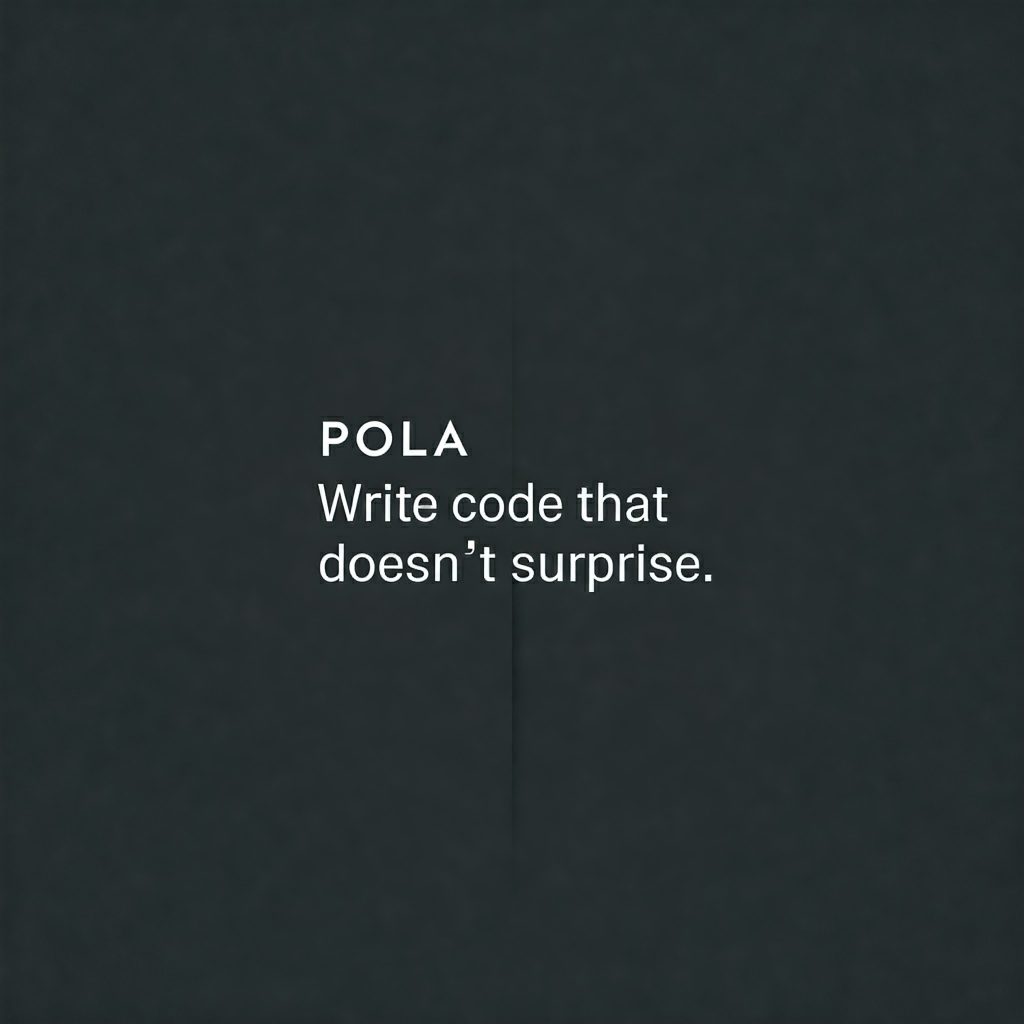
POLA: Write Code That Doesn’t Surprise
The Principle of Least Astonishment (POLA), also known as the Principle of Least Surprise, is a fundamental concept in software design and development that emphasizes creating intuitive and predictable code. This principle states that the behavior of a system should match users’ expectations and experiences, minimizing confusion and surprises. When developers follow POLA, they create code that behaves in ways that other developers can easily understand and anticipate, leading to more maintainable and reliable software systems. This principle becomes increasingly important as software systems grow in complexity and team sizes expand, making it essential for modern software development practices.
Understanding POLA
The Principle of Least Astonishment is rooted in cognitive psychology and user interface design principles. It suggests that when two elements of an interface conflict with each other, the behavior should be as little surprising as possible. In software development, this translates to writing code that follows established conventions, maintains consistency, and produces expected outcomes. When developers encounter code that adheres to POLA, they can make accurate assumptions about its behavior based on their existing knowledge and experience, reducing the cognitive load required to understand and maintain the code.
Key Aspects of POLA
- Consistency in naming conventions
- Predictable function behavior
- Clear and logical organization
- Standard design patterns
- Intuitive interfaces
Common Violations of POLA
Let’s explore some common ways developers inadvertently violate POLA, along with their solutions. Understanding these violations helps in writing better code that adheres to the principle.
1. Inconsistent Method Names
Python Example of Poor Naming:
class UserManager:
def get_user(self, user_id):
return self.fetch_from_database(user_id)
def fetch_customer(self, customer_id):
return self.fetch_from_database(customer_id)
def retrieve_admin(self, admin_id):
return self.fetch_from_database(admin_id)
Improved Version:
class UserManager:
def get_user(self, user_id):
return self.fetch_from_database(user_id)
def get_customer(self, customer_id):
return self.fetch_from_database(customer_id)
def get_admin(self, admin_id):
return self.fetch_from_database(admin_id)
2. Unexpected Side Effects
Java Example with Side Effects:
public class StringProcessor {
private List<String> processedStrings = new ArrayList<>();
public String processString(String input) {
String processed = input.toUpperCase();
processedStrings.add(processed); // Hidden side effect
return processed;
}
}
Improved Version:
public class StringProcessor {
private List<String> processedStrings = new ArrayList<>();
public String processString(String input) {
return input.toUpperCase();
}
public void addToProcessedStrings(String input) {
processedStrings.add(input);
}
}
Best Practices for Writing POLA-Compliant Code
Writing code that follows POLA requires careful consideration and adherence to established best practices. Here are several key principles to follow when writing POLA-compliant code.
1. Consistent Naming Conventions
Python Example:
# Good - Consistent naming pattern
def get_user_by_id(user_id):
pass
def get_user_by_email(email):
pass
def get_user_by_username(username):
pass
# Bad - Inconsistent naming pattern
def fetch_user_id(user_id):
pass
def getUserEmail(email):
pass
def find_username(username):
pass
2. Clear Function Signatures
Java Example:
// Good - Clear and explicit parameters
public class OrderProcessor {
public Order processOrder(
Customer customer,
List<OrderItem> items,
PaymentMethod paymentMethod
) {
// Processing logic
}
}
// Bad - Ambiguous parameters
public class OrderProcessor {
public Order process(
Object customerData,
Object[] items,
String type
) {
// Processing logic
}
}
Implementing POLA in Different Programming Paradigms
POLA implementation varies across different programming paradigms. Let’s explore how to apply POLA in both object-oriented and functional programming contexts.
Object-Oriented Programming Example
# Good POLA implementation in OOP
class BankAccount:
def __init__(self, initial_balance=0):
self._balance = initial_balance
self._transactions = []
def deposit(self, amount):
if amount <= 0:
raise ValueError("Deposit amount must be positive")
self._balance += amount
self._transactions.append(("deposit", amount))
return self._balance
def withdraw(self, amount):
if amount <= 0:
raise ValueError("Withdrawal amount must be positive")
if amount > self._balance:
raise ValueError("Insufficient funds")
self._balance -= amount
self._transactions.append(("withdrawal", amount))
return self._balance
@property
def balance(self):
return self._balance
def get_transaction_history(self):
return self._transactions.copy()
Functional Programming Example
from typing import List, Tuple, Dict
from dataclasses import dataclass
from datetime import datetime
@dataclass(frozen=True)
class Transaction:
type: str
amount: float
timestamp: datetime
def create_account(initial_balance: float = 0) -> Dict:
return {
'balance': initial_balance,
'transactions': []
}
def deposit(
account: Dict,
amount: float
) -> Tuple[Dict, float]:
if amount <= 0:
raise ValueError("Deposit amount must be positive")
new_balance = account['balance'] + amount
new_transactions = account['transactions'] + [
Transaction("deposit", amount, datetime.now())
]
return {
'balance': new_balance,
'transactions': new_transactions
}, new_balance
def withdraw(
account: Dict,
amount: float
) -> Tuple[Dict, float]:
if amount <= 0:
raise ValueError("Withdrawal amount must be positive")
if amount > account['balance']:
raise ValueError("Insufficient funds")
new_balance = account['balance'] - amount
new_transactions = account['transactions'] + [
Transaction("withdrawal", amount, datetime.now())
]
return {
'balance': new_balance,
'transactions': new_transactions
}, new_balance
Testing for POLA Compliance
Testing is crucial for ensuring code adheres to POLA. Here’s an example of how to write tests that verify POLA compliance:
import unittest
class BankAccountTests(unittest.TestCase):
def setUp(self):
self.account = BankAccount(1000)
def test_deposit_positive_amount(self):
initial_balance = self.account.balance
deposit_amount = 500
new_balance = self.account.deposit(deposit_amount)
self.assertEqual(
new_balance,
initial_balance + deposit_amount,
"Deposit should increase balance by exact amount"
)
def test_deposit_negative_amount(self):
with self.assertRaises(ValueError):
self.account.deposit(-100)
def test_withdraw_positive_amount(self):
initial_balance = self.account.balance
withdrawal_amount = 500
new_balance = self.account.withdraw(withdrawal_amount)
self.assertEqual(
new_balance,
initial_balance - withdrawal_amount,
"Withdrawal should decrease balance by exact amount"
)
def test_withdraw_negative_amount(self):
with self.assertRaises(ValueError):
self.account.withdraw(-100)
def test_withdraw_insufficient_funds(self):
with self.assertRaises(ValueError):
self.account.withdraw(2000)
Common Pitfalls and How to Avoid Them
Here’s a comparison table of common POLA violations and their solutions:
Pitfall | Example | Solution |
---|---|---|
Inconsistent Return Types | def get_user(id): return user if user else None | Always return the same type (e.g., Optional[User]) |
Silent Failures | try: process() except: pass | Always handle errors explicitly |
Mixed Responsibilities | def save_and_email_user() | Split into separate functions |
Implicit State Changes | def process(data): global_var = data | Make state changes explicit |
Ambiguous Parameters | def update(data, flag) | Use descriptive parameter names |
Tools and Techniques for Enforcing POLA
Several tools and techniques can help enforce POLA in your codebase:
1. Static Code Analysis
# Using pylint for Python
# .pylintrc configuration
[MESSAGES CONTROL]
disable=C0111
enable=C0103,C0326
# Using checkstyle for Java
<!-- checkstyle.xml configuration -->
<module name="Checker">
<module name="TreeWalker">
<module name="MethodName"/>
<module name="ParameterName"/>
</module>
</module>
2. Code Review Checklist
- [ ] Method names follow consistent patterns
- [ ] Function parameters are explicit and well-typed
- [ ] Return types are consistent
- [ ] Error handling is explicit
- [ ] No hidden side effects
- [ ] Documentation matches implementation
- [ ] Tests verify expected behavior
Measuring POLA Compliance
While POLA compliance can be subjective, here are some metrics to consider:
- Code Review Feedback Rate
- Bug Report Analysis
- Developer Onboarding Time
- Maintenance Time
- Test Coverage
Future Considerations and Emerging Practices
As software development evolves, new considerations for POLA emerge:
- AI-assisted coding tools
- Modern architecture patterns
- Cloud-native applications
- Microservices communication
- Cross-platform development
Conclusion
The Principle of Least Astonishment is a fundamental guideline for writing maintainable, intuitive code. By following POLA, developers can create software that is easier to understand, maintain, and extend. The examples and practices outlined in this blog post provide a solid foundation for implementing POLA in your projects. Remember that writing code that doesn’t surprise is an ongoing process that requires constant attention to detail and a deep understanding of your users’ expectations.
Disclaimer: The code examples and best practices presented in this blog post are based on generally accepted software development principles and may need to be adapted for specific use cases or requirements. While we strive for accuracy, technology evolves rapidly, and some practices may become outdated. Please report any inaccuracies or outdated information to our editorial team for prompt correction.