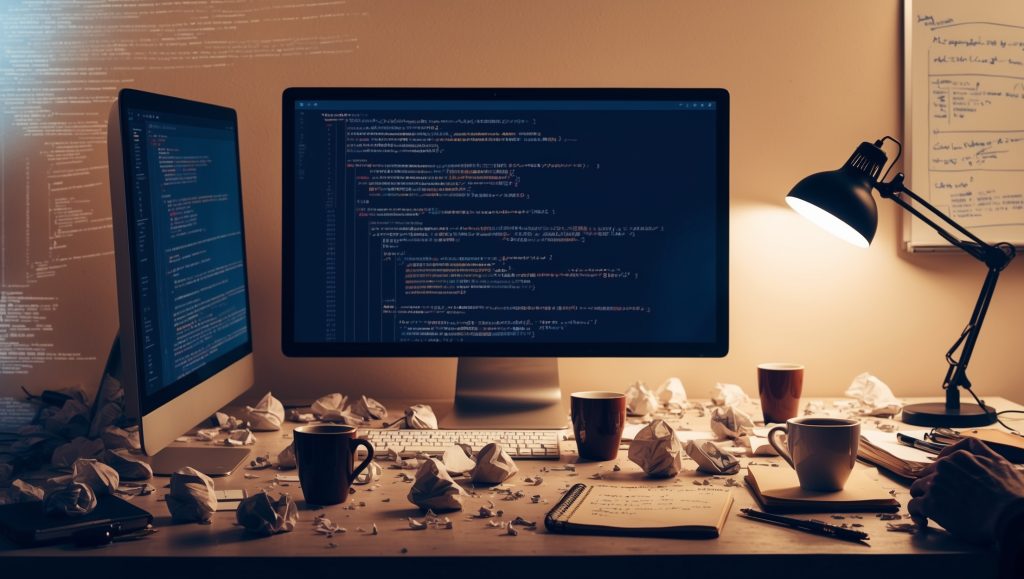
SoC: The Modular Approach to Building Software
Software development has evolved significantly over the years, with various principles and patterns emerging to help developers create more maintainable, scalable, and robust applications. Among these principles, Separation of Concerns (SoC) stands as a fundamental concept that has revolutionized how we approach software architecture and design. This principle, first coined by Edsger W. Dijkstra in 1974, suggests that software should be separated into distinct sections, where each section addresses a specific concern or functionality. In today’s complex software landscape, understanding and implementing SoC has become more crucial than ever, as it helps manage complexity, improve code maintainability, and foster better collaboration among development teams. This comprehensive guide will explore the concept of SoC, its practical applications, and how it contributes to building better software systems.
Understanding Separation of Concerns
Separation of Concerns is a design principle that advocates dividing a computer program into distinct sections, where each section handles a specific aspect of the functionality. This separation allows developers to manage complexity by breaking down complex systems into smaller, more manageable parts. The principle suggests that different aspects of a program should be as independent as possible, reducing coupling between components and making the system easier to maintain and modify. When properly implemented, SoC enables developers to work on individual components without affecting other parts of the system, leading to more reliable and maintainable code.
Key Benefits of Separation of Concerns
- Improved Maintainability: When concerns are properly separated, developers can modify one aspect of the system without affecting others, making maintenance tasks more straightforward and less risky.
- Enhanced Reusability: Isolated components can be reused across different parts of the application or even in different projects, promoting code efficiency and consistency.
- Better Testing: Separated concerns are easier to test in isolation, enabling more effective unit testing and debugging.
- Simplified Development: Teams can work on different concerns simultaneously without interfering with each other’s work, improving development efficiency.
- Increased Flexibility: Changes to one concern don’t necessarily affect others, making the system more adaptable to new requirements.
Common Types of Concerns in Software Development
In software development, concerns can be categorized into several types, each addressing different aspects of the application. Understanding these categories helps developers implement SoC more effectively.
Business Logic Concerns
The business logic layer contains the core functionality and rules of the application. This includes:
- Data validation rules
- Calculations and computations
- Business process workflows
- Domain-specific operations
Data Access Concerns
Data access concerns involve how the application interacts with data storage systems:
- Database operations
- Data persistence
- Caching mechanisms
- Data retrieval and storage logic
Presentation Concerns
Presentation concerns relate to how information is displayed to users:
- User interface components
- Display formatting
- User input handling
- View-specific logic
Cross-cutting Concerns
Cross-cutting concerns affect multiple parts of the application:
- Logging
- Security
- Error handling
- Performance monitoring
Implementing Separation of Concerns in Practice
Let’s explore practical examples of implementing SoC using both Python and Java. We’ll create a simple user management system that demonstrates the separation of different concerns.
Python Implementation Example
# Data Access Layer (DAL)
class UserRepository:
def __init__(self):
self.db_connection = None # Database connection would be initialized here
def get_user(self, user_id):
# Database interaction logic
return {"id": user_id, "name": "John Doe", "email": "john@example.com"}
def save_user(self, user_data):
# Logic to save user to database
pass
# Business Logic Layer
class UserService:
def __init__(self, user_repository):
self.user_repository = user_repository
def get_user_details(self, user_id):
user = self.user_repository.get_user(user_id)
# Apply business rules and transformations
return user
def create_user(self, user_data):
# Validate user data
if not self._validate_user_data(user_data):
raise ValueError("Invalid user data")
# Process and save user
self.user_repository.save_user(user_data)
def _validate_user_data(self, user_data):
# Business validation rules
return True
# Presentation Layer
class UserController:
def __init__(self, user_service):
self.user_service = user_service
def display_user(self, user_id):
try:
user = self.user_service.get_user_details(user_id)
return self._format_user_display(user)
except Exception as e:
return f"Error: {str(e)}"
def _format_user_display(self, user):
return f"User: {user['name']} ({user['email']})"
# Cross-cutting Concerns
class Logger:
@staticmethod
def log(message):
print(f"[LOG] {message}")
# Usage Example
if __name__ == "__main__":
# Initialize components
repository = UserRepository()
service = UserService(repository)
controller = UserController(service)
# Use the system
result = controller.display_user(1)
Logger.log(f"Displayed user information: {result}")
Java Implementation Example
// Data Access Layer
class UserRepository {
private Connection dbConnection; // Database connection
public User getUser(Long userId) {
// Database interaction logic
return new User(userId, "John Doe", "john@example.com");
}
public void saveUser(User user) {
// Logic to save user to database
}
}
// Business Logic Layer
class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserDetails(Long userId) {
User user = userRepository.getUser(userId);
// Apply business rules and transformations
return user;
}
public void createUser(User user) {
if (!validateUserData(user)) {
throw new IllegalArgumentException("Invalid user data");
}
userRepository.saveUser(user);
}
private boolean validateUserData(User user) {
// Business validation rules
return true;
}
}
// Presentation Layer
class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
public String displayUser(Long userId) {
try {
User user = userService.getUserDetails(userId);
return formatUserDisplay(user);
} catch (Exception e) {
return "Error: " + e.getMessage();
}
}
private String formatUserDisplay(User user) {
return String.format("User: %s (%s)", user.getName(), user.getEmail());
}
}
// Cross-cutting Concerns
class Logger {
public static void log(String message) {
System.out.println("[LOG] " + message);
}
}
// Domain Model
class User {
private Long id;
private String name;
private String email;
// Constructor, getters, and setters
}
// Main Application
public class Application {
public static void main(String[] args) {
UserRepository repository = new UserRepository();
UserService service = new UserService(repository);
UserController controller = new UserController(service);
String result = controller.displayUser(1L);
Logger.log("Displayed user information: " + result);
}
}
Best Practices for Implementing Separation of Concerns
Design Patterns that Support SoC
The following table presents common design patterns that help implement SoC effectively:
Pattern | Purpose | Key Benefits |
---|---|---|
MVC | Separates application into Model, View, and Controller | Clear separation of data, presentation, and control logic |
Repository | Abstracts data access logic | Isolates database operations from business logic |
Service Layer | Encapsulates business logic | Creates a boundary between presentation and data access |
Factory | Handles object creation | Separates object creation from business logic |
Observer | Manages event handling | Decouples event producers from consumers |
Guidelines for Maintaining Clean Separation
When implementing SoC, follow these essential guidelines:
- Keep components focused on a single responsibility
- Minimize dependencies between components
- Use interfaces to define clear boundaries
- Implement proper error handling at each layer
- Document component responsibilities and interactions
Common Pitfalls and How to Avoid Them
When implementing Separation of Concerns, developers often encounter several common challenges. Understanding these pitfalls and knowing how to avoid them is crucial for successful implementation.
Over-separation
While separation is important, breaking down components too finely can lead to:
- Excessive complexity
- Increased development time
- Communication overhead between components
- Difficulty in maintaining the overall system architecture
Insufficient Separation
On the other hand, insufficient separation can result in:
- Tightly coupled components
- Difficult-to-maintain code
- Reduced reusability
- Testing challenges
Testing Strategies for Separated Concerns
Proper testing is essential for maintaining the integrity of separated concerns. Here’s a comprehensive approach to testing different layers:
Unit Testing
# Python Example: Unit Testing Business Logic
import unittest
class TestUserService(unittest.TestCase):
def setUp(self):
self.repository = MockUserRepository()
self.service = UserService(self.repository)
def test_get_user_details(self):
user = self.service.get_user_details(1)
self.assertEqual(user['name'], "John Doe")
def test_invalid_user_data(self):
with self.assertRaises(ValueError):
self.service.create_user({"invalid": "data"})
Integration Testing
// Java Example: Integration Testing
@SpringBootTest
public class UserServiceIntegrationTest {
@Autowired
private UserService userService;
@Autowired
private UserRepository userRepository;
@Test
public void testUserCreation() {
User user = new User("John Doe", "john@example.com");
userService.createUser(user);
User savedUser = userRepository.getUser(user.getId());
assertEquals(user.getName(), savedUser.getName());
}
}
Future Trends and Evolution of SoC
The principle of Separation of Concerns continues to evolve with new technologies and development paradigms. Current trends include:
- Microservices architecture
- Serverless computing
- Event-driven architecture
- Domain-driven design
- Containerization and orchestration
Conclusion
Separation of Concerns remains a fundamental principle in software development, providing a structured approach to managing complexity and building maintainable systems. By understanding and properly implementing SoC, developers can create more robust, scalable, and maintainable applications. The examples and best practices discussed in this article serve as a foundation for implementing SoC in real-world projects. As software systems continue to grow in complexity, the importance of proper separation of concerns will only increase, making it an essential skill for modern software developers.
Disclaimer: The code examples and best practices presented in this article are for educational purposes and may need to be adapted for specific use cases. While every effort has been made to ensure accuracy, technology evolves rapidly, and some information may become outdated. Please report any inaccuracies to our editorial team for prompt correction.