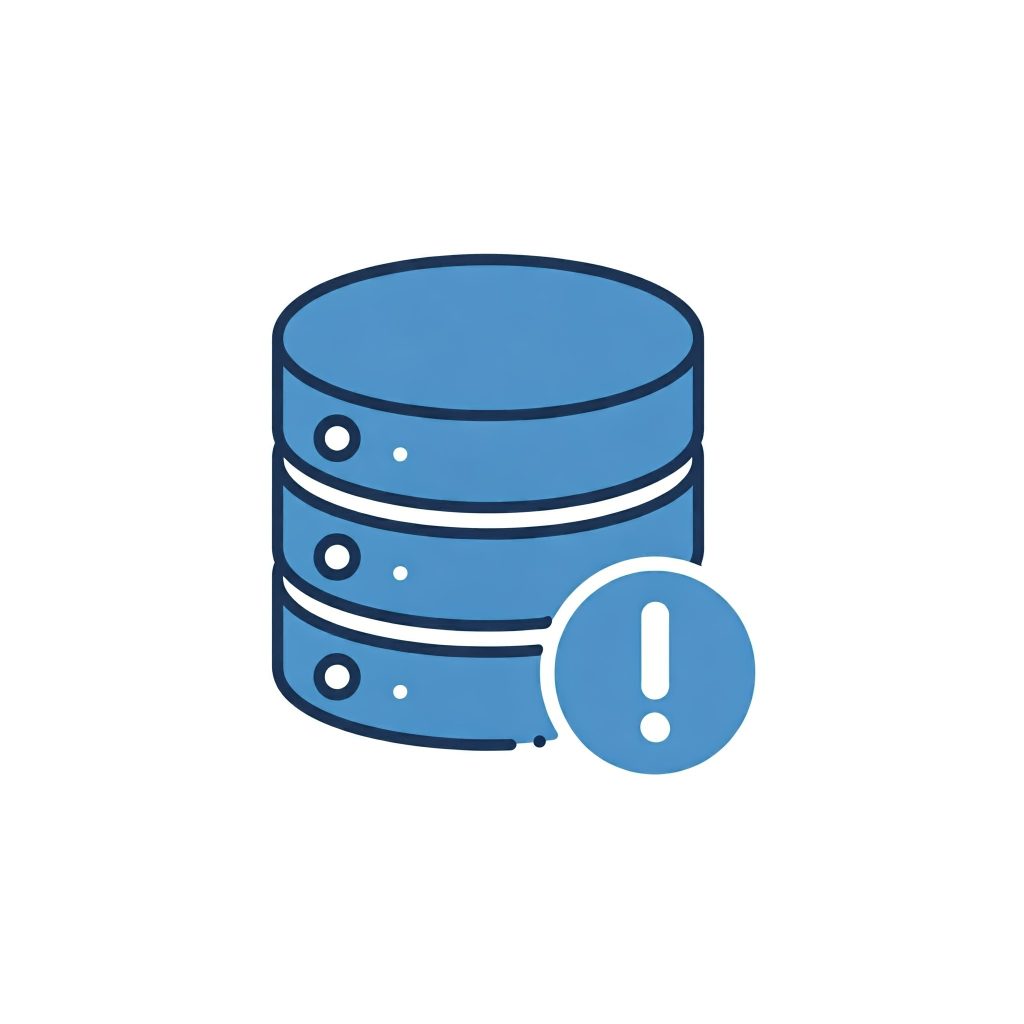
Common SQL Errors and How to Fix Them
Structured Query Language (SQL) is an essential tool for managing and manipulating relational databases. However, even experienced developers can encounter errors when working with SQL. This comprehensive guide will explore common SQL errors and provide practical solutions to help you troubleshoot and resolve these issues efficiently. By understanding these errors and their remedies, you can improve your SQL skills and enhance your database management capabilities.
Syntax Errors
Syntax errors are among the most common issues encountered in SQL. These errors occur when the SQL statement does not conform to the correct syntax rules of the language. Fortunately, most database management systems provide detailed error messages that can help identify the specific syntax problem.
Missing Semicolons
One of the most frequent syntax errors is forgetting to include a semicolon at the end of an SQL statement. In many SQL implementations, semicolons are required to terminate each statement. When this punctuation is missing, the database engine may interpret multiple statements as a single, invalid command.
Example of incorrect syntax:
SELECT * FROM employees
INSERT INTO departments (dept_name) VALUES ('Marketing')
Corrected syntax:
SELECT * FROM employees;
INSERT INTO departments (dept_name) VALUES ('Marketing');
To avoid this error, always remember to end your SQL statements with semicolons. Some developers find it helpful to add the semicolon immediately after writing each statement, rather than waiting until the end of the script.
Misspelled Keywords
Another common syntax error involves misspelling SQL keywords. SQL is case-insensitive for keywords, but it is crucial to spell them correctly. Misspelled keywords will result in syntax errors because the database engine cannot recognize the command.
Example of incorrect syntax:
SELCT * FORM employees WHERE department = 'Sales';
Corrected syntax:
SELECT * FROM employees WHERE department = 'Sales';
To prevent this error, double-check your spelling, especially for frequently used keywords like SELECT, FROM, WHERE, and JOIN. Many modern integrated development environments (IDEs) and text editors offer SQL syntax highlighting, which can help identify misspelled keywords visually.
Incorrect Use of Single and Double Quotes
SQL uses single quotes for string literals and double quotes for identifiers (such as table or column names) in some database systems. Mixing these up or using them inconsistently can lead to syntax errors.
Example of incorrect syntax:
SELECT * FROM "employees" WHERE 'department' = "Sales";
Corrected syntax:
SELECT * FROM employees WHERE department = 'Sales';
To avoid this error, consistently use single quotes for string values and only use double quotes for identifiers when necessary (e.g., when the identifier contains spaces or special characters). Some database systems, like MySQL, allow backticks (`) for identifiers as well.
Logical Errors
Logical errors are more subtle than syntax errors because they don’t prevent the SQL statement from executing. Instead, they produce incorrect or unexpected results. These errors can be particularly challenging to identify and resolve because they require a thorough understanding of both the data and the intended query logic.
Incorrect JOIN Conditions
One common logical error occurs when specifying incorrect join conditions, leading to unexpected result sets. This often happens when joining tables with multiple foreign key relationships or when dealing with complex data models.
Example of incorrect join:
SELECT e.employee_name, d.department_name
FROM employees e
JOIN departments d ON e.employee_id = d.department_id;
In this example, the join condition incorrectly equates the employee_id with the department_id, which are likely not equivalent. A corrected version might look like this:
SELECT e.employee_name, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.department_id;
To prevent join-related logical errors, carefully review your data model and ensure that you’re using the correct columns for join conditions. It’s often helpful to start with smaller result sets and gradually build up to more complex joins, verifying the results at each step.
Misuse of Aggregate Functions
Another common logical error involves the misuse of aggregate functions like COUNT, SUM, AVG, etc. These functions operate on groups of rows, and their improper use can lead to incorrect results or error messages about ungrouped columns.
Example of incorrect usage:
SELECT department, employee_name, AVG(salary)
FROM employees;
This query will produce an error because it attempts to display non-aggregated columns (department and employee_name) alongside an aggregate function (AVG(salary)) without a GROUP BY clause. A corrected version might look like this:
SELECT department, AVG(salary)
FROM employees
GROUP BY department;
To avoid errors with aggregate functions, ensure that all non-aggregated columns in the SELECT list are included in the GROUP BY clause. If you need to include individual employee details, consider using subqueries or window functions, depending on your specific requirements.
Incorrect Use of WHERE vs. HAVING Clauses
Confusion between WHERE and HAVING clauses can lead to logical errors in SQL queries. The WHERE clause filters rows before any grouping occurs, while the HAVING clause filters groups after grouping has taken place.
Example of incorrect usage:
SELECT department, COUNT(*) as employee_count
FROM employees
HAVING COUNT(*) > 5;
While this query will execute, it may not produce the intended results. A more correct version would be:
SELECT department, COUNT(*) as employee_count
FROM employees
GROUP BY department
HAVING COUNT(*) > 5;
To prevent this type of error, remember that WHERE is used for filtering individual rows, while HAVING is used for filtering groups. Use WHERE for conditions on non-aggregated columns and HAVING for conditions on aggregated results.
Data Type Mismatch Errors
Data type mismatch errors occur when you try to perform operations or comparisons between incompatible data types. These errors can cause unexpected results or prevent queries from executing altogether.
Comparing Strings to Numbers
One common data type mismatch error involves comparing string values to numeric values without proper conversion.
Example of incorrect comparison:
SELECT * FROM products WHERE price > '100';
In this case, the string ‘100’ is being compared to a numeric price column. While some database systems might perform implicit conversion, it’s better to explicitly convert the types to ensure consistent behavior:
SELECT * FROM products WHERE price > CAST('100' AS DECIMAL);
To avoid data type mismatch errors, always ensure that you’re comparing or operating on compatible data types. When necessary, use explicit conversion functions like CAST() or database-specific functions to convert between types.
Inserting Incorrect Data Types
Another common data type mismatch error occurs when attempting to insert data of the wrong type into a column.
Example of incorrect insert:
INSERT INTO employees (employee_id, hire_date, salary)
VALUES ('EMP123', '2023-01-15', 'Fifty Thousand');
This insert statement may fail if employee_id is expected to be numeric, or if salary is defined as a numeric column. A corrected version might look like this:
INSERT INTO employees (employee_id, hire_date, salary)
VALUES (123, '2023-01-15', 50000);
To prevent these errors, always verify the data types of your table columns and ensure that the values you’re inserting match those types. When working with user input or data from external sources, implement validation and type conversion as needed before performing database operations.
Constraint Violation Errors
Constraint violation errors occur when an SQL operation attempts to modify data in a way that violates the defined constraints on the database. These constraints are designed to maintain data integrity and enforce business rules.
Primary Key Violations
Primary key constraints ensure that each row in a table can be uniquely identified. Attempting to insert a duplicate primary key value will result in an error.
Example of a primary key violation:
INSERT INTO employees (employee_id, first_name, last_name)
VALUES (101, 'John', 'Doe');
-- Attempting to insert another row with the same employee_id
INSERT INTO employees (employee_id, first_name, last_name)
VALUES (101, 'Jane', 'Smith');
The second INSERT statement will fail if employee_id is the primary key and an employee with ID 101 already exists. To resolve this, ensure that you’re inserting unique values for primary key columns, or use a mechanism like auto-incrementing IDs to generate unique values automatically.
Foreign Key Violations
Foreign key constraints maintain referential integrity between tables. Attempting to insert a value into a foreign key column that doesn’t exist in the referenced table will result in an error.
Example of a foreign key violation:
INSERT INTO orders (order_id, customer_id, order_date)
VALUES (1001, 5000, '2023-03-15');
This INSERT statement will fail if customer_id is a foreign key referencing the customers table, and no customer with ID 5000 exists. To fix this, ensure that the referenced value exists in the parent table before inserting or updating rows in the child table.
Check Constraint Violations
Check constraints enforce specific conditions on column values. Attempting to insert or update data that doesn’t meet these conditions will result in an error.
Example of a check constraint violation:
-- Assuming a check constraint: CHECK (salary > 0)
UPDATE employees SET salary = -5000 WHERE employee_id = 101;
This UPDATE statement will fail if there’s a check constraint ensuring that salary values are positive. To resolve check constraint violations, ensure that the data you’re inserting or updating meets all defined check constraints.
Performance-Related Issues
While not strictly errors, performance-related issues can significantly impact the usability and efficiency of your SQL queries. Addressing these issues is crucial for maintaining a responsive and scalable database system.
Missing Indexes
Queries that perform full table scans instead of using indexes can be extremely slow, especially on large tables. Identifying and creating appropriate indexes can dramatically improve query performance.
Example of a query that might benefit from an index:
SELECT * FROM orders WHERE order_date BETWEEN '2023-01-01' AND '2023-12-31';
If this query is slow and frequently used, you might consider adding an index on the order_date column:
CREATE INDEX idx_order_date ON orders (order_date);
To identify missing indexes, use your database system’s query execution plan tools to analyze slow queries. Look for full table scans or high-cost operations that could be optimized with appropriate indexes.
Inefficient JOIN Operations
Poorly structured JOIN operations can lead to performance issues, especially when dealing with large datasets or complex query logic.
Example of a potentially inefficient query:
SELECT *
FROM orders o
JOIN order_items oi ON o.order_id = oi.order_id
JOIN products p ON oi.product_id = p.product_id
JOIN customers c ON o.customer_id = c.customer_id;
This query might be slow if it’s returning a large number of columns or rows. To optimize it, consider:
- Selecting only necessary columns instead of using SELECT *.
- Adding appropriate indexes on join columns.
- Reordering the joins to start with the most restrictive table.
To improve JOIN performance, analyze your query execution plans, ensure you have appropriate indexes on join columns, and consider denormalizing data if necessary for frequently run queries.
Subquery Performance
Subqueries can sometimes lead to performance issues, especially when they’re executed repeatedly for each row of the outer query (known as correlated subqueries).
Example of a potentially slow correlated subquery:
SELECT employee_name,
(SELECT department_name
FROM departments
WHERE departments.dept_id = employees.dept_id) AS department
FROM employees;
This query might be slow for large employee tables. An alternative using a JOIN could be more efficient:
SELECT e.employee_name, d.department_name
FROM employees e
LEFT JOIN departments d ON e.dept_id = d.dept_id;
To optimize subquery performance, consider rewriting correlated subqueries as JOINs where possible, or use derived tables or common table expressions (CTEs) for complex subqueries that are reused multiple times in a query.
Concurrency and Locking Issues
In multi-user database environments, concurrency control is crucial to maintain data integrity while allowing simultaneous access. However, this can sometimes lead to errors or unexpected behavior.
Deadlocks
Deadlocks occur when two or more transactions are waiting for each other to release locks, resulting in a circular dependency. Most database systems will automatically detect and resolve deadlocks by rolling back one of the transactions, but this can lead to errors in your application.
Example scenario that might cause a deadlock:
Transaction 1:
BEGIN;
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
UPDATE accounts SET balance = balance + 100 WHERE account_id = 2;
COMMIT;
Transaction 2 (running concurrently):
BEGIN;
UPDATE accounts SET balance = balance - 200 WHERE account_id = 2;
UPDATE accounts SET balance = balance + 200 WHERE account_id = 1;
COMMIT;
If these transactions acquire locks in opposite orders, they might deadlock. To prevent deadlocks:
- Ensure consistent ordering of operations across transactions.
- Keep transactions as short as possible.
- Use appropriate isolation levels.
- Implement retry logic in your application to handle deadlock errors.
Lock Timeouts
Lock timeouts occur when a transaction waits too long to acquire a lock on a resource. This can happen in high-concurrency environments or when long-running transactions hold locks for extended periods.
Example of a query that might cause or experience lock timeouts:
BEGIN;
SELECT * FROM large_table WITH (TABLOCKX);
-- Perform long-running operation
COMMIT;
This query acquires an exclusive table lock, which might cause other transactions to time out while waiting. To mitigate lock timeout issues:
- Minimize the duration of transactions, especially those acquiring exclusive locks.
- Use appropriate isolation levels and locking hints judiciously.
- Consider using row-level locking instead of table-level locking when possible.
- Implement retry logic in your application to handle lock timeout errors.
Conclusion
SQL errors are an inevitable part of database development and management. By understanding common errors and their solutions, you can significantly improve your ability to write efficient, correct SQL queries and maintain robust database systems. Remember to use your database system’s built-in tools for analyzing query execution plans and performance metrics to identify and resolve issues proactively.
Regular practice, careful attention to syntax and logic, and a solid understanding of your data model will help you avoid many common pitfalls. When errors do occur, approach them methodically: read error messages carefully, check your syntax, verify your logic, and test your queries with smaller datasets before scaling up to larger operations.
By following the best practices and solutions outlined in this guide, you’ll be better equipped to handle SQL errors efficiently and write more reliable database code. Continue to expand your knowledge of SQL and database management, as the field is constantly evolving with new features and optimizations being introduced regularly.
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, it is provided for educational purposes only. Database systems and SQL implementations may vary, and specific error messages or behaviors might differ across platforms. Always refer to your database system’s official documentation for the most up-to-date and accurate information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.