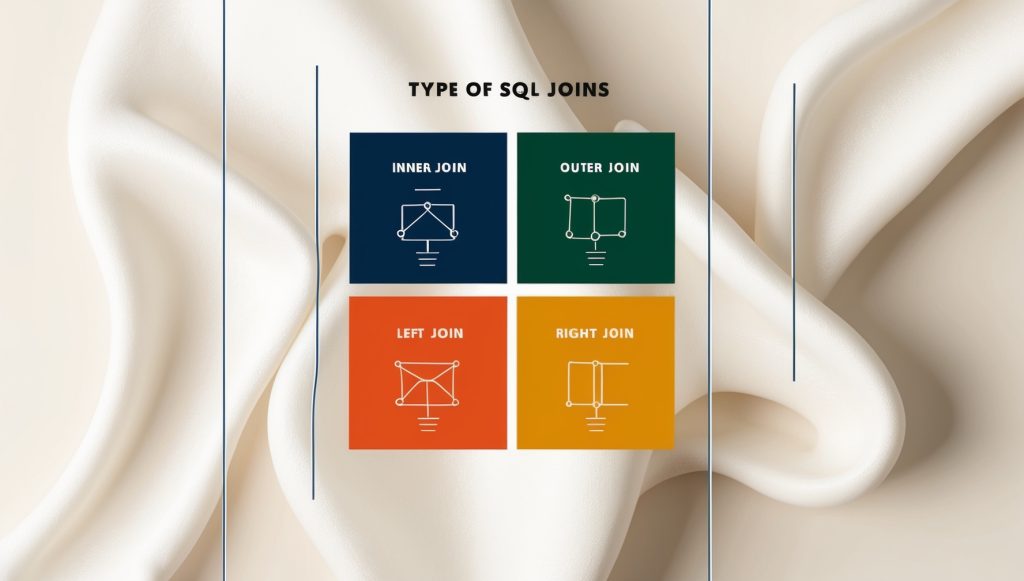
Understanding SQL Joins – Inner, Outer, Left, Right
Structured Query Language (SQL) is the backbone of modern database management systems, enabling developers and data analysts to efficiently manipulate and retrieve data from relational databases. Among the many powerful features of SQL, joins stand out as a crucial concept for working with multiple tables and extracting meaningful insights from complex data structures. This comprehensive guide delves into the intricacies of SQL joins, exploring the various types and their practical applications. Whether you’re a beginner looking to grasp the fundamentals or an experienced professional seeking to refine your skills, this blog post will provide you with a thorough understanding of SQL joins and how to leverage them effectively in your database operations.
The Importance of SQL Joins
In relational databases, data is often distributed across multiple tables to maintain organization and reduce redundancy. While this structure enhances data integrity and efficiency, it also necessitates a mechanism to combine related information from different tables when querying the database. This is where SQL joins come into play. Joins allow us to establish connections between tables based on related columns, enabling us to retrieve and analyze data from multiple sources in a single query.
Understanding SQL joins is crucial for several reasons. First, it empowers developers and analysts to construct complex queries that can extract valuable insights from seemingly disparate data sets. Second, mastering joins leads to more efficient database design and optimization, as it allows for the creation of normalized database structures without sacrificing the ability to retrieve comprehensive information. Lastly, proficiency in SQL joins is a highly sought-after skill in the job market, making it an essential tool for anyone working with databases or aspiring to enter the field of data management and analysis.
Types of SQL Joins
SQL offers several types of joins, each serving a specific purpose and yielding different results based on how the data from multiple tables is combined. The main types of SQL joins are:
- Inner Join
- Left (Outer) Join
- Right (Outer) Join
- Full (Outer) Join
- Cross Join
- Self Join
In the following sections, we will explore each of these join types in detail, providing explanations, examples, and practical use cases to help you understand when and how to apply them in your SQL queries.
Inner Join: The Fundamental Connection
Definition and Purpose
The inner join is perhaps the most commonly used and straightforward type of SQL join. It returns only the rows that have matching values in both tables being joined. In other words, an inner join creates a result set that includes only the data where there is a direct correlation between the specified columns of the participating tables.
Syntax and Usage
The basic syntax for an inner join is as follows:
SELECT column_list
FROM table1
INNER JOIN table2
ON table1.column_name = table2.column_name;
Let’s break down this syntax:
SELECT column_list
: Specifies the columns you want to retrieve from the joined tables.FROM table1
: Indicates the first table in the join operation.INNER JOIN table2
: Specifies the second table and the type of join (inner).ON table1.column_name = table2.column_name
: Defines the join condition, which determines how the tables are related.
Example and Explanation
To illustrate the concept of an inner join, let’s consider a scenario with two tables: Employees
and Departments
. We’ll use these tables throughout our examples for consistency.
-- Create the Employees table
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
DepartmentID INT
);
-- Create the Departments table
CREATE TABLE Departments (
DepartmentID INT PRIMARY KEY,
DepartmentName VARCHAR(50)
);
-- Insert sample data into Employees
INSERT INTO Employees (EmployeeID, FirstName, LastName, DepartmentID)
VALUES
(1, 'John', 'Doe', 1),
(2, 'Jane', 'Smith', 2),
(3, 'Mike', 'Johnson', 1),
(4, 'Emily', 'Brown', 3),
(5, 'David', 'Wilson', NULL);
-- Insert sample data into Departments
INSERT INTO Departments (DepartmentID, DepartmentName)
VALUES
(1, 'HR'),
(2, 'IT'),
(3, 'Finance'),
(4, 'Marketing');
Now, let’s perform an inner join to retrieve employees along with their department names:
SELECT e.EmployeeID, e.FirstName, e.LastName, d.DepartmentName
FROM Employees e
INNER JOIN Departments d
ON e.DepartmentID = d.DepartmentID;
This query will produce the following result:
EmployeeID | FirstName | LastName | DepartmentName |
---|---|---|---|
1 | John | Doe | HR |
2 | Jane | Smith | IT |
3 | Mike | Johnson | HR |
4 | Emily | Brown | Finance |
Notice that David Wilson, who has a NULL value for DepartmentID, is not included in the result set. This is because an inner join only returns rows where there is a match in both tables. Additionally, the Marketing department, which has no associated employees, is also excluded from the result.
Use Cases and Considerations
Inner joins are particularly useful when you need to:
- Combine related data from multiple tables where a direct relationship exists.
- Filter out records that don’t have corresponding entries in both tables.
- Perform calculations or aggregations that require data from multiple related tables.
While inner joins are powerful, it’s important to consider their limitations. They exclude any rows that don’t have matches in both tables, which may lead to data loss if you need to preserve all records from one or both tables. In such cases, you might need to consider outer joins, which we’ll explore in the following sections.
Left (Outer) Join: Preserving the Left Table
Definition and Purpose
A left join, also known as a left outer join, returns all rows from the left table (the first table in the join statement) and the matched rows from the right table. If there is no match in the right table, the result will contain NULL values for columns from the right table. This type of join is particularly useful when you want to ensure that all records from one table are included in the result set, regardless of whether they have corresponding entries in the joined table.
Syntax and Usage
The basic syntax for a left join is as follows:
SELECT column_list
FROM table1
LEFT JOIN table2
ON table1.column_name = table2.column_name;
The structure is similar to an inner join, with the key difference being the LEFT JOIN
keyword instead of INNER JOIN
.
Example and Explanation
Continuing with our Employees
and Departments
tables from the previous example, let’s perform a left join to retrieve all employees, including those without an assigned department:
SELECT e.EmployeeID, e.FirstName, e.LastName, d.DepartmentName
FROM Employees e
LEFT JOIN Departments d
ON e.DepartmentID = d.DepartmentID;
This query will produce the following result:
EmployeeID | FirstName | LastName | DepartmentName |
---|---|---|---|
1 | John | Doe | HR |
2 | Jane | Smith | IT |
3 | Mike | Johnson | HR |
4 | Emily | Brown | Finance |
5 | David | Wilson | NULL |
Notice that David Wilson, who has a NULL value for DepartmentID, is now included in the result set. The DepartmentName for his row is NULL, indicating that there is no matching department for this employee.
Use Cases and Considerations
Left joins are particularly useful in scenarios where you need to:
- Retrieve all records from a primary table, even if they don’t have corresponding entries in a related table.
- Identify missing relationships or gaps in your data.
- Generate reports that include all entities from one table, with additional information from another table where available.
For example, you might use a left join to create a report of all employees, including their department information if available, without excluding employees who haven’t been assigned to a department yet.
It’s important to note that the order of tables in a left join matters. The table specified in the FROM
clause is considered the left table, and all of its rows will be included in the result set. If you need to preserve all rows from the other table instead, you would use a right join, which we’ll discuss in the next section.
Right (Outer) Join: Preserving the Right Table
Definition and Purpose
A right join, also known as a right outer join, is the mirror image of a left join. It returns all rows from the right table (the second table in the join statement) and the matched rows from the left table. If there is no match in the left table, the result will contain NULL values for columns from the left table. This type of join is less commonly used than left joins but can be valuable in specific scenarios where you need to ensure all records from the second table are included in the result set.
Syntax and Usage
The basic syntax for a right join is as follows:
SELECT column_list
FROM table1
RIGHT JOIN table2
ON table1.column_name = table2.column_name;
The structure is similar to a left join, with the key difference being the RIGHT JOIN
keyword instead of LEFT JOIN
.
Example and Explanation
Using our Employees
and Departments
tables, let’s perform a right join to retrieve all departments, including those without any assigned employees:
SELECT e.EmployeeID, e.FirstName, e.LastName, d.DepartmentName
FROM Employees e
RIGHT JOIN Departments d
ON e.DepartmentID = d.DepartmentID;
This query will produce the following result:
EmployeeID | FirstName | LastName | DepartmentName |
---|---|---|---|
1 | John | Doe | HR |
3 | Mike | Johnson | HR |
2 | Jane | Smith | IT |
4 | Emily | Brown | Finance |
NULL | NULL | NULL | Marketing |
Notice that the Marketing department, which has no associated employees, is now included in the result set. The employee columns for this row contain NULL values, indicating that there are no matching employees for this department.
Use Cases and Considerations
Right joins are useful in scenarios where you need to:
- Retrieve all records from a secondary table, even if they don’t have corresponding entries in the primary table.
- Identify unused or unassigned entities in your database.
- Generate reports that include all categories or classifications from one table, with associated data from another table where available.
For example, you might use a right join to create a report of all departments, including information about employees assigned to each department, without excluding departments that currently have no employees.
It’s worth noting that right joins can often be rewritten as left joins by simply switching the order of the tables in the query. For instance, the following query would produce the same result as our right join example:
SELECT e.EmployeeID, e.FirstName, e.LastName, d.DepartmentName
FROM Departments d
LEFT JOIN Employees e
ON d.DepartmentID = e.DepartmentID;
Due to this interchangeability, many SQL developers prefer to consistently use left joins for clarity and maintainability, adjusting the table order as needed to achieve the desired result.
Full (Outer) Join: Combining All Data
Definition and Purpose
A full join, also known as a full outer join, combines the results of both left and right outer joins. It returns all rows from both tables, regardless of whether there is a match between them. When there is no match, the result will contain NULL values for columns from the table that doesn’t have a corresponding row. This type of join is particularly useful when you want to see all possible combinations of data from two tables, including unmatched rows from both sides.
Syntax and Usage
The basic syntax for a full join is as follows:
SELECT column_list
FROM table1
FULL JOIN table2
ON table1.column_name = table2.column_name;
Note that not all database management systems support the FULL JOIN
syntax. In such cases, you can simulate a full join using a combination of left join, union, and right join.
Example and Explanation
Let’s perform a full join on our Employees
and Departments
tables to see all employees and all departments, regardless of whether there are matches between them:
SELECT e.EmployeeID, e.FirstName, e.LastName, d.DepartmentName
FROM Employees e
FULL JOIN Departments d
ON e.DepartmentID = d.DepartmentID;
This query will produce the following result:
EmployeeID | FirstName | LastName | DepartmentName |
---|---|---|---|
1 | John | Doe | HR |
2 | Jane | Smith | IT |
3 | Mike | Johnson | HR |
4 | Emily | Brown | Finance |
5 | David | Wilson | NULL |
NULL | NULL | NULL | Marketing |
Notice that this result set includes:
- All employees with their corresponding department names (when available)
- David Wilson, who has no assigned department (NULL in the DepartmentName column)
- The Marketing department, which has no assigned employees (NULL in the employee columns)
Use Cases and Considerations
Full joins are particularly useful when you need to:
- See all possible combinations of data from two tables, including unmatched rows from both sides.
- Identify discrepancies or gaps in relational data.
- Perform a comprehensive analysis that requires visibility into all data points, regardless of their relationships.
For example, you might use a full join to audit your database, ensuring that all employees are assigned to valid departments and all departments have at least one employee assigned. The NULL values in the result set would quickly highlight any inconsistencies.
It’s important to note that full joins can potentially return large result sets, especially when working with tables that have many unmatched rows. This can impact query performance and may require additional filtering or aggregation to extract meaningful insights from the data.
If your database system doesn’t support the FULL JOIN
syntax, you can achieve the same result using a combination of left and right joins with a UNION
operation:
SELECT e.EmployeeID, e.FirstName, e.LastName, d.DepartmentName
FROM Employees e
LEFT JOIN Departments d ON e.DepartmentID = d.DepartmentID
UNION
SELECT e.EmployeeID, e.FirstName, e.LastName, d.DepartmentName
FROM Employees e
RIGHT JOIN Departments d ON e.DepartmentID = d.DepartmentID
WHERE e.EmployeeID IS NULL;
This alternative approach first performs a left join to get all employees and their departments, then unions it with a right join that only includes departments without employees. The result is equivalent to a full join.
Cross Join: Cartesian Product of Tables
Definition and Purpose
A cross join, also known as a Cartesian join, produces a result set that is the Cartesian product of the two tables involved in the join. In other words, it combines each row from the first table with every row from the second table, resulting in a potentially large number of rows. Unlike other join types, a cross join does not require a join condition.
Syntax and Usage
The basic syntax for a cross join is as follows:
SELECT column_list
FROM table1
CROSS JOIN table2;
Alternatively, you can achieve the same result using an older syntax:
SELECT column_list
FROM table1, table2;
Example and Explanation
Let’s perform a cross join between a simplified version of our Employees
and Departments
tables:
-- Create simplified tables
CREATE TABLE SimpleEmployees (
EmployeeID INT PRIMARY KEY,
EmployeeName VARCHAR(50)
);
CREATE TABLE SimpleDepartments (
DepartmentID INT PRIMARY KEY,
DepartmentName VARCHAR(50)
);
-- Insert sample data
INSERT INTO SimpleEmployees (EmployeeID, EmployeeName)
VALUES (1, 'John Doe'), (2, 'Jane Smith'), (3, 'Mike Johnson');
INSERT INTO SimpleDepartments (DepartmentID, DepartmentName)
VALUES (1, 'HR'), (2, 'IT');
-- Perform a cross join
SELECT e.EmployeeID, e.EmployeeName, d.DepartmentID, d.DepartmentName
FROM SimpleEmployees e
CROSS JOIN SimpleDepartments d;
This query will produce the following result:
Here is the data in a tabular format:
EmployeeID | EmployeeName | DepartmentID | DepartmentName |
---|---|---|---|
1 | John Doe | 1 | HR |
1 | John Doe | 2 | IT |
2 | Jane Smith | 1 | HR |
2 | Jane Smith | 2 | IT |
3 | Mike Johnson | 1 | HR |
3 | Mike Johnson | 2 | IT |
As you can see, the cross join has created all possible combinations of employees and departments, regardless of any actual relationships between them.
Use Cases and Considerations
Cross joins are less commonly used than other join types due to their potential to generate large result sets. However, they can be useful in certain scenarios:
1. Generating combinations: When you need to create all possible combinations of items from two sets.
2. Creating test data: Cross joins can be used to quickly generate large volumes of test data.
3. Solving specific mathematical or analytical problems that require a Cartesian product.
It’s crucial to use cross joins cautiously, as they can produce very large result sets that may impact query performance, especially when working with large tables. Always consider whether a cross join is truly necessary for your specific use case, and be prepared to apply additional filtering or limiting clauses to manage the size of the result set.
Self Join: Joining a Table to Itself
Definition and Purpose
A self join is a regular join, but the table is joined with itself. This type of join is used when a table has a column that references another row in the same table. Self joins are particularly useful for querying hierarchical or tree-like data structures within a single table.
Syntax and Usage
The syntax for a self join is similar to other joins, but the same table is referenced twice with different aliases:
SELECT column_list
FROM table1 t1
JOIN table1 t2
ON t1.column_name = t2.column_name;
Example and Explanation
Let’s consider an `Employees` table that includes a `ManagerID` column, which references the `EmployeeID` of the employee’s manager:
-- Create Employees table with ManagerID
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
ManagerID INT
);
-- Insert sample data
INSERT INTO Employees (EmployeeID, FirstName, LastName, ManagerID)
VALUES
(1, 'John', 'Doe', NULL),
(2, 'Jane', 'Smith', 1),
(3, 'Mike', 'Johnson', 1),
(4, 'Emily', 'Brown', 2),
(5, 'David', 'Wilson', 2);
-- Perform a self join to get employees and their managers
SELECT
e1.EmployeeID,
e1.FirstName || ' ' || e1.LastName AS Employee,
e2.FirstName || ' ' || e2.LastName AS Manager
FROM Employees e1
LEFT JOIN Employees e2 ON e1.ManagerID = e2.EmployeeID;
This query will produce the following result:
EmployeeID | Employee | Manager |
---|---|---|
1 | John Doe | NULL |
2 | Jane Smith | John Doe |
3 | Mike Johnson | John Doe |
4 | Emily Brown | Jane Smith |
5 | David Wilson | Jane Smith |
In this example, we’ve used a left join to ensure that all employees are included in the result, even if they don’t have a manager (like John Doe, who is presumably the top-level manager).
Use Cases and Considerations
Self joins are particularly useful for:
- Querying hierarchical data structures, such as employee-manager relationships or category-subcategory structures.
- Finding pairs or relationships between rows in the same table.
- Comparing rows within the same table.
When using self joins, it’s important to use clear aliases to distinguish between the two instances of the table in your query. This helps prevent ambiguity and makes the query more readable and maintainable.
Optimizing Join Performance
While joins are powerful tools for querying relational databases, they can also be computationally expensive, especially when dealing with large datasets. Here are some tips to optimize the performance of your SQL joins:
- Use appropriate indexes: Ensure that the columns used in join conditions are properly indexed. This can significantly speed up the join operation.
- Join order matters: In complex queries with multiple joins, the order in which tables are joined can affect performance. Start with the largest dataset and join smaller tables to it.
- Limit data before joining: Use WHERE clauses to filter data before performing joins, reducing the amount of data that needs to be processed.
- Avoid unnecessary columns: Only select the columns you need in your result set. Avoiding
SELECT *
can improve query performance. - Use subqueries or derived tables: In some cases, using subqueries or derived tables to pre-filter or aggregate data before joining can improve performance.
- Consider denormalization: For read-heavy operations, denormalizing data (i.e., storing redundant data across tables) can reduce the need for joins and improve query speed.
- Use appropriate join types: Choose the right type of join for your specific use case. Avoid using more complex join types (like full outer joins) when simpler ones will suffice.
- Analyze and optimize query plans: Use your database’s query analyzer to understand how your joins are being executed and optimize accordingly.
Conclusion
SQL joins are fundamental to working with relational databases, allowing us to combine data from multiple tables in meaningful ways. By understanding the different types of joins – inner, left, right, full, cross, and self joins – you can effectively query and analyze complex data structures.
Each type of join serves a specific purpose:
- Inner joins are used for matching records between tables.
- Outer joins (left, right, and full) allow you to include unmatched records from one or both tables.
- Cross joins generate all possible combinations between two tables.
- Self joins are useful for querying hierarchical data within a single table.
Mastering these join types and understanding when to use each one will greatly enhance your ability to work with relational databases. Remember to consider query performance, especially when working with large datasets, and apply optimization techniques as needed.
As you continue to work with SQL, practice using different join types in various scenarios. This hands-on experience will deepen your understanding and help you become more proficient in constructing efficient and effective database queries.
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, it is provided for educational purposes only. Database structures and SQL syntax may vary between different database management systems. Always refer to the documentation specific to your database system for the most accurate and up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.