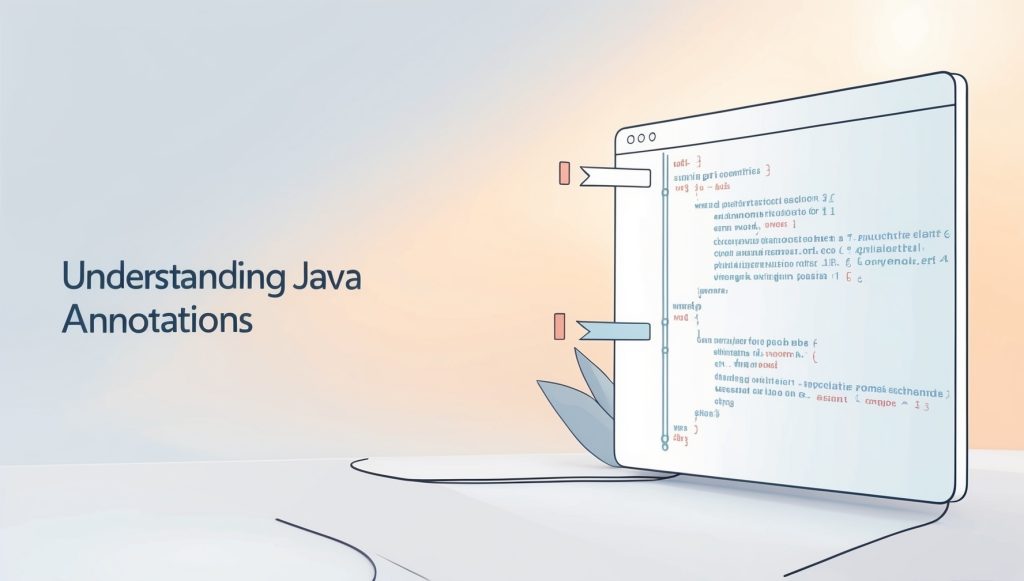
Understanding Java Annotations
Java annotations are a powerful tool that can significantly enhance your code’s readability, maintainability, and functionality. Introduced in Java 5, annotations provide a way to add metadata to your code, which can then be used by the compiler, runtime environment, or other tools. In this comprehensive guide, we’ll delve deep into Java annotations, focusing on custom annotations and their practical applications.
What are Java Annotations?
Annotations in Java are a form of metadata that provides additional information about the code to the compiler, runtime, or other tools. They are represented by the @
symbol followed by the annotation name. Some common built-in annotations in Java include @Override
, @Deprecated
, and @SuppressWarnings
. Annotations can be applied to various program elements, such as classes, methods, fields, parameters, and more.
Types of Annotations
There are three main types of annotations in Java:
- Marker Annotations: These are the simplest form of annotations and do not contain any members. They simply act as a marker or flag to indicate something about the annotated element. For example, the
@Override
annotation is a marker annotation that indicates that a method is overriding a method from a superclass. - Single-Value Annotations: These annotations contain a single member, which is typically a string or a primitive value. For example, the
@SuppressWarnings
annotation is a single-value annotation that takes a string array as its value, specifying the types of warnings to suppress. - Full Annotations: These annotations can have multiple members, allowing you to provide more detailed information about the annotated element. For example, the
@Test
annotation in JUnit is a full annotation that can have various members, such asexpected
andtimeout
.
Creating Custom Annotations
One of the most powerful features of Java annotations is the ability to create your own custom annotations. This allows you to define your own metadata that can be used to enforce specific rules, generate code, or perform other tasks.
To create a custom annotation, you use the @interface
keyword, followed by the annotation name. You can then define members within the annotation, similar to how you define methods in an interface.
Java
public @interface MyAnnotation {
String value() default "";
int count() default 0;
}
In this example, we’ve created a custom annotation called MyAnnotation
with two members: value
and count
. The value
member is a string with a default value of ""
, and the count
member is an integer with a default value of 0
.
Applying Annotations
Once you’ve created a custom annotation, you can apply it to various program elements using the @
symbol followed by the annotation name.
Java
@MyAnnotation(value = "Hello", count = 1)
public class MyClass {
// ...
}
In this example, we’ve applied the MyAnnotation
annotation to the MyClass
class, providing values for both the value
and count
members.
Annotation Retention Policies
Java annotations have different retention policies that determine how long they are retained in the code. There are three retention policies:
- SOURCE: Annotations with this retention policy are only retained in the source code and are discarded by the compiler.
- CLASS: Annotations with this retention policy are retained in the compiled class files but are not available at runtime.
- RUNTIME: Annotations with this retention policy are retained in the compiled class files and are available at runtime.
You can specify the retention policy of a custom annotation using the @Retention
meta-annotation.
Java
@Retention(RetentionPolicy.RUNTIME)
public @interface MyAnnotation {
// ...
}
In this example, we’ve specified that the MyAnnotation
annotation should be retained at runtime.
Annotation Targets
Java annotations can also have different targets that specify which program elements they can be applied to. You can specify the target of a custom annotation using the @Target
meta-annotation.
Java
@Target(ElementType.METHOD)
public @interface MyAnnotation {
// ...
}
In this example, we’ve specified that the MyAnnotation
annotation can only be applied to methods.
Processing Annotations
To process annotations, you can use reflection to access the annotation information at runtime. The java.lang.reflect
package provides various classes and methods for working with annotations.
Java
public class MyProcessor {
public static void main(String[] args) {
MyClass obj = new MyClass();
Class<?> cls = obj.getClass();
if (cls.isAnnotationPresent(MyAnnotation.class)) {
MyAnnotation annotation = cls.getAnnotation(MyAnnotation.class);
System.out.println(annotation.value());
System.out.println(annotation.count());
}
}
}
In this example, we’ve used reflection to access the MyAnnotation
annotation on the MyClass
class and print the values of its members.
Applications of Custom Annotations
Custom annotations have a wide range of applications in Java development. Here are some examples:
- Validation: You can use custom annotations to define validation rules for your code. For example, you could create an annotation called
@NotNull
that ensures that a field is not null. - Code Generation: You can use custom annotations to generate code automatically. For example, you could create an annotation called
@GenerateGetterSetter
that generates getter and setter methods for a field. - Dependency Injection: Frameworks like Spring use annotations for dependency injection. For example, the
@Autowired
annotation in Spring is used to inject dependencies into a class. - Testing: Testing frameworks like JUnit use annotations to define test methods and test suites. For example, the
@Test
annotation in JUnit is used to mark a method as a test method. - Logging: You can use custom annotations to add logging to your code. For example, you could create an annotation called
@Log
that logs the execution of a method.
Example: Using Custom Annotations for Validation
Let’s say we want to create a custom annotation called @Range
that validates whether an integer field falls within a specific range. Here’s how we can define the annotation:
Java
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Range {
int min() default 0;
int max() default 100;
}
Now, we can apply this annotation to an integer field in our class:
Java
public class MyData {
@Range(min = 1, max = 10)
private int value;
// ...
}
We can then use reflection to process this annotation and validate the field’s value at runtime:
Java
public class MyValidator {
public static void validate(Object obj) throws IllegalAccessException {
Class<?> cls = obj.getClass();
for (Field field : cls.getDeclaredFields()) {
if (field.isAnnotationPresent(Range.class)) {
Range range = field.getAnnotation(Range.class);
field.setAccessible(true);
int value = field.getInt(obj);
if (value < range.min() || value > range.max()) {
throw new IllegalArgumentException("Field " + field.getName() + " is out of range.");
}
}
}
}
}
This is a simple example of how custom annotations can be used for validation. You can create more complex annotations to validate different types of data and enforce various rules.
Conclusion
Java annotations are a versatile and powerful tool that can greatly enhance your code. Custom annotations, in particular, allow you to define your own metadata and use it for various purposes, such as validation, code generation, and dependency injection. By understanding how to create and process custom annotations, you can significantly improve your code’s readability, maintainability, and functionality.
Disclaimer: The information provided in this blog is for educational purposes only. While we strive for accuracy, we cannot guarantee that all information is up-to-date or error-free. Please report any inaccuracies so we can correct them promptly.