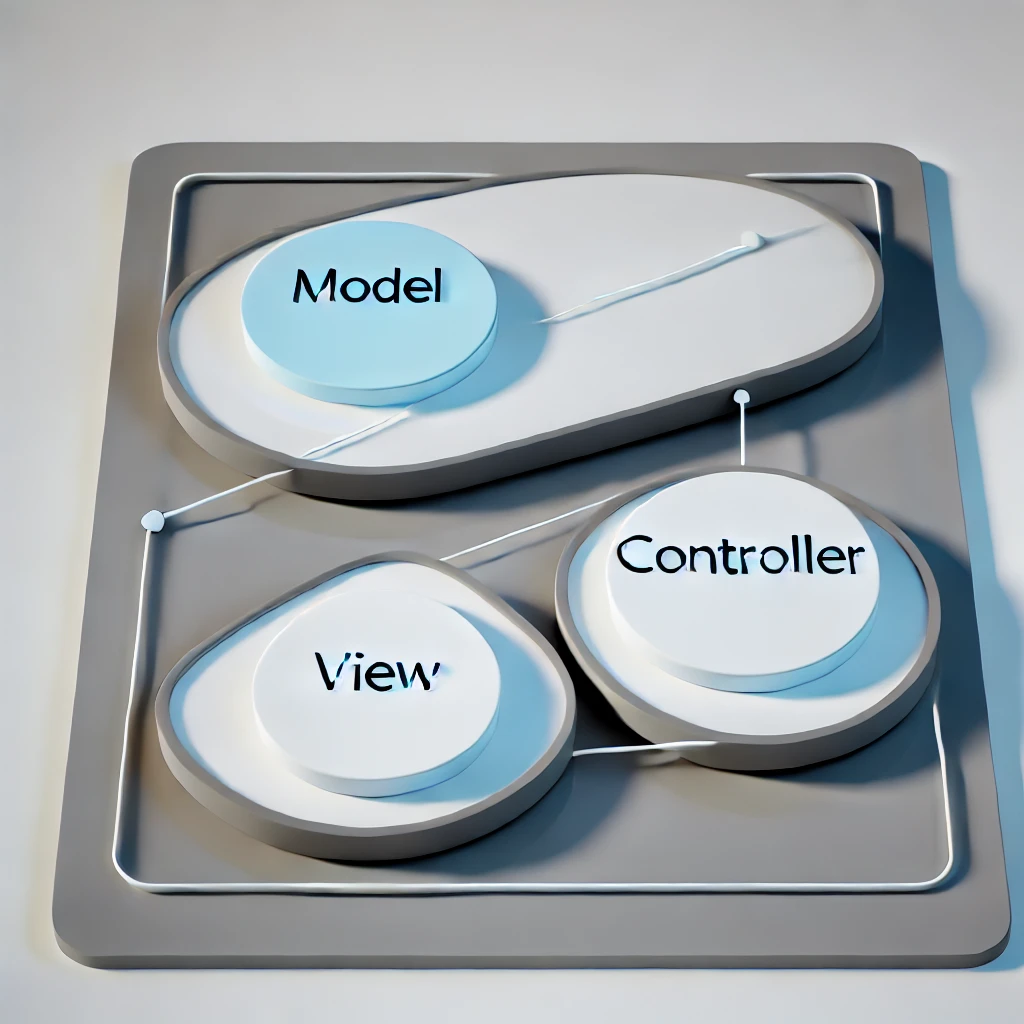
The History of MVC Architecture
The Model-View-Controller (MVC) architectural pattern stands as one of the most influential and enduring design patterns in software development history. Born in the late 1970s, MVC has evolved from its humble beginnings at Xerox PARC to become a fundamental concept in modern web development frameworks. This architectural pattern has revolutionized how developers structure applications by promoting separation of concerns, code reusability, and maintainable software design. Through its journey spanning over four decades, MVC has adapted to changing technological landscapes while maintaining its core principles, making it an essential study for both novice and experienced developers. Understanding the historical context and evolution of MVC provides valuable insights into why it remains relevant in today’s complex software development ecosystem.
Origins at Xerox PARC
The story of MVC begins at Xerox PARC (Palo Alto Research Center) in 1978-79, where Trygve Reenskaug introduced the pattern while working on Smalltalk-80. The original implementation was conceived to solve a fundamental problem in graphical user interface (GUI) development: how to connect user interactions with their underlying data models and visual representations. During his time at PARC, Reenskaug observed that traditional software architectures struggled to maintain clear boundaries between data, user interface, and business logic. This observation led to the development of a pattern that would separate these concerns while maintaining their interconnections in a structured manner. The innovative environment at Xerox PARC, which had already produced groundbreaking technologies like the graphical user interface and the mouse, provided the perfect incubator for this revolutionary software architecture pattern.
The Core Concepts of Original MVC
Components and Their Responsibilities
The original MVC pattern consisted of three distinct components, each with well-defined responsibilities:
- Model: Represents the application’s data and business logic
- View: Handles the visual representation of the data
- Controller: Manages user input and updates both Model and View accordingly
Here’s a simple representation of the original MVC pattern in Python:
class Model:
def __init__(self):
self._data = None
self._observers = [#91;]#93;
def set_data(self, data):
self._data = data
self._notify_observers()
def get_data(self):
return self._data
def add_observer(self, observer):
self._observers.append(observer)
def _notify_observers(self):
for observer in self._observers:
observer.update()
class View:
def __init__(self, model):
self.model = model
self.model.add_observer(self)
def update(self):
print(f"View: Displaying data: {self.model.get_data()}")
class Controller:
def __init__(self, model):
self.model = model
def handle_input(self, data):
self.model.set_data(data)
# Usage Example
model = Model()
view = View(model)
controller = Controller(model)
controller.handle_input("Hello, MVC!")
Evolution in the 1980s and 1990s
The Rise of GUI Applications
The 1980s marked a significant period of evolution for MVC as graphical user interfaces became increasingly prevalent. The pattern gained substantial traction with the rise of object-oriented programming languages and frameworks. During this period, several variations of MVC emerged to address specific needs and challenges in different contexts. The introduction of languages like C++ and later Java provided new opportunities to implement MVC in different ways, leading to various interpretations and adaptations of the original pattern.
Here’s an example of MVC implementation in Java from the 1990s style:
import java.util.ArrayList;
import java.util.List;
// Model
class Model {
private String data;
private List<Observer> observers = new ArrayList<>();
public void setData(String data) {
this.data = data;
notifyObservers();
}
public String getData() {
return data;
}
public void addObserver(Observer observer) {
observers.add(observer);
}
private void notifyObservers() {
for (Observer observer : observers) {
observer.update();
}
}
}
// Observer interface
interface Observer {
void update();
}
// View
class View implements Observer {
private Model model;
public View(Model model) {
this.model = model;
model.addObserver(this);
}
@Override
public void update() {
System.out.println("View: Displaying data: " + model.getData());
}
}
// Controller
class Controller {
private Model model;
public Controller(Model model) {
this.model = model;
}
public void handleInput(String input) {
model.setData(input);
}
}
The Web Era and MVC Transformation
Adaptation for Web Applications
The advent of web development in the late 1990s and early 2000s brought new challenges and opportunities for MVC. The pattern needed to adapt to the stateless nature of HTTP and the client-server architecture of web applications. This period saw the emergence of various web-specific MVC frameworks, each offering its own interpretation of how the pattern should be implemented in a web context.
Modern MVC Frameworks
Popular Implementations
The table below shows some of the most influential MVC frameworks and their key characteristics:
Framework | Language | Year Introduced | Key Features |
---|---|---|---|
Django | Python | 2005 | MTV pattern, ORM, Admin interface |
Ruby on Rails | Ruby | 2004 | Convention over configuration, Active Record |
Spring MVC | Java | 2004 | Dependency Injection, AOP support |
ASP.NET MVC | C# | 2009 | Razor view engine, Strong typing |
Laravel | PHP | 2011 | Eloquent ORM, Blade templating |
Here’s a modern Python Django implementation example:
# models.py
from django.db import models
class Article(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
published_date = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
# views.py
from django.views.generic import ListView, DetailView
from .models import Article
class ArticleListView(ListView):
model = Article
template_name = 'articles/article_list.html'
context_object_name = 'articles'
class ArticleDetailView(DetailView):
model = Article
template_name = 'articles/article_detail.html'
context_object_name = 'article'
# urls.py
from django.urls import path
from .views import ArticleListView, ArticleDetailView
urlpatterns = [#91;
path('articles/', ArticleListView.as_view(), name='article-list'),
path('articles/<int:pk>/', ArticleDetailView.as_view(), name='article-detail'),
]#93;
Variations and Alternative Patterns
Modern Interpretations
Over time, several variations of MVC have emerged to address specific needs and challenges:
- MVP (Model-View-Presenter)
- MVVM (Model-View-ViewModel)
- Clean Architecture
- Flux/Redux Architecture
Here’s a simple MVVM example in Python:
class Model:
def __init__(self):
self._data = None
def set_data(self, data):
self._data = data
def get_data(self):
return self._data
class ViewModel:
def __init__(self, model):
self.model = model
self._observers = [#91;]#93;
def set_data(self, data):
self.model.set_data(data)
self._notify_observers()
def get_formatted_data(self):
raw_data = self.model.get_data()
return f"Formatted: {raw_data.upper()}" if raw_data else ""
def add_observer(self, observer):
self._observers.append(observer)
def _notify_observers(self):
for observer in self._observers:
observer.update()
class View:
def __init__(self, view_model):
self.view_model = view_model
self.view_model.add_observer(self)
def update(self):
print(self.view_model.get_formatted_data())
Impact on Modern Software Development
Influence on Contemporary Architecture
The principles established by MVC continue to influence modern software architecture in several ways:
- Separation of Concerns
- Testability
- Maintainability
- Code Organization
- Team Collaboration
Modern frameworks have built upon these principles while adapting to current development needs. The rise of single-page applications (SPAs) and mobile development has led to new interpretations of MVC, demonstrating its flexibility and enduring relevance.
Best Practices and Implementation Guidelines
Modern MVC Implementation
When implementing MVC in modern applications, several best practices should be followed:
- Clear Separation of Responsibilities
- Loose Coupling
- Event-Driven Communication
- Proper State Management
- Consistent Error Handling
Here’s an example of modern MVC implementation with proper separation:
from abc import ABC, abstractmethod
from typing import List, Observer
class ModelInterface(ABC):
@abstractmethod
def get_data(self): pass
@abstractmethod
def set_data(self, data): pass
class ViewInterface(ABC):
@abstractmethod
def update(self): pass
@abstractmethod
def display(self): pass
class ControllerInterface(ABC):
@abstractmethod
def handle_input(self, input_data): pass
class Model(ModelInterface):
def __init__(self):
self._data = None
self._observers: List[#91;Observer]#93; = [#91;]#93;
def get_data(self):
return self._data
def set_data(self, data):
self._data = data
self._notify_observers()
def add_observer(self, observer):
self._observers.append(observer)
def _notify_observers(self):
for observer in self._observers:
observer.update()
class View(ViewInterface):
def __init__(self, model: ModelInterface):
self.model = model
if hasattr(model, 'add_observer'):
model.add_observer(self)
def update(self):
self.display()
def display(self):
data = self.model.get_data()
print(f"View: Displaying formatted data: {data}")
class Controller(ControllerInterface):
def __init__(self, model: ModelInterface):
self.model = model
def handle_input(self, input_data):
# Add validation and processing logic
processed_data = self._process_input(input_data)
self.model.set_data(processed_data)
def _process_input(self, input_data):
# Add input processing logic
return input_data.strip().upper()
Future Trends and Predictions
The future of MVC architecture appears to be evolving alongside emerging technologies and development paradigms. Several trends are shaping its evolution:
- Microservices Architecture Integration
- Serverless Computing Adaptation
- Real-time Application Support
- AI and Machine Learning Integration
- Progressive Web Applications (PWAs)
Conclusion
The journey of MVC from its inception at Xerox PARC to its current state demonstrates its remarkable adaptability and enduring relevance in software development. While the basic principles remain unchanged, the pattern has evolved to meet the changing needs of modern software development. As we look to the future, MVC’s influence continues to shape new architectural patterns and development practices, proving that well-designed patterns can stand the test of time while adapting to new challenges and technologies.
Disclaimer: This article is based on historical research and current industry practices. While every effort has been made to ensure accuracy, software development patterns and their implementations continue to evolve. Please refer to official documentation and current best practices for specific implementation details. If you notice any inaccuracies in this article, please report them so we can maintain the highest standards of technical accuracy.