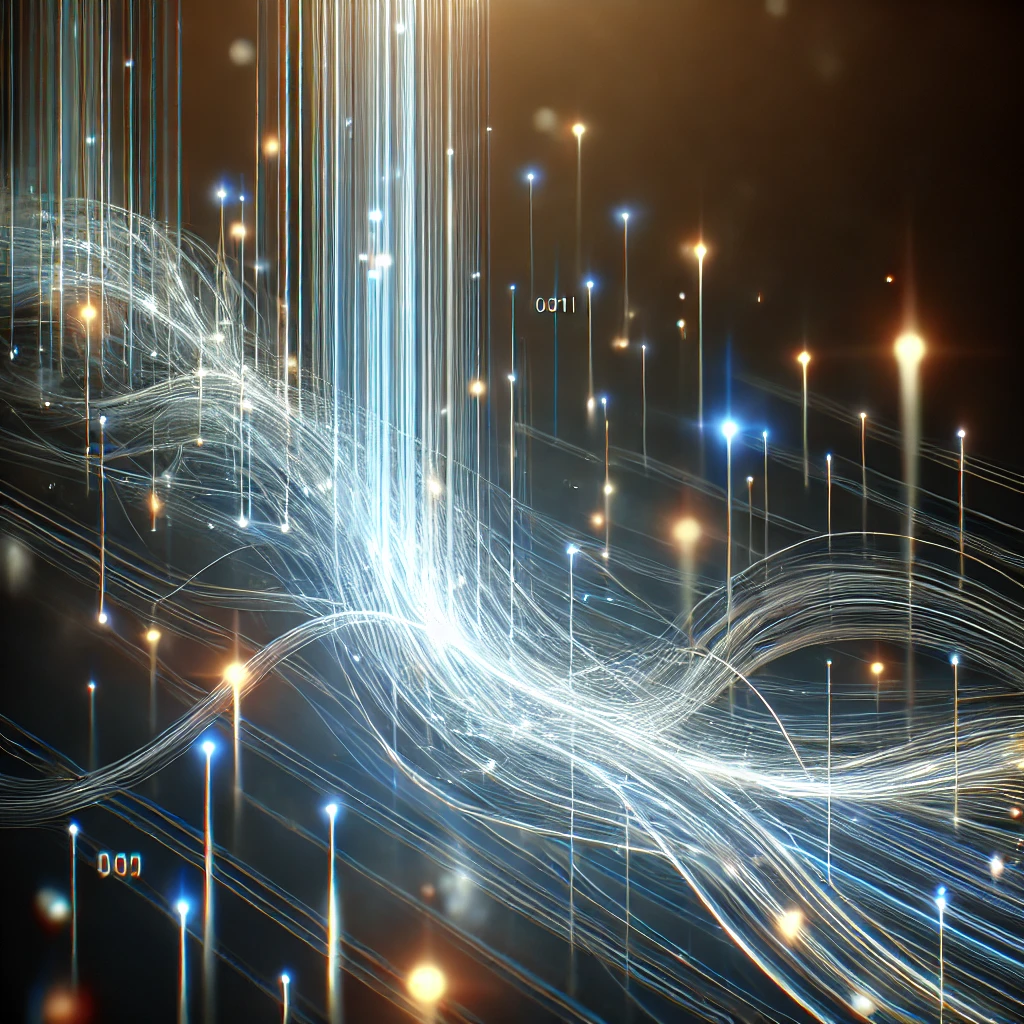
Spring Boot and WebSockets: Building Real-time Applications for Modern Web Development
In today’s fast-paced digital landscape, real-time communication has become an essential requirement for modern web applications. Users expect instant updates, live notifications, and interactive experiences that traditional HTTP request-response patterns struggle to deliver efficiently. Spring Boot, combined with WebSocket technology, provides a robust foundation for building real-time applications that can handle bi-directional communication between clients and servers seamlessly. This comprehensive guide explores the integration of WebSockets with Spring Boot, offering practical insights, implementation strategies, and best practices for developing responsive real-time applications. Whether you’re building a chat application, live dashboard, or collaborative platform, understanding WebSocket implementation in Spring Boot is crucial for delivering exceptional user experiences.
Understanding WebSockets: The Foundation of Real-time Communication
WebSocket protocol represents a significant evolution in web communication, establishing a persistent, full-duplex connection between clients and servers. Unlike traditional HTTP connections, which follow a request-response pattern, WebSockets enable continuous two-way communication over a single TCP connection. This architectural approach dramatically reduces overhead and latency, making it ideal for applications requiring real-time updates. The protocol begins with an HTTP handshake that upgrades to a WebSocket connection, maintaining an open channel for data exchange until either party decides to terminate it. This persistent connection eliminates the need for repeated HTTP requests, resulting in more efficient communication and reduced server load.
Setting Up Spring Boot for WebSocket Integration
Project Configuration
To begin implementing WebSockets in a Spring Boot application, you’ll need to include the necessary dependencies in your project. Here’s the required Maven configuration:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
<dependency>
<groupId>org.webjars</groupId>
<artifactId>webjars-locator-core</artifactId>
</dependency>
<dependency>
<groupId>org.webjars</groupId>
<artifactId>sockjs-client</artifactId>
<version>1.5.1</version>
</dependency>
<dependency>
<groupId>org.webjars</groupId>
<artifactId>stomp-websocket</artifactId>
<version>2.3.4</version>
</dependency>
</dependencies>
WebSocket Configuration Class
After adding the dependencies, you need to create a configuration class to enable WebSocket support:
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic");
config.setApplicationDestinationPrefixes("/app");
}
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/websocket-endpoint")
.setAllowedOrigins("*")
.withSockJS();
}
}
Implementing Message Handling
Message Models
Create data transfer objects (DTOs) to structure your messages:
public class Message {
private String content;
private String sender;
private LocalDateTime timestamp;
// Getters, setters, and constructors
}
public class OutputMessage {
private String content;
private String sender;
private String time;
// Getters, setters, and constructors
}
Controller Implementation
Implement a controller to handle WebSocket messages:
@Controller
public class WebSocketController {
@MessageMapping("/send")
@SendTo("/topic/messages")
public OutputMessage sendMessage(Message message) {
String time = LocalDateTime.now().format(DateTimeFormatter.ofPattern("HH:mm"));
return new OutputMessage(
message.getContent(),
message.getSender(),
time
);
}
}
Building the Client-Side Interface
HTML Structure
<!DOCTYPE html>
<html>
<head>
<title>WebSocket Chat Application</title>
<script src="/webjars/sockjs-client/sockjs.min.js"></script>
<script src="/webjars/stomp-websocket/stomp.min.js"></script>
<script src="/webjars/jquery/jquery.min.js"></script>
</head>
<body>
<div id="chat-container">
<div id="chat-messages"></div>
<input type="text" id="sender" placeholder="Your name">
<input type="text" id="message" placeholder="Type a message...">
<button onclick="sendMessage()">Send</button>
</div>
</body>
</html>
JavaScript Implementation
let stompClient = null;
function connect() {
const socket = new SockJS('/websocket-endpoint');
stompClient = Stomp.over(socket);
stompClient.connect({}, function(frame) {
console.log('Connected: ' + frame);
stompClient.subscribe('/topic/messages', function(response) {
showMessage(JSON.parse(response.body));
});
});
}
function sendMessage() {
const sender = document.getElementById('sender').value;
const content = document.getElementById('message').value;
stompClient.send("/app/send", {}, JSON.stringify({
'sender': sender,
'content': content
}));
}
function showMessage(message) {
const messageContainer = document.getElementById('chat-messages');
const messageElement = document.createElement('div');
messageElement.textContent = `${message.sender} (${message.time}): ${message.content}`;
messageContainer.appendChild(messageElement);
}
// Connect when the page loads
document.addEventListener('DOMContentLoaded', connect);
Security Considerations
When implementing WebSocket communication, security should be a top priority. Spring Security can be integrated with WebSockets to provide authentication and authorization:
@Configuration
@EnableWebSocketSecurity
public class WebSocketSecurityConfig {
@Bean
public SecurityWebSocketServiceRegistration securityWebSocketServiceRegistration() {
return new SecurityWebSocketServiceRegistration()
.addEndpoint("/websocket-endpoint")
.withSockJS()
.setInterceptors(new HttpSessionHandshakeInterceptor());
}
@Bean
public WebSocketMessageBrokerSecurityMetadataSourceRegistry webSocketMessageBrokerSecurityMetadataSourceRegistry() {
return new WebSocketMessageBrokerSecurityMetadataSourceRegistry()
.simpDestMatchers("/topic/**").authenticated()
.anyMessage().authenticated();
}
}
Advanced Features and Optimization
Message Broker Integration
For scalable applications, integrating an external message broker like RabbitMQ can enhance performance:
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void configureMessageBroker(MessageBrokerRegistry registry) {
registry.enableStompBrokerRelay("/topic")
.setRelayHost("localhost")
.setRelayPort(61613)
.setClientLogin("guest")
.setClientPasscode("guest");
registry.setApplicationDestinationPrefixes("/app");
}
}
Connection Management
Implement session handling and connection lifecycle management:
@Component
public class WebSocketEventListener {
private static final Logger logger = LoggerFactory.getLogger(WebSocketEventListener.class);
@EventListener
public void handleWebSocketConnectListener(SessionConnectedEvent event) {
logger.info("Received a new web socket connection");
}
@EventListener
public void handleWebSocketDisconnectListener(SessionDisconnectEvent event) {
StompHeaderAccessor headerAccessor = StompHeaderAccessor.wrap(event.getMessage());
String username = (String) headerAccessor.getSessionAttributes().get("username");
logger.info("User disconnected: " + username);
}
}
Performance Monitoring and Metrics
Spring Boot Actuator can be used to monitor WebSocket connections and performance:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Configure metrics endpoints in application.properties:
management.endpoints.web.exposure.include=*
management.endpoint.metrics.enabled=true
Best Practices and Common Patterns
Category | Best Practice | Description |
---|---|---|
Error Handling | Implement Global Error Handler | Create a centralized error handling mechanism for WebSocket messages |
Connection Management | Handle Reconnection | Implement automatic reconnection on the client side with exponential backoff |
Message Processing | Use Message Queues | Implement message queuing for handling high message volumes |
Security | Enable HTTPS | Always use secure WebSocket connections (wss://) in production |
Performance | Message Batching | Batch messages when possible to reduce network overhead |
Testing WebSocket Applications
Unit Testing
@SpringBootTest
public class WebSocketControllerTest {
private TestStompSessionHandler sessionHandler;
private StompSession stompSession;
@Before
public void setup() {
this.sessionHandler = new TestStompSessionHandler();
WebSocketStompClient stompClient = new WebSocketStompClient(
new SockJsClient(createTransportClient()));
stompClient.connect("ws://localhost:8080/websocket-endpoint",
this.sessionHandler);
this.stompSession = this.sessionHandler.getSession(1, TimeUnit.SECONDS);
}
@Test
public void testMessageBroadcast() throws Exception {
Message testMessage = new Message("Test content", "TestUser");
stompSession.send("/app/send", testMessage);
// Assert message received on subscription
assertNotNull(sessionHandler.getReceivedMessage(1, TimeUnit.SECONDS));
}
}
Troubleshooting and Common Issues
Connection Issues
- Check CORS configuration
- Verify network connectivity
- Ensure proper SSL certificate configuration
- Monitor server logs for connection attempts
Message Handling Problems
- Verify message format
- Check subscription paths
- Monitor message broker status
- Implement proper error handling
Future Considerations and Scaling
When scaling WebSocket applications, consider:
- Load balancing strategies for WebSocket connections
- Message persistence and recovery
- Clustering and high availability
- Monitoring and alerting systems
- Performance optimization techniques
Conclusion
Spring Boot’s WebSocket implementation provides a robust foundation for building real-time applications. Through this comprehensive guide, we’ve explored the essential components, configurations, and best practices necessary for successful WebSocket integration. From basic setup to advanced features and security considerations, the framework offers flexibility and scalability for various use cases. As real-time communication continues to evolve, staying updated with Spring Boot’s WebSocket capabilities and implementing proper security measures remains crucial for developing modern, responsive applications.
Disclaimer: This blog post is intended for educational purposes and represents the author’s understanding of Spring Boot and WebSocket implementation as of the publication date. While we strive for accuracy, technology evolves rapidly, and some information may become outdated. Please consult official Spring documentation for the most up-to-date information. If you notice any inaccuracies or have suggestions for improvement, please report them to our editorial team for prompt correction.