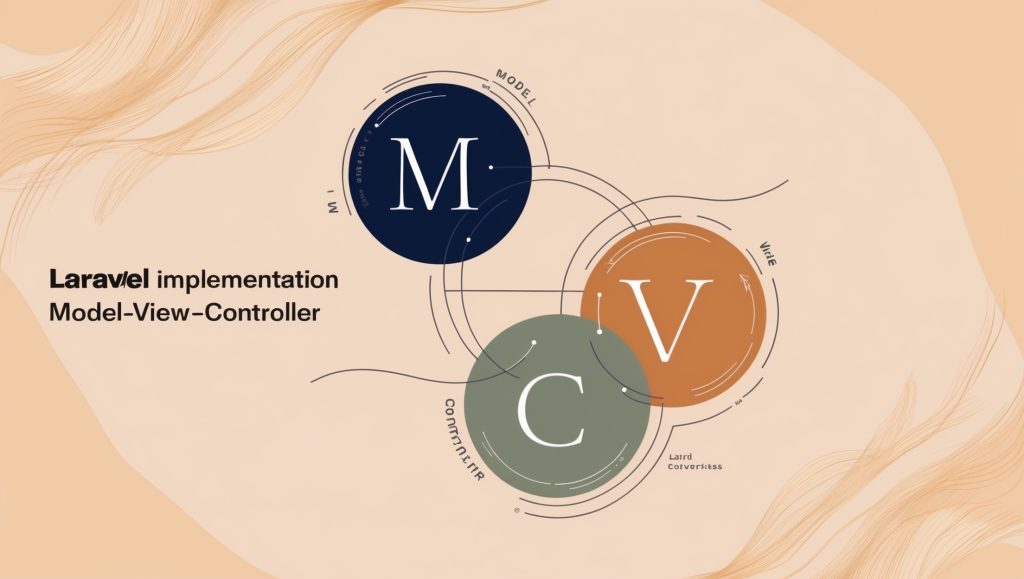
Laravel’s Elegant Implementation of MVC: A Comprehensive Guide
The Model-View-Controller (MVC) architectural pattern has revolutionized web application development by providing a robust structure for organizing code and separating concerns. Laravel, one of the most popular PHP frameworks, has taken this concept to new heights with its elegant implementation of MVC. This comprehensive guide explores Laravel’s sophisticated approach to MVC, showcasing how it enhances developer productivity while maintaining code clarity and maintainability. Through its intuitive conventions, powerful features, and extensive toolset, Laravel has established itself as the go-to framework for building scalable web applications. Whether you’re a seasoned developer or just starting with MVC architecture, understanding Laravel’s implementation will significantly improve your development workflow and code quality.
Understanding Laravel’s MVC Architecture
The Model-View-Controller pattern in Laravel represents a sophisticated implementation that goes beyond the basic MVC concept. Laravel enhances the traditional MVC pattern by introducing additional layers and components that work together seamlessly to create a more robust and maintainable application structure. The framework’s implementation ensures clear separation of concerns while providing powerful tools and conventions that make development more efficient and enjoyable. Laravel’s MVC architecture is designed to handle complex business logic, manage data flow effectively, and present information in a clean and organized manner. The framework’s elegant approach to MVC has made it a preferred choice for developers building everything from simple websites to complex enterprise applications.
Core Components of Laravel’s MVC
- **Models**: Laravel models extend the Eloquent ORM class, providing an intuitive interface for database interactions. Models handle data validation, relationships, and business logic related to data manipulation. Each model typically corresponds to a database table and includes methods for accessing and manipulating that data.
- **Views**: Views in Laravel utilize the Blade templating engine, offering powerful features like template inheritance, components, and slots. Views are responsible for presenting data to users in a formatted way, supporting dynamic content rendering and reusable UI components.
- **Controllers**: Controllers act as the orchestrators of the application, processing requests, coordinating with models, and returning appropriate responses. Laravel controllers can be resource controllers, single-action controllers, or custom controllers based on specific needs.
The Power of Eloquent ORM in Models
Laravel’s Eloquent ORM is a sophisticated ActiveRecord implementation that makes database interactions intuitive and powerful. Unlike traditional ORMs, Eloquent provides an expressive and elegant syntax for working with your database. Each database table has a corresponding Model that is used to interact with that table, providing an object-oriented approach to data manipulation. Eloquent handles complex relationships effortlessly, supports eager loading to prevent N+1 query problems, and includes powerful features like model events, global scopes, and automatic timestamps. The ORM’s fluent interface makes database operations feel natural and reduces the amount of code needed to perform common tasks.
Example of an Eloquent Model:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Product extends Model
{
use SoftDeletes;
protected $fillable = [
'name',
'description',
'price',
'category_id'
];
protected $casts = [
'price' => 'decimal:2',
'is_active' => 'boolean'
];
public function category()
{
return $this->belongsTo(Category::class);
}
public function orders()
{
return $this->belongsToMany(Order::class)
->withPivot('quantity', 'price')
->withTimestamps();
}
public function scopeActive($query)
{
return $query->where('is_active', true);
}
}
Blade Templates: Laravel’s Powerful View Engine
Laravel’s Blade templating engine provides an expressive and elegant syntax that makes working with views a delightful experience. Blade combines the power of PHP with convenient shortcuts and additional features that streamline view creation and maintenance. The engine includes features like template inheritance, components, slots, and directives that help create modular and reusable view components. Blade’s approach to template compilation ensures optimal performance while maintaining developer productivity. The ability to extend Blade with custom directives and components allows teams to create their own abstractions and maintain consistency across projects.
Example of a Blade Template:
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html>
<head>
<title>@yield('title') - My Application</title>
@stack('styles')
</head>
<body>
@include('partials.navigation')
<div class="container">
@yield('content')
</div>
@stack('scripts')
</body>
</html>
<!-- resources/views/products/show.blade.php -->
@extends('layouts.app')
@section('title', 'Product Details')
@section('content')
<x-product-card :product="$product">
<x-slot name="footer">
@if($product->isInStock())
<button class="btn-primary">Add to Cart</button>
@else
<span class="text-red">Out of Stock</span>
@endif
</x-slot>
</x-product-card>
@endsection
Controllers: Orchestrating Application Logic
Laravel controllers serve as the central orchestrators of application logic, handling HTTP requests and coordinating responses. Controllers in Laravel can be organized in various ways to suit different application needs, from resource controllers that follow RESTful conventions to single-action controllers for specific use cases. Laravel’s controller implementation promotes clean code organization while providing powerful features like middleware, form request validation, and dependency injection. Controllers can be grouped and nested to handle complex routing scenarios, and they can utilize Laravel’s service container for managing dependencies.
Example of a Resource Controller:
<?php
namespace App\Http\Controllers;
use App\Models\Product;
use App\Http\Requests\StoreProductRequest;
use App\Http\Resources\ProductResource;
use Illuminate\Http\Request;
class ProductController extends Controller
{
public function __construct()
{
$this->middleware('auth')->except(['index', 'show']);
}
public function index()
{
$products = Product::active()
->with('category')
->paginate(20);
return ProductResource::collection($products);
}
public function store(StoreProductRequest $request)
{
$product = Product::create($request->validated());
event(new ProductCreated($product));
return new ProductResource($product);
}
public function show(Product $product)
{
return new ProductResource($product->load('category'));
}
public function update(StoreProductRequest $request, Product $product)
{
$product->update($request->validated());
return new ProductResource($product);
}
public function destroy(Product $product)
{
$product->delete();
return response()->noContent();
}
}
Laravel’s Service Container and Dependency Injection
Laravel’s service container is a powerful tool for managing class dependencies and performing dependency injection. The container is central to many of Laravel’s features and provides an elegant way to resolve and inject dependencies throughout your application. Understanding the service container is crucial for building maintainable and testable applications in Laravel. The framework’s implementation of dependency injection promotes loose coupling between components and makes testing easier by allowing dependencies to be mocked or substituted. The service container can automatically resolve dependencies based on type-hints and supports binding interfaces to concrete implementations.
Example of Service Container Usage:
<?php
namespace App\Services;
use App\Contracts\PaymentGateway;
use App\Contracts\OrderRepository;
class OrderService
{
protected $paymentGateway;
protected $orderRepository;
public function __construct(
PaymentGateway $paymentGateway,
OrderRepository $orderRepository
) {
$this->paymentGateway = $paymentGateway;
$this->orderRepository = $orderRepository;
}
public function processOrder(array $orderData)
{
$order = $this->orderRepository->create($orderData);
$payment = $this->paymentGateway->process([
'amount' => $order->total,
'currency' => 'USD',
'order_id' => $order->id
]);
return $order->update(['payment_id' => $payment->id]);
}
}
Request Lifecycle and Middleware
Laravel’s request lifecycle provides a clear and organized flow for handling HTTP requests, from initial routing to final response. Middleware serves as a powerful mechanism for filtering HTTP requests entering your application. Laravel’s middleware implementation allows for both global middleware that runs on every request and route-specific middleware that can be applied selectively. The framework includes built-in middleware for common tasks like authentication, CSRF protection, and API rate limiting, while also making it easy to create custom middleware for specific application needs.
Example of Custom Middleware:
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class EnsureUserHasRole
{
public function handle(Request $request, Closure $next, string $role)
{
if (!$request->user() || !$request->user()->hasRole($role)) {
return response()->json([
'message' => 'Unauthorized access'
], 403);
}
return $next($request);
}
}
Database Migrations and Seeding
Laravel’s database migrations provide a version control system for your database schema, allowing teams to define and share database changes. The migration system, combined with database seeding, creates a powerful toolset for managing database structure and initial data. Migrations can be created, modified, and rolled back easily, making database schema changes more manageable and trackable. Laravel’s seeding feature allows for the creation of test data using model factories, making it easier to develop and test applications with realistic data.
Example of Migration and Seeder:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateProductsTable extends Migration
{
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->decimal('price', 10, 2);
$table->foreignId('category_id')
->constrained()
->onDelete('cascade');
$table->boolean('is_active')->default(true);
$table->timestamps();
$table->softDeletes();
});
}
public function down()
{
Schema::dropIfExists('products');
}
}
// Database Seeder
class ProductSeeder extends Seeder
{
public function run()
{
Product::factory()
->count(50)
->create();
}
}
Testing in Laravel
Laravel provides a comprehensive testing suite that makes it easy to write PHPUnit tests for your applications. The framework includes convenient helper methods and assertions specifically designed for testing HTTP requests, database operations, and authentication. Laravel’s testing features support both feature tests for testing entire features and unit tests for testing individual components. The framework’s elegant approach to testing makes it easier to maintain high test coverage and ensure application reliability.
Example of Feature Test:
<?php
namespace Tests\Feature;
use Tests\TestCase;
use App\Models\User;
use App\Models\Product;
use Illuminate\Foundation\Testing\RefreshDatabase;
class ProductManagementTest extends TestCase
{
use RefreshDatabase;
public function test_authorized_user_can_create_product()
{
$user = User::factory()->create();
$productData = Product::factory()->make()->toArray();
$response = $this->actingAs($user)
->postJson('/api/products', $productData);
$response->assertStatus(201)
->assertJsonStructure([
'data' => [
'id',
'name',
'description',
'price',
'created_at'
]
]);
$this->assertDatabaseHas('products', [
'name' => $productData['name']
]);
}
}
Best Practices and Common Patterns
When working with Laravel’s MVC implementation, following established best practices and common patterns can significantly improve code quality and maintainability. These include using repository patterns for data access, implementing service layers for complex business logic, utilizing form requests for validation, and leveraging Laravel’s built-in features for common tasks. Understanding and following these patterns helps create more organized, testable, and scalable applications. Laravel’s ecosystem provides many tools and packages that complement these patterns and make it easier to follow best practices.
Conclusion
Laravel’s implementation of the MVC pattern represents a thoughtful and elegant approach to web application architecture. Through its powerful features, intuitive conventions, and extensive toolset, Laravel makes it easier to build and maintain complex applications while following best practices. The framework’s attention to developer experience, combined with its robust architecture, has created an ecosystem that continues to evolve and improve. Whether you’re building a simple website or a complex enterprise application, Laravel’s MVC implementation provides the structure and tools needed to create successful projects.
Disclaimer: This blog post represents our current understanding of Laravel’s MVC implementation based on the latest stable version available at the time of writing. While we strive for accuracy, Laravel is actively developed, and features or best practices may change. Please consult the official Laravel documentation for the most up-to-date information. If you notice any inaccuracies in this post, please report them to our editorial team at [contact@example.com], and we will update the content promptly.