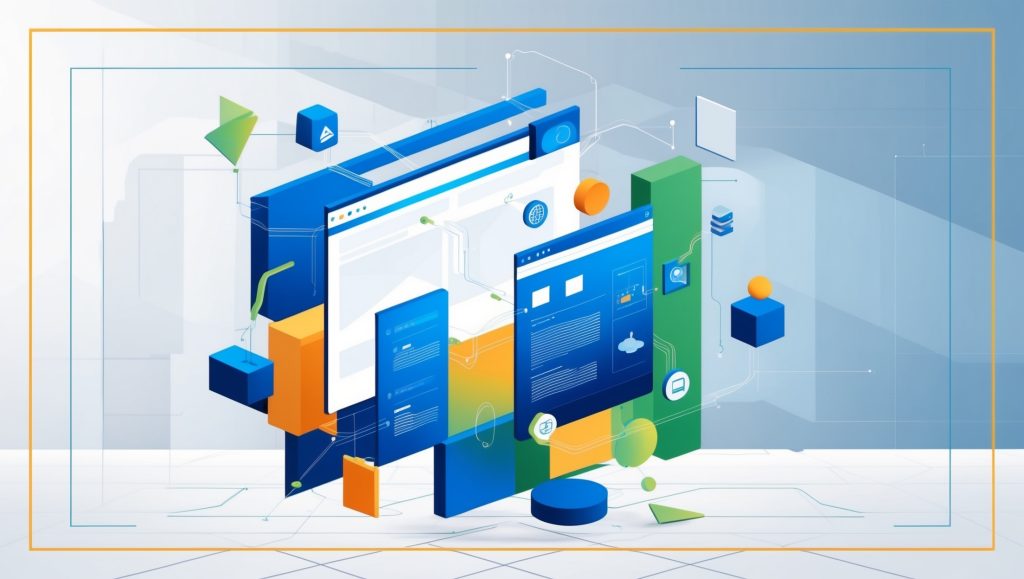
Understanding MVC Architecture and Modern Front-End Framework Integration
The landscape of web development has evolved dramatically over the past decade, with the emergence of sophisticated front-end frameworks and the maturation of MVC (Model-View-Controller) architecture. Modern web applications demand a seamless integration between robust backend systems and responsive front-end interfaces. This comprehensive guide explores the synergy between traditional MVC architecture and popular front-end frameworks like React, Angular, and Vue.js, providing practical insights into their integration strategies.
Understanding MVC Architecture
Core Components of MVC
The Model-View-Controller pattern separates application logic into three distinct components, each serving a specific purpose in the application’s architecture. The Model handles data and business logic, the View manages presentation and user interface elements, and the Controller processes user input and manages communication between the Model and View. This separation of concerns promotes maintainable, scalable, and testable code.
Here’s a basic example of MVC implementation in Python using Flask:
# Model
class User:
def __init__(self, name, email):
self.name = name
self.email = email
@staticmethod
def get_user_by_id(user_id):
# Database interaction logic
return User("John Doe", "john@example.com")
# Controller
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/user/<int:user_id>')
def get_user(user_id):
user = User.get_user_by_id(user_id)
return jsonify({
'name': user.name,
'email': user.email
})
# View (in this case, returning JSON for front-end consumption)
And here’s the equivalent in Java using Spring:
// Model
@Entity
public class User {
@Id
private Long id;
private String name;
private String email;
// Getters and setters
}
// Controller
@RestController
@RequestMapping("/api/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.getUserById(id);
return ResponseEntity.ok(user);
}
}
Modern Front-End Frameworks Overview
React.js
React has revolutionized front-end development with its component-based architecture and virtual DOM implementation. Its unidirectional data flow and JSX syntax provide a powerful foundation for building dynamic user interfaces. React’s ecosystem, including Redux for state management and React Router for navigation, makes it a comprehensive solution for modern web applications.
Example of a React component consuming the MVC backend:
import React, { useState, useEffect } from 'react';
const UserProfile = ({ userId }) => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchUser = async () => {
try {
const response = await fetch(`/api/user/${userId}`);
const data = await response.json();
setUser(data);
setLoading(false);
} catch (error) {
console.error('Error fetching user:', error);
setLoading(false);
}
};
fetchUser();
}, [userId]);
if (loading) return <div>Loading...</div>;
if (!user) return <div>User not found</div>;
return (
<div className="user-profile">
<h2>{user.name}</h2>
<p>{user.email}</p>
</div>
);
};
export default UserProfile;
Angular Framework Integration
Architecture and Features
Angular provides a comprehensive framework with built-in features like dependency injection, TypeScript support, and powerful templating capabilities. Its integration with MVC backends requires careful consideration of data flow and state management patterns. Here’s a comparison of key features:
Feature | Angular | Traditional MVC |
---|---|---|
Data Binding | Two-way | One-way |
Templating | Angular Templates | Server-side Templates |
Routing | Client-side | Server-side |
State Management | Services/RxJS | Server State |
Example of an Angular service interacting with MVC backend:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class UserService {
private apiUrl = '/api/user';
constructor(private http: HttpClient) {}
getUser(id: number): Observable<any> {
return this.http.get(`${this.apiUrl}/${id}`);
}
}
// Component using the service
@Component({
selector: 'app-user-profile',
template: `
<div *ngIf="user$ | async as user">
<h2>{{user.name}}</h2>
<p>{{user.email}}</p>
</div>
`
})
export class UserProfileComponent {
user$ = this.userService.getUser(1);
constructor(private userService: UserService) {}
}
Vue.js Integration Strategies
Vue.js Architecture
Vue.js combines the best aspects of Angular and React, offering a progressive framework that can be adopted incrementally. Its integration with MVC backends is streamlined through Vuex for state management and Vue Router for navigation. The framework’s reactivity system and component composition API provide flexible options for managing application state and UI updates.
Example of a Vue.js component with Vuex store:
// Vuex store
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export const store = new Vuex.Store({
state: {
user: null
},
mutations: {
setUser(state, user) {
state.user = user;
}
},
actions: {
async fetchUser({ commit }, userId) {
try {
const response = await fetch(`/api/user/${userId}`);
const user = await response.json();
commit('setUser', user);
} catch (error) {
console.error('Error fetching user:', error);
}
}
}
});
// Vue component
<template>
<div v-if="user">
<h2>{{ user.name }}</h2>
<p>{{ user.email }}</p>
</div>
</template>
<script>
export default {
name: 'UserProfile',
computed: {
user() {
return this.$store.state.user;
}
},
created() {
this.$store.dispatch('fetchUser', this.$route.params.id);
}
}
</script>
Best Practices for Integration
API Design and Communication
When integrating MVC backends with modern front-end frameworks, following RESTful principles and implementing proper error handling are crucial. Here’s a comprehensive example of a Python Flask backend with proper error handling:
from flask import Flask, jsonify, request
from flask_cors import CORS
from werkzeug.exceptions import NotFound
app = Flask(__name__)
CORS(app)
class APIError(Exception):
def __init__(self, message, status_code):
self.message = message
self.status_code = status_code
@app.errorhandler(APIError)
def handle_api_error(error):
response = jsonify({'error': error.message})
response.status_code = error.status_code
return response
@app.route('/api/user/<int:user_id>')
def get_user(user_id):
try:
user = User.get_user_by_id(user_id)
if not user:
raise APIError('User not found', 404)
return jsonify(user.to_dict())
except Exception as e:
raise APIError(str(e), 500)
Security Considerations
Cross-Origin Resource Sharing (CORS)
Security is paramount when integrating front-end frameworks with MVC backends. Implementing proper CORS policies and authentication mechanisms is essential. Here’s an example of implementing JWT authentication in a Spring Boot backend:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/auth/**").permitAll()
.anyRequest().authenticated()
.and()
.addFilter(new JWTAuthenticationFilter(authenticationManager()))
.addFilter(new JWTAuthorizationFilter(authenticationManager()));
}
@Bean
CorsConfigurationSource corsConfigurationSource() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("http://localhost:3000"));
configuration.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "DELETE"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/api/**", configuration);
return source;
}
}
Performance Optimization
Caching and State Management
Optimizing performance requires careful consideration of caching strategies and efficient state management. Here’s an example of implementing caching in a React application using a custom hook:
import { useState, useEffect } from 'react';
const useCache = (key, fetchData) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const cached = localStorage.getItem(key);
if (cached) {
setData(JSON.parse(cached));
setLoading(false);
} else {
fetchData().then(result => {
localStorage.setItem(key, JSON.stringify(result));
setData(result);
setLoading(false);
});
}
}, [key, fetchData]);
return { data, loading };
};
Testing Strategies
Integration Testing
Comprehensive testing ensures reliable integration between front-end and backend components. Here’s an example of integration testing using Jest and React Testing Library:
import { render, screen, waitFor } from '@testing-library/react';
import UserProfile from './UserProfile';
describe('UserProfile Integration', () => {
beforeEach(() => {
fetch.resetMocks();
});
it('successfully loads and displays user data', async () => {
const mockUser = { name: 'John Doe', email: 'john@example.com' };
fetch.mockResponseOnce(JSON.stringify(mockUser));
render(<UserProfile userId={1} />);
expect(screen.getByText('Loading...')).toBeInTheDocument();
await waitFor(() => {
expect(screen.getByText(mockUser.name)).toBeInTheDocument();
expect(screen.getByText(mockUser.email)).toBeInTheDocument();
});
});
});
Future Trends and Considerations
Micro-Frontend Architecture
The evolution of web development continues with micro-frontend architecture, allowing teams to build and deploy front-end components independently. This approach requires careful consideration of integration patterns and state management strategies across multiple front-end frameworks.
Example of a micro-frontend setup using Module Federation:
// webpack.config.js
const ModuleFederationPlugin = require('webpack/lib/container/ModuleFederationPlugin');
module.exports = {
plugins: [
new ModuleFederationPlugin({
name: 'userProfile',
filename: 'remoteEntry.js',
exposes: {
'./UserProfile': './src/components/UserProfile'
},
shared: ['react', 'react-dom']
})
]
};
Conclusion
The integration of MVC backends with modern front-end frameworks represents a crucial aspect of contemporary web development. By following best practices, implementing proper security measures, and optimizing performance, developers can create robust, scalable applications that leverage the strengths of both architectural patterns. The continuous evolution of web technologies demands ongoing adaptation and learning, but the fundamental principles of clean architecture and separation of concerns remain constant.
Disclaimer: This blog post represents current best practices and implementations as of November 2024. Technologies and frameworks evolve rapidly, and some information may become outdated. Please verify all code examples in your specific context and consult official documentation for the most up-to-date information. If you notice any inaccuracies or have suggestions for improvements, please report them to our editorial team.