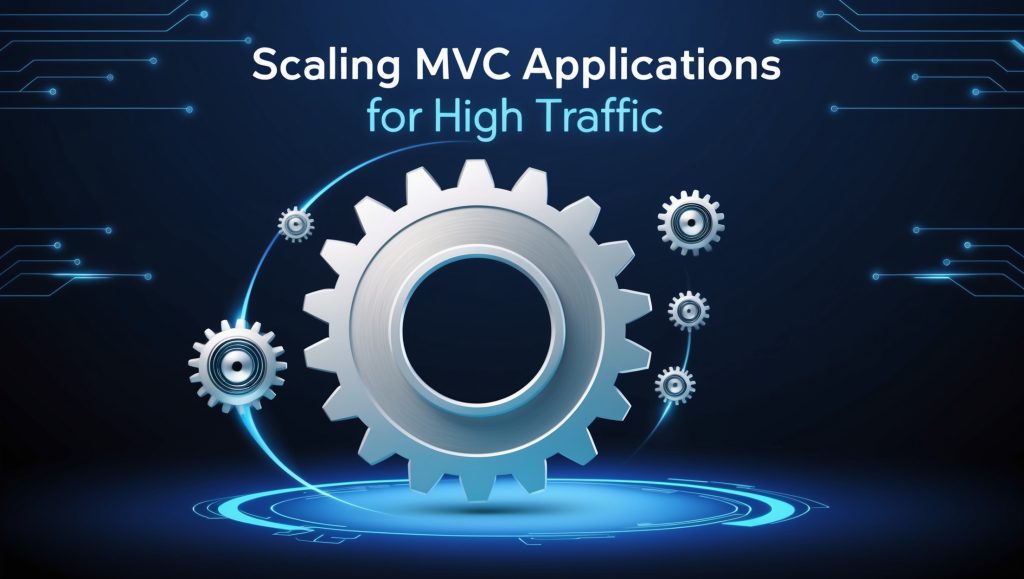
Scaling MVC Applications for High Traffic
Modern web applications face unprecedented challenges in managing high traffic loads while maintaining optimal performance and user experience. As businesses grow and user bases expand, the traditional Model-View-Controller (MVC) architecture must evolve to handle increased demands efficiently. This comprehensive guide explores proven strategies, best practices, and implementation techniques for scaling MVC applications effectively. We’ll delve into both theoretical concepts and practical solutions, supported by real-world examples in Python and Java, to help you build robust, scalable applications that can handle millions of concurrent users while maintaining responsiveness and reliability.
Understanding Scalability Challenges in MVC Applications
The MVC architectural pattern, while excellent for organizing code and separating concerns, can face significant challenges when dealing with high traffic loads. Traditional MVC applications often encounter bottlenecks in database operations, session management, and request handling when user numbers surge. Understanding these challenges is crucial for implementing effective scaling strategies. Common issues include database connection pooling limitations, memory constraints in session management, and increased response times due to complex view rendering processes. These challenges are further compounded by the need to maintain data consistency across multiple servers and ensure seamless user experiences despite growing system complexity.
Horizontal vs. Vertical Scaling Strategies
Vertical Scaling (Scaling Up)
Vertical scaling involves adding more resources (CPU, RAM, Storage) to existing servers. While this approach is straightforward, it has limitations in terms of hardware capabilities and cost-effectiveness. Here’s a practical Java example of utilizing increased resources through optimized thread pooling:
@Configuration
public class ThreadPoolConfig {
@Bean
public Executor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(20);
executor.setQueueCapacity(500);
executor.setThreadNamePrefix("AsyncThread-");
executor.initialize();
return executor;
}
}
Horizontal Scaling (Scaling Out)
Horizontal scaling involves adding more server instances to distribute the load. This approach offers better flexibility and reliability. Here’s a Python example using Flask and Gunicorn for horizontal scaling:
bind = "0.0.0.0:8000"
workers = multiprocessing.cpu_count() * 2 + 1
worker_class = "gevent"
worker_connections = 1000
timeout = 30
keepalive = 2
Implementing Caching Strategies
Application-Level Caching
Implementing effective caching mechanisms is crucial for reducing database load and improving response times. Here’s an example using Redis with Python:
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
cache = Cache(app, config={
'CACHE_TYPE': 'redis',
'CACHE_REDIS_URL': 'redis://localhost:6379/0'
})
@app.route('/user/<user_id>')
@cache.memoize(timeout=300) # Cache for 5 minutes
def get_user(user_id):
return User.query.get(user_id)
Distributed Caching
For multi-server setups, implementing distributed caching ensures consistency across instances. Here’s a Java example using Hazelcast:
@Configuration
public class CacheConfig {
@Bean
public Config hazelcastConfig() {
Config config = new Config();
config.setInstanceName("hazelcast-instance")
.addMapConfig(
new MapConfig()
.setName("userCache")
.setEvictionConfig(
new EvictionConfig()
.setSize(10000)
.setMaxSizePolicy(MaxSizePolicy.PER_NODE)
)
);
return config;
}
}
Database Optimization and Sharding
Database Connection Pooling
Efficient database connection management is essential for handling high traffic. Here’s a Python example using SQLAlchemy:
from sqlalchemy import create_engine
from sqlalchemy.pool import QueuePool
engine = create_engine('postgresql://user:password@localhost/dbname',
poolclass=QueuePool,
pool_size=20,
max_overflow=10,
pool_timeout=30)
Database Sharding Strategies
Sharding Method | Best Use Case | Complexity | Scalability |
---|---|---|---|
Hash-based | Uniform data distribution | Medium | High |
Range-based | Time-series data | Low | Medium |
Geo-based | Location-specific data | High | Very High |
Here’s a Java implementation of a simple sharding strategy:
@Service
public class ShardingService {
private final List<DataSource> shards;
public DataSource getShardForUser(Long userId) {
int shardIndex = (int) (userId % shards.size());
return shards.get(shardIndex);
}
@Transactional
public User saveUser(User user) {
DataSource shard = getShardForUser(user.getId());
JdbcTemplate jdbcTemplate = new JdbcTemplate(shard);
// Perform save operation
return user;
}
}
Load Balancing and Service Discovery
Implementation of Load Balancing
Load balancing is crucial for distributing traffic across multiple server instances. Here’s a Python example using Nginx configuration:
http {
upstream backend {
least_conn; # Least connections algorithm
server backend1.example.com:8000;
server backend2.example.com:8000;
server backend3.example.com:8000;
}
server {
listen 80;
location / {
proxy_pass http://backend;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
}
Service Discovery Implementation
Implementing service discovery helps manage dynamic infrastructure. Here’s a Java example using Spring Cloud Netflix Eureka:
@SpringBootApplication
@EnableEurekaServer
public class ServiceRegistryApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceRegistryApplication.class, args);
}
}
// Client Configuration
@SpringBootApplication
@EnableDiscoveryClient
public class ServiceApplication {
@LoadBalanced
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
Asynchronous Processing and Message Queues
Implementing Message Queues
Message queues help handle heavy workloads asynchronously. Here’s a Python example using RabbitMQ:
import pika
from flask import Flask
app = Flask(__name__)
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
@app.route('/process-task')
def process_task():
channel.queue_declare(queue='task_queue', durable=True)
channel.basic_publish(
exchange='',
routing_key='task_queue',
body='Task data',
properties=pika.BasicProperties(delivery_mode=2)
)
return {"status": "Task queued"}
Background Job Processing
Here’s a Java implementation using Spring Batch for processing large datasets:
@Configuration
@EnableBatchProcessing
public class BatchConfig {
@Bean
public Job processUserDataJob(JobBuilderFactory jobBuilderFactory,
Step step1) {
return jobBuilderFactory.get("processUserDataJob")
.incrementer(new RunIdIncrementer())
.flow(step1)
.end()
.build();
}
@Bean
public Step step1(StepBuilderFactory stepBuilderFactory,
ItemReader<User> reader,
ItemProcessor<User, User> processor,
ItemWriter<User> writer) {
return stepBuilderFactory.get("step1")
.<User, User>chunk(10)
.reader(reader)
.processor(processor)
.writer(writer)
.build();
}
}
Monitoring and Performance Optimization
Implementing Monitoring Systems
Effective monitoring is crucial for maintaining application performance. Here’s a Python example using Prometheus metrics:
from prometheus_client import Counter, Histogram
from flask import Flask, request
import time
app = Flask(__name__)
REQUEST_COUNT = Counter('request_count', 'App Request Count',
['method', 'endpoint', 'http_status'])
REQUEST_LATENCY = Histogram('request_latency_seconds',
'Request latency in seconds')
@app.before_request
def before_request():
request.start_time = time.time()
@app.after_request
def after_request(response):
REQUEST_COUNT.labels(request.method,
request.endpoint,
response.status_code).inc()
REQUEST_LATENCY.observe(time.time() - request.start_time)
return response
Performance Metrics Table
Metric | Warning Threshold | Critical Threshold | Action Required |
---|---|---|---|
Response Time | > 2 seconds | > 5 seconds | Scale horizontally |
CPU Usage | > 70% | > 90% | Investigate bottlenecks |
Memory Usage | > 80% | > 95% | Increase capacity |
Error Rate | > 1% | > 5% | Debug and fix issues |
Security Considerations in Scaled Applications
Implementing Rate Limiting
Protect your scaled application from abuse with rate limiting. Here’s a Java example using Spring Security:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Bean
public RateLimiter rateLimiter() {
return RateLimiter.create(100.0); // 100 requests per second
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.addFilterBefore(new RateLimitFilter(rateLimiter()),
UsernamePasswordAuthenticationFilter.class);
}
}
public class RateLimitFilter extends OncePerRequestFilter {
private final RateLimiter rateLimiter;
@Override
protected void doFilterInternal(HttpServletRequest request,
HttpServletResponse response,
FilterChain filterChain)
throws ServletException, IOException {
if (!rateLimiter.tryAcquire()) {
response.setStatus(HttpStatus.TOO_MANY_REQUESTS.value());
return;
}
filterChain.doFilter(request, response);
}
}
Conclusion
Scaling MVC applications for high traffic requires a multi-faceted approach combining various strategies and technologies. Success depends on choosing the right combination of solutions based on your specific requirements and constraints. Regular monitoring, continuous optimization, and maintaining a balance between performance and complexity are key to building and maintaining scalable applications. Remember that scaling is an iterative process – start with the basics and gradually implement more sophisticated solutions as your application grows.
Disclaimer: The code examples and strategies presented in this blog post are based on current best practices and common implementation patterns. However, technology evolves rapidly, and some approaches may need adaptation for specific use cases. Always test thoroughly in your environment and consult official documentation for the most up-to-date information. Please report any inaccuracies or outdated information to our editorial team for prompt correction.