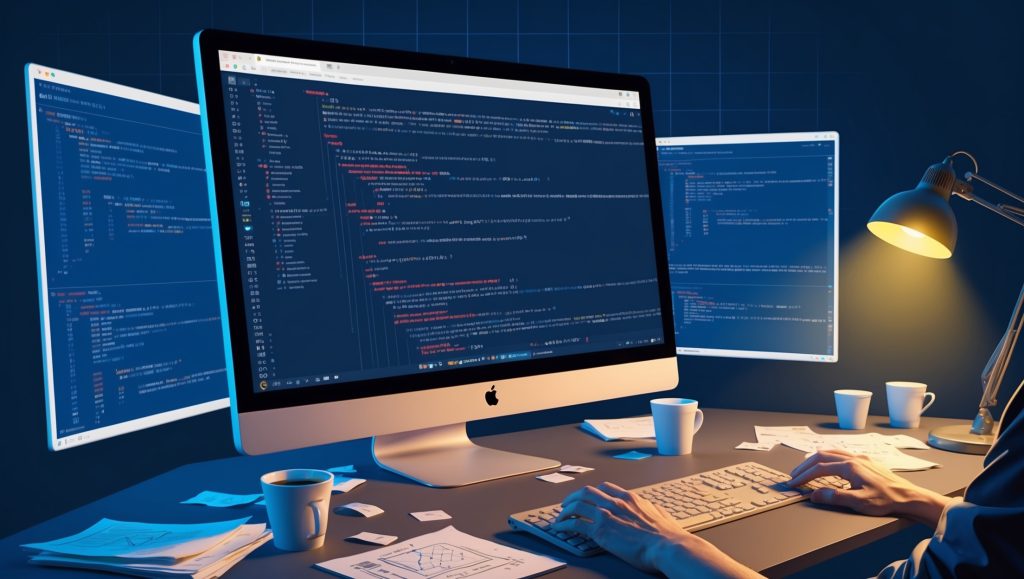
Error Handling and Logging in MVC
In modern web applications, robust error handling and comprehensive logging are not just best practices – they’re essential components for maintaining reliable, maintainable, and secure systems. The Model-View-Controller (MVC) architectural pattern, while providing a clear separation of concerns, introduces its own set of challenges when it comes to managing errors and maintaining detailed logs for debugging purposes. This comprehensive guide explores the intricacies of implementing effective error handling and logging mechanisms in MVC applications, providing practical examples in both Python and Java. By following these practices, developers can create more resilient applications that not only handle errors gracefully but also provide valuable insights for troubleshooting and maintenance.
Understanding Error Handling in MVC
The MVC pattern divides an application into three distinct components, each requiring specific approaches to error handling. Understanding how errors propagate through these layers is crucial for implementing effective error management strategies. In an MVC application, errors can occur at various levels: during data validation in the Model, during business logic processing in the Controller, or during view rendering in the View layer. A well-designed error handling system must account for all these scenarios while maintaining the separation of concerns that makes MVC so valuable.
Implementing Global Exception Handling
Setting Up Exception Handlers
A global exception handling mechanism serves as the last line of defense in your application, catching any unhandled exceptions that might otherwise crash your application. Here’s how to implement global exception handlers in both Python and Java:
Python (Django) Example:
# middleware.py
from django.http import JsonResponse
from django.core.exceptions import ValidationError
import logging
logger = logging.getLogger(__name__)
class GlobalExceptionMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
try:
response = self.get_response(request)
return response
except ValidationError as e:
logger.error(f"Validation error: {str(e)}")
return JsonResponse({
'status': 'error',
'message': 'Validation failed',
'errors': e.message_dict
}, status=400)
except Exception as e:
logger.error(f"Unexpected error: {str(e)}", exc_info=True)
return JsonResponse({
'status': 'error',
'message': 'An unexpected error occurred'
}, status=500)
Java (Spring) Example:
@ControllerAdvice
public class GlobalExceptionHandler extends ResponseEntityExceptionHandler {
private static final Logger logger = LoggerFactory.getLogger(GlobalExceptionHandler.class);
@ExceptionHandler(ValidationException.class)
public ResponseEntity<ErrorResponse> handleValidationException(ValidationException ex) {
logger.error("Validation error occurred: ", ex);
ErrorResponse error = new ErrorResponse(
HttpStatus.BAD_REQUEST.value(),
"Validation Error",
ex.getMessage()
);
return new ResponseEntity<>(error, HttpStatus.BAD_REQUEST);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleGlobalException(Exception ex) {
logger.error("Unexpected error occurred: ", ex);
ErrorResponse error = new ErrorResponse(
HttpStatus.INTERNAL_SERVER_ERROR.value(),
"Internal Server Error",
"An unexpected error occurred"
);
return new ResponseEntity<>(error, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Custom Exception Classes
Creating Domain-Specific Exceptions
Custom exception classes help in categorizing errors and providing more meaningful error messages. They also make it easier to handle specific error conditions differently. Here’s how to implement custom exceptions:
Python Example:
class BusinessLogicException(Exception):
def __init__(self, message, error_code=None):
self.message = message
self.error_code = error_code
super().__init__(self.message)
class ResourceNotFoundException(BusinessLogicException):
def __init__(self, resource_name, resource_id):
message = f"{resource_name} with id {resource_id} not found"
super().__init__(message, "RESOURCE_NOT_FOUND")
class ValidationException(BusinessLogicException):
def __init__(self, validation_errors):
message = "Validation failed"
self.validation_errors = validation_errors
super().__init__(message, "VALIDATION_FAILED")
Java Example:
public class BusinessLogicException extends RuntimeException {
private final String errorCode;
public BusinessLogicException(String message, String errorCode) {
super(message);
this.errorCode = errorCode;
}
public String getErrorCode() {
return errorCode;
}
}
public class ResourceNotFoundException extends BusinessLogicException {
public ResourceNotFoundException(String resourceName, Long resourceId) {
super(
String.format("%s with id %d not found", resourceName, resourceId),
"RESOURCE_NOT_FOUND"
);
}
}
Implementing Logging Strategies
Configuring Logging Systems
A well-configured logging system is crucial for debugging and monitoring applications. Here’s how to set up comprehensive logging in both Python and Java:
Python Example (using Python’s built-in logging):
# logging_config.py
import logging
import logging.handlers
import os
def setup_logging():
# Create logs directory if it doesn't exist
if not os.path.exists('logs'):
os.makedirs('logs')
# Configure root logger
logger = logging.getLogger()
logger.setLevel(logging.INFO)
# Console handler
console_handler = logging.StreamHandler()
console_handler.setLevel(logging.INFO)
console_formatter = logging.Formatter(
'%(asctime)s - %(name)s - %(levelname)s - %(message)s'
)
console_handler.setFormatter(console_formatter)
# File handler
file_handler = logging.handlers.RotatingFileHandler(
'logs/app.log',
maxBytes=1024 * 1024, # 1MB
backupCount=5
)
file_handler.setLevel(logging.DEBUG)
file_formatter = logging.Formatter(
'%(asctime)s - %(name)s - %(levelname)s - %(message)s'
)
file_handler.setFormatter(file_formatter)
# Add handlers to root logger
logger.addHandler(console_handler)
logger.addHandler(file_handler)
Java Example (using Logback with Spring Boot):
<!-- logback-spring.xml -->
<?xml version="1.0" encoding="UTF-8"?>
<configuration>
<property name="LOG_PATH" value="logs"/>
<property name="LOG_PATTERN" value="%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n"/>
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>${LOG_PATTERN}</pattern>
</encoder>
</appender>
<appender name="FILE" class="ch.qos.logback.core.rolling.RollingFileAppender">
<file>${LOG_PATH}/application.log</file>
<encoder>
<pattern>${LOG_PATTERN}</pattern>
</encoder>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>${LOG_PATH}/application.%d{yyyy-MM-dd}.log</fileNamePattern>
<maxHistory>30</maxHistory>
</rollingPolicy>
</appender>
<root level="INFO">
<appender-ref ref="CONSOLE"/>
<appender-ref ref="FILE"/>
</root>
<logger name="com.yourcompany.app" level="DEBUG"/>
</configuration>
Structured Logging and Log Levels
Implementing Structured Logging
Structured logging helps in making logs more searchable and analyzable. Here’s how to implement structured logging in both languages:
Python Example:
import json
import logging
from datetime import datetime
class StructuredLogger:
def __init__(self, logger_name):
self.logger = logging.getLogger(logger_name)
def log(self, level, event, **kwargs):
log_entry = {
'timestamp': datetime.utcnow().isoformat(),
'level': level,
'event': event,
'data': kwargs
}
log_message = json.dumps(log_entry)
if level == 'DEBUG':
self.logger.debug(log_message)
elif level == 'INFO':
self.logger.info(log_message)
elif level == 'WARNING':
self.logger.warning(log_message)
elif level == 'ERROR':
self.logger.error(log_message)
elif level == 'CRITICAL':
self.logger.critical(log_message)
# Usage example
logger = StructuredLogger(__name__)
logger.log('ERROR', 'user_authentication_failed',
user_id='12345',
attempt_count=3,
ip_address='192.168.1.1'
)
Java Example:
import net.logstash.logback.argument.StructuredArguments;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class StructuredLogger {
private final Logger logger;
public StructuredLogger(Class<?> clazz) {
this.logger = LoggerFactory.getLogger(clazz);
}
public void logEvent(String level, String event, Map<String, Object> data) {
String message = String.format("Event: %s", event);
Object[] arguments = data.entrySet().stream()
.map(entry -> StructuredArguments.keyValue(entry.getKey(), entry.getValue()))
.toArray();
switch (level.toUpperCase()) {
case "DEBUG":
logger.debug(message, arguments);
break;
case "INFO":
logger.info(message, arguments);
break;
case "WARN":
logger.warn(message, arguments);
break;
case "ERROR":
logger.error(message, arguments);
break;
}
}
}
// Usage example
Map<String, Object> data = new HashMap<>();
data.put("userId", "12345");
data.put("attemptCount", 3);
data.put("ipAddress", "192.168.1.1");
StructuredLogger logger = new StructuredLogger(getClass());
logger.logEvent("ERROR", "user_authentication_failed", data);
Error Handling Best Practices
Here’s a table summarizing the key best practices for error handling in MVC applications:
Category | Best Practice | Description |
---|---|---|
Exception Design | Use Custom Exceptions | Create specific exception classes for different types of errors |
Error Messages | Be Specific but Safe | Provide detailed error messages in logs, but generic messages to users |
Logging | Use Appropriate Levels | Choose the right log level (DEBUG, INFO, WARN, ERROR) for different situations |
Security | Sanitize Error Data | Remove sensitive information from error messages and logs |
Performance | Consider Async Logging | Use asynchronous logging for better performance in high-traffic applications |
Monitoring | Implement Error Tracking | Use error tracking tools to monitor and analyze application errors |
Monitoring and Alerting
Setting Up Error Monitoring
Implementing proper monitoring and alerting systems helps in detecting and responding to errors quickly. Here’s an example of how to integrate with a monitoring service:
Python Example (using Sentry):
import sentry_sdk
from sentry_sdk.integrations.django import DjangoIntegration
sentry_sdk.init(
dsn="your-sentry-dsn",
integrations=[DjangoIntegration()],
traces_sample_rate=1.0,
send_default_pii=False,
environment="production"
)
class ErrorMonitor:
@staticmethod
def capture_exception(exception, context=None):
if context is None:
context = {}
sentry_sdk.capture_exception(
exception,
extra={
'context': context,
'environment': os.getenv('ENVIRONMENT', 'production')
}
)
Java Example (using Spring Boot Actuator):
@Configuration
public class MonitoringConfig {
@Bean
public HealthIndicator customHealthIndicator() {
return new HealthIndicator() {
@Override
public Health health() {
try {
// Perform health checks
return Health.up()
.withDetail("app", "Healthy")
.withDetail("error_count", getErrorCount())
.build();
} catch (Exception e) {
return Health.down()
.withException(e)
.build();
}
}
};
}
private long getErrorCount() {
// Implementation to get error count from your logging system
return 0;
}
}
Conclusion
Implementing robust error handling and logging in MVC applications is crucial for maintaining reliable and maintainable systems. By following the practices and examples outlined in this guide, developers can create more resilient applications that handle errors gracefully and provide valuable debugging information. Remember to regularly review and update your error handling and logging strategies as your application evolves, and always consider security implications when logging sensitive information.
Disclaimer: The code examples and best practices provided in this blog post are based on current industry standards and common implementations. While we strive for accuracy, specific implementation details may vary based on your application’s requirements and the versions of frameworks and libraries used. Please verify all code examples in a testing environment before implementing them in production. If you notice any inaccuracies or have suggestions for improvements, please report them so we can maintain the accuracy and relevance of this content.