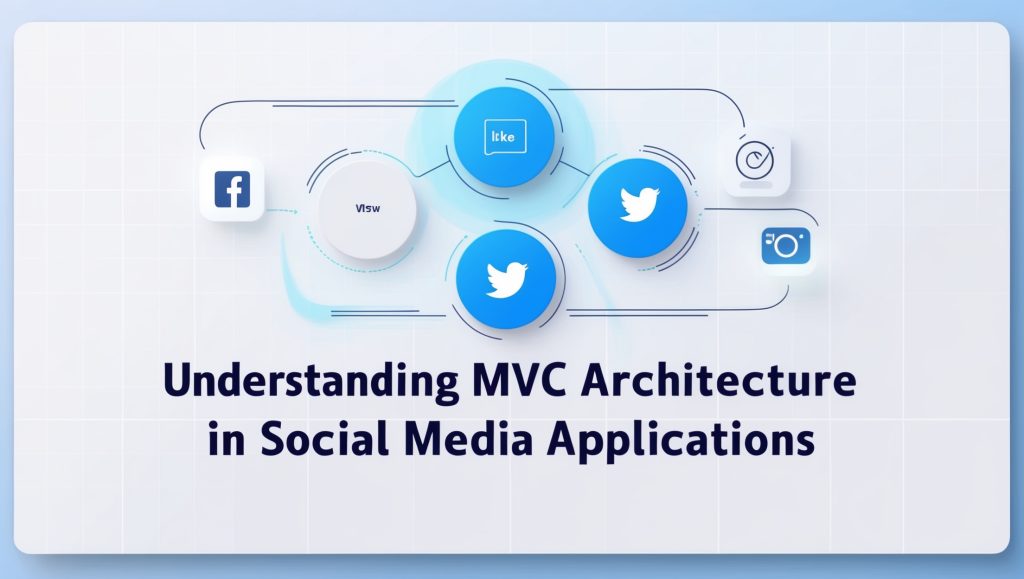
Understanding MVC Architecture in Social Media Applications
The Model-View-Controller (MVC) architectural pattern has become the backbone of modern web applications, particularly in the development of social media platforms. As social networking sites continue to evolve and handle increasingly complex user interactions, the need for a robust and scalable architecture becomes paramount. This comprehensive guide explores how MVC architecture facilitates the development of social media applications, examining its components, benefits, and practical implementations. We’ll delve into real-world examples using both Python and Java, demonstrating how MVC can be leveraged to create maintainable and scalable social networking platforms that can handle millions of users and their interactions efficiently.
Understanding MVC in the Context of Social Media
The MVC architecture separates an application into three distinct components, each serving a specific purpose in the context of social media applications. This separation of concerns is crucial for managing the complexity of social platforms that handle vast amounts of user-generated content, real-time interactions, and complex data relationships. The implementation of MVC in social media applications provides a structured approach to handling user interactions, data management, and presentation logic, making it easier to maintain and scale the application as user needs evolve.
Model Layer in Social Media
The Model layer represents the core business logic and data structures in a social media application. It handles data validation, storage, and retrieval, ensuring data integrity and consistency across the platform. In social media applications, models typically represent entities such as users, posts, comments, likes, and relationships between users. Here’s an example of a Post model implementation in Python using Django:
from django.db import models
from django.contrib.auth.models import User
class Post(models.Model):
author = models.ForeignKey(User, on_delete=models.CASCADE)
content = models.TextField(max_length=5000)
image_url = models.URLField(blank=True, null=True)
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
likes = models.ManyToManyField(User, related_name='liked_posts')
def like_count(self):
return self.likes.count()
def add_like(self, user):
if user not in self.likes.all():
self.likes.add(user)
return True
return False
def remove_like(self, user):
if user in self.likes.all():
self.likes.remove(user)
return True
return False
View Layer in Social Media
The View layer is responsible for presenting data to users and handling user interface components. In social media applications, views manage the presentation of user profiles, news feeds, comment sections, and interactive elements. Here’s an example of a view implementation in Java using Spring MVC:
@Controller
@RequestMapping("/feed")
public class FeedController {
@Autowired
private PostService postService;
@GetMapping
public String getFeed(Model model, @AuthenticationPrincipal User user) {
List<Post> feed = postService.getFeedForUser(user.getId());
model.addAttribute("posts", feed);
model.addAttribute("user", user);
return "feed/index";
}
@PostMapping("/post")
public String createPost(@ModelAttribute PostForm postForm,
@AuthenticationPrincipal User user) {
Post post = new Post();
post.setContent(postForm.getContent());
post.setAuthor(user);
postService.save(post);
return "redirect:/feed";
}
}
Controller Layer in Social Media
The Controller layer acts as an intermediary between the Model and View layers, handling user input and updating both the model and view accordingly. In social media applications, controllers manage user interactions such as posting content, liking posts, and following other users. Here’s an example of a controller implementation in Python using Flask:
from flask import Blueprint, request, jsonify
from models.post import Post
from services.post_service import PostService
post_controller = Blueprint('post_controller', __name__)
post_service = PostService()
@post_controller.route('/api/posts', methods=['POST'])
def create_post():
data = request.get_json()
user_id = get_current_user_id() # Helper function to get authenticated user
try:
post = post_service.create_post(
user_id=user_id,
content=data.get('content'),
image_url=data.get('image_url')
)
return jsonify(post.to_dict()), 201
except ValueError as e:
return jsonify({'error': str(e)}), 400
Benefits of MVC in Social Media Applications
The implementation of MVC architecture in social media applications offers numerous advantages that contribute to the platform’s success and maintainability. Let’s explore these benefits in detail:
Scalability and Maintainability
Aspect | Benefit | Implementation Example |
---|---|---|
Code Organization | Clear separation of concerns | Separate directories for models, views, and controllers |
Module Independence | Easy to modify components | Independent scaling of database, UI, and business logic |
Testing | Simplified unit testing | Isolated testing of each component |
Team Collaboration | Multiple developers can work simultaneously | Different teams can focus on specific components |
MVC architecture allows for efficient performance optimization in social media applications by enabling:
- Caching strategies at different layers
- Asynchronous processing of heavy operations
- Load balancing across multiple servers
- Independent scaling of components
Here’s an example of implementing caching in a Python Django view:
from django.views.decorators.cache import cache_page
from django.core.cache import cache
class FeedView(View):
@cache_page(60 * 15) # Cache for 15 minutes
def get(self, request):
user_id = request.user.id
cache_key = f'user_feed_{user_id}'
# Try to get feed from cache
feed = cache.get(cache_key)
if feed is None:
# If not in cache, generate feed and cache it
feed = self.generate_user_feed(user_id)
cache.set(cache_key, feed, timeout=60*15)
return JsonResponse({'feed': feed})
Implementing Real-time Features with MVC
Real-time features are crucial for social media applications. Here’s how MVC can be implemented to handle real-time updates:
WebSocket Integration
Here’s an example of implementing WebSocket communication in Java using Spring:
@Controller
public class WebSocketController {
@MessageMapping("/post.like")
@SendTo("/topic/feed")
public LikeNotification handleLike(LikeAction likeAction) {
// Process the like action
Post post = postService.findById(likeAction.getPostId());
User user = userService.findById(likeAction.getUserId());
// Update the model
post.addLike(user);
// Broadcast the notification
return new LikeNotification(post, user);
}
}
Push Notifications
Example of implementing push notifications in Python:
class NotificationController:
def __init__(self):
self.firebase = FirebaseAdmin()
def send_push_notification(self, user_id, notification_type, data):
user_tokens = self.get_user_device_tokens(user_id)
for token in user_tokens:
notification = {
'title': self.get_notification_title(notification_type),
'body': self.format_notification_body(data),
'data': data
}
try:
self.firebase.send_notification(token, notification)
except FirebaseError as e:
logger.error(f"Failed to send notification: {str(e)}")
Security Considerations in MVC Social Media Applications
Security is paramount in social media applications. Here’s how MVC helps implement robust security measures:
Authentication and Authorization
Example of implementing authentication middleware in Python Django:
from django.contrib.auth.middleware import AuthenticationMiddleware
from django.core.exceptions import PermissionDenied
class CustomAuthMiddleware(AuthenticationMiddleware):
def process_request(self, request):
super().process_request(request)
# Check if user is authenticated
if not request.user.is_authenticated:
raise PermissionDenied
# Check for account status
if not request.user.is_active:
raise PermissionDenied("Account is deactivated")
# Add rate limiting
self.check_rate_limit(request)
Data Privacy
Example of implementing data privacy controls in Java:
@Entity
public class UserPrivacySettings {
@Id
private Long userId;
@Column
private boolean profilePublic;
@Column
private boolean showEmail;
@Column
@ElementCollection
private List<String> blockedUsers;
public boolean canViewProfile(User viewer) {
if (profilePublic) return true;
if (blockedUsers.contains(viewer.getId())) return false;
return isConnected(viewer);
}
}
Testing MVC Components in Social Media Applications
Comprehensive testing is essential for maintaining reliability. Here’s how to test different MVC components:
Unit Testing Models
Example of testing a Post model in Python:
import unittest
from models.post import Post
class TestPostModel(unittest.TestCase):
def setUp(self):
self.user = create_test_user()
self.post = Post(
author=self.user,
content="Test post content"
)
def test_like_post(self):
other_user = create_test_user()
self.assertTrue(self.post.add_like(other_user))
self.assertEqual(self.post.like_count(), 1)
# Test duplicate like
self.assertFalse(self.post.add_like(other_user))
self.assertEqual(self.post.like_count(), 1)
Integration Testing
Example of integration testing in Java:
@SpringBootTest
@AutoConfigureMockMvc
public class FeedIntegrationTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testFeedGeneration() throws Exception {
// Create test user and posts
User user = createTestUser();
createTestPosts(user);
// Test feed endpoint
mockMvc.perform(get("/api/feed")
.with(user(user)))
.andExpect(status().isOk())
.andExpect(jsonPath("$.posts").isArray())
.andExpect(jsonPath("$.posts.length()").value(10));
}
}
Scaling MVC Architecture for Growing Social Media Platforms
As social media platforms grow, the MVC architecture needs to scale accordingly. Here’s how to implement scaling strategies:
Database Sharding
Example of implementing database sharding in Python:
class ShardedPostRepository:
def __init__(self, shard_count):
self.shard_count = shard_count
self.shards = self.initialize_shards()
def get_shard_for_user(self, user_id):
return user_id % self.shard_count
def save_post(self, post):
shard_id = self.get_shard_for_user(post.author_id)
shard = self.shards[shard_id]
return shard.save_post(post)
def get_user_posts(self, user_id):
shard_id = self.get_shard_for_user(user_id)
shard = self.shards[shard_id]
return shard.get_posts_for_user(user_id)
Conclusion
The MVC architecture provides a robust foundation for building scalable and maintainable social media applications. By separating concerns into distinct components, it enables developers to create complex features while maintaining code organization and allowing for future growth. The examples provided in both Python and Java demonstrate how MVC can be implemented effectively in social media applications, from basic CRUD operations to complex real-time features and scaling strategies.
Disclaimer: The code examples and implementation strategies presented in this blog post are for educational purposes and may need to be adapted based on specific requirements and security considerations. While we strive for accuracy, technologies and best practices evolve rapidly. Please report any inaccuracies or outdated information to our editorial team for prompt correction. The implementation examples provided should be thoroughly tested and secured before deployment in a production environment.