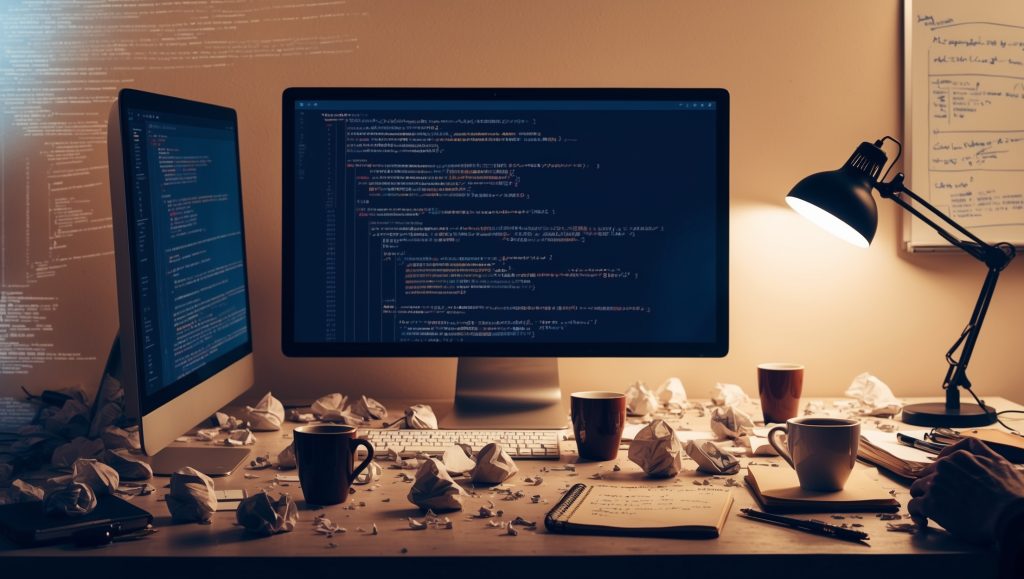
Debugging MVC Applications Effectively
Debugging is an essential skill for any developer working with Model-View-Controller (MVC) applications. As applications grow in complexity, identifying and resolving issues becomes increasingly challenging, requiring a systematic approach and the right set of tools. This comprehensive guide explores various debugging techniques, tools, and best practices specifically tailored for MVC applications. We’ll delve into both traditional debugging methods and modern approaches, providing practical examples in both Python and Java. Understanding these debugging strategies will help developers maintain code quality, reduce development time, and create more reliable applications. Whether you’re dealing with data flow issues, view rendering problems, or controller logic bugs, this guide will equip you with the knowledge to tackle debugging challenges effectively.
Understanding MVC Architecture for Effective Debugging
The Model-View-Controller (MVC) pattern separates applications into three main components, each with its unique debugging challenges. Understanding these components and their interactions is crucial for effective debugging. The Model handles data and business logic, the View manages presentation, and the Controller coordinates between them. When an issue arises, identifying which component is responsible can significantly streamline the debugging process. Common problems often stem from incorrect data flow between components, improper state management, or mishandled user interactions. By understanding the MVC architecture deeply, developers can more easily isolate problems and apply appropriate debugging techniques.
Essential Debugging Tools for MVC Applications
Integrated Development Environment (IDE) Debuggers
Modern IDEs offer powerful debugging capabilities that are essential for MVC development. Visual Studio, Eclipse, PyCharm, and IntelliJ IDEA provide features like breakpoints, watch windows, and step-through debugging. These tools allow developers to inspect variable values, monitor program flow, and evaluate expressions in real-time. IDE debuggers are particularly useful for tracking data as it moves through different MVC components.
Browser Developer Tools
For web-based MVC applications, browser developer tools are indispensable. Chrome DevTools, Firefox Developer Tools, and Safari Web Inspector offer capabilities for debugging JavaScript, inspecting network requests, and analyzing DOM elements. These tools help identify view-related issues, monitor AJAX calls, and debug client-side interactions.
Logging Frameworks
Here’s a comparison of popular logging frameworks:
Framework | Language | Key Features | Best Use Case |
---|---|---|---|
Log4j | Java | Hierarchical logging, multiple output targets | Enterprise applications |
Python logging | Python | Built-in, flexible configuration | Python web applications |
SLF4J | Java | Facade pattern, multiple backend support | Large-scale applications |
Winston | JavaScript | Async logging, multiple transports | Node.js applications |
Debugging data persistence and business logic requires careful attention to database interactions and data transformations. Here’s a systematic approach:
# Python SQLAlchemy debugging example
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger('sqlalchemy.engine')
class UserModel:
def save_user(self, user_data):
try:
# Enable SQL query logging
logger.setLevel(logging.DEBUG)
session = Session()
# Add debug statements for data validation
logger.debug(f"Validating user data: {user_data}")
new_user = User(**user_data)
session.add(new_user)
# Log before commit
logger.info("Attempting to commit new user")
session.commit()
return new_user
except Exception as e:
logger.exception("Error saving user")
session.rollback()
raise
View-Related Issues
View debugging often involves template rendering and data presentation problems:
@Controller
public class ViewController {
private static final Logger logger = LoggerFactory.getLogger(ViewController.class);
@GetMapping("/dashboard")
public String getDashboard(Model model, HttpSession session) {
try {
// Debug view model attributes
logger.debug("Session attributes: {}", session.getAttributeNames());
// Add debugging information to view
model.addAttribute("debugInfo", getDebugInfo());
// Log template selection
String template = determineTemplate(session);
logger.info("Selected template: {}", template);
return template;
} catch (Exception e) {
logger.error("Error preparing dashboard view", e);
return "error";
}
}
private Map<String, Object> getDebugInfo() {
return Map.of(
"timestamp", LocalDateTime.now(),
"environment", env.getActiveProfiles(),
"renderMode", config.getRenderMode()
);
}
}
Performance Debugging in MVC Applications
Profiling Tools and Techniques
Performance debugging requires specialized tools and approaches. Here’s a practical example using Python’s cProfile:
import cProfile
import pstats
from functools import wraps
def profile_endpoint(func):
@wraps(func)
def wrapper(*args, **kwargs):
profiler = cProfile.Profile()
try:
return profiler.runcall(func, *args, **kwargs)
finally:
stats = pstats.Stats(profiler)
stats.sort_stats('cumulative')
stats.print_stats(20) # Print top 20 time-consuming operations
return wrapper
class ProductController:
@profile_endpoint
def get_products(self, category_id):
# Complex operation with potential performance issues
products = self.product_service.find_by_category(category_id)
return self.transform_products(products)
Error Handling and Logging Best Practices
Structured Logging Pattern
Implement structured logging for better debugging capabilities:
@Service
@Slf4j
public class OrderService {
public Order processOrder(OrderRequest request) {
MDC.put("orderId", request.getOrderId());
MDC.put("userId", request.getUserId());
try {
log.info("Beginning order processing");
// Validate order
validateOrder(request);
// Process payment
PaymentResult payment = processPayment(request);
log.debug("Payment processed: {}", payment);
// Create order
Order order = createOrder(request, payment);
log.info("Order processed successfully");
return order;
} catch (ValidationException e) {
log.warn("Order validation failed: {}", e.getMessage());
throw e;
} catch (Exception e) {
log.error("Order processing failed", e);
throw new OrderProcessingException("Failed to process order", e);
} finally {
MDC.clear();
}
}
}
Testing and Debugging Integration
Unit Test Debugging Example
import unittest
from unittest.mock import Mock, patch
import logging
class UserServiceTest(unittest.TestCase):
@classmethod
def setUpClass(cls):
logging.basicConfig(level=logging.DEBUG)
cls.logger = logging.getLogger(__name__)
def setUp(self):
self.user_repository = Mock()
self.user_service = UserService(self.user_repository)
@patch('services.email_service.EmailService')
def test_user_registration(self, mock_email_service):
# Arrange
user_data = {
'email': 'test@example.com',
'password': 'secure123'
}
# Act with debug logging
self.logger.debug(f"Testing user registration with data: {user_data}")
result = self.user_service.register_user(user_data)
# Assert with detailed logging
self.logger.info(f"Registration result: {result}")
self.assertTrue(result.success)
self.user_repository.save.assert_called_once()
mock_email_service.send_welcome_email.assert_called_once()
Security Debugging Considerations
Security Logging Pattern
@Aspect
@Component
public class SecurityAuditAspect {
private static final Logger securityLogger = LoggerFactory.getLogger("SECURITY_AUDIT");
@Around("@annotation(secured)")
public Object auditSecurityAccess(ProceedingJoinPoint joinPoint, Secured secured) throws Throwable {
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
String method = joinPoint.getSignature().getName();
try {
// Log security-relevant information
securityLogger.info("Security check - Method: {}, User: {}, Roles: {}",
method,
auth.getName(),
auth.getAuthorities()
);
Object result = joinPoint.proceed();
// Log successful access
securityLogger.info("Access granted - Method: {}, User: {}", method, auth.getName());
return result;
} catch (AccessDeniedException e) {
// Log security violations
securityLogger.warn("Access denied - Method: {}, User: {}, Reason: {}",
method,
auth.getName(),
e.getMessage()
);
throw e;
}
}
}
Conclusion
Effective debugging of MVC applications requires a combination of tools, techniques, and best practices. By following the structured approach outlined in this guide, developers can more efficiently identify and resolve issues across all layers of their applications. Remember to implement comprehensive logging, utilize appropriate debugging tools, and maintain a systematic approach to troubleshooting. Regular testing and monitoring help catch issues early, reducing the need for extensive debugging in production environments.
Disclaimer: The code examples and techniques presented in this blog post are based on common debugging practices and may need to be adapted to specific project requirements and environments. While we strive for accuracy, technology evolves rapidly, and some information may become outdated. Please verify current best practices and tool versions for your specific use case. If you notice any inaccuracies or have suggestions for improvements, please report them to our editorial team.