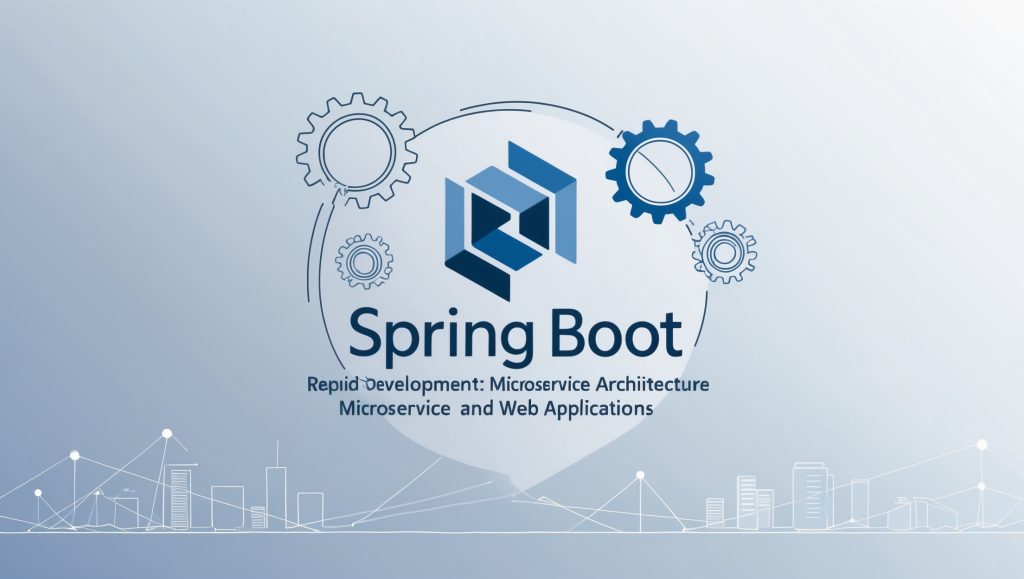
Spring Boot for Rapid Application Development – Building microservices and web applications
Spring Boot has revolutionized the way developers build Java applications by simplifying the development process and providing a robust foundation for creating enterprise-grade applications. In today’s fast-paced technology landscape, businesses need to quickly adapt and deploy applications that are both scalable and maintainable. Spring Boot addresses these requirements by offering a convention-over-configuration approach, embedded servers, and a comprehensive ecosystem of starter dependencies. This comprehensive guide will explore how Spring Boot facilitates rapid application development, with a particular focus on building microservices and web applications. We’ll dive deep into real-world examples, best practices, and practical implementations that will help you leverage Spring Boot’s full potential.
Understanding Spring Boot’s Core Features
Spring Boot’s architecture is built upon several key features that make it an ideal choice for rapid application development. The framework eliminates much of the boilerplate configuration required in traditional Spring applications by providing sensible defaults and auto-configuration capabilities. These features include the embedded server container, which allows applications to be deployed as standalone JARs, and the extensive starter dependencies that automatically configure required libraries based on your project’s needs. The Spring Boot Actuator provides production-ready features for monitoring and managing your application, while the Spring Boot DevTools offers automatic restart capabilities during development. Together, these features create a powerful development environment that significantly reduces time-to-market for new applications.
Setting Up Your First Spring Boot Application
Project Initialization
Let’s start by creating a basic Spring Boot application. The easiest way to bootstrap a new project is using the Spring Initializer (start.spring.io) or your preferred IDE. Here’s an example of a basic project structure:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.0</version>
</parent>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
</project>
Basic Application Structure
Here’s a simple Spring Boot application structure:
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
Building RESTful Web Services
Spring Boot excels at creating RESTful web services quickly and efficiently. The spring-boot-starter-web dependency provides everything needed to build web applications, including an embedded Tomcat server and Spring MVC support. Let’s create a simple REST controller:
@RestController
@RequestMapping("/api/v1")
public class ProductController {
private final ProductService productService;
@Autowired
public ProductController(ProductService productService) {
this.productService = productService;
}
@GetMapping("/products")
public List<Product> getAllProducts() {
return productService.findAll();
}
@PostMapping("/products")
public ResponseEntity<Product> createProduct(@RequestBody Product product) {
Product savedProduct = productService.save(product);
return new ResponseEntity<>(savedProduct, HttpStatus.CREATED);
}
@GetMapping("/products/{id}")
public ResponseEntity<Product> getProductById(@PathVariable Long id) {
return productService.findById(id)
.map(product -> new ResponseEntity<>(product, HttpStatus.OK))
.orElse(new ResponseEntity<>(HttpStatus.NOT_FOUND));
}
}
Implementing Microservices Architecture
Service Discovery and Registration
Spring Boot makes it easy to implement microservices using Spring Cloud. Here’s an example of setting up service discovery using Netflix Eureka:
@SpringBootApplication
@EnableEurekaServer
public class ServiceRegistryApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceRegistryApplication.class, args);
}
}
Circuit Breaker Implementation
Implementing circuit breakers using Resilience4j in Spring Boot:
@RestController
public class ServiceController {
@CircuitBreaker(name = "serviceA", fallbackMethod = "fallbackMethod")
@GetMapping("/service-a")
public String serviceA() {
// Service call that might fail
return restTemplate.getForObject("http://service-a/api/data", String.class);
}
public String fallbackMethod(Exception ex) {
return "Fallback response due to service failure";
}
}
Data Access and Persistence
Spring Boot simplifies database operations through its excellent integration with Spring Data JPA. Here’s an example of implementing data persistence:
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private BigDecimal price;
// Getters and setters
}
@Repository
public interface ProductRepository extends JpaRepository<Product, Long> {
List<Product> findByNameContaining(String name);
List<Product> findByPriceLessThan(BigDecimal price);
}
Security Implementation
Basic Security Configuration
Spring Boot provides comprehensive security features through Spring Security. Here’s a basic security configuration:
@Configuration
@EnableWebSecurity
public class SecurityConfig {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(auth -> auth
.requestMatchers("/api/public/**").permitAll()
.requestMatchers("/api/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
)
.oauth2ResourceServer()
.jwt();
return http.build();
}
}
Testing Spring Boot Applications
Spring Boot provides excellent testing support through spring-boot-starter-test. Here’s an example of different testing approaches:
@SpringBootTest
class ProductServiceTest {
@Autowired
private ProductService productService;
@MockBean
private ProductRepository productRepository;
@Test
void whenValidProduct_thenProductShouldBeSaved() {
// Given
Product product = new Product("Test Product", new BigDecimal("99.99"));
when(productRepository.save(any(Product.class))).thenReturn(product);
// When
Product savedProduct = productService.save(product);
// Then
assertNotNull(savedProduct);
assertEquals("Test Product", savedProduct.getName());
verify(productRepository).save(any(Product.class));
}
}
Configuration Management
Spring Boot offers various ways to manage application configuration. Here’s a comparison of different approaches:
Method | Use Case | Advantages |
---|---|---|
application.properties | Simple configurations | Easy to read and modify |
application.yml | Complex hierarchical configs | Better structure and readability |
Environment Variables | Production deployments | Security and flexibility |
Config Server | Microservices architecture | Centralized configuration |
Spring Boot Actuator provides production-ready features for monitoring your application. Here’s how to implement custom metrics:
@Component
public class CustomMetricsConfiguration {
private final MeterRegistry registry;
public CustomMetricsConfiguration(MeterRegistry registry) {
this.registry = registry;
}
@Bean
public Counter requestCounter() {
return Counter.builder("api.requests.total")
.description("Total API requests")
.register(registry);
}
}
Deployment and DevOps Integration
Docker Integration
Example Dockerfile for a Spring Boot application:
FROM openjdk:17-jdk-slim
VOLUME /tmp
COPY target/*.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
Kubernetes Configuration
Example Kubernetes deployment configuration:
apiVersion: apps/v1
kind: Deployment
metadata:
name: spring-boot-app
spec:
replicas: 3
selector:
matchLabels:
app: spring-boot-app
template:
metadata:
labels:
app: spring-boot-app
spec:
containers:
- name: spring-boot-app
image: spring-boot-app:latest
ports:
- containerPort: 8080
Best Practices and Performance Optimization
Application Performance
Here are key considerations for optimizing Spring Boot applications:
- Use appropriate connection pools for database operations
- Implement caching strategies using Spring Cache
- Optimize JVM settings for container environments
- Use async processing for long-running tasks
- Implement proper logging levels and strategies
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
SimpleCacheManager cacheManager = new SimpleCacheManager();
cacheManager.setCaches(Arrays.asList(
new ConcurrentMapCache("products"),
new ConcurrentMapCache("categories")
));
return cacheManager;
}
}
Conclusion
Spring Boot has established itself as an invaluable framework for rapid application development, offering a perfect balance of convenience and flexibility. Its robust ecosystem, extensive documentation, and active community make it an excellent choice for building both microservices and web applications. By following the practices and examples outlined in this guide, developers can create scalable, maintainable, and production-ready applications efficiently. Remember to stay updated with the latest Spring Boot releases and best practices as the framework continues to evolve and improve.
Disclaimer: This blog post is intended for educational purposes only. While we strive to provide accurate and up-to-date information, technologies and best practices may change over time. Please refer to the official Spring Boot documentation for the most current information. If you notice any inaccuracies in this post, please report them to our editorial team at [editorialteam@felixrante.com], and we will update the content promptly.