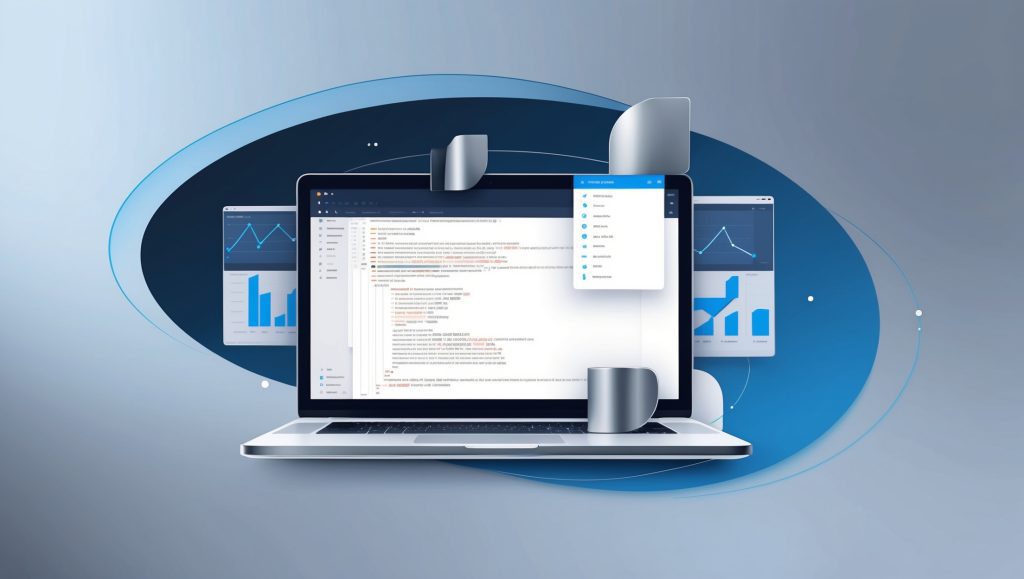
Performance Tuning Java Applications – Identifying and resolving bottlenecks
Performance tuning is a critical aspect of Java application development that can make the difference between a sluggish application and one that runs smoothly at scale. As applications grow in complexity and user base, the need for optimal performance becomes increasingly important. Poor performance can lead to frustrated users, increased infrastructure costs, and lost business opportunities. This comprehensive guide will walk you through the essential aspects of performance tuning in Java applications, from identifying bottlenecks to implementing effective solutions. We’ll explore various tools, techniques, and best practices that can help you optimize your Java applications for maximum efficiency.
Understanding Performance Metrics
Before diving into performance tuning, it’s crucial to understand the key metrics that indicate your application’s health and performance. These metrics serve as benchmarks for improvement and help identify areas that require optimization. Response time, throughput, latency, and resource utilization are fundamental metrics that provide insights into application performance. Different types of applications may prioritize different metrics, but understanding these basic measurements is essential for effective performance tuning.
Key Performance Indicators (KPIs)
Metric | Description | Target Range |
---|---|---|
Response Time | Time taken to process a request and send response | < 1 second |
Throughput | Number of requests processed per unit time | Application specific |
CPU Utilization | Percentage of CPU resources used | 70-80% peak |
Memory Usage | Amount of heap and non-heap memory consumed | < 80% of max heap |
Garbage Collection | Time spent in GC pauses | < 1% of total time |
Performance bottlenecks can occur at various levels in a Java application, from code-level inefficiencies to system-level constraints. Understanding these common bottlenecks is the first step toward effective optimization. The most frequent bottlenecks include inefficient database queries, memory leaks, excessive garbage collection, unoptimized code, and resource contention. External factors such as network latency and disk I/O can also significantly impact application performance.
Memory Management Issues
Memory-related problems are among the most common performance bottlenecks in Java applications. These issues can manifest as memory leaks, excessive garbage collection, or inefficient object creation and disposal. Let’s look at an example of how to identify and fix a memory leak:
public class MemoryLeakExample {
// Problematic implementation
private static final List<Object> leakyList = new ArrayList<>();
public void addItem(Object item) {
leakyList.add(item); // Items are never removed
}
// Fixed implementation
private final Map<String, WeakReference<Object>> cache = new WeakHashMap<>();
public void addItemToCache(String key, Object item) {
cache.put(key, new WeakReference<>(item));
}
public Object getItemFromCache(String key) {
WeakReference<Object> ref = cache.get(key);
return ref != null ? ref.get() : null;
}
}
Profiling Tools and Techniques
Profiling is essential for identifying performance bottlenecks in Java applications. Modern profiling tools provide detailed insights into application behavior, resource usage, and performance metrics. These tools help developers make data-driven decisions about optimization strategies.
Popular Java Profiling Tools
Tool | Features | Best Used For |
---|---|---|
JProfiler | CPU, memory, thread profiling | Detailed analysis |
VisualVM | Lightweight monitoring | Quick diagnostics |
YourKit | Advanced memory analysis | Memory leak detection |
Java Flight Recorder | Low-overhead profiling | Production monitoring |
Database operations often become a significant bottleneck in Java applications. Optimizing database access patterns, query execution, and connection management can dramatically improve application performance. Here’s an example of implementing connection pooling using HikariCP:
public class DatabaseConfig {
public DataSource setupDataSource() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost:5432/mydb");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(10);
config.setMinimumIdle(5);
config.setIdleTimeout(300000);
config.setConnectionTimeout(20000);
return new HikariDataSource(config);
}
// Example of optimized query execution
public List<User> getActiveUsers(DataSource dataSource) {
String sql = "SELECT id, name, email FROM users WHERE active = true";
try (Connection conn = dataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement(sql)) {
List<User> users = new ArrayList<>();
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
users.add(new User(
rs.getLong("id"),
rs.getString("name"),
rs.getString("email")
));
}
return users;
} catch (SQLException e) {
throw new RuntimeException("Error fetching active users", e);
}
}
}
Optimizing Garbage Collection
Garbage collection (GC) performance can significantly impact application responsiveness. Understanding GC behavior and tuning its parameters is crucial for optimal performance. Modern Java applications have several garbage collector options, each with its own strengths and use cases.
Garbage Collector Comparison
Collector | Pros | Cons | Best For |
---|---|---|---|
G1GC | Balanced performance | Memory overhead | General use |
ZGC | Low latency | Higher CPU usage | Low latency apps |
Parallel GC | High throughput | Higher pauses | Batch processing |
Thread pool configuration can significantly impact application performance. Here’s an example of creating an optimized thread pool:
public class ThreadPoolConfig {
public ExecutorService createOptimizedThreadPool() {
int corePoolSize = Runtime.getRuntime().availableProcessors();
int maxPoolSize = corePoolSize * 2;
long keepAliveTime = 60L;
return new ThreadPoolExecutor(
corePoolSize,
maxPoolSize,
keepAliveTime,
TimeUnit.SECONDS,
new ArrayBlockingQueue<>(100),
new ThreadPoolExecutor.CallerRunsPolicy()
);
}
// Example usage with async processing
public CompletableFuture<Result> processAsync(Task task) {
return CompletableFuture.supplyAsync(() -> {
// Process task
return new Result();
}, executorService);
}
}
Caching Strategies
Implementing effective caching strategies can significantly improve application performance. Here’s an example using Caffeine cache:
public class CacheConfig {
private final Cache<String, User> userCache = Caffeine.newBuilder()
.maximumSize(10_000)
.expireAfterWrite(10, TimeUnit.MINUTES)
.recordStats()
.build();
public User getUser(String userId) {
return userCache.get(userId, this::loadUserFromDatabase);
}
private User loadUserFromDatabase(String userId) {
// Database loading logic
return new User();
}
}
Network Optimization
Network performance can be optimized through various techniques:
Connection Pooling
public class HttpClientConfig {
public HttpClient createOptimizedClient() {
return HttpClient.newBuilder()
.connectTimeout(Duration.ofSeconds(10))
.executor(createOptimizedThreadPool())
.version(HttpClient.Version.HTTP_2)
.build();
}
// Example of concurrent requests
public List<String> fetchMultipleUrls(List<String> urls) {
return urls.parallelStream()
.map(url -> {
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create(url))
.GET()
.build();
try {
HttpResponse<String> response = httpClient
.send(request, HttpResponse.BodyHandlers.ofString());
return response.body();
} catch (Exception e) {
return null;
}
})
.filter(Objects::nonNull)
.collect(Collectors.toList());
}
}
Performance Testing
Regular performance testing is crucial for maintaining application health. Here’s an example using JMH (Java Microbenchmark Harness):
@State(Scope.Thread)
public class PerformanceTest {
@Benchmark
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.MICROSECONDS)
public void testMethod() {
// Method to benchmark
}
public static void main(String[] args) throws Exception {
Options opt = new OptionsBuilder()
.include(PerformanceTest.class.getSimpleName())
.forks(1)
.warmupIterations(5)
.measurementIterations(5)
.build();
new Runner(opt).run();
}
}
Best Practices and Recommendations
Following are key best practices for maintaining optimal application performance:
- Regular Monitoring: Implement comprehensive monitoring solutions to track application performance metrics continuously.
- Proactive Optimization: Address performance issues before they become critical problems.
- Load Testing: Regularly conduct load tests to ensure application stability under stress.
- Documentation: Maintain detailed documentation of performance optimizations and configurations.
- Code Reviews: Include performance considerations in code review processes.
Conclusion
Performance tuning is an ongoing process that requires regular monitoring, analysis, and optimization. By following the strategies and best practices outlined in this guide, you can significantly improve your Java application’s performance. Remember that performance optimization should be data-driven and focused on addressing specific bottlenecks rather than premature optimization. Regular testing, monitoring, and maintenance are key to maintaining optimal performance over time.
Disclaimer: This blog post represents general guidelines and best practices for Java application performance tuning as of 2024. Specific requirements and optimal configurations may vary based on your application’s needs and infrastructure. The code examples provided are for illustration purposes and may need to be adapted for production use. Please report any inaccuracies to our editorial team for prompt correction. Always test performance optimizations thoroughly in a staging environment before applying them to production systems.