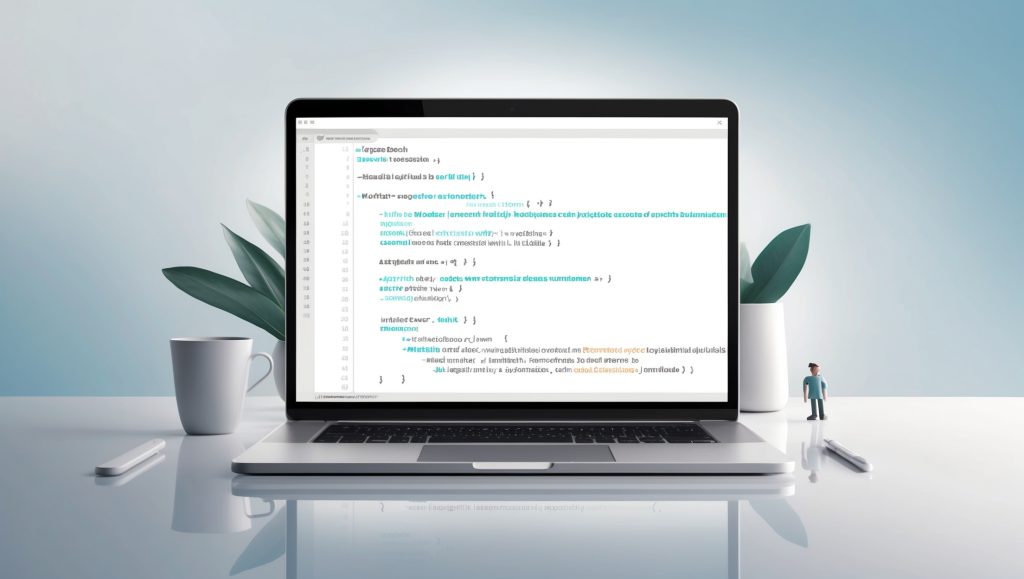
Clean Code Practices in Java – Writing readable and understandable code
Writing clean code is an essential skill that distinguishes exceptional developers from average ones. In the Java ecosystem, where applications can grow tremendously complex, maintaining code readability and understandability becomes crucial for long-term project success. This comprehensive guide explores best practices, principles, and practical examples to help you write cleaner, more maintainable Java code that your fellow developers will appreciate.
Understanding Clean Code Fundamentals
Clean code is not just about making your code work; it’s about making it work elegantly and efficiently while ensuring it remains maintainable and scalable. The concept revolves around writing code that is easy to understand, easy to modify, and easy to test. Clean code should read like well-written prose, telling a clear story about its purpose and functionality.
What Makes Code “Clean”?
Clean code exhibits several key characteristics that set it apart from merely functional code. It should be focused, simple, and testable. Each function, class, and module should have a single, well-defined purpose. The code should be written in a way that makes it easy for other developers to understand and modify. Most importantly, clean code should be maintainable over time, reducing the technical debt that often accumulates in software projects.
Naming Conventions and Best Practices
Proper naming is perhaps the most crucial aspect of writing clean code. Good names can make code self-documenting, while poor names can make even simple code difficult to understand. In Java, following established naming conventions is particularly important due to the language’s verbose nature.
Class Names
Classes should be named using nouns or noun phrases in PascalCase. The name should clearly indicate the class’s responsibility and purpose.
// Good examples
class CustomerAccount {}
class PaymentProcessor {}
class EmailValidator {}
// Bad examples
class Process {} // Too vague
class DoThings {} // Verb phrase, not descriptive
class data {} // Not PascalCase, not descriptive
Method Names
Methods should be named using verbs or verb phrases in camelCase. The name should clearly describe the action being performed.
// Good examples
public void processPayment(Payment payment) {}
public Customer findCustomerById(Long id) {}
public boolean isEligibleForDiscount() {}
// Bad examples
public void data() {} // Not descriptive
public void Payment() {} // Looks like a constructor
public void DOSomething() {} // Incorrect casing
Code Organization and Structure
Well-organized code is easier to navigate and maintain. Java provides several mechanisms for organizing code effectively.
Package Structure
A good package structure helps in managing large codebases efficiently. Here’s a recommended approach:
com.company.project/
├── domain/
│ ├── Customer.java
│ ├── Order.java
│ └── Product.java
├── repository/
│ ├── CustomerRepository.java
│ ├── OrderRepository.java
│ └── ProductRepository.java
├── service/
│ ├── CustomerService.java
│ ├── OrderService.java
│ └── ProductService.java
└── util/
└── ValidationUtils.java
Class Structure
Within classes, maintain a consistent order of elements:
- Static fields
- Instance fields
- Constructors
- Public methods
- Private methods
- Inner classes
public class OrderProcessor {
// Static fields
private static final int MAX_RETRIES = 3;
// Instance fields
private final OrderRepository orderRepository;
private final PaymentService paymentService;
// Constructor
public OrderProcessor(OrderRepository orderRepository, PaymentService paymentService) {
this.orderRepository = orderRepository;
this.paymentService = paymentService;
}
// Public methods
public void processOrder(Order order) {
validateOrder(order);
saveOrder(order);
processPayment(order);
}
// Private methods
private void validateOrder(Order order) {
// Validation logic
}
}
Method Design and Implementation
Methods are the building blocks of any Java application. Writing clean, focused methods is crucial for maintaining code quality.
Single Responsibility Principle
Each method should do one thing and do it well. This principle helps in creating more maintainable and testable code.
// Bad example - method doing multiple things
public void processOrderAndSendEmail(Order order) {
// Validate order
if (order == null || order.getItems().isEmpty()) {
throw new IllegalArgumentException("Invalid order");
}
// Calculate total
double total = 0;
for (OrderItem item : order.getItems()) {
total += item.getPrice() * item.getQuantity();
}
// Save order
orderRepository.save(order);
// Send email
EmailService.sendOrderConfirmation(order);
}
// Good example - separated responsibilities
public void processOrder(Order order) {
validateOrder(order);
double total = calculateOrderTotal(order);
saveOrder(order);
notifyCustomer(order);
}
private void validateOrder(Order order) {
if (order == null || order.getItems().isEmpty()) {
throw new IllegalArgumentException("Invalid order");
}
}
private double calculateOrderTotal(Order order) {
return order.getItems().stream()
.mapToDouble(item -> item.getPrice() * item.getQuantity())
.sum();
}
private void notifyCustomer(Order order) {
EmailService.sendOrderConfirmation(order);
}
Error Handling and Exception Management
Proper error handling is crucial for writing robust and maintainable code. Clean code should handle errors gracefully and provide meaningful feedback.
Custom Exceptions
Create custom exceptions for specific error scenarios to make error handling more meaningful and maintainable.
public class OrderProcessingException extends RuntimeException {
public OrderProcessingException(String message) {
super(message);
}
public OrderProcessingException(String message, Throwable cause) {
super(message, cause);
}
}
public class OrderService {
public void processOrder(Order order) {
try {
validateOrder(order);
saveOrder(order);
} catch (ValidationException e) {
throw new OrderProcessingException("Invalid order data", e);
} catch (DatabaseException e) {
throw new OrderProcessingException("Failed to save order", e);
}
}
}
Comments and Documentation
While clean code should be self-documenting, there are times when comments and documentation are necessary.
When to Comment
Use comments to explain:
- Complex business rules
- Non-obvious design decisions
- Workarounds for known issues
- API documentation
/**
* Processes a customer order with the following steps:
* 1. Validates order details
* 2. Checks inventory availability
* 3. Processes payment
* 4. Updates inventory
* 5. Sends confirmation email
*
* @param order The order to process
* @throws OrderProcessingException if any step fails
*/
public void processOrder(Order order) {
// Implementation
}
// Complex business rule explanation
private boolean isEligibleForDiscount(Customer customer) {
// Premium customers get discount on orders above $1000
// Regular customers get discount on orders above $5000
return (customer.isPremium() && customer.getOrderTotal() > 1000) ||
(!customer.isPremium() && customer.getOrderTotal() > 5000);
}
Unit Testing and Test-Driven Development
Clean code is inherently testable. Following test-driven development (TDD) principles can help ensure your code remains clean and maintainable.
Writing Clean Tests
Tests should be clear, focused, and follow the Arrange-Act-Assert pattern.
public class OrderProcessorTest {
@Test
void shouldProcessValidOrder() {
// Arrange
Order order = new Order();
order.addItem(new OrderItem("Product1", 2, 50.0));
OrderProcessor processor = new OrderProcessor();
// Act
OrderResult result = processor.processOrder(order);
// Assert
assertTrue(result.isSuccessful());
assertEquals(100.0, result.getTotalAmount());
verify(emailService).sendConfirmation(order);
}
}
Code Review Checklist
When reviewing code for cleanliness, consider the following aspects:
Readability and Maintainability
- Is the code self-documenting?
- Are naming conventions followed consistently?
- Is the code properly formatted?
-
Are complex operations adequately documented?
Structure and Organization
- Does each class have a single responsibility?
- Is the code properly organized in packages?
-
Are related functions grouped together logically?
Error Handling
- Are exceptions handled appropriately?
- Are custom exceptions used where appropriate?
-
Is error feedback meaningful and helpful?
Testing
- Are unit tests present and comprehensive?
- Do tests cover edge cases?
- Are tests readable and maintainable?
Performance Considerations
While clean code primarily focuses on readability and maintainability, it shouldn’t come at the expense of performance.
Efficient Collections Usage
Choose the right collection type for your needs:
// Good - Using appropriate collection types
Set<String> uniqueCustomers = new HashSet<>(); // For unique values
List<Order> orderHistory = new ArrayList<>(); // For ordered elements
Map<String, Customer> customerLookup = new HashMap<>(); // For key-value pairs
// Bad - Using wrong collection types
List<String> uniqueCustomers = new ArrayList<>(); // Inefficient for checking duplicates
Conclusion
Writing clean code is an essential skill that requires continuous practice and refinement. By following these practices and principles, you can create more maintainable, readable, and robust Java applications. Remember that clean code is not just about following rules—it’s about writing code that others (including your future self) can understand and maintain easily.
Key takeaways:
- Focus on meaningful names and consistent conventions
- Keep methods and classes focused and single-purpose
- Write self-documenting code with minimal but meaningful comments
- Handle errors gracefully and appropriately
- Write comprehensive tests
- Consider both maintainability and performance
Disclaimer: This guide represents current best practices in Java development as of 2024. While we strive for accuracy, programming practices evolve over time. If you notice any inaccuracies or have suggestions for improvements, please report them to our editorial team at [editorialteam@felixrante.com]. Your feedback helps us maintain the quality and relevance of our content.