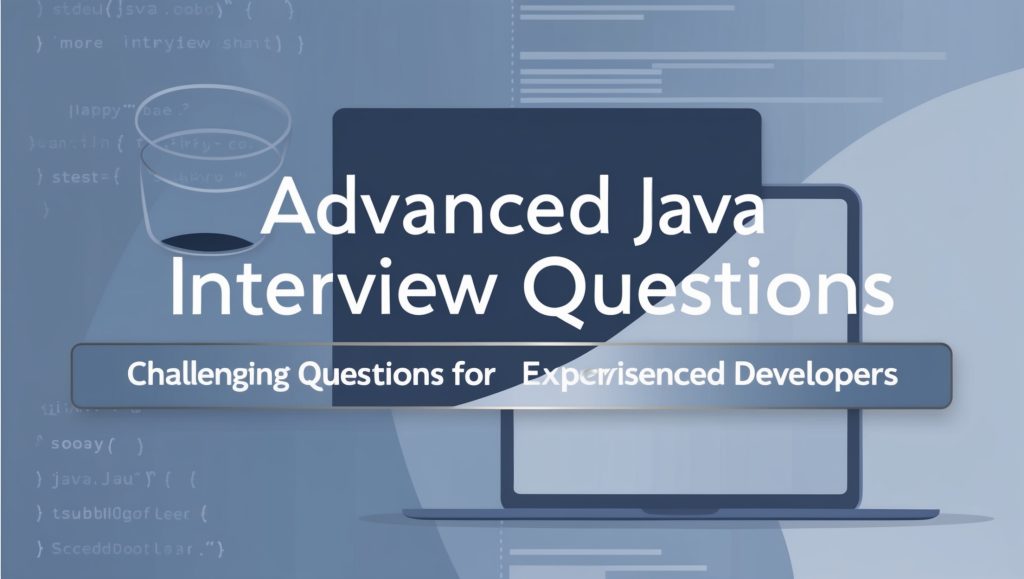
Advanced Java Interview Questions – Challenging questions for experienced developers
In today’s competitive tech landscape, Java continues to be one of the most sought-after programming languages, especially for enterprise applications. This comprehensive guide delves into challenging Java interview questions specifically tailored for experienced developers. Whether you’re preparing for a senior developer position or looking to refresh your advanced Java knowledge, these questions and detailed explanations will help you demonstrate your expertise during technical interviews.
Concurrency and Multithreading
One of the most critical aspects of Java development is understanding concurrency and multithreading. These concepts are fundamental to building scalable and efficient applications, and interviewers often focus heavily on these topics to assess a candidate’s expertise.
Thread Safety and Synchronization
Thread safety is a crucial concept that every experienced Java developer must master. When multiple threads access shared resources simultaneously, proper synchronization becomes essential to prevent race conditions and ensure data consistency. Consider the following example of a thread-safe singleton implementation using double-checked locking:
public class ThreadSafeSingleton {
private static volatile ThreadSafeSingleton instance;
private ThreadSafeSingleton() {}
public static ThreadSafeSingleton getInstance() {
if (instance == null) {
synchronized (ThreadSafeSingleton.class) {
if (instance == null) {
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
}
ExecutorService and Thread Pools
Understanding thread pool management is essential for optimizing application performance. The ExecutorService framework provides a high-level abstraction for working with threads. Here’s an example demonstrating various thread pool implementations:
public class ThreadPoolExample {
public static void main(String[] args) {
// Fixed thread pool
ExecutorService fixedPool = Executors.newFixedThreadPool(5);
// Cached thread pool
ExecutorService cachedPool = Executors.newCachedThreadPool();
// Scheduled thread pool
ScheduledExecutorService scheduledPool = Executors.newScheduledThreadPool(3);
// Submit tasks
Future<String> future = fixedPool.submit(() -> {
Thread.sleep(1000);
return "Task completed";
});
// Shutdown pools
fixedPool.shutdown();
cachedPool.shutdown();
scheduledPool.shutdown();
}
}
Memory Management and Garbage Collection
Memory management is a critical aspect of Java development that directly impacts application performance and stability. Understanding how the JVM manages memory and performs garbage collection is essential for optimizing applications.
Understanding Different Memory Areas
The JVM memory structure consists of several key areas:
Memory Area | Description | Common Issues |
---|---|---|
Heap | Stores objects and arrays | Memory leaks, OutOfMemoryError |
Stack | Stores primitive values and references | StackOverflowError |
Metaspace | Stores class metadata | Class loader leaks |
Code Cache | Stores compiled code | JIT compilation issues |
Understanding different garbage collection algorithms and their impact on application performance is crucial. Here’s an example of configuring G1 garbage collector:
public class GCExample {
public static void main(String[] args) {
// JVM arguments for G1 GC
// -XX:+UseG1GC
// -XX:MaxGCPauseMillis=200
// -XX:G1HeapRegionSize=16M
List<byte[]> memory = new ArrayList<>();
while (true) {
memory.add(new byte[1024 * 1024]); // Allocate 1MB
Thread.sleep(100);
}
}
}
Design Patterns and Best Practices
Design patterns are essential tools in a developer’s arsenal for solving common software design problems. Understanding when and how to apply these patterns is crucial for creating maintainable and scalable applications.
Behavioral Design Patterns
The Observer pattern is commonly used in event-driven programming. Here’s an implementation example:
public interface Observer {
void update(String message);
}
public class NewsAgency {
private List<Observer> observers = new ArrayList<>();
public void addObserver(Observer observer) {
observers.add(observer);
}
public void notifyObservers(String news) {
observers.forEach(observer -> observer.update(news));
}
}
public class NewsChannel implements Observer {
private String name;
public NewsChannel(String name) {
this.name = name;
}
@Override
public void update(String news) {
System.out.println(name + " received news: " + news);
}
}
Advanced Collections and Data Structures
Understanding the internals of Java collections and choosing the right data structure is crucial for application performance. This section covers advanced concepts related to collections framework.
Concurrent Collections
Java provides several thread-safe collections for concurrent applications:
public class ConcurrentCollectionsExample {
public void demonstrateConcurrentCollections() {
// Thread-safe map with high concurrency
ConcurrentHashMap<String, Integer> concurrentMap = new ConcurrentHashMap<>();
// Thread-safe queue
ConcurrentLinkedQueue<String> concurrentQueue = new ConcurrentLinkedQueue<>();
// Thread-safe deque
ConcurrentLinkedDeque<String> concurrentDeque = new ConcurrentLinkedDeque<>();
// Skip list set for concurrent access
ConcurrentSkipListSet<Integer> skipListSet = new ConcurrentSkipListSet<>();
}
}
Custom Collections Implementation
Understanding how to implement custom collections is important. Here’s an example of a thread-safe bounded queue:
public class BoundedQueue<T> {
private final Object[] items;
private int head, tail, count;
public BoundedQueue(int capacity) {
items = new Object[capacity];
}
public synchronized void put(T item) throws InterruptedException {
while (count == items.length) {
wait();
}
items[tail] = item;
tail = (tail + 1) % items.length;
count++;
notifyAll();
}
public synchronized T take() throws InterruptedException {
while (count == 0) {
wait();
}
@SuppressWarnings("unchecked")
T item = (T) items[head];
head = (head + 1) % items.length;
count--;
notifyAll();
return item;
}
}
Performance Optimization and Profiling
Performance optimization is a critical skill for experienced Java developers. Understanding how to identify and resolve performance bottlenecks is essential for maintaining high-performing applications.
JVM Tuning Parameters
Common JVM tuning parameters and their effects:
Parameter | Description | Use Case |
---|---|---|
-Xmx | Maximum heap size | Large applications |
-Xms | Initial heap size | Reducing GC overhead |
-XX:NewRatio | Ratio of old/new generation | Memory-intensive apps |
-XX:SurvivorRatio | Ratio of eden/survivor spaces | Fine-tuning GC |
Understanding how to use profiling tools is crucial. Here’s an example using JMH for microbenchmarking:
@State(Scope.Thread)
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.NANOSECONDS)
public class StringConcatenationBenchmark {
@Benchmark
public String testStringBuilder() {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 100; i++) {
sb.append(i);
}
return sb.toString();
}
@Benchmark
public String testStringConcat() {
String result = "";
for (int i = 0; i < 100; i++) {
result += i;
}
return result;
}
}
Microservices and Distributed Systems
Modern Java applications often operate in distributed environments. Understanding microservices architecture and distributed systems concepts is crucial for senior developers.
Service Discovery and Load Balancing
Here’s an example of service registration using Spring Cloud Netflix Eureka:
@SpringBootApplication
@EnableEurekaServer
public class ServiceRegistryApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceRegistryApplication.class, args);
}
}
@SpringBootApplication
@EnableDiscoveryClient
public class MicroserviceApplication {
@LoadBalanced
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
Distributed Tracing
Understanding distributed tracing is essential for debugging microservices. Here’s an example using Spring Cloud Sleuth:
@RestController
public class OrderController {
private static final Logger logger = LoggerFactory.getLogger(OrderController.class);
@Autowired
private RestTemplate restTemplate;
@GetMapping("/order/{id}")
public Order getOrder(@PathVariable String id) {
logger.info("Fetching order details for id: {}", id);
// Trace ID and Span ID are automatically included in logs
return restTemplate.getForObject("http://payment-service/payment/" + id, Order.class);
}
}
Testing and Quality Assurance
Advanced testing techniques are crucial for maintaining code quality. This section covers various testing approaches and best practices.
Property-Based Testing
Here’s an example using JUnit QuickCheck for property-based testing:
public class StringReverseTest {
@Property
public void reverseReverseShouldBeOriginal(@ForAll String original) {
String reversed = new StringBuilder(original).reverse().toString();
String doubleReversed = new StringBuilder(reversed).reverse().toString();
assertEquals(original, doubleReversed);
}
}
Integration Testing
Example of integration testing with TestContainers:
@Testcontainers
public class DatabaseIntegrationTest {
@Container
private static final PostgreSQLContainer<?> postgres = new PostgreSQLContainer<>("postgres:13")
.withDatabaseName("test-db")
.withUsername("test")
.withPassword("test");
@Test
void testDatabaseConnection() {
try (Connection conn = DriverManager.getConnection(postgres.getJdbcUrl(),
postgres.getUsername(),
postgres.getPassword())) {
assertTrue(conn.isValid(5));
}
}
}
Security Best Practices
Security is paramount in enterprise applications. Understanding security best practices and common vulnerabilities is essential for senior developers.
Secure Coding Practices
Example of secure password hashing using BCrypt:
public class PasswordUtils {
private static final int STRENGTH = 10;
public static String hashPassword(String plainTextPassword) {
return BCrypt.hashpw(plainTextPassword, BCrypt.gensalt(STRENGTH));
}
public static boolean verifyPassword(String plainTextPassword, String hashedPassword) {
return BCrypt.checkpw(plainTextPassword, hashedPassword);
}
}
OAuth 2.0 Implementation
Example of implementing OAuth 2.0 with Spring Security:
@Configuration
@EnableResourceServer
public class OAuth2ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.antMatchers("/public/**").permitAll()
.and()
.csrf().disable();
}
}
Disclaimer: The code examples and explanations provided in this blog post are meant for educational purposes and may need to be adapted based on specific requirements and contexts. While we strive for accuracy, technology evolves rapidly, and some information may become outdated. Please report any inaccuracies to our editorial team, and we will update the content promptly. Always refer to official documentation and conduct thorough testing before implementing these concepts in production environments.