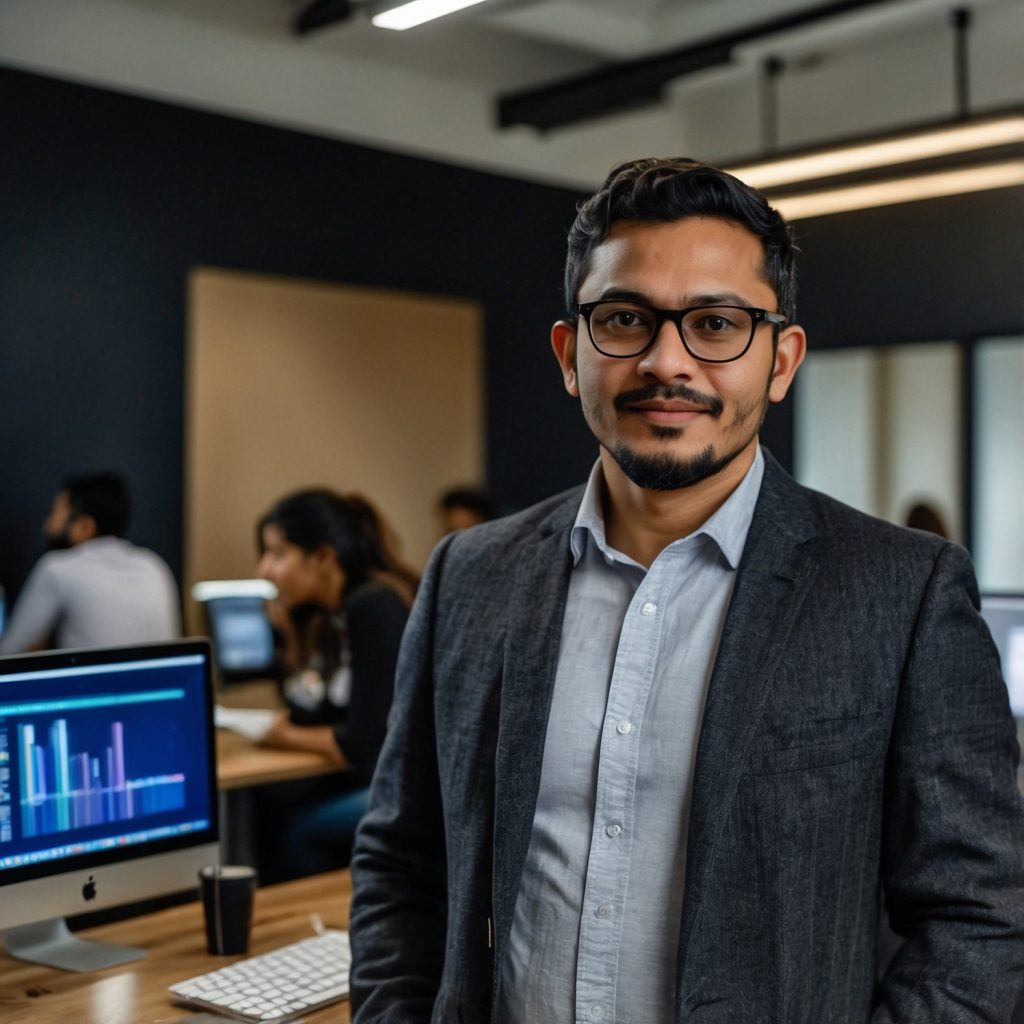
An Introduction to Algorithm Design
Algorithm design is a fundamental aspect of computer science and software engineering, forming the backbone of how problems are solved and tasks are automated. This blog aims to introduce you to the world of algorithms, their importance, and how to design them effectively. We’ll cover the basics, provide practical examples, and explore some common algorithms used in programming.
What is an Algorithm?
Defining an Algorithm
An algorithm is a step-by-step procedure or formula for solving a problem. It consists of a finite set of instructions, which, when executed, produce a solution to a given problem. Algorithms are essential in programming as they define the logic for problem-solving and task automation.
Importance of Algorithms
Algorithms are crucial because they provide a clear method for solving problems efficiently. They help in optimizing performance, managing resources, and ensuring that tasks are completed in a reasonable amount of time. Understanding and designing algorithms can significantly improve the efficiency of your code.
Basic Example of an Algorithm
Consider a simple algorithm to add two numbers:
def add_two_numbers(a, b):
return a + b
# Example usage
result = add_two_numbers(3, 5)
print(result) # Output: 8
This basic example illustrates the concept of an algorithm: a series of steps (in this case, addition) that solve a specific problem (adding two numbers).
Characteristics of a Good Algorithm
Efficiency
A good algorithm should be efficient in terms of both time and space. Time efficiency refers to the amount of time an algorithm takes to complete, while space efficiency refers to the amount of memory it uses.
Correctness
An algorithm must be correct, meaning it should produce the expected output for all possible inputs. This involves rigorous testing and validation to ensure accuracy.
Readability
The algorithm should be easy to understand and maintain. Clear, well-documented code helps others (and yourself) to grasp the logic and purpose of the algorithm quickly.
Generality
A robust algorithm should be general enough to handle a variety of inputs and edge cases. This ensures the algorithm can be applied to a wide range of problems.
Designing Algorithms: A Step-by-Step Guide
Step 1: Understand the Problem
Before designing an algorithm, it’s essential to thoroughly understand the problem you’re trying to solve. This involves identifying the inputs, outputs, and any constraints or requirements.
Example: Finding the Maximum Number in a List
Let’s design an algorithm to find the maximum number in a list of integers.
Step 2: Plan the Algorithm
Planning involves outlining the steps needed to solve the problem. For our example, the steps might include:
- Initialize a variable to hold the maximum value.
- Iterate through each number in the list.
- Compare each number to the current maximum value.
- Update the maximum value if a larger number is found.
- Return the maximum value after completing the iteration.
Step 3: Write the Algorithm
Convert the plan into actual code. Here’s how the algorithm looks in Python:
def find_maximum(numbers):
if not numbers:
return None # Handle the edge case of an empty list
max_number = numbers[0]
for number in numbers:
if number > max_number:
max_number = number
return max_number
# Example usage
numbers = [3, 5, 7, 2, 8, 1]
print(find_maximum(numbers)) # Output: 8
Step 4: Analyze the Algorithm
Evaluate the algorithm’s efficiency and correctness. Our find_maximum
function is linear in time complexity (O(n)), as it iterates through the list once. It is also correct, as it returns the largest number in the list.
Common Algorithmic Paradigms
Divide and Conquer
This paradigm involves breaking a problem into smaller subproblems, solving each subproblem, and combining their solutions to solve the original problem. A classic example is the merge sort algorithm.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i = j = 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [3, 9, 10, 27, 38, 43, 82]
Dynamic Programming
Dynamic programming is used to solve problems by breaking them down into simpler subproblems and storing the results of these subproblems to avoid redundant calculations. An example is the Fibonacci sequence.
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n - 1) + fibonacci(n - 2)
return memo[n]
# Example usage
print(fibonacci(10)) # Output: 55
Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. An example is the algorithm for finding the minimum number of coins for a given amount of money.
def min_coins(coins, amount):
coins.sort(reverse=True)
coin_count = 0
for coin in coins:
while amount >= coin:
amount -= coin
coin_count += 1
return coin_count
# Example usage
coins = [1, 2, 5, 10, 20, 50, 100]
amount = 93
print(min_coins(coins, amount)) # Output: 5
Practical Applications of Algorithms
Sorting and Searching
Algorithms for sorting (like quicksort and mergesort) and searching (like binary search) are fundamental in computer science. They are used in various applications, from database indexing to improving the efficiency of other algorithms.
Graph Algorithms
Graph algorithms, such as Dijkstra’s shortest path algorithm and Kruskal’s minimum spanning tree algorithm, are essential for network analysis, routing, and many optimization problems.
Machine Learning Algorithms
Algorithms play a crucial role in machine learning. Examples include decision trees, neural networks, and clustering algorithms like k-means. These algorithms help in making predictions, classifications, and discovering patterns in data.
Tips for Learning and Implementing Algorithms
Start with Simple Problems
Begin with basic problems to build your understanding. Practice writing algorithms for common tasks like sorting, searching, and basic arithmetic operations.
Understand the Theory
Grasp the theoretical concepts behind algorithms, such as time complexity (Big O notation), space complexity, and algorithmic paradigms. This theoretical knowledge is essential for designing efficient algorithms.
Practice Coding
Implement algorithms in your preferred programming language. Regular practice will help you become proficient and confident in writing algorithms.
Analyze and Optimize
Always analyze your algorithms for efficiency and correctness. Look for ways to optimize your code, such as reducing time complexity or improving readability.
Study Advanced Algorithms
Once you’re comfortable with basic algorithms, explore more advanced topics like dynamic programming, graph theory, and machine learning algorithms. These advanced topics will deepen your understanding and open up new possibilities in problem-solving.
Algorithm design is a critical skill for any programmer or computer scientist. By understanding the basics, practicing regularly, and exploring advanced topics, you can master the art of algorithm design. This not only makes you a better problem solver but also enhances the performance and efficiency of your code. Whether you’re sorting data, finding the shortest path, or making predictions, algorithms are at the heart of it all. Happy coding!