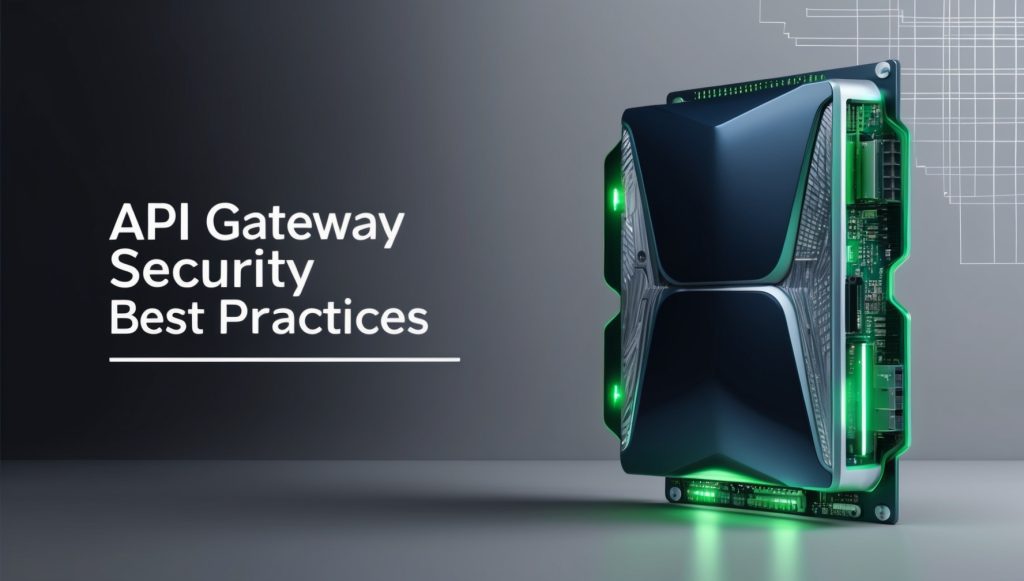
API Gateway Security Best Practices
In today’s interconnected digital landscape, APIs have become the backbone of modern applications, enabling seamless communication between different systems and services. But with great power comes great responsibility – and in this case, that responsibility is ensuring the security of your API gateway. As the central point of entry for all API traffic, your gateway is both your first line of defense and a potential Achilles’ heel if not properly secured. So, buckle up as we dive deep into the world of API gateway security best practices, exploring everything from authentication methods to rate limiting and beyond.
The API Gateway: Your Digital Bouncer
Before we jump into the nitty-gritty of security practices, let’s take a moment to understand what an API gateway is and why it’s so crucial. Think of your API gateway as the bouncer at an exclusive club. It’s the first point of contact for anyone trying to access your APIs, and it’s responsible for deciding who gets in, who stays out, and what they’re allowed to do once they’re inside.
An API gateway acts as a reverse proxy, routing requests to the appropriate backend services. But it’s not just a simple traffic director. Oh no, it’s so much more! It’s a Swiss Army knife of functionality, capable of handling authentication, rate limiting, request/response transformation, monitoring, and analytics. In essence, it’s your API’s best friend and protector rolled into one.
Now that we’ve set the stage, let’s dive into the best practices that will turn your API gateway from a flimsy screen door into an impenetrable fortress.
Authentication: The VIP List of API Security
OAuth 2.0 and OpenID Connect: The Dynamic Duo
When it comes to API authentication, OAuth 2.0 and OpenID Connect (OIDC) are the cool kids on the block. OAuth 2.0 provides a framework for authorizing third-party applications to access resources on behalf of a user, while OIDC adds an identity layer on top of OAuth 2.0. Together, they form a powerful duo that can handle a wide range of authentication scenarios.
Implementing OAuth 2.0 and OIDC in your API gateway allows you to offload the complex task of authentication and authorization to specialized identity providers. This not only improves security but also enhances user experience by enabling single sign-on (SSO) capabilities. Here’s a quick example of how you might configure an API gateway to use OAuth 2.0:
@Configuration
public class APIGatewayConfig {
@Bean
public SecurityWebFilterChain springSecurityFilterChain(ServerHttpSecurity http) {
http
.authorizeExchange()
.pathMatchers("/public/**").permitAll()
.anyExchange().authenticated()
.and()
.oauth2ResourceServer()
.jwt();
return http.build();
}
}
This configuration sets up a Spring Cloud Gateway to use OAuth 2.0 with JWT tokens for authentication. It allows public access to paths starting with “/public/” and requires authentication for all other routes.
API Keys: The Old Reliable
While OAuth 2.0 and OIDC are great for complex scenarios, sometimes you just need a simple, no-frills approach to authentication. Enter API keys. These long, unique strings act like a password for your API, allowing you to identify and authenticate clients quickly and easily.
API keys are perfect for server-to-server communication or scenarios where you don’t need fine-grained access control. They’re easy to implement and manage, but remember – with great simplicity comes great responsibility. Always transmit API keys securely (over HTTPS) and implement proper key rotation policies to minimize the risk of compromised keys.
Here’s a simple example of how you might validate an API key in your gateway:
@Component
public class APIKeyFilter implements GlobalFilter {
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
String apiKey = request.getHeaders().getFirst("X-API-Key");
if (isValidApiKey(apiKey)) {
return chain.filter(exchange);
} else {
exchange.getResponse().setStatusCode(HttpStatus.UNAUTHORIZED);
return exchange.getResponse().setComplete();
}
}
private boolean isValidApiKey(String apiKey) {
// Implement your API key validation logic here
}
}
This filter checks for the presence of a valid API key in the “X-API-Key” header and rejects requests with invalid or missing keys.
Authorization: The Velvet Rope of Access Control
Authentication is just the beginning. Once you know who’s knocking at your API’s door, you need to decide what they’re allowed to do inside. This is where authorization comes into play.
Role-Based Access Control (RBAC): The Classic Approach
RBAC is like assigning different backstage passes at a concert. Depending on your role (fan, VIP, band member, etc.), you get access to different areas. In the API world, roles might include “read-only”, “editor”, or “admin”, each with its own set of permissions.
Implementing RBAC in your API gateway allows you to centralize access control decisions, making it easier to manage and audit permissions across your entire API ecosystem. Here’s a simple example of how you might implement RBAC in a Spring Cloud Gateway:
@Configuration
public class APIGatewayConfig {
@Bean
public SecurityWebFilterChain springSecurityFilterChain(ServerHttpSecurity http) {
http
.authorizeExchange()
.pathMatchers("/public/**").permitAll()
.pathMatchers("/api/admin/**").hasRole("ADMIN")
.pathMatchers("/api/editor/**").hasAnyRole("EDITOR", "ADMIN")
.anyExchange().authenticated()
.and()
.oauth2ResourceServer()
.jwt();
return http.build();
}
}
This configuration sets up different access rules based on the user’s role, ensuring that only users with the appropriate permissions can access certain endpoints.
Attribute-Based Access Control (ABAC): The New Kid on the Block
While RBAC is great for many scenarios, sometimes you need more flexibility. That’s where ABAC comes in. ABAC allows you to make access decisions based on a wide range of attributes, including user attributes, resource attributes, and environmental conditions.
For example, you might want to allow access to certain resources only during business hours, or restrict access based on the user’s location. ABAC gives you the power to implement these complex access control scenarios with ease.
Implementing ABAC in your API gateway can be a bit more complex than RBAC, but the flexibility it offers can be well worth the effort. Here’s a simplified example of how you might implement ABAC using Spring Security:
@Component
public class ABACFilter implements GlobalFilter {
@Autowired
private ABACPolicyEnforcer policyEnforcer;
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
Authentication authentication = ReactiveSecurityContextHolder.getContext()
.map(SecurityContext::getAuthentication)
.block();
if (policyEnforcer.isAllowed(authentication, request)) {
return chain.filter(exchange);
} else {
exchange.getResponse().setStatusCode(HttpStatus.FORBIDDEN);
return exchange.getResponse().setComplete();
}
}
}
@Component
public class ABACPolicyEnforcer {
public boolean isAllowed(Authentication authentication, ServerHttpRequest request) {
// Implement your ABAC policy logic here
// Consider user attributes, resource attributes, and environmental conditions
}
}
This example shows a custom filter that uses an ABAC policy enforcer to make access control decisions based on various attributes.
Rate Limiting: The Bouncer’s Clipboard
Imagine if a nightclub let everyone in at once – chaos would ensue! The same principle applies to your API. Rate limiting is like the bouncer’s clipboard, controlling the flow of traffic to prevent abuse and ensure fair usage.
Token Bucket Algorithm: The Classic Approach
The token bucket algorithm is a simple yet effective way to implement rate limiting. Think of it as giving each client a bucket of tokens. Each API request consumes a token, and the bucket refills at a steady rate. Once the bucket is empty, further requests are denied until more tokens become available.
Here’s a simple implementation of the token bucket algorithm in Java:
public class TokenBucket {
private final long capacity;
private final double refillRate;
private double tokens;
private long lastRefillTimestamp;
public TokenBucket(long capacity, double refillRate) {
this.capacity = capacity;
this.refillRate = refillRate;
this.tokens = capacity;
this.lastRefillTimestamp = System.nanoTime();
}
public synchronized boolean tryConsume(int numTokens) {
refill();
if (tokens < numTokens) {
return false;
}
tokens -= numTokens;
return true;
}
private void refill() {
long now = System.nanoTime();
double tokensToAdd = (now - lastRefillTimestamp) * refillRate / 1e9;
tokens = Math.min(capacity, tokens + tokensToAdd);
lastRefillTimestamp = now;
}
}
You can integrate this token bucket implementation into your API gateway to enforce rate limits on a per-client basis.
Adaptive Rate Limiting: The Smart Bouncer
While fixed rate limiting is effective, it can sometimes be too rigid. Adaptive rate limiting takes things a step further by dynamically adjusting limits based on current system load, time of day, or other factors. It’s like having a bouncer who can read the room and adjust the entry rate accordingly.
Implementing adaptive rate limiting requires more complex logic and monitoring of your system’s resources. Here’s a simplified example of how you might implement adaptive rate limiting:
public class AdaptiveRateLimiter {
private final TokenBucket tokenBucket;
private final LoadMonitor loadMonitor;
public AdaptiveRateLimiter(long initialCapacity, double initialRefillRate, LoadMonitor loadMonitor) {
this.tokenBucket = new TokenBucket(initialCapacity, initialRefillRate);
this.loadMonitor = loadMonitor;
}
public boolean tryConsume(int numTokens) {
adjustRateLimit();
return tokenBucket.tryConsume(numTokens);
}
private void adjustRateLimit() {
double currentLoad = loadMonitor.getCurrentLoad();
double adjustmentFactor = calculateAdjustmentFactor(currentLoad);
tokenBucket.setRefillRate(tokenBucket.getRefillRate() * adjustmentFactor);
}
private double calculateAdjustmentFactor(double currentLoad) {
// Implement your adjustment logic here
// This could be based on predefined thresholds or more complex algorithms
}
}
public interface LoadMonitor {
double getCurrentLoad();
}
This adaptive rate limiter adjusts the refill rate of the token bucket based on the current system load, allowing for more flexible and responsive rate limiting.
Input Validation: The Security Pat-Down
Just as a bouncer might pat down guests for prohibited items, your API gateway needs to thoroughly inspect incoming requests for potential threats. Input validation is your first line of defense against a wide range of attacks, including injection attacks, cross-site scripting (XSS), and more.
Schema Validation: The Checklist
Schema validation is like having a detailed checklist of what’s allowed in your API requests. By defining a schema for your API inputs, you can automatically reject requests that don’t meet your specified criteria. This not only improves security but also helps maintain data integrity and reduces the load on your backend services.
Here’s an example of how you might implement schema validation using JSON Schema and the Everit JSON Schema library:
import org.everit.json.schema.Schema;
import org.everit.json.schema.loader.SchemaLoader;
import org.json.JSONObject;
import org.json.JSONTokener;
public class SchemaValidator {
private final Schema schema;
public SchemaValidator(String schemaJson) {
JSONObject rawSchema = new JSONObject(new JSONTokener(schemaJson));
this.schema = SchemaLoader.load(rawSchema);
}
public void validate(String jsonData) throws ValidationException {
JSONObject data = new JSONObject(new JSONTokener(jsonData));
schema.validate(data);
}
}
// Usage in API Gateway filter
@Component
public class SchemaValidationFilter implements GlobalFilter {
private final SchemaValidator validator;
public SchemaValidationFilter() {
String schemaJson = // Load your JSON Schema here
this.validator = new SchemaValidator(schemaJson);
}
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
return exchange.getRequest().getBody()
.map(dataBuffer -> dataBuffer.toString(StandardCharsets.UTF_8))
.flatMap(body -> {
try {
validator.validate(body);
return chain.filter(exchange);
} catch (ValidationException e) {
exchange.getResponse().setStatusCode(HttpStatus.BAD_REQUEST);
return exchange.getResponse().setComplete();
}
});
}
}
This example shows how to create a schema validator and use it in an API gateway filter to validate incoming request bodies against a predefined JSON Schema.
Content-Based Validation: The Smart Inspector
While schema validation is great for structured data, sometimes you need to go deeper. Content-based validation involves inspecting the actual content of requests for potential threats. This might include checking for SQL injection attempts, validating email addresses, or sanitizing HTML input to prevent XSS attacks.
Here’s an example of how you might implement some basic content-based validation:
public class ContentValidator {
private static final Pattern EMAIL_PATTERN =
Pattern.compile("^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$");
private static final Pattern SQL_INJECTION_PATTERN =
Pattern.compile("(?i)(\\b(SELECT|INSERT|UPDATE|DELETE|FROM|WHERE|DROP)\\b)");
public static boolean isValidEmail(String email) {
return EMAIL_PATTERN.matcher(email).matches();
}
public static boolean containsSqlInjection(String input) {
return SQL_INJECTION_PATTERN.matcher(input).find();
}
public static String sanitizeHtml(String html) {
return Jsoup.clean(html, Whitelist.basic());
}
}
// Usage in API Gateway filter
@Component
public class ContentValidationFilter implements GlobalFilter {
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
String email = request.getQueryParams().getFirst("email");
String searchTerm = request.getQueryParams().getFirst("search");
if (email != null && !ContentValidator.isValidEmail(email)) {
exchange.getResponse().setStatusCode(HttpStatus.BAD_REQUEST);
return exchange.getResponse().setComplete();
}
if (searchTerm != null && ContentValidator.containsSqlInjection(searchTerm)) {
exchange.getResponse().setStatusCode(HttpStatus.BAD_REQUEST);
return exchange.getResponse().setComplete();
}
return chain.filter(exchange);
}
}
This example demonstrates basic email validation and SQL injection detection. In a real-world scenario, you’d want to implement more comprehensive validation logic and possibly use specialized libraries for tasks like HTML sanitization.
Encryption: The Invisible Shield
While authentication and authorization control who can access your API, encryption ensures that the data flowing through your API gateway remains confidential and tamper-proof. It’s like having an invisible shield protecting your API traffic from prying eyes and malicious actors.
Transport Layer Security (TLS): The Baseline
TLS (formerly known as SSL) is the foundation of secure communication on the internet. It provides encryption, data integrity, and authentication between clients and servers. Implementing TLS in your API gateway is non-negotiable –
it’s the bare minimum you should be doing to protect your API traffic.
When implementing TLS in your API gateway, consider the following best practices:
- Use the latest version of TLS (currently TLS 1.3) and disable older, vulnerable versions.
- Configure strong cipher suites and key exchange mechanisms.
- Implement HSTS (HTTP Strict Transport Security) to prevent downgrade attacks.
- Use properly signed certificates from trusted Certificate Authorities.
Here’s an example of how you might configure TLS in a Spring Cloud Gateway:
@Configuration
public class TLSConfig {
@Bean
public SecurityWebFilterChain springSecurityFilterChain(ServerHttpSecurity http) {
http
.redirectToHttps()
.httpsRedirectWhen(e -> e.getRequest().getHeaders().containsKey("X-Forwarded-Proto"))
.and()
.headers()
.hsts()
.includeSubdomains(true)
.maxAge(Duration.ofDays(365));
return http.build();
}
}
This configuration enables HTTPS redirect and HSTS with a one-year max-age and includeSubdomains flag.
End-to-End Encryption: The Fort Knox Approach
While TLS secures the connection between the client and your API gateway, what about the communication between your gateway and backend services? For highly sensitive data, consider implementing end-to-end encryption. This ensures that data remains encrypted even as it passes through your API gateway.
Implementing end-to-end encryption can be complex, but here’s a simplified example of how you might handle encrypted payloads in your API gateway:
public class EncryptionService {
private final Key secretKey;
public EncryptionService(String secretKey) {
this.secretKey = new SecretKeySpec(secretKey.getBytes(), "AES");
}
public String encrypt(String data) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedBytes = cipher.doFinal(data.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public String decrypt(String encryptedData) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedData));
return new String(decryptedBytes);
}
}
@Component
public class EncryptionFilter implements GlobalFilter {
@Autowired
private EncryptionService encryptionService;
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
return request.getBody().map(dataBuffer -> {
try {
String body = dataBuffer.toString(StandardCharsets.UTF_8);
String decryptedBody = encryptionService.decrypt(body);
return new ServerHttpRequestDecorator(request) {
@Override
public Flux<DataBuffer> getBody() {
return Flux.just(exchange.getResponse().bufferFactory().wrap(decryptedBody.getBytes()));
}
};
} catch (Exception e) {
throw new RuntimeException("Failed to decrypt request body", e);
}
}).flatMap(chain::filter);
}
}
This example demonstrates a basic encryption service and a filter that decrypts incoming request bodies. In a real-world scenario, you’d need to handle key management, implement proper error handling, and consider performance implications.
Logging and Monitoring: The All-Seeing Eye
A secure API gateway isn’t just about preventing attacks – it’s also about detecting and responding to them quickly. Comprehensive logging and monitoring are like having an all-seeing eye watching over your API traffic, ready to alert you at the first sign of trouble.
Structured Logging: The Organized Approach
Structured logging involves recording log events in a consistent, machine-readable format. This makes it much easier to search, analyze, and visualize your log data. When implementing logging in your API gateway, consider capturing the following information:
- Request metadata (timestamp, IP address, user agent, etc.)
- Authentication and authorization decisions
- Rate limiting events
- Input validation results
- Response status codes and times
Here’s an example of how you might implement structured logging using the SLF4J and Logstash Logback encoder:
import net.logstash.logback.argument.StructuredArguments;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Component
public class StructuredLoggingFilter implements GlobalFilter {
private static final Logger logger = LoggerFactory.getLogger(StructuredLoggingFilter.class);
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
ServerHttpRequest request = exchange.getRequest();
long startTime = System.currentTimeMillis();
return chain.filter(exchange).then(Mono.fromRunnable(() -> {
ServerHttpResponse response = exchange.getResponse();
long duration = System.currentTimeMillis() - startTime;
logger.info("API Request",
StructuredArguments.keyValue("method", request.getMethod()),
StructuredArguments.keyValue("path", request.getPath()),
StructuredArguments.keyValue("status", response.getStatusCode()),
StructuredArguments.keyValue("duration", duration),
StructuredArguments.keyValue("clientIp", request.getRemoteAddress())
);
}));
}
}
This filter logs structured information about each API request, including the HTTP method, path, response status, request duration, and client IP address.
Real-time Monitoring: The Vigilant Guardian
While logs are great for post-mortem analysis, real-time monitoring allows you to detect and respond to issues as they happen. Consider implementing the following monitoring strategies:
- Health checks: Regularly ping your API endpoints to ensure they’re responsive.
- Performance metrics: Monitor response times, error rates, and resource utilization.
- Anomaly detection: Use machine learning algorithms to identify unusual patterns in API traffic.
- Alerting: Set up notifications for critical events or threshold breaches.
Here’s a simple example of how you might implement basic health checks and performance monitoring using Spring Boot Actuator and Micrometer:
@Configuration
public class MonitoringConfig {
@Bean
public TimedAspect timedAspect(MeterRegistry registry) {
return new TimedAspect(registry);
}
}
@RestController
@Timed
public class ApiController {
private final MeterRegistry meterRegistry;
public ApiController(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
@GetMapping("/api/data")
public ResponseEntity<String> getData() {
meterRegistry.counter("api.requests", "endpoint", "getData").increment();
// Your API logic here
return ResponseEntity.ok("Data");
}
}
This configuration enables timing of all methods in the ApiController
and manually increments a counter for each request to the /api/data
endpoint. You can then use tools like Prometheus and Grafana to visualize and alert on these metrics.
Putting It All Together: The Holistic Approach
Securing your API gateway isn’t about implementing a single solution – it’s about layering multiple security measures to create a robust, resilient system. Think of it as building a medieval castle: you have the outer walls (TLS), the gatehouse (authentication and authorization), the moat (rate limiting), the guards (input validation), and the watchtowers (logging and monitoring).
Here’s a high-level overview of how all these components might work together in a secure API gateway:
- TLS Termination: All incoming requests are decrypted at the gateway level.
- Authentication: Requests are authenticated using OAuth 2.0, API keys, or other methods.
- Rate Limiting: Authenticated requests are checked against rate limits.
- Input Validation: Request payloads are validated against schemas and checked for malicious content.
- Authorization: The gateway checks if the authenticated user has permission to access the requested resource.
- Logging: All of the above steps are logged for auditing and analysis.
- Backend Routing: If all checks pass, the request is routed to the appropriate backend service.
- Response Handling: The response from the backend is logged and returned to the client.
- Monitoring: Throughout this process, various metrics are collected and analyzed in real-time.
Implementing this level of security requires careful planning, thorough testing, and ongoing maintenance. But the payoff – a secure, reliable API gateway that protects your valuable data and services – is well worth the effort.
Stay Vigilant, Stay Secure
Securing your API gateway is not a one-time task – it’s an ongoing process that requires constant vigilance and adaptation. As new threats emerge and your API ecosystem evolves, your security measures must evolve with them.
Remember, security is only as strong as its weakest link. Regularly audit your API gateway security, conduct penetration testing, and stay up-to-date with the latest security best practices and vulnerabilities. By treating security as a core feature of your API gateway, rather than an afterthought, you can build a robust foundation for your digital services that will stand the test of time – and the onslaught of would-be attackers.
So, go forth and secure those gateways! Your data, your users, and your future self will thank you.
Disclaimer: While we strive to provide accurate and up-to-date information, the field of cybersecurity is constantly evolving. The practices described in this article should be considered as general guidelines and may need to be adapted to your specific use case and security requirements. Always consult with security professionals and stay informed about the latest developments in API security. If you notice any inaccuracies in this post, please report them so we can correct them promptly.