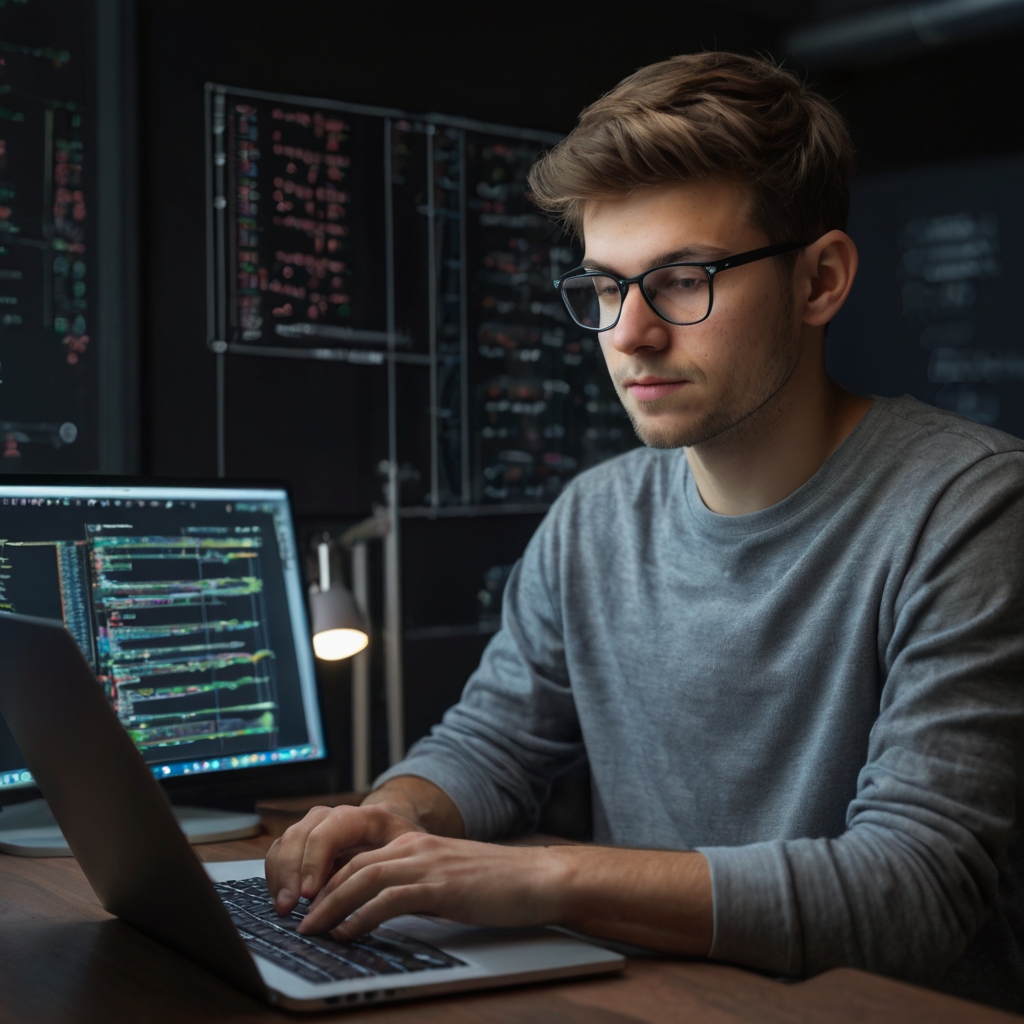
Applying Separation of Concerns in Java
Separation of Concerns (SoC) is a fundamental principle in software design that promotes the division of a software solution into distinct, self-contained components, each addressing a specific aspect or functionality. By adhering to SoC principles, Java developers can create modular, maintainable, and extensible software solutions that are easier to understand, modify, and scale. In this blog post, we’ll explore the concept of SoC in Java development, discuss its benefits, and provide practical tips and examples to help you harness the power of SoC in your projects.
Understanding Separation of Concerns
Separation of Concerns (SoC) is a design principle that seeks to break down a software system into distinct components or modules, each responsible for a specific aspect of the system’s functionality. This separation allows developers to work on individual components independently, making the overall system easier to understand, maintain, and extend. SoC is closely related to other software design principles, such as modularity, encapsulation, and the Single Responsibility Principle (SRP), which are all aimed at creating well-structured and organized code.
Benefits of Separation of Concerns
Implementing SoC in Java development offers several benefits:
- Improved maintainability: By separating concerns, developers can create more focused and self-contained components, making it easier to understand, modify, and maintain the code.
- Enhanced modularity: SoC promotes modularity, enabling developers to create reusable and interchangeable components that can be easily combined and extended to build complex software systems.
- Increased scalability: A well-structured system with separated concerns is easier to scale, as individual components can be independently modified or extended without affecting the rest of the system.
- Simplified testing: Separation of concerns allows developers to test individual components in isolation, simplifying the testing process and improving overall software quality.
Tips for Implementing Separation of Concerns in Java Development
To effectively implement SoC in your Java projects, consider the following tips:
- Organize code by functionality: Structure your Java code by functionality or domain, grouping related classes and interfaces into packages. This approach makes it easier to locate, understand, and modify the code related to a specific concern.
- Use object-oriented principles: Leverage object-oriented programming concepts, such as encapsulation, inheritance, and polymorphism, to create modular and self-contained components that adhere to SoC principles.
- Apply design patterns: Design patterns are reusable solutions to common problems in software design that can help you achieve SoC in your Java code. By incorporating design patterns, you can create well-structured and maintainable software solutions.
- Leverage Java frameworks: Java offers a variety of frameworks, such as Spring and Java EE, that support SoC through features like dependency injection and aspect-oriented programming. By using these frameworks, you can more easily implement SoC in your projects.
- Follow best practices: Adopt Java best practices, such as adhering to established naming conventions and code organization, to make your code more consistent, maintainable, and in line with SoC principles.
Practical Examples of Separation of Concerns in Java
To illustrate SoC in Java development, let’s examine a few practical examples:
- Model-View-Controller (MVC) pattern: The MVC pattern is a popular design pattern for building user interfaces that separates an application into three main components: Model, View, and Controller. By applying the MVC pattern in your Java projects, you can achieve SoC and create modular, maintainable, and extensible software solutions.
// Model
public class Customer {
private String name;
private String email;
// Getters and setters
}
// View
public class CustomerView {
public void displayCustomerDetails(String name, String email) {
System.out.println("Customer: " + name);
System.out.println("Email: " + email);
}
}
// Controller
public class CustomerController {
private Customer model;
private CustomerView view;
public CustomerController(Customer model, CustomerView view) {
this.model = model;
this.view = view;
}
// Methods to update the model and view
}
// Usage
Customer model = new Customer();
CustomerView view = new CustomerView();
CustomerController controller = new CustomerController(model, view);
Layered architecture: A layered architecture is a common approach to organizing software systems into distinct layers, such as presentation, business logic, and data access. By implementing a layered architecture in your Java projects, you can separate concerns and create more maintainable and scalable software solutions.
// Data access layer
public interface CustomerRepository {
Customer findById(long id);
void save(Customer customer);
}
// Business logic layer
public class CustomerService {
private CustomerRepository repository;
public CustomerService(CustomerRepository repository) {
this.repository = repository;
}
public Customer getCustomer(long id) {
return repository.findById(id);
}
public void updateCustomer(Customer customer) {
repository.save(customer);
}
}
// Presentation layer
public class CustomerController {
private CustomerService service;
public CustomerController(CustomerService service) {
this.service = service;
}
public void displayCustomer(long id) {
Customer customer = service.getCustomer(id);
// Display customer details
}
}
Balancing Separation of Concerns with Other Design Concerns
While SoC is an important goal in Java development, it’s essential to balance separation of concerns with other software design considerations, such as readability, maintainability, and performance. Striving for SoC at all costs can lead to overly abstract or complex code, which may be difficult to understand, maintain, or optimize. By considering the specific needs and requirements of your project, you can make informed decisions about when and how to prioritize separation of concerns.
Separation of Concerns (SoC) is a powerful principle in software design that can help Java developers create modular, maintainable, and extensible software solutions. By embracing SoC principles and best practices, you can build software that is easier to understand, modify, and scale, resulting in more robust and efficient solutions.
To effectively implement SoC in your Java projects, remember to:
- Organize code by functionality to create a well-structured system.
- Use object-oriented principles to create modular and self-contained components.
- Apply design patterns to enhance SoC and maintainability.
- Leverage Java frameworks that support SoC, such as Spring and Java EE.
- Follow best practices to ensure consistent, maintainable, and SoC-compliant code.
By mastering Separation of Concerns and incorporating it into your Java development practices, you can build high-quality, modular, and maintainable software solutions that stand the test of time.