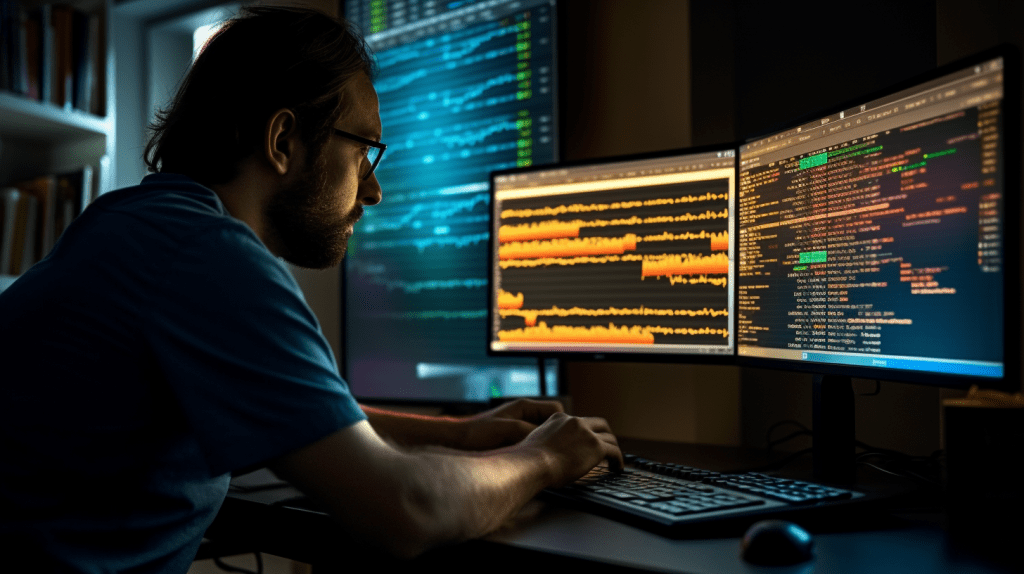
Applying the DRY Principle in Java
In Java development, the DRY (Don’t Repeat Yourself) principle is a fundamental concept that helps developers write efficient, maintainable, and reusable code. This principle, introduced by Andy Hunt and Dave Thomas in their book “The Pragmatic Programmer,” emphasizes the importance of eliminating duplication and improving overall software design. In this blog post, we’ll explore the DRY principle in Java development and showcase various techniques to ensure your code remains clean, maintainable, and scalable.
Understanding the DRY Principle
The DRY principle asserts that every piece of code should have a single, unambiguous representation within a system. In simpler terms, it means avoiding code duplication, where the same logic or functionality is repeated in multiple parts of the software. Adhering to the DRY principle ensures that your code is easier to understand, refactor, and maintain, and reduces the risk of introducing bugs or inconsistencies.
Leveraging Methods to Encapsulate Common Logic
One of the most straightforward techniques for adhering to the DRY principle in Java is to encapsulate common logic within methods. By creating a method that handles a specific functionality, you can reuse it across your application without duplicating code. Here’s an example:
Calculating interest for different types of accounts.
public abstract class BankAccount {
protected double balance;
// Encapsulates common logic in a method
protected double calculateInterest(double rate) {
return balance * rate / 100;
}
}
class SavingsAccount extends BankAccount {
private static final double INTEREST_RATE = 2.5;
public double addInterest() {
double interest = calculateInterest(INTEREST_RATE);
balance += interest;
return interest;
}
}
class FixedDepositAccount extends BankAccount {
private static final double INTEREST_RATE = 5.0;
public double addInterest() {
double interest = calculateInterest(INTEREST_RATE);
balance += interest;
return interest;
}
}
Explanation: The calculateInterest
method encapsulates the common logic for interest calculation, which is reused by both SavingsAccount
and FixedDepositAccount
.
Utilizing Inheritance and Polymorphism
Inheritance and polymorphism are powerful object-oriented programming (OOP) concepts that help developers adhere to the DRY principle in Java. By creating base classes with common functionality and extending them in derived classes, you can eliminate code duplication and promote code reuse.
Processing different transaction types.
public abstract class Transaction {
protected double amount;
public Transaction(double amount) {
this.amount = amount;
}
// Polymorphic method to be implemented by subclasses
public abstract void process(BankAccount account);
}
class DepositTransaction extends Transaction {
public DepositTransaction(double amount) {
super(amount);
}
@Override
public void process(BankAccount account) {
account.deposit(amount);
}
}
class WithdrawalTransaction extends Transaction {
public WithdrawalTransaction(double amount) {
super(amount);
}
@Override
public void process(BankAccount account) {
account.withdraw(amount);
}
}
Explanation: Transaction
is an abstract class that provides a common structure for different transaction types. DepositTransaction
and WithdrawalTransaction
inherit from Transaction
and implement the process
method differently, demonstrating polymorphism.
Employing Design Patterns
Design patters provide proven solutions to common software design problems, often adhering to the DRY principle. By leveraging design patterns in your Java development, you can minimize code duplication and create maintainable, scalable, and efficient software.
For instance, let’s look at the Singleton pattern, which ensures that a class has only one instance and provides a global point of access to it:
Singleton pattern for a Database Connection
public class DatabaseConnection {
private static DatabaseConnection instance;
private DatabaseConnection() {
// private constructor to prevent instantiation
}
public static synchronized DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
public void executeQuery(String query) {
// Implementation for executing a query on the database
}
}
Explanation: The Singleton pattern ensures that only one instance of the DatabaseConnection
class is created, providing a global point of access to it. This is useful for managing resources like database connections.
Adopting Template Method Pattern
The Template Method pattern is another design pattern that helps adhere to the DRY principle in Java development. This pattern defines the skeleton of an algorithm in a base class, allowing subclasses to override specific steps without changing the algorithm’s overall structure.
Defining a template for running banking operations
public abstract class BankingOperation {
// Template method
public final void executeOperation() {
preOperation();
performOperation();
postOperation();
}
protected void preOperation() {
// Default implementation (can be overridden)
}
protected abstract void performOperation(); // Specific operation implementation
protected void postOperation() {
// Default implementation (can be overridden)
}
}
class TransferOperation extends BankingOperation {
@Override
protected void performOperation() {
// Logic for transferring funds between accounts
}
@Override
protected void preOperation() {
// Transfer-specific pre-operation steps
}
@Override
protected void postOperation() {
// Transfer-specific post-operation steps
}
}
Explanation: The Template Method Pattern defines the skeleton of an algorithm in a method, deferring some steps to subclasses. BankingOperation
defines a template method executeOperation
that includes hooks (preOperation
and postOperation
) and an abstract method performOperation
for the core operation. Subclasses like TransferOperation
implement these methods according to their specific requirements.
Violating DRY Principle
Before refactoring, the code snippet might look something like this, where similar code is repeated for different types of banking transactions:
public class BankAccount {
private double balance;
// Constructor, getters and setters omitted for brevity
public void deposit(double amount) {
balance += amount;
// Log deposit info
System.out.println("Deposited: " + amount);
// Perform additional deposit-related operations...
}
public void withdraw(double amount) {
if(balance >= amount) {
balance -= amount;
// Log withdrawal info
System.out.println("Withdrawn: " + amount);
// Perform additional withdrawal-related operations...
} else {
System.out.println("Insufficient funds");
}
}
// Assume more methods with similar logging and operations for other transactions
}
Refactoring to adhere to DRY Principle
To adhere to the DRY principle, we can refactor the code by abstracting the repetitive logging and operations into separate methods. This not only makes the code cleaner but also easier to maintain and update.
public class BankAccount {
private double balance;
// Constructor, getters and setters omitted for brevity
public void deposit(double amount) {
balance += amount;
logTransaction("Deposited", amount);
performAdditionalOperations();
}
public void withdraw(double amount) {
if(balance >= amount) {
balance -= amount;
logTransaction("Withdrawn", amount);
performAdditionalOperations();
} else {
System.out.println("Insufficient funds");
}
}
private void logTransaction(String transactionType, double amount) {
// Centralized logging for transactions
System.out.println(transactionType + ": " + amount);
}
private void performAdditionalOperations() {
// Placeholder for additional common operations after transactions
}
// Now, any new transaction method can reuse logTransaction and performAdditionalOperations
}
Explanation
In the refactored version, logTransaction
and performAdditionalOperations
methods centralize the repetitive tasks of logging and performing additional operations post-transaction, which were previously duplicated in each transaction method (deposit
and withdraw
). This approach makes the code more maintainable, as changes to the logging or additional operations need to be made in only one place, reducing the risk of errors and inconsistencies.
This example simplifies the complexities of real production code in a retail banking system but illustrates the core of the DRY principle: abstracting and reusing code to reduce redundancy, making it easier to maintain and extend.
Embracing Code Refactoring
Code refactoring is an essential practice in Java development to ensure adherence to the DRY principle. Regularly reviewing and improving your codebase helps identify areas of duplication and opportunities to consolidate logic. Tools such as IntelliJ IDEA and Eclipse offer built-in support for identifying and resolving code duplicates.
The DRY principle is a powerful concept that helps Java developers write clean, maintainable, and efficient code. By understanding and implementing this principle, you’ll be better equipped to tackle complex software design challenges and produce high-quality applications. Through the use of methods, inheritance, polymorphism, design patterns, and regular code refactoring, you can ensure that your Java code remains DRY, scalable, and robust, setting the foundation for successful software development projects.