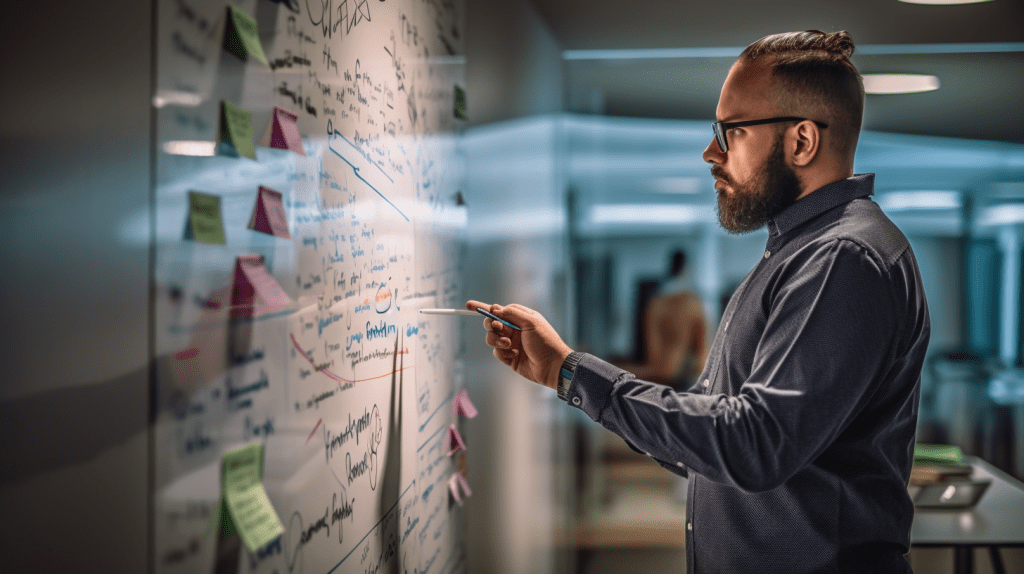
Applying the SOLID Principles in Java
SOLID principles have become an essential tool for Java developers in designing and building scalable, maintainable, and efficient software solutions. These five principles, created by Robert C. Martin, are a set of guidelines that ensure object-oriented programming (OOP) best practices, improving code readability and modularity. In this blog post, we’ll delve deep into the SOLID principles and explore how they apply to Java development to create robust software designs.
Single Responsibility Principle (SRP)
The first SOLID principle, the Single Responsibility Principle, dictates that a class should have only one reason to change. In other words, a class should be responsible for a single piece of functionality. This principle promotes code cohesion and reduces coupling, leading to easier maintenance and refactoring.
In Java, we can achieve SRP by breaking down complex classes into smaller ones, each handling a specific functionality. Here’s an example:
Code Violating SRP
In this example, a single class handles both account transactions and account data persistence, which violates the SRP.
// Violates SRP
public class AccountService {
public void deposit(Account account, double amount) {
account.balance += amount;
saveAccount(account);
System.out.println("Deposit of " + amount + " made to account " + account.getId());
}
public void withdraw(Account account, double amount) throws Exception {
if (account.balance >= amount) {
account.balance -= amount;
saveAccount(account);
System.out.println("Withdrawal of " + amount + " made from account " + account.getId());
} else {
throw new Exception("Insufficient funds");
}
}
public void saveAccount(Account account) {
// Logic to save account data to a database
System.out.println("Account saved to database");
}
}
This AccountService
class violates the SRP because it is responsible for both managing account transactions and handling account data persistence. This design flaw means the class has more than one reason to change: changes to the transaction logic or the persistence mechanism will both require modifications to the AccountService
.
Code Adhering to SRP
To adhere to the SRP, we refactor the code into two separate classes, each with a single responsibility.
public class AccountTransactionService {
public void deposit(Account account, double amount) {
account.balance += amount;
System.out.println("Deposit of " + amount + " made to account " + account.getId());
}
public void withdraw(Account account, double amount) throws Exception {
if (account.balance >= amount) {
account.balance -= amount;
System.out.println("Withdrawal of " + amount + " made from account " + account.getId());
} else {
throw new Exception("Insufficient funds");
}
}
}
public class AccountPersistenceService {
public void saveAccount(Account account) {
// Logic to save account data to a database
System.out.println("Account saved to database");
}
}
The initial AccountService
class violated the SRP by combining the responsibilities of handling account transactions (deposits and withdrawals) with the responsibility of persisting account changes to a database. This coupling of functionalities meant the class had multiple reasons to change: changes in the logic of transactions or in the persistence mechanism would necessitate modifications to this class.
By refactoring, we separated the concerns into two distinct classes. AccountTransactionService
now focuses solely on account transactions, encapsulating the business logic of deposits and withdrawals. AccountPersistenceService
is dedicated to the persistence of account data, handling interactions with the database. This separation means that changes to how transactions are processed or how accounts are saved will affect only the relevant class, aligning with the SRP. Each class now has one reason to change: transaction rules for AccountTransactionService
and persistence mechanism for AccountPersistenceService
.
This adherence to the SRP makes the system more maintainable and understandable, as each class is focused on a single responsibility. It facilitates easier updates and bug fixes since changes are isolated to specific areas of the codebase.
Open-Closed Principle (OCP)
The Open-Closed Principle states that classes should be open for extension but closed for modification. It encourages developers to extend existing functionality through inheritance or composition rather than altering the original class. This principle minimizes the risk of introducing bugs and promotes the reusability of code.
In Java, we can apply OCP by using inheritance, interfaces, and abstract classes. Here’s an example:
Without Applying OCP
Suppose we have a simple system that calculates interest for accounts, but it’s not designed with OCP in mind. This means that when new account types are introduced, the system needs to be modified.
public class InterestCalculator {
public double calculateInterest(Account account) {
switch (account.getType()) {
case SAVINGS:
return account.getBalance() * 0.03; // 3% interest for savings accounts
case CHECKING:
return account.getBalance() * 0.01; // 1% interest for checking accounts
// If a new account type is added, this code needs to be modified.
default:
throw new IllegalArgumentException("Unknown account type");
}
}
}
Refactored Scenario
To adhere to the OCP, we refactor the system to allow adding new account types and their interest calculation methods without modifying the existing code. We use polymorphism and define a common interface for interest calculation.
public interface InterestCalculationStrategy {
double calculateInterest(Account account);
}
public class SavingsAccountInterestCalculation implements InterestCalculationStrategy {
@Override
public double calculateInterest(Account account) {
return account.getBalance() * 0.03; // 3% interest
}
}
public class CheckingAccountInterestCalculation implements InterestCalculationStrategy {
@Override
public double calculateInterest(Account account) {
return account.getBalance() * 0.01; // 1% interest
}
}
public class Account {
private double balance;
private InterestCalculationStrategy interestCalculationStrategy;
public Account(double balance, InterestCalculationStrategy strategy) {
this.balance = balance;
this.interestCalculationStrategy = strategy;
}
public double calculateInterest() {
return interestCalculationStrategy.calculateInterest(this);
}
public double getBalance() {
return balance;
}
// Other account methods...
}
The initial InterestCalculator
class directly implements interest calculation logic within a switch
statement. This design requires the class to be modified each time a new type of account is introduced, violating the OCP.
The refactored design introduces an InterestCalculationStrategy
interface, with different strategies for different account types (e.g., SavingsAccountInterestCalculation
, CheckingAccountInterestCalculation
). The Account
class now uses a reference to InterestCalculationStrategy
for interest calculation. This design adheres to the OCP, as new interest calculation strategies can be added without modifying existing classes. To introduce a new account type, one would simply implement a new InterestCalculationStrategy
and instantiate the Account
with it, without changing any of the existing code.
The refactored design is a clear application of the OCP. It allows the Retail Banking System to be extended with new types of accounts and interest calculation methods without needing to alter the existing codebase, thus making the system more robust, scalable, and easier to maintain.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP) is a fundamental concept in object-oriented programming that states objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. It emphasizes that a subclass should override the base class methods in a way that does not alter the expected behavior of the base class.
In Java, we can adhere to LSP by using method overriding cautiously and following the “Design by Contract” concept. Here’s an example:
Violating LSP
Let’s start with an example that violates LSP in the context of a Retail Banking System, focusing on account withdrawals:
public class Account {
protected double balance;
public Account(double balance) {
this.balance = balance;
}
public void withdraw(double amount) throws Exception {
if (balance >= amount) {
balance -= amount;
} else {
throw new Exception("Insufficient funds");
}
}
public double getBalance() {
return balance;
}
}
public class FixedDepositAccount extends Account {
public FixedDepositAccount(double balance) {
super(balance);
}
@Override
public void withdraw(double amount) throws Exception {
throw new Exception("Withdrawals are not allowed from a fixed deposit account");
}
}
In this example, FixedDepositAccount
overrides the withdraw
method of its superclass Account
to throw an exception because withdrawals are not typically allowed from fixed deposit accounts. This violates LSP because FixedDepositAccount
objects cannot be used as a direct substitute for Account
objects without altering the behavior of the program, especially in contexts expecting a successful withdrawal operation.
Adhering to LSP
To adhere to LSP, we should refactor the design to ensure that subclasses can be used interchangeably with their base class without changing the program’s behavior. One way to do this is by establishing a more flexible class hierarchy that correctly represents the capabilities of different account types:
public abstract class Account {
protected double balance;
public Account(double balance) {
this.balance = balance;
}
public abstract void withdraw(double amount) throws Exception;
public double getBalance() {
return balance;
}
}
public class WithdrawableAccount extends Account {
public WithdrawableAccount(double balance) {
super(balance);
}
@Override
public void withdraw(double amount) throws Exception {
if (balance >= amount) {
balance -= amount;
} else {
throw new Exception("Insufficient funds");
}
}
}
public class FixedDepositAccount extends Account {
public FixedDepositAccount(double balance) {
super(balance);
}
@Override
public void withdraw(double amount) throws Exception {
throw new Exception("Withdrawals are not allowed from a fixed deposit account");
}
}
The first example violates LSP because FixedDepositAccount
changes the behavior of the withdraw
method in such a way that it can no longer be used interchangeably with its superclass Account
. Clients using Account
references would not expect a withdraw
operation to always throw an exception, as is the case with FixedDepositAccount
.
In the refactored example, we adhere to LSP by clearly distinguishing between accounts that allow withdrawals (WithdrawableAccount
) and those that do not (FixedDepositAccount
). This design makes the capabilities and restrictions of each account type explicit. Instead of expecting all account types to support withdrawal operations, we define a base class Account
with an abstract withdraw
method, allowing subclasses to provide their own implementations. This setup ensures that when a subclass is used in place of a superclass, it behaves in a manner consistent with the superclass’s intended behavior.
The adherence to LSP in the refactored example allows for more flexible and maintainable code, where subclasses can be substituted for their superclass without unexpected behavior, adhering to the principle’s core objective.
Interface Segregation Principle (ISP)
The Interface Segregation Principle (ISP), one of the SOLID principles, suggests that no client should be forced to depend on methods it does not use. In simpler terms, instead of having one large interface, it’s often better to have several smaller interfaces, so that clients only need to know about the methods that are of interest to them.
Let’s imagine a Retail Banking System where various operations like account management, loan processing, and customer notifications are handled. In a system that does not adhere to the ISP, you might have a large, monolithic interface that combines all these responsibilities. This can lead to issues where a class implementing this interface has to provide implementations for methods it doesn’t need or use.
To demonstrate the ISP let’s examine the following code snippets:
Before Applying ISP
Let’s start with a non-ISP-compliant interface:
public interface BankingOperations {
void openAccount();
void closeAccount();
void processLoan();
void approveLoan();
void sendNotification();
}
An AccountManager
class implementing this interface might look like this, even if it doesn’t need all the methods:
public class AccountManager implements BankingOperations {
@Override
public void openAccount() {
// Implementation
}
@Override
public void closeAccount() {
// Implementation
}
// These methods are not relevant to AccountManager
@Override
public void processLoan() {
throw new UnsupportedOperationException();
}
@Override
public void approveLoan() {
throw new UnsupportedOperationException();
}
@Override
public void sendNotification() {
// Implementation
}
}
After Applying ISP
Now, let’s refactor this by segregating the BankingOperations
interface into smaller, more specific interfaces:
public interface AccountService {
void openAccount();
void closeAccount();
}
public interface LoanService {
void processLoan();
void approveLoan();
}
public interface NotificationService {
void sendNotification();
}
The AccountManager
can now implement only the interfaces relevant to it:
public class AccountManager implements AccountService, NotificationService {
@Override
public void openAccount() {
// Implementation
}
@Override
public void closeAccount() {
// Implementation
}
@Override
public void sendNotification() {
// Implementation
}
}
A LoanProcessor
class might look like this:
public class LoanProcessor implements LoanService {
@Override
public void processLoan() {
// Implementation
}
@Override
public void approveLoan() {
// Implementation
}
}
Benefits
- Decoupling: Classes are not forced to implement methods they don’t use.
- Flexibility: It’s easier to add new features or modify existing ones without affecting unrelated parts of the system.
- Maintainability: Smaller, focused interfaces are easier to understand, implement, and maintain.
This example illustrates how adhering to the Interface Segregation Principle can make a system more modular, easier to understand, and maintain, especially in complex domains like retail banking.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) is one of the SOLID principles of object-oriented design, which states two key things:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
This principle helps in decoupling the software modules, making the system more flexible and easier to maintain and extend.
To demonstrate how it works, Imagine a retail banking system that needs to process customer transactions, such as deposits and withdrawals, and then log these transactions. Without adhering to DIP, the high-level module (e.g., transaction processing) might directly depend on low-level modules (e.g., a specific logging mechanism or a specific database). This tight coupling makes it hard to change the logging mechanism or switch to a different data storage system without modifying the transaction processing code.
Before Applying DIP
Here’s how you might have a transaction processing system without DIP:
// Low-level module
public class DatabaseLogger {
public void log(String message) {
// Log message to a database
System.out.println("Logging to database: " + message);
}
}
// High-level module
public class TransactionProcessor {
private DatabaseLogger logger = new DatabaseLogger();
public void processTransaction(String transactionDetails) {
// Process the transaction...
System.out.println("Processing transaction: " + transactionDetails);
// Logging directly with the low-level logging mechanism
logger.log(transactionDetails);
}
}
After Applying DIP
To adhere to DIP, we introduce abstractions for both the logging mechanism and the transaction processing logic, allowing them to depend on these abstractions rather than concrete implementations.
// Abstraction for the logging
public interface Logger {
void log(String message);
}
// Concrete implementation of Logger
public class DatabaseLogger implements Logger {
@Override
public void log(String message) {
// Log message to a database
System.out.println("Logging to database: " + message);
}
}
// Another concrete implementation of Logger
public class FileLogger implements Logger {
@Override
public void log(String message) {
// Log message to a file
System.out.println("Logging to file: " + message);
}
}
// High-level module with dependency on abstraction
public class TransactionProcessor {
private Logger logger;
// Dependency is injected, rather than hard-coded
public TransactionProcessor(Logger logger) {
this.logger = logger;
}
public void processTransaction(String transactionDetails) {
// Process the transaction...
System.out.println("Processing transaction: " + transactionDetails);
// Use the injected logger
logger.log(transactionDetails);
}
}
Usage
This allows you to easily switch between logging strategies without changing the TransactionProcessor
class.
public class BankingApplication {
public static void main(String[] args) {
// Using a database logger
TransactionProcessor processorWithDbLogger = new TransactionProcessor(new DatabaseLogger());
processorWithDbLogger.processTransaction("Deposit: 100");
// Switching to a file logger without modifying TransactionProcessor
TransactionProcessor processorWithFileLogger = new TransactionProcessor(new FileLogger());
processorWithFileLogger.processTransaction("Withdrawal: 50");
}
}
Benefits
By following DIP, the TransactionProcessor
(a high-level module) doesn’t depend directly on DatabaseLogger
or FileLogger
(low-level modules). Instead, it depends on the Logger
interface, an abstraction that can be fulfilled by any concrete implementation. This makes the system more flexible and maintainable, allowing changes to the logging mechanism without affecting the transaction processing logic.
Incorporating SOLID principles into Java development can significantly improve the quality of your software design. By adhering to these principles, developers can create maintainable, scalable, and efficient solutions that stand the test of time. With a deeper understanding of SOLID principles, you’ll be well on your way to mastering object-oriented programming in Java and crafting robust software designs.