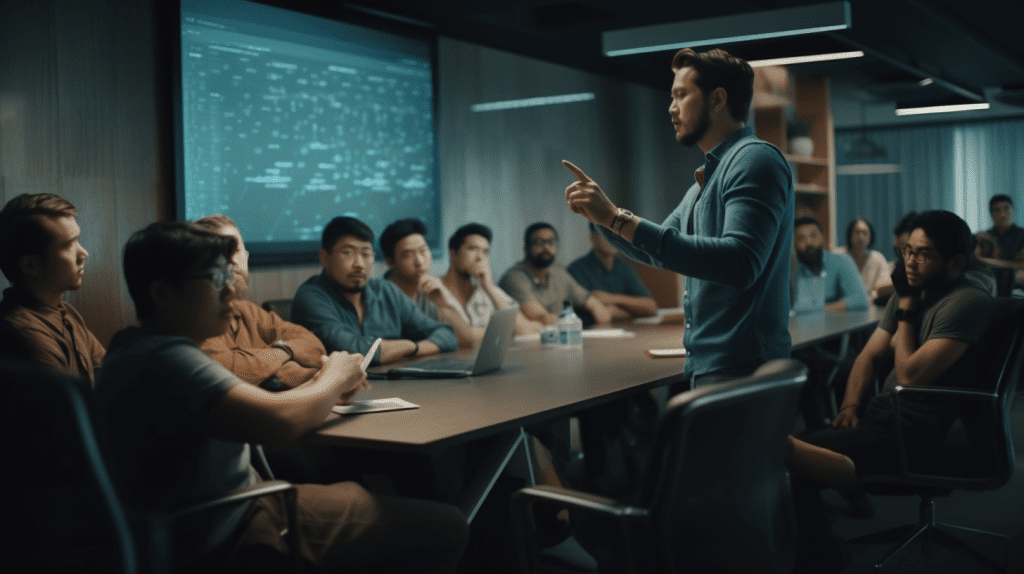
Applying the YAGNI Principle in Java
As a Java developer, it’s essential to understand and apply the YAGNI (You Aren’t Gonna Need It) principle to create efficient, maintainable, and agile software solutions. This principle emphasizes the importance of focusing on current requirements and avoiding unnecessary features or complexity. In this blog post, we’ll explore the YAGNI principle in Java, discuss its benefits, and share practical tips for applying this essential software design principle in your projects.
Understanding the YAGNI Principle
The YAGNI principle asserts that developers should only implement functionality that is currently required and avoid adding features or complexity based on speculative future needs. By adhering to this principle, Java developers can create software that is easier to understand, maintain, and extend. YAGNI is closely related to other software development principles such as KISS (Keep It Simple, Stupid) and DRY (Don’t Repeat Yourself), which also advocate for simplicity and a focus on the essential aspects of a software solution.
Benefits of the YAGNI Principle
Applying the YAGNI principle in Java development offers several benefits:
- Reduced complexity: By focusing on current requirements, developers can create simpler and more manageable code, reducing the likelihood of bugs and making the software easier to understand and maintain.
- Faster development: By avoiding unnecessary features, developers can save time and effort, allowing them to deliver software more quickly and efficiently.
- Improved adaptability: When future requirements change, software built with the YAGNI principle is more adaptable, as it contains fewer dependencies and less complexity to be reworked or removed.
- Lower cost: By eliminating unneeded features and complexity, developers can reduce development and maintenance costs, providing a more cost-effective solution for their clients or organizations.
Tips for Applying the YAGNI
To effectively apply the YAGNI principle in your Java projects, consider the following tips:
- Focus on current requirements: When planning and implementing features, concentrate on the present needs of the software and avoid the temptation to add functionality based on speculative future requirements. This approach ensures that your code remains simple, focused, and relevant to the problem at hand.
- Use agile methodologies: Agile methodologies, such as Scrum or Kanban, complement the YAGNI principle by promoting iterative development and continuous improvement. By embracing these methodologies, developers can focus on delivering small increments of functionality that meet current requirements, rather than trying to predict and implement future features.
- Prioritize refactoring: As new requirements emerge, it’s essential to refactor your code to maintain simplicity and avoid accumulating technical debt. By prioritizing refactoring, you can ensure that your software remains maintainable, adaptable, and aligned with current needs.
- Communicate with stakeholders: Maintain open communication with project stakeholders, such as clients or product owners, to ensure that you have a clear understanding of the current requirements and can avoid implementing unnecessary features. By fostering a collaborative environment, you can align your development efforts with the actual needs of the project and ensure that the software delivers the desired value.
- Embrace simplicity in design: When designing your Java classes and interfaces, strive for simplicity and avoid introducing unnecessary abstractions or dependencies. By creating simple, focused components, you can ensure that your code remains easy to understand, maintain, and extend.
- Apply the KISS and DRY principles: The YAGNI principle goes hand-in-hand with other software design principles like KISS (Keep It Simple, Stupid) and DRY (Don’t Repeat Yourself). By adhering to these principles, you can further promote simplicity, maintainability, and efficiency in your Java code.
Example: Applying YAGNI
To illustrate the YAGNI principle in practice, let’s consider a simple example below.
Business Requirement
Create a system that allows a bank to manage customer accounts. Initially, the system needs to support creating a new account and checking the account balance.
Without Applying YAGNI Principle
In this non-YAGNI approach, we anticipate future features such as overdraft protection, loan management, and investment portfolios, even though the current requirement is simply to manage accounts and check balances.
class BankAccount {
private String accountNumber;
private double balance;
private double overdraftLimit; // Anticipating overdraft feature
private List<Loan> loans; // Anticipating loan management
private InvestmentPortfolio portfolio; // Anticipating investment feature
public BankAccount(String accountNumber) {
this.accountNumber = accountNumber;
this.balance = 0.0;
this.overdraftLimit = 0.0; // Default, not currently needed
this.loans = new ArrayList<>(); // Not currently needed
this.portfolio = new InvestmentPortfolio(); // Not currently needed
}
public void deposit(double amount) {
this.balance += amount;
}
public double getBalance() {
return balance;
}
// Placeholder methods for future features
public void applyForLoan(double amount) { /* ... */ }
public void investInStocks(String stockSymbol, int shares) { /* ... */ }
}
class Loan { /* ... */ }
class InvestmentPortfolio { /* ... */ }
Applying YAGNI Principle
Now, let’s focus only on the current requirements: creating an account and checking the balance, strictly applying the YAGNI principle.
class BankAccount {
private String accountNumber;
private double balance;
public BankAccount(String accountNumber) {
this.accountNumber = accountNumber;
this.balance = 0.0; // Starting balance for every new account
}
public void deposit(double amount) {
this.balance += amount; // Only necessary operation for now
}
public double getBalance() {
return balance; // Only required feature
}
}
Applying YAGNI helps in focusing development efforts on immediate requirements, ensuring a lean and maintainable codebase. As the system needs evolve, additional features can be incrementally added with a clear understanding of the requirements, thereby avoiding speculative generality.
Benefits of Applying YAGNI
- Simplicity: The YAGNI-compliant version is simpler and easier to understand, focusing solely on the current requirements.
- Maintainability: With fewer lines of code and less complexity, the system is easier to maintain and debug.
- Development Speed: Developers can deliver the required features faster since they are not working on unnecessary features.
- Reduced Risk: Each additional, unused feature introduces potential bugs and increases the testing scope. Focusing only on what’s necessary reduces these risks.
- Ease of Changes: Without the burden of unnecessary features, modifying the system to accommodate future requirements is easier and less risky.
Balancing YAGNI with Good Design
While the YAGNI principle promotes simplicity and a focus on current requirements, it’s essential to balance this approach with good software design practices. For example, adhering to the SOLID principles can help you create flexible, maintainable, and extensible code that is better prepared to accommodate future changes.
By combining the YAGNI principle with other software design principles, you can create Java software that is both simple and adaptable, providing the best possible solution for your project.
The YAGNI principle is a powerful guideline that can help Java developers create efficient, maintainable, and agile software solutions by focusing on current requirements and avoiding unnecessary complexity. By embracing the YAGNI principle in your Java development projects, you can ensure that your software remains relevant, adaptable, and cost-effective.
To effectively apply the YAGNI principle, remember to:
- Focus on current requirements and avoid speculative features.
- Use agile methodologies to promote iterative development and continuous improvement.
- Prioritize refactoring to maintain simplicity and avoid technical debt.
- Communicate with stakeholders to align development efforts with actual needs.
- Embrace simplicity in design and apply other software design principles, such as KISS and DRY.
By mastering the YAGNI principle and incorporating it into your Java development practices, you can build robust and efficient software solutions that stand the test of time. Always remember: You Aren’t Gonna Need It.