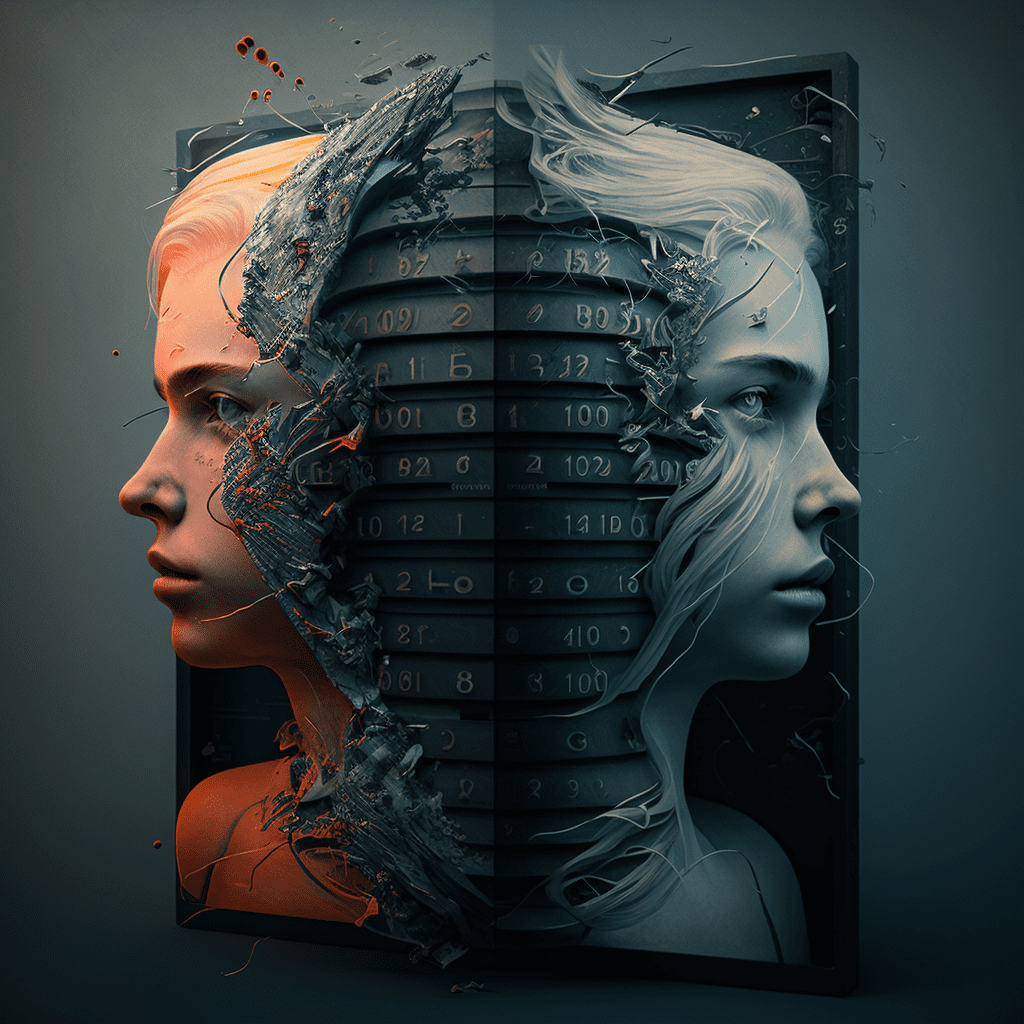
Array vs ArrayList Efficiency in Java: A Big O Notation Comparison
Arrays and ArrayLists are two of the most commonly used data structures in Java. Both are used to store collections of elements, but they have different characteristics that can impact their performance. In this article, we will discuss Array vs ArrayList Efficiency in Java: A Big O Notation Comparison.
What is Big O Notation?
Big O notation is a mathematical notation used to describe the time complexity of an algorithm. It represents the upper bound on the number of operations required to perform a given task, as the input size approaches infinity. In other words, it describes the worst-case scenario for the performance of an algorithm.
Arrays in Java
Arrays are a fixed-size data structure that stores a collection of elements of the same data type. They are created using a specified size and can be accessed using an index. Arrays have O(1) time complexity for accessing an element by index, since the location of the element in memory can be calculated using simple arithmetic.
However, arrays have some limitations that can impact their performance. They are fixed in size, which means that resizing an array requires creating a new array and copying the elements over. This can be an expensive operation, especially for large arrays. Additionally, inserting or deleting elements in the middle of an array requires shifting the elements, which can also be expensive.
ArrayLists in Java
ArrayLists are a dynamic data structure that can grow and shrink as needed. They are implemented using an array, but the size of the array can be increased or decreased as elements are added or removed. ArrayLists have O(1) time complexity for appending an element to the end of the list, since there is usually available space in the array.
However, ArrayLists have some overhead that can impact their performance. They must allocate new memory when they need to grow the array, which can be an expensive operation. Additionally, inserting or deleting elements in the middle of an ArrayList requires shifting the elements, which can also be expensive. This makes ArrayLists slower than arrays for random access, since each element must be traversed sequentially to reach a specific index.
Comparison of Array vs ArrayList Efficiency
Based on their characteristics, we can compare the efficiency of arrays and ArrayLists in Java using Big O notation:
Accessing an element by index: Both arrays and ArrayLists have O(1) time complexity for accessing an element by index.
Appending an element to the end: ArrayLists have O(1) time complexity for appending an element to the end, while arrays have O(n) time complexity since the array may need to be resized.
Inserting or deleting an element in the middle: Both arrays and ArrayLists have O(n) time complexity for inserting or deleting an element in the middle, since the elements may need to be shifted.
In general, arrays are more efficient than ArrayLists for accessing elements by index and appending elements to the end. However, ArrayLists are more flexible and efficient for adding or removing elements in the middle of the list.
Arrays and ArrayLists are both useful data structures in Java, but they have different characteristics that can impact their performance. When choosing between them, consider the specific requirements of your application and the trade-offs between performance and flexibility. By understanding the Big O notation for arrays and ArrayLists, you can make informed decisions about which data structure to use in your Java programs.