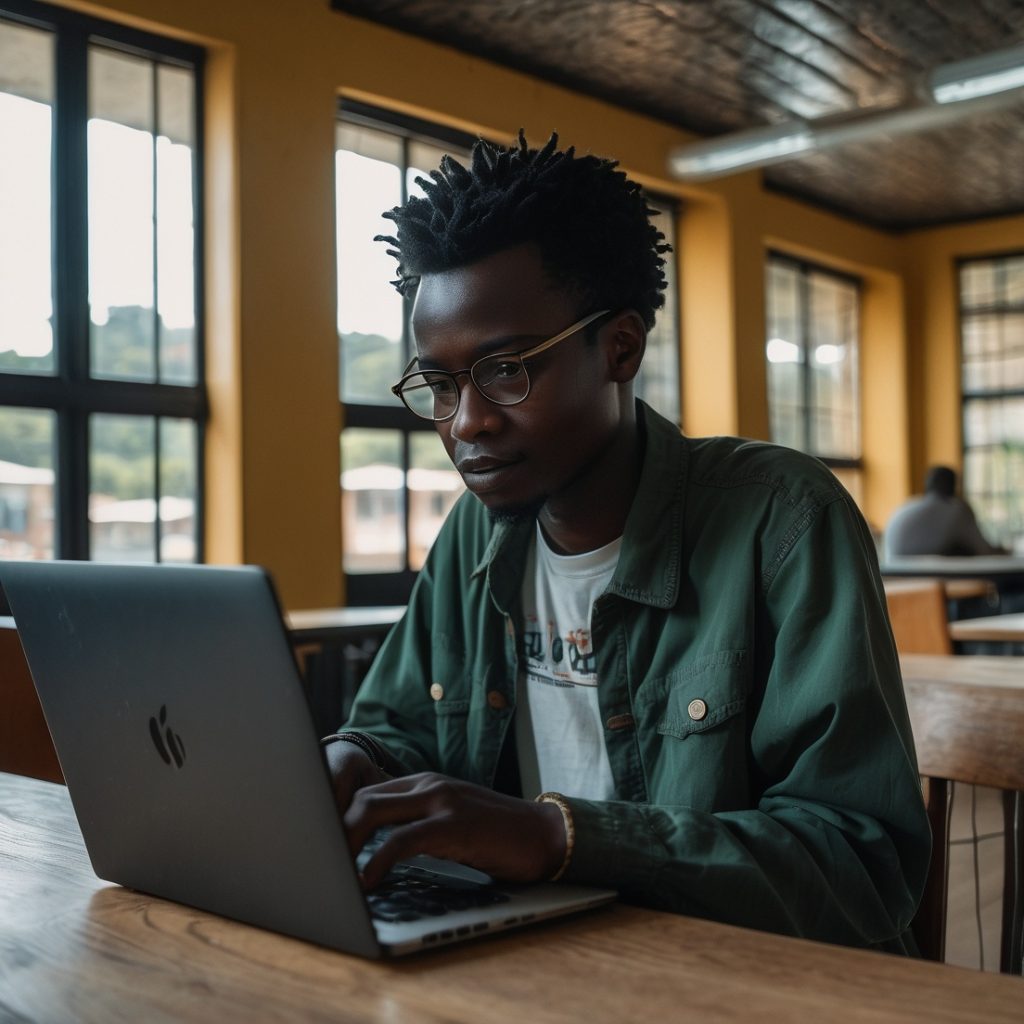
Async Awesomeness: Mastering Asynchronous Programming in Python
Asynchronous programming in Python can feel like diving into an ocean of unknown depths. But fear not, fellow coder! With the right guidance, we can navigate these waters and unlock the full potential of Python’s async capabilities. By the end of this journey, you’ll not only understand asynchronous programming but also wield it like a seasoned pro. So, grab your favorite beverage, settle in, and let’s embark on this adventure together.
What is Asynchronous Programming?
Before we dive into the code, let’s demystify what asynchronous programming actually is. In simple terms, asynchronous programming allows your program to handle multiple tasks at the same time. This is particularly useful for I/O-bound tasks, such as reading files, making network requests, or querying databases.
The Synchronous Way
In synchronous programming, tasks are executed one after the other. This means that if one task takes a long time to complete, the entire program waits for it to finish before moving on to the next task.
import time
def fetch_data():
print("Fetching data...")
time.sleep(2)
print("Data fetched!")
def process_data():
print("Processing data...")
time.sleep(3)
print("Data processed!")
def main():
fetch_data()
process_data()
print("All tasks completed.")
main()
In this example, fetch_data
and process_data
are executed sequentially. The program waits for each task to complete before starting the next one.
The Asynchronous Way
Asynchronous programming allows tasks to run concurrently, which can significantly improve the efficiency of your program.
import asyncio
async def fetch_data():
print("Fetching data...")
await asyncio.sleep(2)
print("Data fetched!")
async def process_data():
print("Processing data...")
await asyncio.sleep(3)
print("Data processed!")
async def main():
await asyncio.gather(fetch_data(), process_data())
print("All tasks completed.")
asyncio.run(main())
Here, fetch_data
and process_data
are executed concurrently. The await
keyword allows other tasks to run while waiting for the current task to complete.
Getting Started with asyncio
Python’s asyncio
library is the foundation of asynchronous programming. It provides a framework for writing single-threaded concurrent code using coroutines, event loops, and more.
Coroutines
A coroutine is a function that can be paused and resumed, making it the building block of asynchronous programming. Coroutines are defined using the async def
syntax.
import asyncio
async def say_hello():
print("Hello")
await asyncio.sleep(1)
print("World")
asyncio.run(say_hello())
In this example, say_hello
is a coroutine. The await
keyword pauses the execution of the coroutine, allowing other tasks to run in the meantime.
Event Loop
The event loop is responsible for executing asynchronous tasks and managing their states. You can think of it as a conductor orchestrating a symphony of tasks.
import asyncio
async def greet(name):
print(f"Hello, {name}!")
await asyncio.sleep(1)
print(f"Goodbye, {name}!")
async def main():
await asyncio.gather(greet("Alice"), greet("Bob"))
asyncio.run(main())
In this code, the greet
coroutine is executed for both “Alice” and “Bob” concurrently. The asyncio.gather
function is used to run multiple coroutines concurrently.
Handling I/O-bound Tasks
Asynchronous programming shines when dealing with I/O-bound tasks. Let’s explore how to perform network requests and file I/O asynchronously.
Asynchronous Network Requests
For network requests, you can use libraries like aiohttp
that are designed to work with asyncio
.
import aiohttp
import asyncio
async def fetch_url(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
async with aiohttp.ClientSession() as session:
html = await fetch_url(session, 'https://www.example.com')
print(html[:100]) # Print the first 100 characters of the response
asyncio.run(main())
Here, aiohttp
is used to fetch a URL asynchronously. The async with
syntax ensures that resources are properly managed.
Asynchronous File I/O
For file I/O, aiofiles
is a great library that provides asynchronous file handling.
import aiofiles
import asyncio
async def read_file(file_path):
async with aiofiles.open(file_path, 'r') as file:
contents = await file.read()
print(contents)
asyncio.run(read_file('example.txt'))
In this example, aiofiles
is used to read a file asynchronously, allowing other tasks to run while waiting for the file read operation to complete.
Error Handling in Asynchronous Code
Error handling is crucial in any programming paradigm, and asynchronous programming is no exception. Let’s look at how to handle errors gracefully in async code.
Catching Exceptions
You can use try-except blocks within coroutines to catch and handle exceptions.
import asyncio
async def might_fail():
await asyncio.sleep(1)
raise ValueError("Something went wrong")
async def main():
try:
await might_fail()
except ValueError as e:
print(f"Caught an exception: {e}")
asyncio.run(main())
Here, any exceptions raised within might_fail
are caught and handled in the main
coroutine.
Task Management
When dealing with multiple tasks, it’s important to handle exceptions for each task individually. You can use asyncio.gather
with the return_exceptions
parameter.
import asyncio
async def task1():
await asyncio.sleep(1)
raise ValueError("Task 1 failed")
async def task2():
await asyncio.sleep(2)
return "Task 2 succeeded"
async def main():
results = await asyncio.gather(task1(), task2(), return_exceptions=True)
for result in results:
if isinstance(result, Exception):
print(f"Caught an exception: {result}")
else:
print(result)
asyncio.run(main())
In this example, exceptions from task1
are caught and handled, while the result from task2
is processed normally.
Asynchronous Database Operations
Working with databases is another area where asynchronous programming can greatly improve performance. Libraries like asyncpg
and databases
provide support for asynchronous database operations.
Using asyncpg with PostgreSQL
asyncpg
is a fast PostgreSQL client library for Python that supports asynchronous operations.
import asyncpg
import asyncio
async def fetch_data():
conn = await asyncpg.connect('postgresql://user:password@localhost/dbname')
rows = await conn.fetch('SELECT * FROM my_table')
await conn.close()
for row in rows:
print(row)
asyncio.run(fetch_data())
In this code, asyncpg
is used to connect to a PostgreSQL database and fetch data asynchronously.
Using databases with SQLAlchemy
The databases
library provides an asynchronous interface for interacting with databases using SQLAlchemy.
from databases import Database
import asyncio
DATABASE_URL = "sqlite:///./test.db"
database = Database(DATABASE_URL)
async def fetch_data():
await database.connect()
query = "SELECT * FROM my_table"
rows = await database.fetch_all(query=query)
await database.disconnect()
for row in rows:
print(row)
asyncio.run(fetch_data())
Here, databases
is used to perform asynchronous database operations with SQLAlchemy.
Building Asynchronous Web Applications with FastAPI
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It’s designed to be easy to use and highly efficient.
Creating a Basic FastAPI Application
Let’s start by creating a simple FastAPI application that responds to HTTP requests asynchronously.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"message": "Hello, World!"}
@app.get("/items/{item_id}")
async def read_item(item_id: int, q: str = None):
return {"item_id": item_id, "q": q}
Running the Application
To run the FastAPI application, you can use uvicorn
, an ASGI server.
uvicorn myapp:app --reload
Replace myapp
with the name of your Python file. The --reload
flag enables auto-reload, so you don’t have to restart the server manually after making changes.
Connecting to a Database
FastAPI can be combined with async database libraries to handle database operations efficiently. Let’s connect FastAPI to a SQLite database using databases
.
from fastapi import FastAPI
from databases import Database
DATABASE_URL = "sqlite:///./test.db"
database = Database(DATABASE_URL)
app = FastAPI()
@app.on_event("startup")
async def startup():
await database.connect()
@app.on_event("shutdown")
async def shutdown():
await database.disconnect()
@app.get("/users/{user_id}")
async def read_user(user_id: int):
query = "SELECT * FROM users WHERE id = :user_id"
user = await database.fetch_one(query, values={"user_id": user_id})
return user
Asynchronous Testing
Testing asynchronous code requires some additional considerations. Fortunately, Python’s pytest
framework, combined with pytest-asyncio
, makes it straightforward to test asynchronous functions.
Setting Up pytest-asyncio
First, you’ll need to install pytest-asyncio
:
pip install pytest-asyncio
Writing Asynchronous Tests
Here’s an example of how to write and run tests for asynchronous functions using pytest-asyncio
:
import pytest
import asyncio
async def fetch_data():
await asyncio.sleep(1)
return "data"
@pytest.mark.asyncio
async def test_fetch_data():
result = await fetch_data()
assert result == "data"
In this example, pytest.mark.asyncio
is used to indicate that test_fetch_data
is an asynchronous test.
Testing FastAPI Endpoints
Testing FastAPI endpoints asynchronously can be done using the httpx
library, which is an HTTP client for Python that supports async requests.
from fastapi import FastAPI
from fastapi.testclient import TestClient
import pytest
app = FastAPI()
@app.get("/items/{item_id}")
async def read_item(item_id: int):
return {"item_id": item_id}
client = TestClient(app)
def test_read_item():
response = client.get("/items/42")
assert response.status_code == 200
assert response.json() == {"item_id": 42}
In this test, TestClient
from FastAPI’s testclient
module is used to send a synchronous request to the /items/{item_id}
endpoint and validate the response.
Debugging Asynchronous Code
Debugging asynchronous code can be challenging, but there are tools and techniques to help you troubleshoot effectively.
Using Logging
Logging is a powerful way to understand what’s happening in your code. The logging
module in Python provides a flexible framework for emitting log messages from your program.
import logging
import asyncio
logging.basicConfig(level=logging.INFO)
async def fetch_data():
logging.info("Fetching data...")
await asyncio.sleep(1)
logging.info("Data fetched")
return "data"
async def main():
result = await fetch_data()
logging.info(f"Result: {result}")
asyncio.run(main())
In this example, logging is used to track the progress of the fetch_data
coroutine.
Using Debuggers
Modern IDEs like PyCharm and Visual Studio Code support debugging asynchronous code. Set breakpoints and step through your code to see how coroutines are executed and how control is transferred between them.
Real-World Examples
To solidify our understanding, let’s look at some real-world scenarios where asynchronous programming can be beneficial.
Web Scraping with Asyncio and aiohttp
Web scraping often involves making many HTTP requests, which can be slow if done synchronously. Using aiohttp
with asyncio
, we can scrape websites concurrently.
import aiohttp
import asyncio
async def fetch_page(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = ["https://example.com/page1", "https://example.com/page2", "https://example.com/page3"]
async with aiohttp.ClientSession() as session:
tasks = [fetch_page(session, url) for url in urls]
pages = await asyncio.gather(*tasks)
for page in pages:
print(page[:100]) # Print the first 100 characters of each page
asyncio.run(main())
In this script, aiohttp
is used to fetch multiple web pages concurrently, significantly speeding up the web scraping process.
Chat Server with WebSockets
Asynchronous programming is ideal for real-time applications like chat servers. Using the websockets
library, we can create a simple chat server that handles multiple clients concurrently.
import asyncio
import websockets
clients = set()
async def handle_client(websocket, path):
clients.add(websocket)
try:
async for message in websocket:
await asyncio.gather(*(client.send(message) for client in clients if client != websocket))
finally:
clients.remove(websocket)
start_server = websockets.serve(handle_client, "localhost", 12345)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
In this example, the chat server broadcasts messages from each client to all other connected clients asynchronously.
Best Practices for Asynchronous Programming
Asynchronous programming can greatly improve the performance of your applications, but it’s important to follow best practices to avoid common pitfalls.
Avoid Blocking Code
Ensure that you don’t block the event loop with long-running synchronous operations. If you need to perform CPU-bound tasks, consider using concurrent.futures.ThreadPoolExecutor
to run them in a separate thread.
import asyncio
import concurrent.futures
def cpu_bound_task():
return sum(i * i for i in range(10000000))
async def main():
loop = asyncio.get_running_loop()
with concurrent.futures.ThreadPoolExecutor() as pool:
result = await loop.run_in_executor(pool, cpu_bound_task)
print(f"Result: {result}")
asyncio.run(main())
Use Timeouts
When performing I/O operations, always use timeouts to prevent your program from hanging indefinitely.
import aiohttp
import asyncio
async def fetch_url(session, url):
try:
async with session.get(url, timeout=10) as response:
return await response.text()
except asyncio.TimeoutError:
print(f"Timeout fetching {url}")
async def main():
async with aiohttp.ClientSession() as session:
html = await fetch_url(session, 'https://www.example.com')
if html:
print(html[:100])
asyncio.run(main())
Graceful Shutdown
Ensure that your application handles shutdowns gracefully, cleaning up any resources and closing connections properly.
import signal
import asyncio
async def main():
print("Running...")
def shutdown():
print("Shutting down...")
loop.stop()
loop = asyncio.get_event_loop()
loop.add_signal_handler(signal.SIGINT, shutdown)
try:
loop.run_until_complete(main())
finally:
loop.close()
Conclusion
Asynchronous programming in Python is a powerful tool that can greatly enhance the performance and responsiveness of your applications. By understanding the fundamentals of asyncio
, leveraging libraries like aiohttp
and aiofiles
, and following best practices, you can master asynchronous programming and take your Python skills to the next level.
From making network requests and handling I/O operations to building real-time web applications, asynchronous programming opens up a world of possibilities. So dive in, experiment with the examples provided, and embrace the async awesomeness in Python! Happy coding!