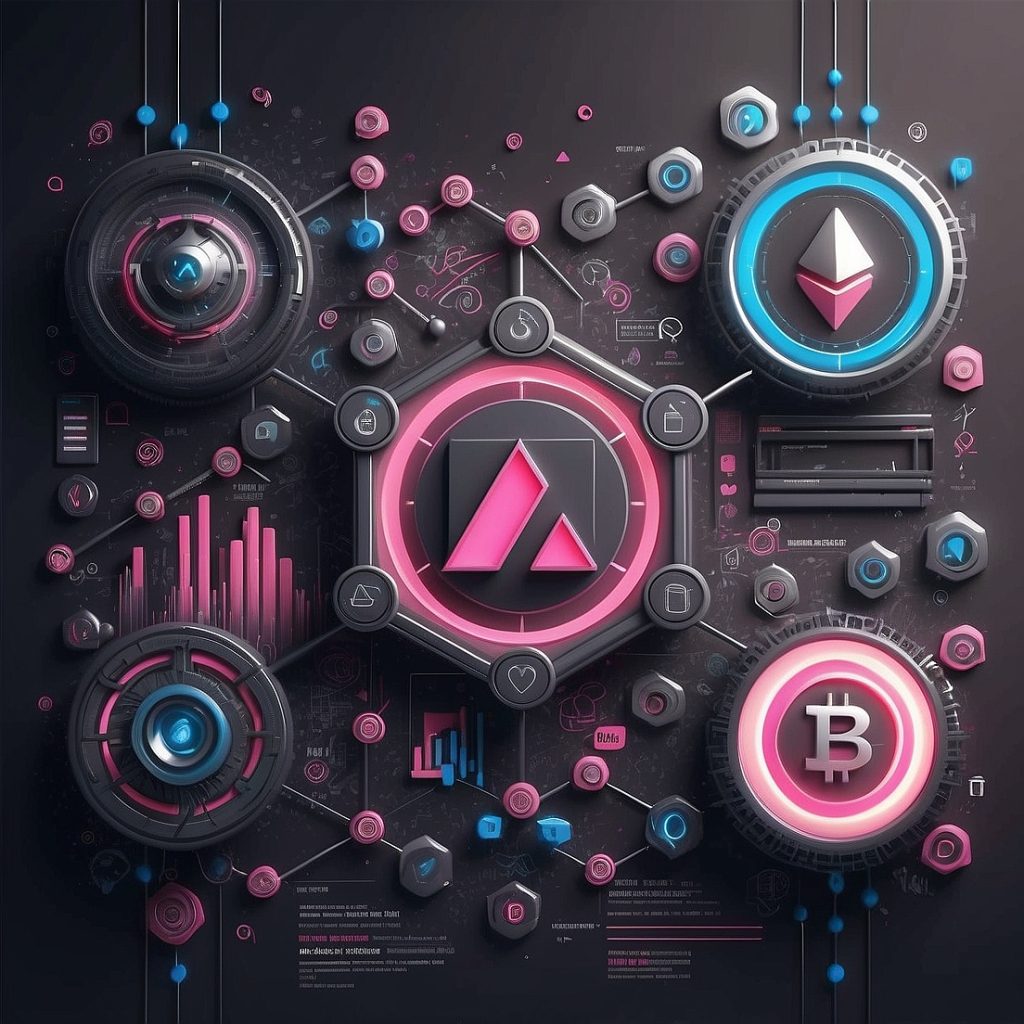
Avalanche vs. Byzantine Generals: Understanding the Problem the Consensus Solves
When we dive into the fascinating world of blockchain and distributed systems, two intriguing terms often surface: Avalanche and Byzantine Generals. These concepts are pivotal in understanding how consensus mechanisms ensure security, trust, and reliability in decentralized networks. In this blog, we’ll explore these ideas, break down the complex terminology, and see how they play a crucial role in the broader blockchain ecosystem.
What is the Byzantine Generals Problem?
The Byzantine Generals Problem is a fundamental issue in distributed systems, describing the difficulty of achieving consensus in the presence of faulty or malicious actors. Imagine a scenario where several Byzantine generals surround a city and must agree on a common plan of action. Some generals might be traitors, sending conflicting messages to create confusion and prevent a coordinated attack.
Origins and Importance
- The term originates from a 1982 paper by Leslie Lamport, Robert Shostak, and Marshall Pease.
- It’s crucial because it illustrates the challenges of achieving consensus in distributed networks, where not all participants can be trusted.
Core Concept
- The generals can only communicate through messengers.
- To avoid failure, they must reach a unanimous agreement.
- The presence of traitors (faulty nodes) complicates this process, making it hard to achieve consensus.
The Solution: Byzantine Fault Tolerance (BFT)
To tackle the Byzantine Generals Problem, the concept of Byzantine Fault Tolerance was introduced. BFT mechanisms ensure that a distributed system can function correctly and reach consensus even when some nodes fail or act maliciously.
Key Characteristics
- Resilience: Can tolerate a certain number of faulty nodes.
- Redundancy: Multiple nodes perform the same task to ensure accuracy.
- Majority Agreement: Consensus is reached through majority voting.
Example: Practical Byzantine Fault Tolerance (PBFT)
- PBFT is a widely used BFT algorithm.
- It operates efficiently with fewer than one-third of the nodes being faulty.
def pbft_consensus(nodes, messages):
honest_nodes = [node for node in nodes if node.is_honest()]
if len(honest_nodes) > (2/3) * len(nodes):
return True
return False
Enter Avalanche: A Modern Consensus Mechanism
Avalanche is a consensus protocol that addresses some limitations of traditional BFT algorithms. It is designed to be scalable, robust, and energy-efficient, making it suitable for modern blockchain applications.
Key Innovations
- Metastability: Uses a metastable mechanism to achieve consensus quickly.
- Sub-sampling: Each node queries a small, random subset of nodes, significantly reducing communication overhead.
- Acyclic Graph (DAG): Avalanche operates on a Directed Acyclic Graph, ensuring parallel processing of transactions.
How Avalanche Works
- Transaction Propagation: A node proposes a transaction and broadcasts it to the network.
- Query Sub-sampling: Each node randomly selects a subset of nodes and queries them about the transaction.
- Gossip Protocol: Nodes gossip the information to others, propagating the consensus decision.
- Finalization: Once a transaction receives a majority of affirmative responses, it is considered finalized.
Sample Code: Avalanche Query
def avalanche_query(node, transaction, network):
responses = []
sample_nodes = random.sample(network, k=subsample_size)
for n in sample_nodes:
responses.append(n.query(transaction))
if responses.count(True) > majority_threshold:
return True
return False
Comparing Avalanche and Byzantine Fault Tolerance
Understanding the differences between Avalanche and traditional BFT mechanisms helps us appreciate the innovation and applicability of each approach.
Scalability
- BFT: Limited scalability due to high communication overhead.
- Avalanche: Highly scalable with sub-sampling and DAG structure.
Energy Efficiency
- BFT: Often requires significant computational resources.
- Avalanche: More energy-efficient due to streamlined consensus process.
Fault Tolerance
- BFT: Robust against a predetermined number of faulty nodes.
- Avalanche: Provides high fault tolerance with a probabilistic approach.
Example Scenario
Consider a blockchain network with 1000 nodes. In a BFT system, reaching consensus might involve extensive communication among all nodes, leading to delays and inefficiencies. In contrast, Avalanche’s sub-sampling approach reduces this communication overhead, allowing faster and more efficient consensus.
Practical Applications and Use Cases
Blockchain Networks
- Avalanche: Used by the Avalanche blockchain for DeFi, asset issuance, and more.
- BFT: Utilized in networks like Hyperledger Fabric and Tendermint.
Distributed Databases
- Ensuring data consistency and reliability in distributed databases often relies on BFT mechanisms.
Secure Communications
- Byzantine fault tolerance principles are employed in secure communication systems to ensure message integrity.
Implementing Consensus Mechanisms: A Step-by-Step Guide
Let’s walk through a simplified implementation of both BFT and Avalanche consensus mechanisms.
Byzantine Fault Tolerance Implementation
- Node Initialization: Create nodes with predefined roles (honest or faulty).
- Message Broadcasting: Nodes broadcast their messages to others.
- Majority Voting: Nodes vote on the received messages.
- Consensus Decision: If the majority agrees, consensus is reached.
Sample Code: BFT Implementation
class Node:
def __init__(self, is_honest):
self.is_honest = is_honest
def broadcast(self, message, network):
for node in network:
node.receive(message)
def receive(self, message):
# Handle received message
pass
def bft_consensus(network):
messages = []
for node in network:
node.broadcast("message", network)
# Majority voting logic
return True # Simplified consensus decision
Avalanche Implementation
- Transaction Proposal: A node proposes a transaction.
- Query Sub-sampling: Node queries a random subset of nodes.
- Gossip Protocol: Nodes propagate the query results.
- Transaction Finalization: Once a transaction receives sufficient affirmative responses, it is finalized.
Sample Code: Avalanche Implementation
class AvalancheNode:
def __init__(self, network):
self.network = network
def propose_transaction(self, transaction):
responses = self.query_subsample(transaction)
if responses.count(True) > majority_threshold:
self.finalize_transaction(transaction)
def query_subsample(self, transaction):
sample_nodes = random.sample(self.network, k=subsample_size)
responses = [node.query(transaction) for node in sample_nodes]
return responses
def query(self, transaction):
# Return True/False based on transaction validity
return True
def finalize_transaction(self, transaction):
# Finalize the transaction
pass
Conclusion
In the ever-evolving landscape of blockchain and distributed systems, understanding the Byzantine Generals Problem and the innovations brought by consensus mechanisms like Avalanche is crucial. These mechanisms ensure the reliability, security, and efficiency of decentralized networks, paving the way for a future where trustless systems can flourish.
Disclaimer: The information provided in this blog is for educational purposes only and should not be considered as financial or investment advice. The examples and code snippets are simplified representations intended to illustrate the concepts discussed. Always conduct your own research and consult with professionals before making any decisions related to blockchain technologies and investments.