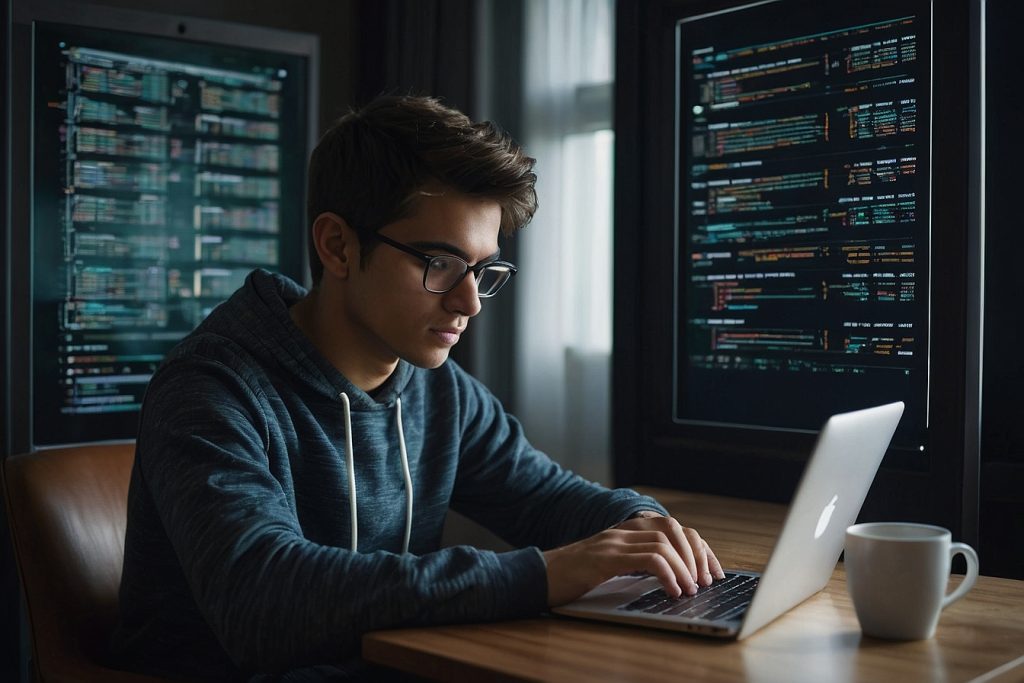
Batch File Magic: Effortlessly Create Sequential Folders
In the world of computing, automation is key to streamlining repetitive tasks and boosting productivity. One such task that can quickly become tedious is creating multiple folders with sequential naming conventions. Fear not, for we have a nifty batch file script that will handle this task with ease, saving you precious time and effort.
@echo off
setlocal enabledelayedexpansion
set "name=Backup"
set "start=1"
set "end=10"
for /l %%i in (!start!, 1, !end!) do (
set "folder_name=!name!%%i"
if not exist "!folder_name!" (
mkdir "!folder_name!"
echo Created folder: !folder_name!
) else (
echo Folder !folder_name! already exists.
)
)
endlocal
This batch file script is designed to create a series of folders with a prefix followed by a sequential number. In the provided example, it will create 10 folders named “Backup1” through “Backup10”. However, you can easily modify the script to suit your specific needs by changing the values of the name
, start
, and end
variables.
Here’s a breakdown of what the script does:
- The script starts by suppressing the echoing of commands to the console with
@echo off
. setlocal enabledelayedexpansion
enables delayed expansion of environment variables, which is necessary for the loop to work correctly.- The
name
,start
, andend
variables are set to the desired values. - The
for
loop iterates from thestart
value to theend
value, incrementing by 1 with each iteration. - Inside the loop, the
folder_name
variable is constructed by concatenating thename
value with the current loop counter. - An
if
statement checks if the folder with the constructed name already exists. If it doesn’t, themkdir
command creates the folder, and a message is printed to the console confirming the creation. - If the folder already exists, a message is printed to the console indicating that the folder already exists.
- Finally,
endlocal
restores the previous environment variable settings.
This script can be incredibly useful in various scenarios, such as creating backup folders for different dates or versions, organizing files based on categories or projects, or any situation where you need to create a series of folders with a consistent naming pattern.
Now, let’s take a look at the Linux/Mac and Python versions of the script:
Linux/Mac Bash Script
#!/bin/bash
name="Backup"
start=1
end=10
for ((i=$start; i<=$end; i++))
do
folder_name="$name$i"
if [ ! -d "$folder_name" ]; then
mkdir "$folder_name"
echo "Created folder: $folder_name"
else
echo "Folder $folder_name already exists."
fi
done
Python Script
import os
name = "Backup"
start = 1
end = 10
for i in range(start, end + 1):
folder_name = f"{name}{i}"
if not os.path.exists(folder_name):
os.makedirs(folder_name)
print(f"Created folder: {folder_name}")
else:
print(f"Folder {folder_name} already exists.")
These versions follow the same logic as the batch file script but adapt the syntax to the respective languages and platforms.
Whether you’re a Windows user relying on batch files or a Linux/Mac user working with Bash scripts, or a Python developer, this simple yet powerful script can save you valuable time and effort when dealing with the creation of sequential folders. Give it a try and let the automation do the work for you!