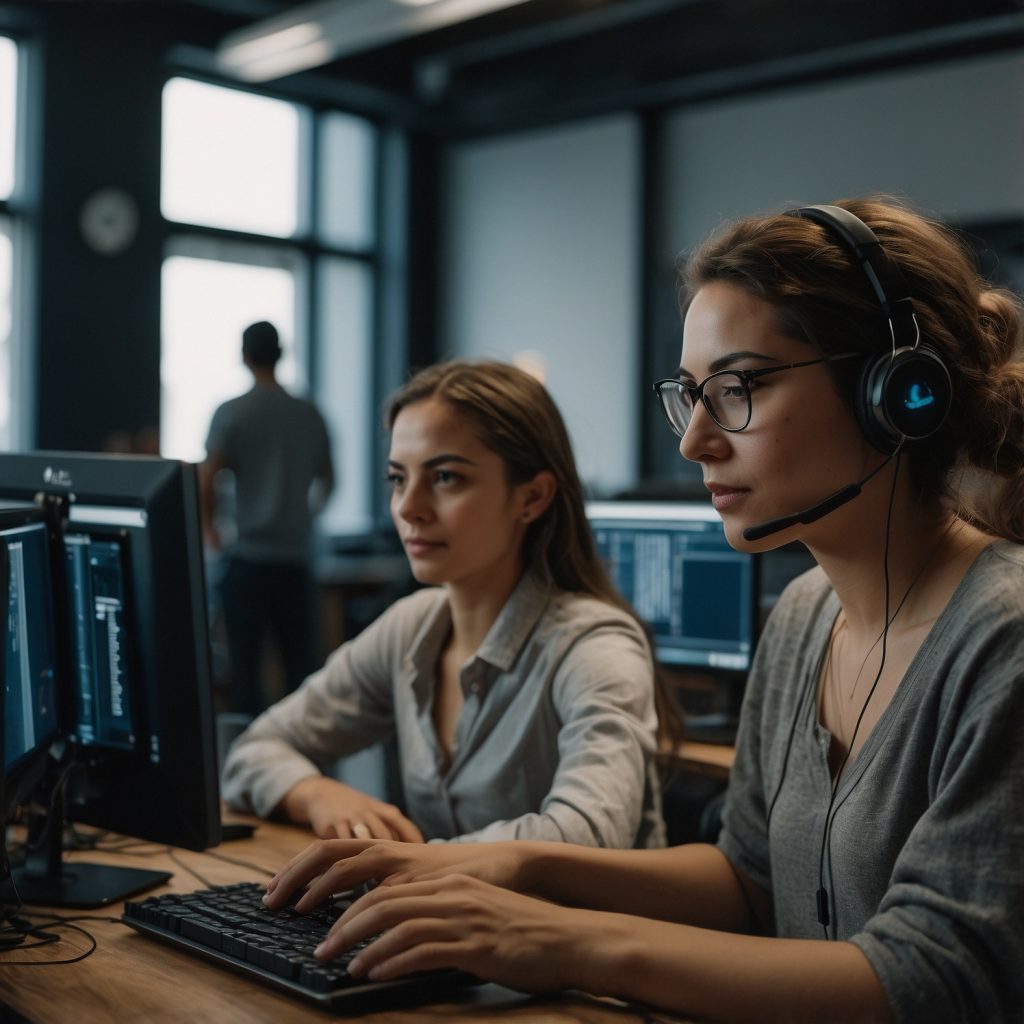
Best Practices for Code Review
Code review is a critical process in software development, ensuring that code quality is maintained and that bugs are caught before they reach production. Effective code reviews can lead to better code, improved team collaboration, and increased knowledge sharing. In this blog, we will discuss the best practices for conducting code reviews, with a focus on making the process efficient, thorough, and collaborative. We will also provide plenty of sample codes and scripts to illustrate these practices.
The Importance of Code Reviews
Why Code Reviews Matter
Code reviews are essential for maintaining high standards of code quality. They help identify bugs, enforce coding standards, and ensure that the code is maintainable and understandable by the entire team. Moreover, code reviews foster a collaborative environment where developers can learn from each other and share best practices.
Benefits of Code Reviews
- Improved Code Quality: Regular reviews help catch bugs and improve the overall quality of the codebase.
- Knowledge Sharing: Reviews are a great way to share knowledge among team members, helping junior developers learn from more experienced colleagues.
- Consistency: Enforcing coding standards and best practices ensures that the codebase remains consistent and easy to maintain.
- Reduced Risk: Early detection of issues can prevent costly problems in production.
Setting Up the Code Review Process
Define Clear Goals and Guidelines
Before starting the code review process, it’s crucial to have clear goals and guidelines. These should outline the objectives of the code review, the responsibilities of the reviewers, and the standards that the code should meet.
Example Guidelines:
1. Ensure that the code adheres to the project's coding standards.
2. Check for potential bugs and logical errors.
3. Review the code for readability and maintainability.
4. Provide constructive feedback.
5. Ensure that the code has adequate test coverage.
Choosing the Right Tools
Using the right tools can make the code review process more efficient. Popular tools include GitHub, GitLab, Bitbucket, and Phabricator. These tools provide features like inline comments, automated checks, and integration with CI/CD pipelines.
Conducting Effective Code Reviews
Focus on the Code, Not the Author
It’s important to remember that code reviews should be about the code, not the person who wrote it. Avoid personal criticisms and focus on providing constructive feedback.
Constructive Feedback Example:
Instead of saying, "This function is poorly written," say, "Consider refactoring this function to improve readability."
Review Code in Small Chunks
Reviewing large pull requests can be overwhelming and less effective. Encourage developers to submit smaller, more manageable chunks of code. This makes it easier to identify issues and provide thorough feedback.
Code Snippet for a Small Pull Request:
def add(a, b):
"""Adds two numbers and returns the result."""
return a + b
def subtract(a, b):
"""Subtracts the second number from the first and returns the result."""
return a - b
Prioritize Critical Issues
Not all issues are created equal. Prioritize critical issues that can lead to bugs or significantly impact the code’s functionality over minor stylistic concerns. Use a tagging system to categorize the severity of issues.
Example Tagging System:
1. Critical: Bugs or security vulnerabilities that must be fixed before merging.
2. Major: Significant issues that should be addressed but are not blockers.
3. Minor: Stylistic concerns or minor improvements.
Encouraging Collaboration and Learning
Create a Positive Review Culture
Foster a positive review culture by encouraging respectful and constructive communication. Celebrate good practices and well-written code to motivate developers.
Example Positive Feedback:
"Great job on this function! It's well-documented and easy to understand."
Pair Programming and Peer Reviews
Pair programming and peer reviews can be highly effective for knowledge sharing and collaborative problem-solving. This practice allows developers to work together in real-time, making the review process more interactive and engaging.
Pair Programming Example:
def calculate_area(length, width):
"""Calculates the area of a rectangle."""
return length * width
# Reviewer and developer discussing an improvement
# Developer: "What if we add a check for negative values?"
# Reviewer: "Good idea! We should ensure length and width are positive."
def calculate_area(length, width):
"""Calculates the area of a rectangle, ensuring positive dimensions."""
if length <= 0 or width <= 0:
raise ValueError("Length and width must be positive values.")
return length * width
Leveraging Automation in Code Reviews
Automate Repetitive Checks
Automating repetitive checks can save time and reduce the burden on reviewers. Tools like linters, static code analyzers, and CI/CD pipelines can automatically check for coding standards, syntax errors, and other common issues.
Example CI/CD Pipeline Script:
stages:
- lint
- test
lint:
stage: lint
script:
- flake8 . # Python linter for checking coding standards
test:
stage: test
script:
- pytest # Running unit tests
Continuous Integration and Deployment
Integrating continuous integration (CI) and continuous deployment (CD) systems ensures that code is automatically tested and deployed, making it easier to catch issues early and maintain a high-quality codebase.
CI/CD Integration Example:
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
pytest
Continuous Improvement and Feedback Loop
Regularly Review the Review Process
The code review process itself should be reviewed and improved regularly. Gather feedback from the team on what is working well and what can be improved.
Feedback Example:
"We've noticed that reviews are taking longer than expected. Can we streamline the process by focusing on the most critical issues first?"
Training and Mentorship
Provide training and mentorship to help team members improve their code review skills. This can include workshops, code review guidelines, and pairing junior developers with senior reviewers.
Training Session Outline:
1. Introduction to Code Reviews
2. Importance of Code Reviews
3. Code Review Best Practices
4. Hands-On Review Session
5. Q&A and Discussion
Ensuring Thorough Reviews
Check for Understanding and Maintainability
Ensure that the code is understandable and maintainable by others. If the code is complex, suggest adding comments or documentation to clarify its purpose and functionality.
Example of Adding Comments for Clarity:
def calculate_total_price(items):
"""
Calculates the total price of items in a cart.
Args:
items (list): List of tuples, where each tuple contains item price and quantity.
Returns:
float: Total price of items.
"""
total = 0.0
for price, quantity in items:
total += price * quantity # Calculate the total price for each item
return total
Ensure Adequate Test Coverage
Verify that the code has adequate test coverage. This includes unit tests, integration tests, and any other relevant tests to ensure that the code works as expected.
Example Unit Test:
import unittest
class TestCalculateTotalPrice(unittest.TestCase):
def test_calculate_total_price(self):
items = [(10, 2), (5, 3)]
result = calculate_total_price(items)
self.assertEqual(result, 35)
if __name__ == '__main__':
unittest.main()
Effective code reviews are crucial for maintaining high standards of code quality, fostering collaboration, and ensuring the long-term success of a project. By following best practices such as defining clear guidelines, focusing on constructive feedback, encouraging collaboration, leveraging automation, and continuously improving the process, teams can make code reviews an integral part of their development workflow. Remember, the goal of code reviews is not just to find bugs but to build a culture of quality, learning, and continuous improvement.