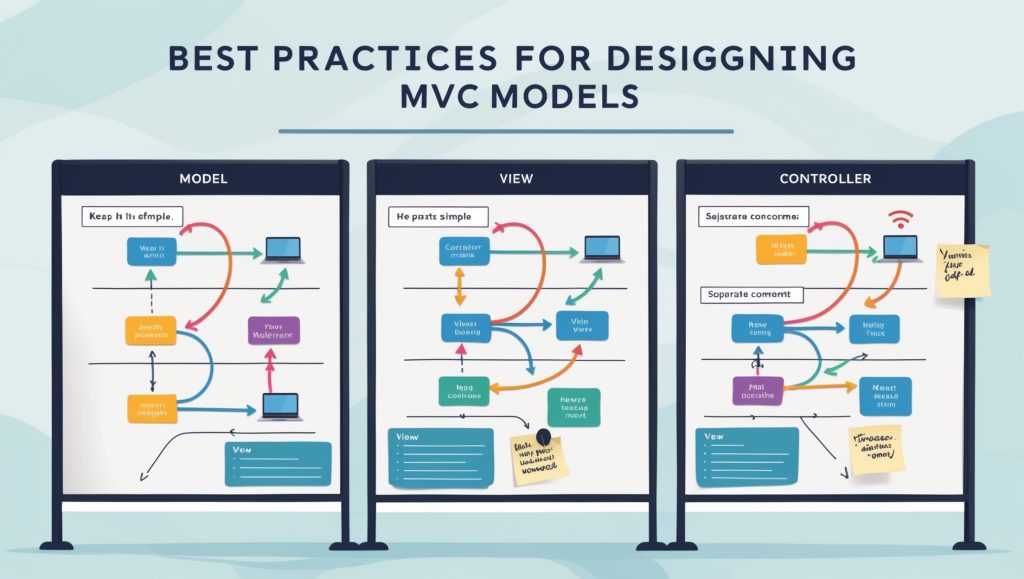
Best Practices for Designing MVC Models
The Model-View-Controller (MVC) architectural pattern has become a cornerstone of modern software development, providing a robust framework for creating maintainable and scalable applications. At the heart of this pattern lies the Model component, which serves as the backbone of your application’s business logic and data management. A well-designed model layer can significantly impact your application’s maintainability, scalability, and overall performance. This comprehensive guide explores the best practices for designing MVC models, offering practical insights and concrete examples to help developers create more efficient and maintainable applications. Whether you’re building a small web application or a large-scale enterprise system, these principles will help you establish a solid foundation for your software architecture.
Understanding the Role of Models in MVC
The Model component in MVC architecture represents the application’s data structure and business logic. It acts as a bridge between your database and the application’s business rules, ensuring data integrity and enforcing business constraints. A well-structured model should handle data validation, business rules implementation, and data manipulation operations while remaining independent of the user interface and control flow logic. Models should be self-contained, reusable, and maintainable, following the principle of separation of concerns. They should also be designed to work seamlessly with various data storage solutions, from traditional relational databases to modern NoSQL systems, ensuring flexibility and adaptability as your application evolves.
Core Principles for Model Design
Single Responsibility Principle (SRP)
Each model class should have a single, well-defined responsibility within your application. This fundamental principle helps maintain code clarity and reduces the complexity of your codebase. Consider the following example in Python:
# Bad Example - Multiple responsibilities
class User:
def __init__(self):
self.db_connection = Database()
def validate_email(self, email):
# Email validation logic
pass
def save_to_database(self):
# Database operations
pass
def generate_report(self):
# Report generation logic
pass
# Good Example - Separated responsibilities
class User:
def __init__(self, email, username):
self.email = email
self.username = username
def validate(self):
return self.validate_email() and self.validate_username()
def validate_email(self):
# Email validation logic
pass
def validate_username(self):
# Username validation logic
pass
class UserRepository:
def __init__(self):
self.db_connection = Database()
def save(self, user):
# Database operations
pass
def find_by_email(self, email):
# Database query logic
pass
class UserReportGenerator:
def generate_report(self, user):
# Report generation logic
pass
Encapsulation and Data Hiding
Models should protect their internal state and implementation details while providing a clean, well-defined interface for interacting with their data. Here’s an example in Java:
public class Product {
private Long id;
private String name;
private BigDecimal price;
private int stockQuantity;
// Constructor
public Product(String name, BigDecimal price, int stockQuantity) {
this.name = name;
this.price = price;
this.stockQuantity = stockQuantity;
}
// Public methods with business logic
public boolean isInStock() {
return stockQuantity > 0;
}
public void decreaseStock(int quantity) {
if (quantity > stockQuantity) {
throw new IllegalArgumentException("Insufficient stock");
}
stockQuantity -= quantity;
}
public void increaseStock(int quantity) {
if (quantity <= 0) {
throw new IllegalArgumentException("Quantity must be positive");
}
stockQuantity += quantity;
}
// Getters and setters with validation
public void setPrice(BigDecimal price) {
if (price.compareTo(BigDecimal.ZERO) <= 0) {
throw new IllegalArgumentException("Price must be positive");
}
this.price = price;
}
// Other getters and setters
}
Implementing Data Validation and Business Rules
Input Validation
Models should implement comprehensive input validation to ensure data integrity. This includes both simple type checking and complex business rule validation. Here’s an example combining both Python and Java approaches:
# Python Example
class Order:
def __init__(self, customer_id, items, shipping_address):
self.customer_id = customer_id
self.items = items
self.shipping_address = shipping_address
self.status = "pending"
self.created_at = datetime.now()
def validate(self):
errors = []
if not self.customer_id:
errors.append("Customer ID is required")
if not self.items:
errors.append("Order must contain at least one item")
if not self.shipping_address:
errors.append("Shipping address is required")
for item in self.items:
if not item.validate():
errors.append(f"Invalid item: {item.id}")
return len(errors) == 0, errors
def calculate_total(self):
return sum(item.price * item.quantity for item in self.items)
// Java Example
public class Order {
private final Long customerId;
private final List<OrderItem> items;
private final Address shippingAddress;
private OrderStatus status;
private LocalDateTime createdAt;
public Order(Long customerId, List<OrderItem> items, Address shippingAddress) {
this.customerId = customerId;
this.items = new ArrayList<>(items);
this.shippingAddress = shippingAddress;
this.status = OrderStatus.PENDING;
this.createdAt = LocalDateTime.now();
}
public ValidationResult validate() {
ValidationResult result = new ValidationResult();
if (customerId == null) {
result.addError("Customer ID is required");
}
if (items == null || items.isEmpty()) {
result.addError("Order must contain at least one item");
}
if (shippingAddress == null) {
result.addError("Shipping address is required");
}
items.forEach(item -> {
ValidationResult itemResult = item.validate();
if (!itemResult.isValid()) {
result.addErrors(itemResult.getErrors());
}
});
return result;
}
public BigDecimal calculateTotal() {
return items.stream()
.map(item -> item.getPrice().multiply(new BigDecimal(item.getQuantity())))
.reduce(BigDecimal.ZERO, BigDecimal::add);
}
}
Database Integration and Data Access Patterns
Repository Pattern Implementation
The Repository pattern provides a clean separation between the data access logic and business logic. Here’s an implementation example:
# Python Example using SQLAlchemy
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
class UserRepository:
def __init__(self):
self.engine = create_engine('postgresql://localhost/dbname')
self.Session = sessionmaker(bind=self.engine)
def find_by_id(self, user_id):
session = self.Session()
try:
return session.query(User).filter(User.id == user_id).first()
finally:
session.close()
def save(self, user):
session = self.Session()
try:
session.add(user)
session.commit()
return user
except:
session.rollback()
raise
finally:
session.close()
def update(self, user):
session = self.Session()
try:
session.merge(user)
session.commit()
return user
except:
session.rollback()
raise
finally:
session.close()
// Java Example using Spring Data JPA
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByEmail(String email);
List<User> findByLastName(String lastName);
@Query("SELECT u FROM User u WHERE u.status = :status")
List<User> findByStatus(@Param("status") UserStatus status);
@Modifying
@Query("UPDATE User u SET u.status = :status WHERE u.id = :id")
int updateUserStatus(@Param("id") Long id, @Param("status") UserStatus status);
}
Model Relationships and Associations
Implementing Model Relationships
Proper handling of model relationships is crucial for maintaining data integrity and implementing business logic effectively. Here’s how to implement various types of relationships:
Relationship Type | Description | Implementation Approach |
---|---|---|
One-to-One | Each record in the first table has exactly one matching record in the second table | Use foreign key with unique constraint |
One-to-Many | A record in one table can have multiple matching records in another table | Use foreign key in the “many” table |
Many-to-Many | Multiple records in one table can match multiple records in another table | Use junction/pivot table |
Example implementation in both Python and Java:
# Python Example using SQLAlchemy
from sqlalchemy import Column, Integer, String, ForeignKey
from sqlalchemy.orm import relationship
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String, unique=True)
# One-to-One relationship
profile = relationship("UserProfile", uselist=False, back_populates="user")
# One-to-Many relationship
orders = relationship("Order", back_populates="user")
# Many-to-Many relationship
roles = relationship("Role", secondary="user_roles", back_populates="users")
class UserProfile(Base):
__tablename__ = 'user_profiles'
id = Column(Integer, primary_key=True)
user_id = Column(Integer, ForeignKey('users.id'), unique=True)
address = Column(String)
phone = Column(String)
user = relationship("User", back_populates="profile")
// Java Example using JPA
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// One-to-One relationship
@OneToOne(mappedBy = "user", cascade = CascadeType.ALL)
private UserProfile profile;
// One-to-Many relationship
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL)
private List<Order> orders = new ArrayList<>();
// Many-to-Many relationship
@ManyToMany
@JoinTable(
name = "user_roles",
joinColumns = @JoinColumn(name = "user_id"),
inverseJoinColumns = @JoinColumn(name = "role_id")
)
private Set<Role> roles = new HashSet<>();
}
Error Handling and Exception Management
Implementing Custom Exceptions
Create domain-specific exceptions to handle business logic errors effectively:
# Python Example
class ModelException(Exception):
pass
class ValidationException(ModelException):
def __init__(self, errors):
self.errors = errors
super().__init__(str(errors))
class BusinessRuleException(ModelException):
pass
class User:
def save(self):
if not self.is_valid():
raise ValidationException(self.validation_errors)
if not self.can_be_saved():
raise BusinessRuleException("User cannot be saved due to business rules")
// Java Example
public class ModelException extends RuntimeException {
public ModelException(String message) {
super(message);
}
}
public class ValidationException extends ModelException {
private final List<String> errors;
public ValidationException(List<String> errors) {
super("Validation failed: " + String.join(", ", errors));
this.errors = errors;
}
public List<String> getErrors() {
return new ArrayList<>(errors);
}
}
Testing Models
Unit Testing Best Practices
Implement comprehensive unit tests for your models:
# Python Example using pytest
import pytest
def test_user_validation():
user = User(email="invalid-email")
valid, errors = user.validate()
assert not valid
assert "Invalid email format" in errors
def test_order_calculation():
items = [
OrderItem(price=10.0, quantity=2),
OrderItem(price=15.0, quantity=1)
]
order = Order(customer_id=1, items=items)
assert order.calculate_total() == 35.0
@pytest.mark.parametrize("price,quantity,expected", [
(10.0, 2, 20.0),
(15.0, 3, 45.0),
(0.0, 1, 0.0)
])
def test_order_item_total(price, quantity, expected):
item = OrderItem(price=price, quantity=quantity)
assert item.calculate_total() == expected
// Java Example using JUnit
@Test
void testUserValidation() {
User user = new User("invalid-email");
ValidationResult result = user.validate();
assertFalse(result.isValid());
assertTrue(result.getErrors().contains("Invalid email format"));
}
@Test
void testOrderCalculation() {
List<OrderItem> items = Arrays.asList(
new OrderItem(new BigDecimal("10.00"), 2),
new OrderItem(new BigDecimal("15.00"), 1)
);
Order order = new Order(1L, items);
assertEquals(new BigDecimal("35.00"), order.calculateTotal());
}
@ParameterizedTest
@CsvSource({
"10.00, 2, 20.00",
"15.00, 3, 45.00",
"0.00, 1, 0.00"
})
void testOrderItemTotal(BigDecimal price, int quantity, BigDecimal expected) {
OrderItem item = new OrderItem(price, quantity);
assertEquals(expected, item.calculateTotal());
}
Conclusion
Designing robust and well-structured MVC models requires careful consideration of various aspects, from basic principles like Single Responsibility and Encapsulation to more complex concerns like relationship management and error handling. By following these best practices and implementing appropriate patterns, you can create maintainable, scalable, and reliable applications that stand the test of time. Remember to regularly review and refactor your model layer as your application evolves, and always prioritize code clarity and maintainability over premature optimization.
Disclaimer: The code examples and best practices presented in this blog post are based on current industry standards and common practices as of 2024. While we strive for accuracy and completeness, software development practices evolve rapidly, and some recommendations may need to be adapted for specific use cases or newer technologies. If you notice any inaccuracies or have suggestions for improvements, please report them to our editorial team for prompt review and correction.