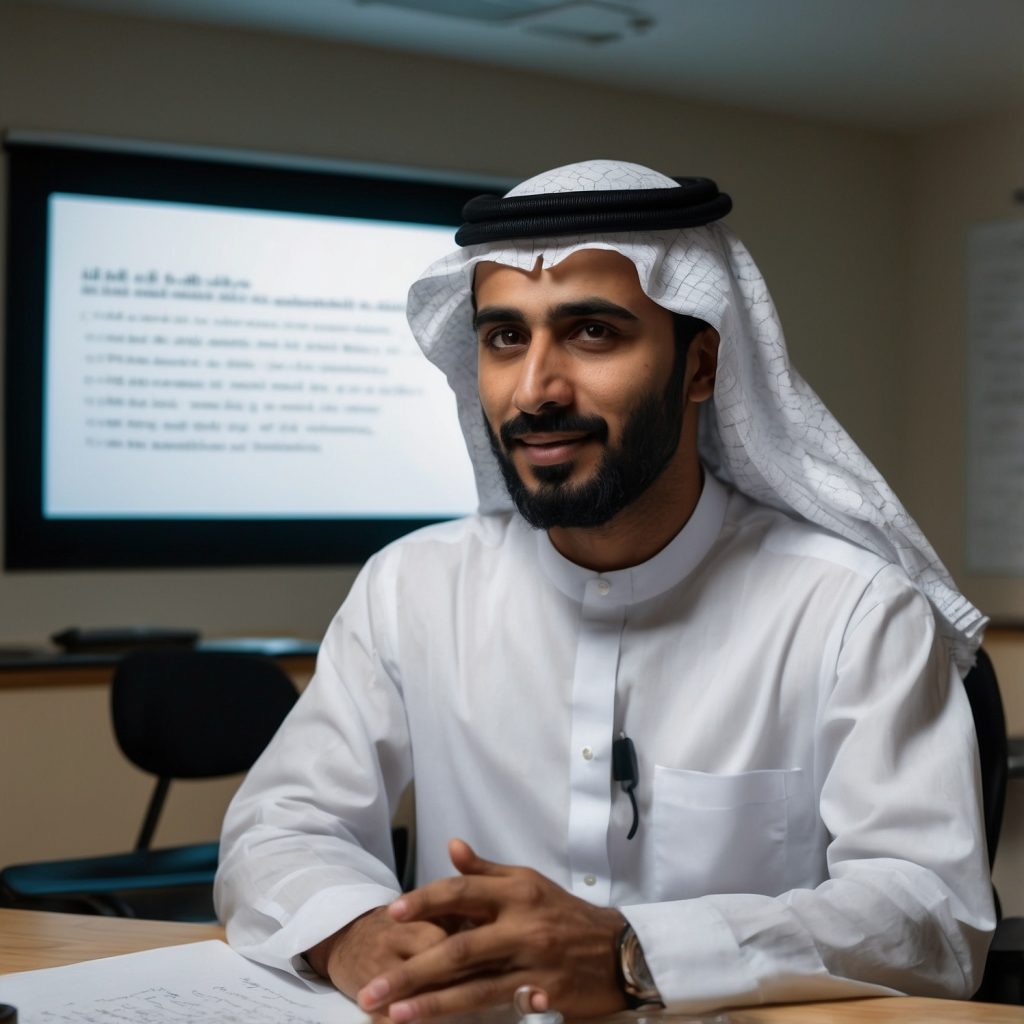
Best Practices for Writing Clean and Maintainable Code
Writing clean and maintainable code is essential for software development. It ensures that the codebase is easy to understand, extend, and maintain over time. Clean code not only improves the efficiency of development but also reduces the likelihood of bugs and errors. This blog will delve into the best practices for writing clean and maintainable code, covering various aspects from naming conventions to documentation, with plenty of sample codes and code snippets.
Importance of Clean Code
Improved Readability
Clean code is easier to read and understand. This is crucial because most developers spend more time reading code than writing it. Code that is easy to read reduces the time needed to understand what the code does, making it easier to maintain and extend. Consider the following example:
# Bad example
def a(b, c):
return b * c
# Good example
def calculate_area(width, height):
return width * height
In the bad example, it is unclear what a
stands for or what b
and c
represent. The good example uses descriptive names, making the purpose of the function clear.
Easier Debugging and Testing
When code is clean, it is easier to debug and test. Clean code follows a consistent structure and naming convention, making it straightforward to locate and fix bugs. It also facilitates writing unit tests, as well-organized code is more predictable and modular.
Enhances Collaboration
In a team environment, clean code enhances collaboration. It ensures that all team members can understand each other’s code, leading to better teamwork and faster development cycles. Adopting a uniform coding standard across the team helps in maintaining consistency.
Naming Conventions
Descriptive Names
Use descriptive names for variables, functions, and classes. Descriptive names convey the purpose of the element, making the code self-explanatory. For example:
# Bad example
x = 10
y = 20
z = x + y
# Good example
length = 10
width = 20
area = length + width
Consistent Naming Schemes
Adopt a consistent naming scheme throughout the codebase. Whether it’s camelCase, PascalCase, or snake_case, consistency helps in maintaining uniformity. For instance, in Python, the common convention is to use snake_case for variable and function names and PascalCase for class names:
# Function and variable names
def calculate_total_price(item_price, tax_rate):
total_price = item_price + (item_price * tax_rate)
return total_price
# Class names
class ShoppingCart:
def __init__(self):
self.items = []
Writing Modular Code
Single Responsibility Principle
Each function or class should have a single responsibility. This principle makes code more modular and easier to manage. For example:
# Bad example
class User:
def __init__(self, name, email):
self.name = name
self.email = email
def send_email(self, subject, body):
print(f"Sending email to {self.email}")
# email sending logic
# Good example
class User:
def __init__(self, name, email):
self.name = name
self.email = email
class EmailService:
def send_email(self, email, subject, body):
print(f"Sending email to {email}")
# email sending logic
Avoid Large Functions
Large functions are difficult to understand and maintain. Break down large functions into smaller, more manageable functions. This approach not only enhances readability but also makes the code easier to test.
# Bad example
def process_data(data):
# Step 1: Clean data
cleaned_data = [item.strip() for item in data]
# Step 2: Transform data
transformed_data = [item.upper() for item in cleaned_data]
# Step 3: Save data
with open('data.txt', 'w') as f:
for item in transformed_data:
f.write(f"{item}\n")
# Good example
def clean_data(data):
return [item.strip() for item in data]
def transform_data(data):
return [item.upper() for item in data]
def save_data(data, filename):
with open(filename, 'w') as f:
for item in data:
f.write(f"{item}\n")
def process_data(data):
cleaned_data = clean_data(data)
transformed_data = transform_data(cleaned_data)
save_data(transformed_data, 'data.txt')
Commenting and Documentation
Use Comments Sparingly
Comments should be used sparingly and only when necessary. The code itself should be self-explanatory as much as possible. Comments are useful for explaining why certain decisions were made or for complex logic that isn’t immediately clear from the code.
# Bad example
# This function calculates the square of a number
def square(n):
return n * n
# Good example
def square(n):
return n * n # Return the square of the number
Write Documentation
Documentation is crucial for understanding the overall design and functionality of the code. Use docstrings in functions and classes to describe their purpose and usage:
def calculate_total_price(item_price, tax_rate):
"""
Calculate the total price including tax.
Parameters:
item_price (float): The price of the item
tax_rate (float): The tax rate as a decimal
Returns:
float: The total price including tax
"""
return item_price + (item_price * tax_rate)
Consistent Formatting
Follow a Style Guide
Adhering to a style guide like PEP 8 for Python or Google’s JavaScript Style Guide ensures consistency in the code. These guides cover various aspects like indentation, spacing, and line length, promoting a uniform coding style.
Use Proper Indentation
Proper indentation is vital for readability. Consistent indentation helps in visually separating code blocks, making the code structure clear:
# Bad example
if x > 10:
print("X is greater than 10")
else:
print("X is 10 or less")
# Good example
if x > 10:
print("X is greater than 10")
else:
print("X is 10 or less")
Limit Line Length
Keep line length to a reasonable limit (typically 80-100 characters). Long lines can be hard to read and may not display well on all devices. Use line breaks appropriately to maintain readability:
# Bad example
def calculate_total_price(item_price, tax_rate): return item_price + (item_price * tax_rate)
# Good example
def calculate_total_price(item_price, tax_rate):
return item_price + (item_price * tax_rate)
Code Reviews and Refactoring
Regular Code Reviews
Conduct regular code reviews to ensure code quality. Code reviews help in identifying potential issues, improving code quality, and sharing knowledge among team members. During code reviews, focus on readability, correctness, and adherence to coding standards.
Refactor Regularly
Refactoring involves restructuring existing code without changing its external behavior. Regular refactoring helps in maintaining clean code by simplifying complex logic, improving performance, and removing redundancies:
# Before refactoring
def calculate_discount(price, discount):
if discount == 10:
return price * 0.9
elif discount == 20:
return price * 0.8
elif discount == 30:
return price * 0.7
else:
return price
# After refactoring
def calculate_discount(price, discount):
discount_rate = 1 - (discount / 100)
return price * discount_rate
Testing
Write Unit Tests
Unit tests are essential for verifying the functionality of individual components. Writing unit tests helps in catching bugs early and ensures that the code behaves as expected. Use testing frameworks like unittest or pytest for Python:
import unittest
def add(a, b):
return a + b
class TestMathFunctions(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
Test-Driven Development (TDD)
Test-Driven Development (TDD) involves writing tests before writing the actual code. This approach ensures that the code is written to pass the tests, leading to more robust and error-free code. The TDD cycle includes writing a test, writing code to pass the test, and refactoring:
# Step 1: Write a test
def test_calculate_area():
assert calculate_area(5, 10) == 50
# Step 2: Write the code
def calculate_area(width, height):
return width * height
# Step 3: Run the test and refactor if necessary
Avoiding Code Smells
Duplicate Code
Duplicate code is a common code smell that indicates the same code logic is repeated in multiple places. This not only increases the codebase size but also makes maintenance harder. Refactor duplicate code into a single function or module:
# Bad example
def print_user_info(user):
print(f"Name: {user.name}")
print(f"Email: {user.email}")
def print_admin_info(admin):
print(f"Name: {admin.name}")
print(f"Email: {admin.email}")
# Good example
def print_info(person):
print(f"Name: {person.name}")
print(f"Email: {person.email}")
Long Parameter Lists
Long parameter lists can make functions difficult to understand and use. Instead, use objects or dictionaries to group related parameters, reducing the complexity of the function signature:
# Bad example
def create_user(name, age, email, address, phone, birthday):
# function logic
# Good example
class User:
def __init__(self, name, age, email, address, phone, birthday):
self.name = name
self.age = age
self.email = email
self.address = address
self.phone = phone
self.birthday = birthday
def create_user(user):
# function logic using user object
Complex Conditionals
Complex conditional statements can make code hard to read and maintain. Use guard clauses, helper functions, or simplify conditions to improve readability:
# Bad example
def determine_discount(price, membership_level):
if membership_level == 'gold':
if price > 100:
return price * 0.8
else:
return price * 0.9
elif membership_level == 'silver':
if price > 100:
return price * 0.85
else:
return price * 0.95
else:
return price
# Good example
def determine_discount(price, membership_level):
if membership_level == 'gold':
return price * 0.8 if price > 100 else price * 0.9
elif membership_level == 'silver':
return price * 0.85 if price > 100 else price * 0.95
return price
Handling Errors and Exceptions
Use Exceptions Appropriately
Use exceptions to handle errors and exceptional cases in your code. Avoid using exceptions for control flow; instead, use them for truly exceptional conditions. Always provide informative error messages:
# Bad example
def divide(a, b):
try:
return a / b
except:
return None
# Good example
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
raise ValueError("Division by zero is not allowed")
Graceful Degradation
Ensure your application can handle errors gracefully without crashing. Provide meaningful feedback to the user and log errors for debugging purposes. This approach improves the robustness and user experience of your application:
# Example of logging errors
import logging
def divide(a, b):
try:
return a / b
except ZeroDivisionError as e:
logging.error(f"Error occurred: {e}")
raise ValueError("Division by zero is not allowed")
Code Optimization
Efficient Algorithms
Choose efficient algorithms to improve performance. Optimize code by using appropriate data structures and algorithms that best suit your use case. For example, use dictionaries for fast lookups instead of lists:
# Bad example (using list for lookups)
users = ["Alice", "Bob", "Charlie"]
def is_user_present(username):
return username in users
# Good example (using dictionary for lookups)
users = {"Alice": True, "Bob": True, "Charlie": True}
def is_user_present(username):
return username in users
Avoid Premature Optimization
Optimize code only when necessary. Premature optimization can lead to complex code that is hard to maintain. Focus on writing clear and correct code first, then profile and optimize critical sections if performance becomes an issue.
Version Control
Use Version Control Systems (VCS)
Adopt a version control system like Git to manage your codebase. Version control systems help in tracking changes, collaborating with team members, and maintaining a history of your code:
# Example Git commands
git init # Initialize a new repository
git add . # Stage all changes
git commit -m "Initial commit" # Commit changes with a message
git push origin main # Push changes to the remote repository
Meaningful Commit Messages
Write meaningful and descriptive commit messages. A good commit message explains what changes were made and why, helping team members understand the history of the project:
# Bad example
git commit -m "Fix bug"
# Good example
git commit -m "Fix issue with user login when password contains special characters"
Automated Builds and Continuous Integration
Automated Builds
Set up automated builds to ensure that your code compiles and passes tests whenever changes are made. Automated builds help in catching errors early and maintaining a stable codebase. Tools like Jenkins, Travis CI, or GitHub Actions can be used for this purpose:
# Example GitHub Actions workflow
name: CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.8'
- name: Install dependencies
run: pip install -r requirements.txt
- name: Run tests
run: pytest
Continuous Integration (CI)
Implement continuous integration to automatically test and merge code changes. CI helps in maintaining code quality and ensuring that new changes do not break existing functionality. Integrate CI tools into your workflow to streamline development:
# Example Travis CI configuration
language: python
python:
- "3.8"
install:
- pip install -r requirements.txt
script:
- pytest
Using Design Patterns
Common Design Patterns
Utilize common design patterns to solve recurring problems. Design patterns provide tested, proven development paradigms that improve code structure and maintainability. Examples include the Singleton, Factory, and Observer patterns:
# Example of Singleton pattern
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
Appropriate Usage
Apply design patterns appropriately. Not all patterns are suitable for every situation. Evaluate the problem at hand and choose the most suitable pattern to ensure the code remains clean and maintainable.
Writing clean and maintainable code is an essential skill for any developer. It not only improves the readability and usability of the code but also ensures long-term maintainability and ease of collaboration. By following best practices such as adopting consistent naming conventions, writing modular and well-documented code, and utilizing tools like version control and automated testing, you can create a robust codebase that is easy to understand, maintain, and extend. Remember that clean code is a continuous effort and requires regular refactoring and adherence to established standards and practices. By implementing these best practices, you can significantly improve the quality of your software and the efficiency of your development process.